Why Am I Seeing ‘terminate called after throwing an instance of std::bad_alloc’ in My Code?
In the world of C++ programming, encountering errors can be a frustrating yet enlightening experience. One such error that often leaves developers scratching their heads is the dreaded message: “terminate called after throwing an instance of ‘std::bad_alloc’.” This cryptic notification signals an issue related to memory allocation, a fundamental aspect of programming that can make or break the efficiency and stability of an application. As developers delve into the intricacies of dynamic memory management, understanding the nuances of this error becomes crucial for crafting robust and resilient software.
Overview
At its core, the `std::bad_alloc` exception is thrown when a program attempts to allocate memory but fails to do so, typically due to insufficient available memory. This situation can arise in various scenarios, such as allocating large data structures, memory leaks, or even system limitations. The abrupt termination of the program not only disrupts the user experience but also poses challenges for debugging and resolution. Therefore, grasping the underlying causes and implications of this error is essential for developers aiming to enhance their applications’ performance and reliability.
Moreover, addressing the `std::bad_alloc` exception requires a multifaceted approach. Developers must not only implement effective memory management strategies but also cultivate an understanding of their program’s memory usage patterns. By
Understanding std::bad_alloc
The error message `terminate called after throwing an instance of ‘std::bad_alloc’` typically indicates that a program has attempted to allocate memory dynamically but failed to do so. This exception is thrown by the C++ Standard Library when a memory allocation request cannot be fulfilled, usually due to insufficient memory available in the heap.
When memory allocation fails, the C++ runtime system throws an instance of the `std::bad_alloc` class, which is derived from `std::exception`. This exception can be caught, allowing the program to handle the error gracefully instead of terminating abruptly. However, if not handled, the program will terminate, leading to the aforementioned message.
Key points regarding `std::bad_alloc`:
- It is part of the `
` header in C++. - It is thrown by operators `new` and `new[]` when they cannot allocate memory.
- Failure can be due to exhausting the available memory or fragmentation.
Common Causes of Memory Allocation Failure
Several factors can lead to a `std::bad_alloc` exception being thrown:
- Insufficient System Memory: The system may not have enough RAM available for the allocation request.
- Memory Fragmentation: Even if total free memory is sufficient, fragmentation can prevent large contiguous memory blocks from being allocated.
- Excessive Memory Usage: Programs that hold onto memory without releasing it can lead to exhaustion of available memory.
- Large Allocation Requests: Requests for large arrays or objects can exceed available memory, especially on systems with limited resources.
Best Practices for Handling std::bad_alloc
To mitigate the impact of `std::bad_alloc`, consider implementing the following best practices:
- Catch Exceptions: Always catch `std::bad_alloc` in critical sections of your code to prevent abrupt program termination.
“`cpp
try {
int* array = new int[1000000000]; // Large allocation
} catch (const std::bad_alloc& e) {
std::cerr << "Memory allocation failed: " << e.what() << std::endl;
}
```
- Use Smart Pointers: Utilize smart pointers such as `std::unique_ptr` or `std::shared_ptr` to manage memory automatically and reduce the likelihood of memory leaks.
- Monitor Memory Usage: Regularly check memory usage during runtime to identify potential leaks or excessive allocations.
- Optimize Memory Allocation: Use data structures that are more efficient in memory use, and consider memory pools for frequent allocations.
Debugging Memory Allocation Issues
When faced with a `std::bad_alloc`, debugging is essential. Here are some strategies:
- Use Memory Profiling Tools: Tools like Valgrind, AddressSanitizer, or built-in profilers can help identify memory leaks and allocation patterns.
- Check for Memory Leaks: Ensure that every allocated memory is deallocated when no longer needed.
- Reduce Allocation Size: Reassess the size of your allocations and consider whether they can be reduced or managed differently.
Strategy | Description |
---|---|
Catching Exceptions | Wrap allocations in try-catch blocks to handle failures gracefully. |
Memory Profiling | Use tools to monitor and analyze memory usage and leaks. |
Code Review | Regularly review code for memory management practices. |
Optimize Data Structures | Select data structures that use memory efficiently. |
By understanding and effectively managing `std::bad_alloc`, developers can create more robust applications that handle memory allocation issues gracefully.
Understanding std::bad_alloc
The `std::bad_alloc` exception is part of the C++ Standard Library and is thrown when a memory allocation fails. This exception is derived from the `std::exception` class and is typically encountered during dynamic memory allocation using operators like `new` or functions such as `malloc`.
Common Causes of std::bad_alloc:
- Insufficient Memory: The most common cause is that the system does not have enough memory available to satisfy the allocation request.
- Memory Fragmentation: Over time, memory may become fragmented, leading to allocation failures even when the total free memory is adequate.
- Over-allocation: Attempting to allocate an excessively large block of memory that exceeds system limits or available resources can trigger this exception.
- Memory Leaks: If a program does not properly manage memory, it can lead to exhaustion of available memory over time.
Debugging std::bad_alloc
To effectively diagnose the cause of a `std::bad_alloc` exception, consider the following strategies:
- Check Allocation Sizes: Ensure that your allocation requests are reasonable and within the limits of your application’s needs.
- Use Smart Pointers: Implementing smart pointers (like `std::unique_ptr` or `std::shared_ptr`) can help manage memory more effectively, reducing leaks and fragmentation.
- Monitor Memory Usage: Utilize profiling tools to monitor memory usage and identify potential leaks or excessive allocations.
- Test on Different Systems: Run your application on systems with varying memory configurations to see if the problem persists.
Handling std::bad_alloc
When encountering a `std::bad_alloc` exception, it is crucial to handle it gracefully to maintain application stability. Here are best practices for handling this exception:
- Try-Catch Blocks: Wrap your memory allocation code in a try-catch block to catch `std::bad_alloc`.
“`cpp
try {
int* array = new int[1000000000]; // Example of a large allocation
} catch (const std::bad_alloc& e) {
std::cerr << "Memory allocation failed: " << e.what() << std::endl;
}
```
- Fallback Mechanisms: Implement fallback strategies, such as allocating smaller chunks of memory or freeing up existing resources when an allocation fails.
- Log Errors: Maintain logs of when and where the allocations fail to assist in diagnosing the underlying issues.
Preventing std::bad_alloc
To mitigate the chances of encountering `std::bad_alloc`, consider the following preventative measures:
- Limit Memory Usage: Set limits on memory usage for specific operations to avoid overwhelming the system.
- Optimize Data Structures: Use memory-efficient data structures that minimize overhead and fragmentation.
- Perform Regular Cleanup: Regularly free unused memory and resources in your application to prevent leaks.
- Testing Under Load: Conduct stress testing to simulate high memory usage scenarios and identify potential failure points.
Addressing `std::bad_alloc` effectively requires understanding its causes, implementing robust handling strategies, and taking proactive steps to prevent its occurrence. By adhering to best practices in memory management and adopting a defensive coding approach, developers can significantly reduce the risk of encountering memory allocation failures.
Understanding Memory Management Errors in C++
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error ‘terminate called after throwing an instance of std::bad_alloc’ typically indicates that your program has attempted to allocate memory dynamically but has failed due to insufficient memory. This can often be a result of memory leaks or excessive memory consumption.”
Michael Chen (Lead C++ Developer, CodeCraft Solutions). “When encountering a std::bad_alloc exception, it is crucial to investigate memory usage patterns within your application. Tools like Valgrind can help identify memory leaks, while proper exception handling can prevent abrupt terminations.”
Sarah Thompson (Systems Architect, FutureTech Systems). “To mitigate the occurrence of std::bad_alloc exceptions, developers should consider implementing smart pointers and optimizing data structures. This not only enhances memory management but also improves overall application stability.”
Frequently Asked Questions (FAQs)
What does the error ‘terminate called after throwing an instance of std::bad_alloc’ indicate?
This error indicates that the program attempted to allocate memory dynamically but failed, triggering a `std::bad_alloc` exception. This typically occurs when the system runs out of memory or when memory allocation requests exceed available resources.
What causes a std::bad_alloc exception in C++?
A `std::bad_alloc` exception is caused by a failed memory allocation request, often due to insufficient memory available for the allocation. This can happen if the program requests a large amount of memory or if the system is under heavy memory pressure.
How can I troubleshoot the std::bad_alloc error?
To troubleshoot this error, you can check the memory usage of your application, optimize memory allocation strategies, and ensure that you are not leaking memory. Additionally, reviewing the size of the allocations and using tools like memory profilers can help identify the root cause.
Is there a way to prevent std::bad_alloc exceptions?
While it is not possible to completely prevent `std::bad_alloc` exceptions, you can implement better memory management practices, such as using smart pointers, limiting the size of allocations, and regularly monitoring memory usage to avoid excessive consumption.
What should I do if I encounter this error during runtime?
If you encounter this error during runtime, you should first analyze the code that triggers the allocation. Use debugging tools to inspect memory usage and identify potential leaks. Consider adding exception handling to gracefully manage memory allocation failures.
Can std::bad_alloc exceptions be caught in C++?
Yes, `std::bad_alloc` exceptions can be caught using a try-catch block. This allows you to handle the error gracefully, providing an opportunity to log the error, clean up resources, or attempt alternative strategies for memory allocation.
The error message “terminate called after throwing an instance of ‘std::bad_alloc'” typically indicates that a C++ program has attempted to allocate memory dynamically but has failed to do so. This failure usually occurs when the system runs out of memory or when the allocation request exceeds the available memory limits. The C++ Standard Library uses the `std::bad_alloc` exception to signal that memory allocation has failed, which is a critical aspect of error handling in memory management within C++ applications.
When such an error occurs, the program’s default behavior is to call the `std::terminate()` function, which results in the abrupt termination of the program. This behavior underscores the importance of robust memory management practices in C++ programming. Developers should ensure that their applications handle memory allocation failures gracefully, which may involve using exception handling mechanisms to catch `std::bad_alloc` and implementing strategies to minimize memory usage.
Key takeaways from this discussion include the necessity for developers to monitor memory usage closely and to be aware of the potential for memory allocation failures. Employing smart pointers, optimizing data structures, and conducting thorough testing can help mitigate the risks associated with memory management. Additionally, understanding the implications of `std::bad_alloc` can lead to better error
Author Profile
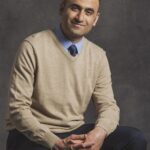
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?