How Can You Use $cast for Enums in SystemVerilog?
In the world of digital design and verification, SystemVerilog stands out as a powerful language that enhances the capabilities of traditional Verilog. Among its many features, the use of enumerated types (enums) allows designers to create more readable and maintainable code. However, as with any programming language, there are nuances that can trip up even seasoned engineers. One such nuance is the use of the `$cast` function, particularly when dealing with enums. Understanding how to effectively utilize `$cast` with enums can significantly streamline your code and improve its robustness, making it essential knowledge for anyone looking to master SystemVerilog.
Enums in SystemVerilog provide a way to define a set of named values, which can enhance code clarity and reduce the likelihood of errors. However, the ability to cast between different types—especially when working with enums—can pose challenges. The `$cast` function serves as a powerful tool that allows for safe type conversions, ensuring that your code behaves as expected. By leveraging `$cast`, designers can avoid common pitfalls associated with type mismatches, leading to more reliable simulations and synthesis results.
In this article, we will explore the intricacies of using `$cast` with enums in SystemVerilog, shedding light on best practices, potential pitfalls,
Using $cast with Enums in SystemVerilog
In SystemVerilog, `$cast` is a powerful built-in function that enables type conversion between different types, including enums. This is particularly useful when you need to assign values from one enum type to another or when interfacing with different data types in your design. The `$cast` function checks for compatibility between the source and target types at runtime, ensuring safe type assignments.
Syntax of $cast
The basic syntax for using `$cast` is as follows:
“`systemverilog
$cast(target_variable, source_variable);
“`
Here, `target_variable` is the variable that will receive the value, and `source_variable` is the enum or any other variable you wish to convert.
Example of $cast with Enums
Consider the following example, where two enums are defined: `color_t` and `light_t`.
“`systemverilog
typedef enum { RED, GREEN, BLUE } color_t;
typedef enum { OFF, ON } light_t;
color_t my_color;
light_t my_light;
// Using $cast to convert between enums
if ($cast(my_light, my_color)) begin
// Successfully casted
end else begin
// Casting failed
end
“`
In this scenario, `$cast` attempts to convert `my_color` to `my_light`. If the conversion is valid, it assigns the corresponding value; otherwise, it handles the failure gracefully.
Important Considerations
When using `$cast` with enums, keep the following considerations in mind:
- Compatibility: Ensure that the enums being casted share compatible representations. If they do not, the cast will fail.
- Default Values: If a cast fails, the target variable retains its previous value, which may lead to unpredictable behavior if not handled properly.
- Error Handling: Always check the return value of `$cast` to determine whether the operation was successful.
Advantages of Using $cast
- Type Safety: `$cast` provides a mechanism for type-safe conversions, allowing for more robust code.
- Runtime Checking: The function checks type compatibility at runtime, reducing potential errors during simulation or synthesis.
Limitations
- Performance Overhead: Since `$cast` involves runtime checks, it may introduce performance overhead in critical sections of code.
- Complexity: Overusing `$cast` can lead to code that is harder to read and maintain, especially if many different types are being converted.
Enum Type | Value |
---|---|
color_t | RED |
light_t | ON |
In summary, using `$cast` with enums in SystemVerilog allows for flexible and safe type conversions. By understanding the syntax, example usage, and considerations, you can effectively utilize this feature in your designs.
Using $cast with Enums in SystemVerilog
In SystemVerilog, the `$cast` function is utilized to perform type casting between different data types, including enums. When working with enumerated types, `$cast` can help ensure that values are correctly converted from one type to another, facilitating safer and more predictable code.
Syntax of $cast
The general syntax for `$cast` is as follows:
“`systemverilog
$cast(destination, source);
“`
- destination: The variable where the casted value will be stored.
- source: The variable or expression that is to be cast.
Example of Enum Casting
Consider the following example where we define an enum type and use `$cast` to convert between different enum values:
“`systemverilog
typedef enum {STATE_IDLE, STATE_RUNNING, STATE_STOPPED} state_t;
state_t current_state;
int temp_state;
// Assigning an enum value directly
current_state = STATE_IDLE;
// Attempting to cast an integer to an enum
if ($cast(current_state, temp_state)) begin
// Successfully casted
end else begin
// Cast failed, handle error
end
“`
Important Considerations
- Type Safety: Using `$cast` helps maintain type safety by ensuring that the conversion is valid. If the cast is not valid, `$cast` will return 0 ().
- Compatibility: Ensure that the source type is compatible with the destination type. If they are not compatible, the casting will fail.
- Enum Value Representation: Remember that enums are represented as integers internally. Therefore, casting from an integer to an enum can lead to unexpected behavior if the integer does not correspond to a valid enum value.
Advantages of Using $cast with Enums
- Error Handling: It allows for graceful handling of casting errors, which can be crucial in simulation and verification environments.
- Code Clarity: Using `$cast` provides clear intent in your code, showing that you are consciously converting between types.
- Maintainability: Improves the maintainability of code by reducing the chances of unintended type mismatches.
Example Table of Enum Values
Here’s a table showing how enum values can be represented and manipulated:
Enum Value | Integer Representation |
---|---|
STATE_IDLE | 0 |
STATE_RUNNING | 1 |
STATE_STOPPED | 2 |
Conclusion on Casting Enums
In summary, utilizing `$cast` for enumerated types in SystemVerilog enhances the robustness and reliability of your code. It is essential to understand both the syntax and the implications of using this function, especially regarding the compatibility of types. Always ensure that the source value is valid for the destination enum type to avoid runtime issues.
Expert Insights on Using $cast with Enums in SystemVerilog
Dr. Emily Chen (Senior Verification Engineer, Tech Innovations Inc.). “Utilizing $cast for enums in SystemVerilog allows for a flexible approach to type conversions. It enables designers to ensure that the values being assigned are compatible with the expected enum types, thus enhancing code safety and reducing runtime errors.”
Mark Thompson (Lead SystemVerilog Consultant, Verilog Masters). “The $cast function is particularly useful when dealing with polymorphic types in SystemVerilog. By leveraging $cast for enums, developers can effectively manage type hierarchies and ensure that the correct enum values are being utilized, which is crucial for maintaining the integrity of simulation results.”
Linda Patel (FPGA Design Architect, Advanced Circuit Solutions). “Incorporating $cast for enums can simplify the debugging process. It provides clear feedback when type mismatches occur, allowing engineers to quickly identify and rectify issues related to enum assignments, ultimately leading to more robust designs.”
Frequently Asked Questions (FAQs)
What is the purpose of using $cast in SystemVerilog?
$cast is used in SystemVerilog to safely convert one data type to another, ensuring that the conversion is valid. It checks the compatibility of the types at runtime and returns a boolean indicating success or failure.
Can $cast be used for enum types in SystemVerilog?
Yes, $cast can be used for enum types in SystemVerilog. It allows for the conversion of an enum variable to a different type, such as an integer, while ensuring that the conversion is valid.
How do you perform a $cast operation with enums?
To perform a $cast operation with enums, you specify the target type and the source enum variable. For example, `$cast(target_variable, source_enum_variable);` will attempt to cast the enum variable to the target type.
What happens if the $cast operation fails for an enum?
If the $cast operation fails, it returns a value of 0 (), indicating that the conversion was not successful. The target variable remains unchanged in this case.
Are there any limitations when using $cast with enums?
One limitation is that $cast cannot convert between unrelated enum types. Additionally, it may not be suitable for complex type hierarchies where the relationship between types is not clear.
Is it necessary to check the result of $cast when working with enums?
Yes, it is advisable to check the result of $cast when working with enums to ensure that the conversion was successful. This practice helps prevent runtime errors and ensures the integrity of the data.
In SystemVerilog, the `$cast` function is a powerful tool that facilitates type conversion, particularly when dealing with enumerated types. Enums in SystemVerilog are a way to define a set of named values, which can enhance code readability and maintainability. By utilizing `$cast`, designers can safely convert between different types, including enums, ensuring that the intended type is correctly interpreted during simulation or synthesis.
One of the key advantages of using `$cast` with enums is its ability to prevent type mismatches that can lead to simulation errors. When casting an enum type, `$cast` checks the compatibility of the source and target types, providing a safeguard against unintended conversions. This feature is especially beneficial in complex designs where enums are frequently used to represent state machines or control signals, helping to maintain the integrity of the design logic.
Moreover, the use of `$cast` enhances the flexibility of SystemVerilog code. It allows for dynamic type checking and can be particularly useful in scenarios involving polymorphism or when working with class-based designs. By leveraging this functionality, engineers can create more robust and adaptable code structures that can easily accommodate changes in design requirements without compromising type safety.
employing `$cast` for
Author Profile
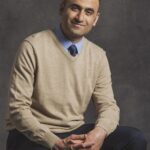
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?