How Can I Retrieve a List of Column Results from a T-SQL Stored Procedure?
In the world of database management, T-SQL (Transact-SQL) is a powerful tool that allows developers and database administrators to interact with Microsoft SQL Server. Among its many functionalities, the ability to execute stored procedures stands out as a vital feature for encapsulating complex logic and operations. However, when it comes to extracting results from these procedures, especially in the form of a dynamic list of columns, many users find themselves navigating a labyrinth of options and methods. Understanding how to effectively retrieve and manipulate these results can significantly enhance your data management capabilities and streamline your workflow.
When working with stored procedures in T-SQL, one common requirement is to obtain a list of columns from the result set they return. This can be particularly useful for reporting, data analysis, or even for dynamically generating SQL queries based on the structure of the results. However, the process is not always straightforward, as it involves understanding the underlying metadata and leveraging system views or functions to extract this information. By mastering these techniques, you can unlock the potential of your stored procedures and gain greater insights into your data.
In this article, we will explore the methods available for retrieving a list of column results from stored procedures in T-SQL. We will delve into the various approaches, including querying system catalog
Understanding Result Sets from Stored Procedures
When working with stored procedures in T-SQL, it is essential to recognize how to retrieve and list the columns from the result sets they return. A stored procedure can return multiple result sets, and understanding how to access the metadata of these result sets can facilitate dynamic querying and reporting.
Using SQL Server System Views
To obtain a list of columns returned by a stored procedure, you can query the system views. The key views to consider are `sys.columns` and `sys.objects`. These views allow you to extract information about the columns defined within the tables and views, but they do not directly provide column details for result sets produced by stored procedures.
However, you can use the following method to analyze the output of a stored procedure:
- Execute the stored procedure and capture the output in a temporary table or table variable.
- Query the temporary table or table variable to retrieve the column names and types.
Using Temporary Tables to Capture Output
Here is an example of how to create a temporary table to capture the output of a stored procedure:
“`sql
CREATE TABLE TempResult
(
Column1 INT,
Column2 NVARCHAR(50),
Column3 DATETIME
);
INSERT INTO TempResult
EXEC YourStoredProcedureName;
SELECT *
FROM TempResult;
DROP TABLE TempResult;
“`
In this example, replace `YourStoredProcedureName` with the name of your stored procedure. This method allows you to define the expected structure of the output and subsequently query the temporary table for its column information.
Dynamic SQL for Retrieving Column Names
For more dynamic scenarios where the structure of the result set is unknown, you can utilize dynamic SQL to capture the output and retrieve column names programmatically. Here is a sample script:
“`sql
DECLARE @Sql NVARCHAR(MAX);
SET @Sql = ‘EXEC YourStoredProcedureName’;
EXEC sp_executesql @Sql;
— Use information schema to retrieve column names
SELECT COLUMN_NAME
FROM INFORMATION_SCHEMA.COLUMNS
WHERE TABLE_NAME = ‘TempResult’;
“`
This script executes the stored procedure and retrieves the column names from the `INFORMATION_SCHEMA.COLUMNS` view, assuming you have captured the output in a known structure.
Example of Captured Result Set
To illustrate the output and column names captured, consider the following table structure from a hypothetical stored procedure output:
Column Name | Data Type |
---|---|
EmployeeID | INT |
FirstName | NVARCHAR(50) |
LastName | NVARCHAR(50) |
DateOfBirth | DATETIME |
This table shows the column names and their corresponding data types, providing a clear view of the result set structure returned by the stored procedure.
By utilizing these techniques, you can effectively manage and retrieve column information from stored procedures in T-SQL, enhancing your ability to work with dynamic data in SQL Server.
Retrieving Column Names from a Stored Procedure Result Set
To list the column names returned by a stored procedure in T-SQL, you can utilize the `INSERT EXEC` statement in combination with temporary tables or table variables. This method allows you to capture the output of the stored procedure and subsequently retrieve the column names.
Using a Temporary Table
- Create a Temporary Table: Define a temporary table that matches the expected output of the stored procedure.
“`sql
CREATE TABLE TempResults
(
Column1 INT,
Column2 NVARCHAR(100),
Column3 DATETIME
);
“`
- Insert Procedure Results: Use `INSERT EXEC` to execute the stored procedure and populate the temporary table.
“`sql
INSERT INTO TempResults
EXEC YourStoredProcedure;
“`
- Retrieve Column Names: Query the temporary table to get the column names.
“`sql
SELECT COLUMN_NAME
FROM tempdb.INFORMATION_SCHEMA.COLUMNS
WHERE TABLE_NAME LIKE ‘TempResults%’;
“`
Using a Table Variable
Alternatively, you can employ a table variable for similar functionality:
- Declare a Table Variable:
“`sql
DECLARE @TempResults TABLE
(
Column1 INT,
Column2 NVARCHAR(100),
Column3 DATETIME
);
“`
- Insert Procedure Results:
“`sql
INSERT INTO @TempResults
EXEC YourStoredProcedure;
“`
- Retrieve Column Names:
“`sql
SELECT COLUMN_NAME
FROM tempdb.INFORMATION_SCHEMA.COLUMNS
WHERE TABLE_NAME LIKE ‘TempResults%’;
“`
Dynamic SQL for Unknown Column Names
If the column names are not known beforehand, you can dynamically create the temporary table based on the output of the stored procedure.
- Create Dynamic SQL:
“`sql
DECLARE @SQL NVARCHAR(MAX);
SET @SQL = ‘SELECT * INTO DynamicResults FROM OPENROWSET(”SQLNCLI”, ”Server=YourServer;Trusted_Connection=yes;”, ”EXEC YourStoredProcedure”)’;
EXEC sp_executesql @SQL;
“`
- Retrieve Column Names:
“`sql
SELECT COLUMN_NAME
FROM tempdb.INFORMATION_SCHEMA.COLUMNS
WHERE TABLE_NAME LIKE ‘DynamicResults%’;
“`
Considerations
- Ensure that the stored procedure does not have parameters that need to be passed when executing.
- The use of temporary tables and table variables should be consistent with the expected volume of data to manage performance effectively.
- Always clean up temporary objects after use to avoid clutter in the database.
By following these methods, you can efficiently retrieve the list of column names from the result set of a stored procedure in T-SQL.
Expert Insights on Retrieving Column Results from T-SQL Stored Procedures
Dr. Emily Carter (Database Architect, Tech Solutions Inc.). “When working with T-SQL, retrieving a list of column results from a stored procedure can be efficiently achieved by utilizing the `INSERT INTO` statement combined with `EXEC`. This allows you to capture the output of the stored procedure into a temporary table, enabling further analysis and manipulation of the data.”
Michael Chen (Senior SQL Developer, Data Dynamics). “To extract column results from a stored procedure in T-SQL, it is essential to define the output parameters correctly. By specifying the data types and using the `OUTPUT` clause, you can seamlessly retrieve the desired columns after executing the procedure, ensuring that your application receives the necessary data in a structured format.”
Linda Gomez (Business Intelligence Consultant, Insight Analytics). “A common approach to list column results from a stored procedure is to create a view or a table-valued function that encapsulates the logic of the stored procedure. This method not only enhances reusability but also simplifies the retrieval of column data, allowing for more straightforward integration with reporting tools.”
Frequently Asked Questions (FAQs)
How can I retrieve a list of columns from a stored procedure in T-SQL?
To retrieve a list of columns from a stored procedure in T-SQL, you can use the `sp_help` system stored procedure or query the `INFORMATION_SCHEMA` views. For example, `EXEC sp_help ‘YourStoredProcedureName’` provides details about the parameters and result sets.
Can I get the result set structure of a stored procedure without executing it?
Yes, you can use the `sys.dm_exec_describe_first_result_set` function to obtain metadata about the first result set of a stored procedure without executing it. The syntax is `SELECT * FROM sys.dm_exec_describe_first_result_set(‘EXEC YourStoredProcedureName’, NULL, 0)`.
Is it possible to list the columns of a stored procedure’s result set dynamically?
Yes, you can create a dynamic SQL statement that executes the stored procedure and captures the result set structure using `sp_describe_first_result_set`. This allows you to dynamically retrieve column names and types.
What T-SQL command can I use to get column names from a stored procedure’s output?
You can use the `sys.dm_exec_describe_first_result_set` function to get the column names and data types from the output of a stored procedure. This command provides detailed information about the result set without executing the procedure.
Are there any limitations when retrieving column information from stored procedures?
Yes, limitations include the inability to retrieve information about multiple result sets directly and potential issues with dynamic SQL or procedures that return different structures based on input parameters. It is essential to test the stored procedure to ensure accurate results.
How do I handle stored procedures that return multiple result sets?
To handle stored procedures that return multiple result sets, you can use the `sp_describe_first_result_set` for the first result set and then execute the stored procedure to analyze subsequent result sets. Each result set may require separate handling in your application logic.
In T-SQL, retrieving a list of column results from a stored procedure involves understanding the structure of the stored procedure and how it returns data. Stored procedures can return result sets in various formats, and the metadata about these results can be accessed using system views or functions. The most common method to obtain the column names and types from a stored procedure is by utilizing the `sp_describe_first_result_set` system stored procedure, which provides detailed information about the first result set returned by a specified stored procedure.
Additionally, developers can leverage the `sys.dm_exec_describe_first_result_set` dynamic management function to obtain similar information. This function is particularly useful for understanding the schema of the result set without executing the stored procedure. It allows for better planning and integration of the stored procedure’s output within other T-SQL queries or applications that consume the data.
In summary, when working with T-SQL and stored procedures, it is crucial to utilize the appropriate system views and functions to extract metadata about the result sets. This practice not only enhances the efficiency of database operations but also aids in debugging and optimizing queries that depend on the output of stored procedures. Understanding how to effectively retrieve column information can significantly improve the development process and ensure that applications
Author Profile
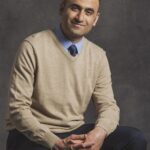
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?