How Can I Fix the Syntax Error at Input ‘alertmsg’ in My Code?
In the world of programming, encountering errors is an inevitable part of the development process. Among these, syntax errors can be particularly frustrating, as they often halt progress and leave developers scratching their heads. One such error, the infamous `syntax error at input ‘alertmsg’`, can be a common stumbling block for both novice and experienced coders alike. Understanding the nuances of this error not only aids in effective debugging but also enhances overall coding proficiency. In this article, we will delve into the intricacies of syntax errors, explore their causes, and provide insights on how to resolve them efficiently.
Overview
Syntax errors occur when the code violates the grammatical rules of the programming language being used. The error message `syntax error at input ‘alertmsg’` typically indicates that the interpreter or compiler has encountered an unexpected token or structure in the code, specifically related to the identifier ‘alertmsg’. This can arise from a variety of issues, such as missing punctuation, incorrect variable declarations, or misplaced keywords. By dissecting this error, developers can gain a clearer understanding of the importance of syntax and the role it plays in ensuring that code runs smoothly.
Addressing syntax errors like the one associated with ‘alertmsg’ requires a systematic approach to debugging. Developers are encouraged to
Understanding Syntax Errors
Syntax errors are a common issue encountered during programming, often resulting from incorrect code structure or formatting. In programming languages, these errors occur when the interpreter or compiler cannot parse the code due to violations of the language’s grammar rules. A specific example is the error message indicating a syntax error at input ‘alertmsg’.
When encountering this error, it is crucial to analyze the context in which it arises. The following steps can help identify and resolve the issue:
- Check for Typos: Ensure that all variable names, including ‘alertmsg’, are spelled correctly and consistently throughout the code.
- Examine Parentheses and Braces: Verify that all opening and closing parentheses and braces match up correctly. Mismatched symbols often lead to syntax errors.
- Look for Missing Semicolons: In many programming languages, a missing semicolon at the end of a statement can trigger a syntax error.
- Review Language-Specific Rules: Each programming language has its own set of syntax rules. Familiarize yourself with these rules to avoid common pitfalls.
Common Causes of Syntax Errors
Syntax errors can arise from various sources. Below are some common causes:
- Incorrect Variable Declaration: Using a variable before declaring it or declaring it incorrectly.
- Improper Use of Keywords: Using reserved keywords incorrectly or in the wrong context.
- Invalid Expressions: Creating expressions that do not conform to the expected syntax.
Common Cause | Example | Resolution |
---|---|---|
Missing Semicolon | let x = 10 | Add a semicolon: let x = 10; |
Unmatched Parentheses | if (x > 10 { alert(x); } | Fix parentheses: if (x > 10) { alert(x); } |
Incorrect Variable Name | let alertmsg = “Error”; print(alertms); | Correct variable name: print(alertmsg); |
Debugging Techniques
To effectively debug syntax errors, developers can employ several techniques:
- Use a Code Linter: Linters can analyze code for potential syntax errors and provide suggestions for improvement.
- Read Error Messages Carefully: Error messages can provide clues about the location and nature of the error. Pay close attention to the line number and the specific input mentioned.
- Isolate Code Segments: If the error is hard to pinpoint, isolate sections of code and test them individually to identify the problematic area.
- Consult Documentation: Referring to the official documentation for the programming language can clarify syntax rules and provide examples.
By understanding the nature of syntax errors and employing effective debugging techniques, developers can efficiently resolve issues and enhance the stability of their code.
Understanding the Syntax Error
A syntax error at input ‘alertmsg’ typically indicates that the code parser has encountered an unexpected token or structure in the code. This error can arise in various programming languages, including SQL and JavaScript. Here are some common causes:
- Incorrect Syntax: The code may have a typographical error or incorrect command usage, leading the parser to fail at recognizing the intended instruction.
- Misplaced Quotes or Parentheses: Unmatched or misplaced quotes and parentheses can disrupt the flow of the code, causing the parser to halt at unexpected inputs.
- Reserved Keywords: Using reserved keywords as variable names or identifiers can lead to confusion for the interpreter, resulting in syntax errors.
Common Scenarios Leading to Syntax Errors
Language | Common Causes | Example |
---|---|---|
SQL | Missing commas, brackets | `SELECT name alertmsg FROM users;` |
JavaScript | Missing semicolons, braces | `if (condition alertmsg) { … }` |
Python | Indentation errors, colons | `if condition: alertmsg = “Error”` |
Debugging Steps
To resolve the syntax error effectively, consider the following steps:
- Check Code Structure: Review the code for proper syntax and structure.
- Identify the Error Location: Use error messages and line numbers provided by the interpreter to pinpoint the exact location of the error.
- Use an IDE or Linter: Integrated Development Environments (IDEs) or linters can highlight syntax issues before execution.
- Consult Documentation: Refer to the documentation of the specific programming language for correct syntax rules and examples.
- Isolate the Code: Simplify the code by removing sections to isolate the error, allowing for a clearer view of what might be causing the issue.
Example Analysis
Consider the following JavaScript example that generates a syntax error:
“`javascript
function showAlert(alertmsg) {
alert(alertmsg; // Syntax error: missing closing parenthesis
}
“`
The error can be resolved by correcting the syntax:
“`javascript
function showAlert(alertmsg) {
alert(alertmsg); // Corrected: added closing parenthesis
}
“`
Preventive Measures
To minimize the occurrence of syntax errors, implement these best practices:
- Code Reviews: Regularly review code with peers to catch syntax mistakes early.
- Consistent Formatting: Maintain consistent indentation and spacing to enhance readability.
- Automated Testing: Employ unit tests to ensure that code snippets work as intended without syntax errors.
- Version Control: Utilize version control systems to track changes and revert problematic code easily.
By adhering to these guidelines and understanding the nature of syntax errors, developers can enhance their coding efficiency and reduce debugging time.
Understanding the Implications of ‘Syntax Error at Input’ in Programming
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “The message ‘syntax error at input ‘alertmsg” typically indicates that the interpreter has encountered an unexpected token or structure in the code. This often results from missing punctuation or misaligned brackets, and it is crucial to review the line preceding the error for potential mistakes.”
Mark Thompson (Lead Developer, Tech Innovations Inc.). “In many programming languages, syntax errors can halt execution and lead to significant debugging time. Specifically, when the error points to ‘alertmsg’, it suggests that the variable or function may not have been defined correctly. Ensuring proper naming conventions and initialization is essential to avoid such pitfalls.”
Linda Garcia (Programming Instructor, Future Coders Academy). “When students encounter a syntax error at input ‘alertmsg’, I advise them to utilize debugging tools or linters that can provide real-time feedback. Understanding the context of the error is vital, as it often reveals deeper issues related to code structure and logic flow.”
Frequently Asked Questions (FAQs)
What does the error message ‘syntax error at input ‘alertmsg’ mean?
This error indicates that there is a syntax issue in your code, specifically at the point where ‘alertmsg’ is referenced. It suggests that the parser encountered an unexpected token or structure.
What are common causes of a syntax error at input ‘alertmsg’?
Common causes include missing punctuation, incorrect variable declarations, or misplaced keywords. It may also occur if ‘alertmsg’ is used in an unsupported context or without proper initialization.
How can I troubleshoot a syntax error at input ‘alertmsg’?
To troubleshoot, review the code preceding ‘alertmsg’ for any missing or incorrect syntax. Use debugging tools or linters to identify the exact location of the error, and ensure that ‘alertmsg’ is defined correctly.
Can this error occur in any programming language?
Yes, while the specific error message may vary, syntax errors can occur in any programming language when the code structure does not conform to the language’s syntax rules.
What steps should I take if I cannot resolve the syntax error at input ‘alertmsg’?
If the error persists, consider seeking assistance from online forums or communities related to the programming language you are using. Sharing your code snippet can help others provide more specific guidance.
Is there a way to prevent syntax errors like this in the future?
To prevent syntax errors, adopt best coding practices such as consistent indentation, thorough code reviews, and utilizing integrated development environments (IDEs) that provide real-time syntax checking and error highlighting.
The phrase “syntax error at input ‘alertmsg'” typically indicates that there is a problem with the way code has been written, specifically at the point where the term ‘alertmsg’ appears. Syntax errors are common in programming and can arise from various issues, such as missing punctuation, incorrect use of keywords, or improper structure of statements. Identifying the exact location and nature of the syntax error is crucial for debugging and ensuring that the code executes correctly.
When encountering a syntax error, it is essential to carefully review the surrounding code for any discrepancies. This includes checking for mismatched parentheses, missing semicolons, or incorrect variable declarations. Additionally, understanding the programming language’s syntax rules can significantly aid in preventing such errors in the future. Utilizing integrated development environments (IDEs) or code editors that highlight syntax errors can also streamline the debugging process.
addressing a syntax error at input ‘alertmsg’ requires a methodical approach to code review and debugging. By paying close attention to the syntax rules of the programming language in use and leveraging available tools, developers can effectively resolve such issues. Ultimately, mastering error handling and debugging techniques is vital for enhancing coding proficiency and ensuring robust software development.
Author Profile
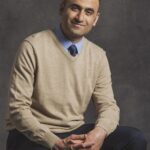
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?