Why Must the Target Type of a Lambda Conversion Be an Interface?
In the world of Java programming, the of lambda expressions has revolutionized the way developers write code, offering a more concise and expressive means of implementing functional interfaces. However, with this powerful feature comes a set of rules and nuances that every programmer should understand. One of the most critical aspects of lambda expressions is the necessity for their target type to be an interface. This requirement not only shapes how we design our code but also influences the overall architecture of Java applications. In this article, we will delve into the significance of this rule, exploring its implications and the underlying principles that govern lambda conversions.
At its core, a lambda expression is a shorthand notation for a function that can be passed around as a first-class citizen in Java. However, to harness the full potential of lambdas, developers must recognize that they can only be assigned to variables or passed as arguments to methods that expect a functional interface—a special type of interface that contains exactly one abstract method. This characteristic is what allows lambda expressions to seamlessly integrate into the Java programming landscape, enabling cleaner and more readable code while adhering to the principles of functional programming.
Understanding why the target type of a lambda conversion must be an interface is essential for any Java developer looking to leverage this feature effectively. It not only clarifies the constraints under
Understanding Lambda Expressions
Lambda expressions are a key feature in Java, introduced in Java 8, that allow for the creation of anonymous methods. They enable a more functional programming style by providing a concise way to express instances of single-method interfaces (commonly known as functional interfaces). The core principle behind lambda expressions is that they can be treated as instances of these interfaces, simplifying the coding process and improving readability.
Functional Interfaces
A functional interface is defined as an interface that contains exactly one abstract method. Lambda expressions can be used to implement these interfaces. This is crucial because it allows developers to pass behavior as parameters and create more flexible and reusable code.
Characteristics of functional interfaces include:
- Single Abstract Method: Must have exactly one abstract method.
- Default and Static Methods: Can contain multiple default and static methods.
- @FunctionalInterface Annotation: It is a good practice to use this annotation, which helps to enforce the single abstract method rule at compile time.
Example of a functional interface:
“`java
@FunctionalInterface
public interface MyFunctionalInterface {
void execute();
}
“`
Lambda Conversion and Target Types
The target type of a lambda expression must be a functional interface. This is vital because the Java compiler needs to know what type of interface the lambda is supposed to implement. If the target type is not an interface, the lambda expression cannot be converted, leading to a compilation error.
The process of lambda conversion involves the following:
- Type Inference: The Java compiler infers the type of the lambda expression from the context in which it is used.
- Target Type Requirement: The target type must be a functional interface, ensuring that the lambda can be assigned to a variable of that type.
Examples of Lambda Conversion
Consider the following examples illustrating lambda conversion in action:
“`java
// Functional interface
@FunctionalInterface
interface Calculator {
int add(int a, int b);
}
// Using a lambda expression
Calculator calculator = (a, b) -> a + b;
“`
In this scenario, the lambda `(a, b) -> a + b` is converted to an instance of the `Calculator` interface.
Common Errors with Lambda Expressions
Several common issues may arise when working with lambda expressions:
- Target Type Not an Interface: Attempting to assign a lambda expression to a non-interface type will result in a compilation error.
- Multiple Abstract Methods: If the target type has more than one abstract method, a lambda expression cannot be used.
Error Type | Description | Resolution |
---|---|---|
Target type not an interface | Lambda assigned to a class instead of an interface | Change target type to an interface |
More than one abstract method | Lambda expression cannot implement multiple methods | Ensure the interface is functional |
By adhering to the principles of functional interfaces and understanding the requirements for lambda conversion, developers can leverage the power of lambda expressions effectively within their Java applications.
Understanding Lambda Conversions
In programming, particularly in Java, a lambda expression is a concise way to represent an anonymous function. The conversion of a lambda expression to a functional interface is a crucial aspect of this feature. A functional interface is defined as an interface that contains exactly one abstract method. The conversion process is necessary to enable the lambda expression to be treated as an instance of the functional interface.
Functional Interfaces
Functional interfaces play a vital role in lambda expressions. Here are some characteristics and examples of functional interfaces:
- Single Abstract Method: A functional interface must have exactly one abstract method.
- @FunctionalInterface Annotation: This annotation can be used to indicate that an interface is intended to be a functional interface.
Examples of Functional Interfaces:
Interface Name | Abstract Method | Purpose |
---|---|---|
Runnable | void run() | Represents a task that can be run |
Callable |
T call() throws Exception | Represents a task that returns a value |
Consumer |
void accept(T t) | Represents an operation that takes a single input argument |
Function |
R apply(T t) | Represents a function that accepts one argument and produces a result |
Predicate |
boolean test(T t) | Represents a boolean-valued function of one argument |
Lambda Expression Conversion
The target type of a lambda expression must always be a functional interface. This requirement is pivotal for the compiler to correctly interpret the lambda expression. The process includes:
- Type Inference: The Java compiler infers the target type from the context where the lambda expression is used.
- Contextual Use: For example, passing a lambda expression as an argument to a method that expects a functional interface.
Example Code:
“`java
import java.util.function.Function;
public class LambdaExample {
public static void main(String[] args) {
Function
System.out.println(intToString.apply(5)); // Outputs “5”
}
}
“`
In this example, the lambda expression `(Integer i) -> String.valueOf(i)` is converted to the `Function
Implications of Target Type Requirement
The requirement that the target type must be a functional interface has several implications:
- Flexibility in Coding: Developers can create custom functional interfaces tailored to specific needs.
- Encapsulation of Behavior: Lambda expressions encapsulate behavior, allowing for cleaner and more maintainable code.
- Limitations: If an interface has more than one abstract method or is not an interface, the lambda cannot be converted, leading to a compile-time error.
Common Errors:
Error Type | Description |
---|---|
Multiple Abstract Methods | The interface has more than one abstract method. |
Non-Interface Type | The target type is not an interface. |
Conclusion on Lambda Conversion
Understanding that the target type of a lambda conversion must be an interface is essential for effective Java programming. This principle guides developers in creating functional interfaces and utilizing lambda expressions efficiently, promoting a functional programming style within the Java language.
Understanding Lambda Conversions and Interface Requirements
Dr. Emily Carter (Software Architect, Tech Innovations Inc.). “The requirement that the target type of a lambda conversion must be an interface stems from the need for functional programming constructs in Java. Interfaces provide a way to define a contract for behavior, allowing lambda expressions to be treated as first-class citizens in the language.”
Mark Thompson (Java Language Specialist, CodeCraft Academy). “In Java, lambda expressions are inherently tied to functional interfaces—interfaces with a single abstract method. This design choice not only simplifies the syntax but also enhances code readability and maintainability, making it crucial for developers to understand this requirement.”
Linda Zhao (Senior Developer, Open Source Solutions). “The necessity for lambda expressions to target interfaces is a fundamental aspect of Java’s type system. It ensures that lambdas can be seamlessly integrated with existing APIs that rely on functional interfaces, promoting a more functional programming style within the object-oriented paradigm.”
Frequently Asked Questions (FAQs)
What is a lambda expression in Java?
A lambda expression in Java is a concise way to represent an anonymous function that can be used to implement functional interfaces. It allows for more readable and maintainable code, particularly in the context of collections and streams.
Why must the target type of a lambda conversion be an interface?
The target type of a lambda conversion must be an interface because lambda expressions are designed to provide implementations for functional interfaces, which are interfaces that contain a single abstract method. This aligns with the principles of functional programming.
What is a functional interface?
A functional interface is an interface that contains exactly one abstract method. It can have multiple default or static methods, but the presence of a single abstract method allows it to be instantiated using a lambda expression.
Can a lambda expression be assigned to a non-functional interface?
No, a lambda expression cannot be assigned to a non-functional interface because non-functional interfaces do not conform to the requirement of having a single abstract method. Attempting to do so will result in a compilation error.
What happens if a lambda expression is used without a functional interface?
If a lambda expression is used without a functional interface, the Java compiler will not be able to determine the target type, leading to a compilation error. The lambda must be assigned to a compatible functional interface type.
Are there any built-in functional interfaces in Java?
Yes, Java provides several built-in functional interfaces in the `java.util.function` package, such as `Predicate`, `Function`, `Consumer`, and `Supplier`. These interfaces facilitate common operations and can be used with lambda expressions for various functional programming tasks.
The concept of lambda expressions in Java is intrinsically linked to functional interfaces. A lambda expression is essentially a concise way to represent an instance of a functional interface, which is defined as an interface containing a single abstract method. This requirement for a target type to be an interface ensures that lambda expressions can be seamlessly integrated into Java’s type system, allowing for cleaner and more readable code. The use of functional interfaces promotes a functional programming style within Java, enabling developers to write more expressive and less verbose code.
One of the key insights regarding the requirement for lambda expressions to target interfaces is the facilitation of polymorphism. By allowing lambda expressions to be assigned to functional interfaces, Java enables developers to pass behavior as parameters, return behavior from methods, and store behavior in data structures. This capability enhances code flexibility and reusability, making it easier to implement callbacks and event handling mechanisms.
Additionally, the emphasis on interfaces as the target type for lambda expressions encourages a design pattern that separates the definition of behavior from its implementation. This separation fosters better abstraction and encapsulation, leading to code that is easier to maintain and extend. Overall, the requirement that the target type of a lambda conversion must be an interface is a foundational aspect of Java’s approach to
Author Profile
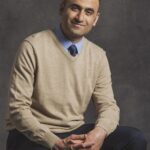
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?