Why Am I Getting a SyntaxError: Unexpected End of Input?
In the world of programming, encountering errors is an inevitable part of the development process. Among the myriad of error messages that can pop up during coding, the `SyntaxError: unexpected end of input` stands out as a particularly perplexing and frustrating issue. This error often leaves developers scratching their heads, wondering where their code went wrong. Whether you’re a seasoned programmer or just starting out, understanding this error is crucial for debugging and refining your coding skills. In this article, we will delve into the nuances of this syntax error, exploring its causes, implications, and strategies for resolution.
Overview
The `SyntaxError: unexpected end of input` typically arises when the interpreter reaches the end of a script or function but finds that the code is incomplete or improperly structured. This can happen for a variety of reasons, such as missing brackets, unclosed strings, or even misplaced comments. As a result, the interpreter is unable to parse the code correctly, leading to confusion and halted execution. Understanding the common culprits behind this error is essential for any developer aiming to write clean, functional code.
Moreover, this error serves as a reminder of the importance of meticulous coding practices. By adopting strategies such as code reviews, consistent formatting, and thorough testing, developers can minimize the
Understanding SyntaxError in JavaScript
Syntax errors in JavaScript, particularly the “unexpected end of input” error, commonly occur during the development process. This error suggests that the JavaScript interpreter has reached the end of the input unexpectedly, often due to missing or mismatched brackets, parentheses, or other delimiters. When the JavaScript engine parses the code, it expects a certain structure and will throw this error if it encounters an incomplete statement.
Common Causes of SyntaxError: Unexpected End of Input
Several scenarios can lead to this specific syntax error. Understanding these can help developers troubleshoot more effectively:
- Mismatched Brackets: Unmatched braces `{}`, brackets `[]`, or parentheses `()` can lead to this error. For example:
“`javascript
function example() {
console.log(“Hello, World!”;
}
“`
- Incomplete Statements: If a statement is left incomplete, such as missing a closing quote or not completing a function definition, the interpreter will throw an error.
“`javascript
let str = “Incomplete string;
“`
- Comments: Improperly closed comments can also lead to this error. For instance:
“`javascript
/* This is a comment
console.log(“Hello”);
“`
- Missing Return Statements: In functions that expect a return value, missing a return statement can lead to confusion in interpreting the code flow.
“`javascript
function sum(a, b) {
a + b; // No return statement
}
“`
Debugging the SyntaxError
To effectively debug and resolve the “unexpected end of input” error, consider the following strategies:
- Use a Code Linter: Employ tools such as ESLint or JSHint to analyze your code for potential syntax issues. These tools provide real-time feedback and can identify missing brackets or mismatched quotes.
- Careful Code Review: Perform a line-by-line review of your code, paying special attention to the opening and closing of all delimiters.
- Console Logging: Utilize console logs to track the flow of execution and identify where the code fails. This practice can help narrow down the scope of the issue.
- Integrated Development Environment (IDE) Features: Leverage features in modern IDEs, which often highlight syntax errors in real-time, helping you catch issues early in the development process.
Error Type | Description | Common Fixes |
---|---|---|
Mismatched Brackets | Braces or parentheses do not match | Add missing brackets or correct the mismatches |
Incomplete Statements | Statements are left unfinished | Ensure all statements are complete |
Improper Comments | Comments are not properly closed | Close all comments correctly |
Missing Returns | Functions that should return values do not | Add return statements where necessary |
By thoroughly understanding the root causes and implementing effective debugging strategies, developers can significantly reduce the occurrence of the “syntaxerror: unexpected end of input” and improve the overall quality of their JavaScript code.
Understanding the Error
The `SyntaxError: unexpected end of input` typically occurs in programming languages like JavaScript and Python when the interpreter encounters an unexpected termination of the input. This error indicates that the code structure is incomplete, which prevents the execution of the program.
Common Causes
- Unclosed Brackets or Parentheses: Missing closing brackets, braces, or parentheses can lead to this error.
- Incomplete Statements: If a statement is not properly concluded, such as missing a closing quote in a string.
- Improper Nesting: Incorrectly nested functions or structures can also result in this error.
- Multiline Strings: Failing to properly close a multiline string can trigger this message.
Identifying the Issue
To resolve a `SyntaxError: unexpected end of input`, it is crucial to identify where the code is incomplete. Here are methods to pinpoint the issue:
- Code Review: Manually review the code line by line to check for any unclosed structures.
- Use of Linters: Employing linting tools can help identify syntax errors before execution.
- Incremental Testing: Run smaller sections of the code to isolate the section causing the error.
Examples of the Error
Here are some code snippets that can produce a `SyntaxError: unexpected end of input`.
JavaScript Example:
“`javascript
function greet(name) {
console.log(“Hello, ” + name;
}
“`
*Error*: Missing closing parenthesis in the `console.log()` statement.
Python Example:
“`python
def add(a, b):
return a + b
“`
*Error*: If the function is called but the code block is not properly indented.
Best Practices to Avoid the Error
To prevent encountering `SyntaxError: unexpected end of input`, consider the following best practices:
- Consistent Code Formatting: Maintain consistent indentation and formatting to enhance readability.
- Use IDE Features: Leverage features in Integrated Development Environments (IDEs) that highlight syntax errors.
- Comment Unused Code: Comment out sections of code that are not being used rather than deleting them, which can help keep track of unclosed structures.
Troubleshooting Steps
If you encounter this error, follow these troubleshooting steps:
- Check for Matching Brackets: Ensure that every opening bracket has a corresponding closing bracket.
- Review String Closures: Verify that all strings are properly closed with quotes.
- Examine Function Definitions: Ensure all functions are properly defined and closed.
- Utilize Debugging Tools: Use debugging tools that provide hints and error messages to guide you.
By understanding the nature of `SyntaxError: unexpected end of input`, identifying common causes, and implementing best practices, developers can effectively troubleshoot and prevent this error in their coding projects.
Understanding the Causes of SyntaxError: Unexpected End of Input
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “The ‘SyntaxError: unexpected end of input’ often arises when a developer inadvertently leaves out closing brackets or parentheses in their code. This oversight can disrupt the parsing process, leading to confusion in the execution flow.”
Michael Chen (Lead Developer, Tech Innovations Inc.). “In JavaScript, this error typically indicates that the interpreter reached the end of a script without finding a complete statement. It is crucial for developers to ensure that all code blocks are properly closed and that there are no missing semicolons.”
Sarah Patel (Coding Instructor, Future Coders Academy). “For beginners, encountering ‘SyntaxError: unexpected end of input’ can be particularly frustrating. I always advise students to use code linters and formatters, which can help highlight syntax issues before they escalate into runtime errors.”
Frequently Asked Questions (FAQs)
What does the error message “syntaxerror: unexpected end of input” indicate?
This error message typically indicates that the JavaScript interpreter has reached the end of a script or function without finding a complete statement. It often suggests that there is a missing closing bracket, parenthesis, or quotation mark.
What are common causes of “syntaxerror: unexpected end of input”?
Common causes include missing closing braces, parentheses, or quotation marks in your code. It can also occur if a script is prematurely terminated or if there are issues with the code structure, such as an incomplete function or control statement.
How can I troubleshoot “syntaxerror: unexpected end of input”?
To troubleshoot, carefully check your code for any unclosed brackets, parentheses, or quotes. Use a code editor with syntax highlighting to identify mismatched pairs. Additionally, reviewing the last few lines of code where the error occurs can provide insights into the issue.
Does this error occur in programming languages other than JavaScript?
While the specific error message is common in JavaScript, similar syntax errors can occur in other programming languages. Each language may have its own terminology, but the underlying issue of incomplete code structure remains consistent.
Can using a linter help prevent “syntaxerror: unexpected end of input”?
Yes, using a linter can help prevent this error by analyzing your code for syntax issues and providing real-time feedback. Linters can catch missing brackets, parentheses, and other common mistakes before the code is executed.
Is there a way to identify the exact location of the error in my code?
Many development environments and browsers provide error messages that include line numbers or specific locations in the code where the error occurs. Utilize these tools to pinpoint the exact location and examine the surrounding code for potential issues.
The “syntaxerror: unexpected end of input” is a common error encountered in programming, particularly in languages such as JavaScript and Python. This error typically arises when the interpreter reaches the end of a script or function without finding a complete statement. It often indicates that there is a missing closing bracket, parenthesis, or quotation mark, which leads to an incomplete code structure. Understanding this error is crucial for developers as it can significantly hinder the debugging process and overall code execution.
One of the key takeaways from the discussion surrounding this error is the importance of proper code formatting and structure. Maintaining clear indentation, consistent use of brackets, and thorough commenting can help prevent such syntax errors. Additionally, utilizing code editors or integrated development environments (IDEs) that provide real-time syntax checking can be invaluable in identifying potential issues before execution. Emphasizing these practices can enhance code quality and reduce the frequency of encountering this error.
Moreover, recognizing the context in which this error occurs can aid in more efficient troubleshooting. Developers should familiarize themselves with the specific syntax rules of the programming language they are using. By doing so, they can quickly identify where the code may be lacking and take corrective action. Ultimately, a proactive approach to coding, combined with a
Author Profile
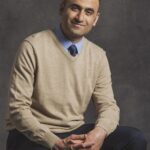
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?