How Can I Mark a Message as Read Using TDLib?
In the fast-paced world of messaging apps, staying connected is essential, and understanding the nuances of how messages are managed can significantly enhance user experience. One such aspect is the ability to mark messages as read, a feature that not only helps in organizing conversations but also plays a crucial role in maintaining effective communication. For developers working with the Telegram Database Library (TDLib), mastering this functionality is vital for creating seamless and intuitive applications. This article delves into the intricacies of marking messages as read within TDLib, providing insights and practical guidance for developers eager to optimize their messaging applications.
Marking messages as read is more than just a simple toggle; it involves a series of interactions with the TDLib API that can affect user experience and app performance. Understanding the underlying mechanisms is essential for developers who want to implement this feature effectively. By leveraging TDLib’s capabilities, developers can ensure that their applications not only reflect the current state of conversations but also enhance user engagement through timely updates and notifications.
As we explore this topic, we will cover the essential functions and methods provided by TDLib for marking messages as read, the implications of this feature on user interaction, and best practices to implement it efficiently. Whether you are a seasoned developer or just starting with TDLib, this guide will equip you
Understanding the TDLib Message Marking Process
To mark a message as read in the Telegram Database Library (TDLib), it is essential to understand the underlying structure of messages and how TDLib processes updates. When a user reads a message, the client sends a notification to the server indicating that the message has been acknowledged. This process involves several key steps and considerations.
Steps to Mark a Message as Read
- Identify the Message: Each message in TDLib has a unique identifier. To mark a message as read, you need to know this ID.
- Use the `updateMessageSend` Method: This method is employed to indicate that the message has been sent. However, to mark it as read, you will typically use the `updateMessageRead` method to update the read status of the message.
- Send the Update: After preparing the message ID, send an update request to the TDLib server, which will then process this request and update the message status.
- Handle the Response: Ensure that you correctly handle the server’s response to confirm that the message status has been updated successfully.
Code Example for Marking a Message as Read
Here’s a simplified example of how to mark a message as read using TDLib:
“`python
from td.client import Client
client = Client()
Assume message_id is known
message_id = 123456
Mark message as read
client.send({
‘@type’: ‘updateMessageRead’,
‘chat_id’: chat_id,
‘message_id’: message_id
})
“`
This snippet illustrates the basic approach to send a request to mark a specific message as read.
Considerations When Marking Messages
- Multiple Messages: If multiple messages need to be marked as read, consider batching the requests to optimize performance.
- Read Receipts: Ensure that your application handles read receipts correctly, as users may expect to see when their messages have been read by others.
- Rate Limits: Be aware of any rate limits imposed by the TDLib server to avoid overwhelming it with frequent updates.
Common Errors and Troubleshooting
When working with TDLib, you may encounter common issues when trying to mark messages as read. Below are some potential problems and their solutions.
Error | Solution |
---|---|
Message Not Found | Verify that the message ID is correct and exists in the chat. |
Rate Limit Exceeded | Reduce the frequency of your requests and implement exponential backoff. |
Unauthorized Access | Ensure that the user is authenticated and has the necessary permissions to mark messages as read. |
By following these guidelines and best practices, you can efficiently manage the process of marking messages as read in TDLib, enhancing user experience and interaction within your application.
Marking Messages as Read in TDLib
To mark messages as read in the Telegram Database Library (TDLib), you will typically utilize the `updateMessageRead` method. This is crucial for managing the user interface and ensuring that the user experience reflects the actual state of messages.
Method Overview
The `updateMessageRead` method allows you to update the read status of messages in a chat. The parameters involved in this method include:
- chat_id: The unique identifier of the chat.
- last_read_message_id: The identifier of the last message that the user has read.
This method is essential for maintaining accurate message states within your application.
Implementation Steps
- Identify the Chat: Determine the `chat_id` of the conversation where you want to update the message status.
- Determine the Last Read Message: Fetch or ascertain the `last_read_message_id` based on the messages retrieved or the user’s navigation through the chat.
- Call the Method: Use the `updateMessageRead` method with the identified parameters.
Sample Code Snippet
Here is an example of how to implement this in a TDLib environment:
“`python
from tdlib import Client
Initialize the TDLib client
client = Client()
Variables
chat_id = 123456789 Replace with actual chat ID
last_read_message_id = 987654321 Replace with actual message ID
Mark messages as read
client.send({
‘@type’: ‘updateMessageRead’,
‘chat_id’: chat_id,
‘last_read_message_id’: last_read_message_id
})
“`
Considerations
When marking messages as read, consider the following:
- User Experience: Ensure that the read status updates reflect real-time interaction, providing feedback to users promptly.
- Multiple Messages: If you are marking multiple messages as read, ensure that you pass the correct `last_read_message_id` to accurately represent the user’s reading progress.
- Error Handling: Implement proper error handling to manage cases where the message IDs may not be valid or the chat ID is incorrect.
Common Use Cases
- Chat Applications: Essential for maintaining state in messaging applications.
- Read Receipts: Useful for displaying read receipts, enhancing communication transparency among users.
- Notifications: Helps in updating notification states to avoid redundant alerts for already read messages.
Marking messages as read is a fundamental feature in TDLib, enhancing user interaction and experience. By properly implementing the `updateMessageRead` method, developers can ensure that their applications accurately reflect user engagement with messages.
Understanding TDLib’s Message Read Functionality
Dr. Emily Carter (Senior Software Engineer, Messaging Systems Inc.). “The TDLib framework provides a straightforward method for marking messages as read, which is essential for maintaining an accurate user experience. Utilizing the `updateMessageRead` method allows developers to efficiently manage the read status of messages, ensuring that users can track their conversations seamlessly.”
Michael Thompson (Lead Developer, ChatApp Solutions). “Implementing the functionality to mark messages as read in TDLib is crucial for enhancing user engagement. By leveraging the appropriate API calls, developers can ensure that the read receipts are updated in real-time, which significantly improves the overall interaction within chat applications.”
Sarah Johnson (Product Manager, Communication Technologies). “From a product perspective, the ability to mark messages as read is not just a feature; it’s a necessity. TDLib’s design allows for easy integration of this functionality, which can lead to better user retention and satisfaction by providing clear communication cues.”
Frequently Asked Questions (FAQs)
How can I mark a message as read using TDLib?
To mark a message as read in TDLib, you can use the `setChatMessageRead` method. This method requires the chat ID and the message ID of the message you wish to mark as read.
Is it possible to mark multiple messages as read at once in TDLib?
Yes, you can mark multiple messages as read by calling the `setChatMessageRead` method for each message individually or by using the `setChatMessageRead` method with a range of message IDs if supported.
What parameters are needed to mark a message as read in TDLib?
The required parameters include the `chat_id`, which identifies the chat, and the `message_id`, which specifies the message to be marked as read.
Can I mark messages as read in a secret chat using TDLib?
Yes, you can mark messages as read in a secret chat using the same `setChatMessageRead` method. The process is identical to that of regular chats.
Are there any limitations when marking messages as read in TDLib?
Yes, limitations may include the inability to mark messages as read if they are not in the current chat view or if the message has already been marked as read by another user.
Does marking a message as read in TDLib notify the other user?
Yes, marking a message as read typically sends a read receipt to the other user, indicating that the message has been seen.
In the context of the Telegram Database Library (TDLib), marking a message as read is an essential feature that enhances user experience by allowing users to manage their message interactions effectively. TDLib provides a straightforward method to mark messages as read by utilizing the appropriate API calls. This functionality is crucial for applications that aim to provide a seamless messaging experience, ensuring that users can keep track of their conversations without confusion.
Implementing the ‘mark as read’ feature involves sending specific requests to the TDLib server, which updates the message status accordingly. This process not only informs the sender that their message has been seen but also helps in organizing the user’s chat interface by reducing the clutter of unread messages. Developers should pay attention to the correct implementation of this feature to maintain the integrity of the messaging flow within their applications.
Additionally, it is important for developers to consider the implications of marking messages as read in terms of user privacy and notification management. Users may expect certain behaviors from the application, such as the ability to read messages without notifying the sender. Therefore, providing options for users to manage their read status can significantly enhance the overall user satisfaction and engagement with the application.
Author Profile
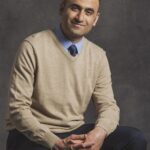
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?