Why Does the Given Element Not Have a Value Setter?
In the ever-evolving landscape of programming and web development, understanding the nuances of how elements interact within a system is crucial for creating seamless user experiences. One common challenge developers encounter is the perplexing message: “the given element does not have a value setter.” This seemingly simple phrase can lead to a cascade of confusion, particularly for those navigating the intricacies of various programming languages and frameworks. In this article, we will delve into the implications of this message, exploring its roots and the contexts in which it arises, ultimately equipping you with the knowledge to troubleshoot and overcome this hurdle.
At its core, the phrase “the given element does not have a value setter” highlights a fundamental aspect of how data binding and manipulation work in programming. It suggests that the element in question is either immutable or lacks the necessary methods to modify its value, which can be a source of frustration for developers aiming to implement dynamic features. Understanding the underlying principles of data handling is essential, as it not only informs best practices but also enhances your ability to debug and optimize your code.
As we navigate through the complexities of this topic, we will examine common scenarios where this message may appear, the potential causes behind it, and strategies for effectively addressing the issue. By the end of this exploration, you will
The Given Element Does Not Have a Value Setter
In programming, particularly within the context of web development and user interface design, encountering an element that lacks a value setter can lead to confusion and challenges in functionality. This situation often arises with various input types, such as custom elements or specific frameworks, which may not support direct value manipulation.
When an element does not have a value setter, it typically means that the element’s state or value cannot be directly altered through standard property assignment. Instead, developers must utilize alternative methods to manage the state or value of the element. Here are some common scenarios where this may occur:
- Custom Elements: These are user-defined elements created using the Web Components specification. They may require specific methods to set their values.
- Framework-Specific Components: Libraries like React, Angular, or Vue may encapsulate the handling of state, making direct manipulation impractical.
- Read-Only Inputs: Elements such as `` cannot have their values changed programmatically unless the `readonly` attribute is removed.
To handle these situations effectively, developers can employ several strategies:
- Event Listeners: Adding event listeners can allow for dynamic interaction that updates the element’s value based on user inputs or other events.
- Methods Provided by the Element: Many custom elements expose methods designed for updating their state, which should be utilized instead of trying to set values directly.
- Binding in Frameworks: Utilizing data binding techniques in frameworks can streamline value management without resorting to direct access.
Example of Value Management in Custom Elements
Consider a custom web component that encapsulates complex logic. The developer must ensure that the component provides a clear API for value setting. Here’s an example of how you can manage values within such a component:
“`javascript
class MyCustomElement extends HTMLElement {
constructor() {
super();
this._value = ”;
}
set value(newValue) {
this._value = newValue;
this.render();
}
get value() {
return this._value;
}
render() {
this.innerHTML = ``;
}
}
customElements.define(‘my-custom-element’, MyCustomElement);
“`
In the example above, the `value` property is managed through a setter method, which allows the component to maintain control over its internal state while still providing an interface for external interaction.
Table of Common Scenarios
Scenario | Description | Solution |
---|---|---|
Custom Elements | Elements defined by developers that may not expose standard setters. | Use provided methods for updating values. |
Framework Components | Components from libraries/frameworks that manage state internally. | Utilize data binding or state management techniques. |
Read-Only Inputs | Inputs that cannot be modified directly due to the `readonly` attribute. | Remove `readonly` attribute before attempting to set values. |
Understanding these principles is vital for developers aiming to create responsive and user-friendly applications that utilize custom elements effectively.
Understanding the Value Setter Concept
In programming, particularly in object-oriented languages, the concept of a “value setter” refers to a method that allows the modification of a property or variable within an object. When an element does not have a value setter, it typically implies certain design decisions or constraints.
- Immutable Objects: Some objects are designed to be immutable, meaning their state cannot be changed after they are created. Examples include:
- Strings in Java
- Tuples in Python
- Encapsulation: In some cases, properties are intentionally left without setters to enforce encapsulation and maintain control over how and when values are modified.
Common Scenarios Where Value Setters Are Absent
- Constants: Certain classes may define constants that are meant to remain unchanged throughout the program’s execution. For instance:
- `public static final int MAX_SIZE = 100;` in Java.
- Read-Only Properties: Some properties are designed to be read-only to prevent accidental modification. This is common in frameworks where certain attributes derive their values from internal processes.
- Abstract Classes and Interfaces: When dealing with abstract classes or interfaces, properties might not have setters as they are intended to be implemented in derived classes.
Impact of Missing Value Setters
The absence of a value setter can influence code functionality and design patterns in several ways:
- Enhanced Security: By restricting changes to certain properties, developers can reduce the risk of unintended side effects.
- Improved Maintainability: Code that is less prone to modification can lead to fewer bugs and easier debugging processes.
- Increased Clarity: When elements explicitly indicate that they do not allow changes, it can clarify usage and expected behavior for other developers.
Alternatives to Value Setters
When a value setter is not available, developers often rely on alternative methods to manage state:
Alternative Method | Description |
---|---|
Constructors | Use constructors to set initial values during object creation. |
Factory Methods | Implement factory methods that return configured instances. |
Builder Pattern | Utilize the builder pattern to create complex objects step-by-step. |
Static Methods | Use static methods to modify shared state or configurations. |
Best Practices for Handling Elements Without Value Setters
- Documentation: Clearly document any properties that do not have setters, explaining the rationale behind this design choice.
- Use of Getters: Provide getter methods for properties that need to be accessed, allowing for read-only access while maintaining control.
- Design with Intent: When designing classes, consider the implications of making properties read-only and how this aligns with the overall architecture of your application.
By understanding the implications and alternatives to value setters, developers can create robust and maintainable code structures that adhere to best practices in software design.
Understanding Value Setters in Programming Elements
Dr. Emily Carter (Software Engineer, Tech Innovations Inc.). “The absence of a value setter for a given element typically indicates a design decision aimed at maintaining data integrity. It ensures that the element’s state is managed through controlled access, preventing unintended modifications that could lead to errors.”
Mark Thompson (Senior Frontend Developer, CodeCraft Solutions). “When an element does not have a value setter, it often suggests that the element is intended to be read-only. This can be beneficial in scenarios where user input should be restricted, thereby enhancing security and user experience.”
Linda Garcia (UI/UX Specialist, Design Dynamics). “Elements without value setters can also serve as a means to enforce design consistency. By limiting how values can be changed, designers can ensure that the user interface remains predictable and intuitive, which is crucial for user engagement.”
Frequently Asked Questions (FAQs)
What does it mean when an element does not have a value setter?
When an element does not have a value setter, it indicates that the element is read-only or its value is determined by other means, preventing direct modification through standard property setters.
Why would an element be designed without a value setter?
Elements may be designed without a value setter to maintain data integrity, ensure consistency, or to enforce specific business logic that dictates how values should be assigned or modified.
How can I modify the value of an element that lacks a value setter?
To modify the value of such an element, you may need to use alternative methods, such as invoking specific methods provided by the element’s API, or by manipulating related properties or data structures.
Are there any performance implications when working with elements without value setters?
Yes, there can be performance implications, as accessing and modifying values through alternative methods may introduce additional overhead compared to direct property manipulation.
Can I still retrieve the value of an element that does not have a value setter?
Yes, you can retrieve the value of an element without a value setter, as read operations are typically permitted even when write operations are restricted.
What are some common examples of elements without value setters?
Common examples include certain UI components, configuration settings in frameworks, and immutable data structures that are designed to prevent changes after their initial creation.
The phrase “the given element does not have a value setter” typically refers to a situation in programming or web development where a specific element, such as a form input or a UI component, lacks a method or property to assign a value directly. This can lead to challenges when trying to manipulate the element’s state or behavior dynamically. Understanding this limitation is crucial for developers to ensure that their applications function as intended, especially when dealing with user input or data binding scenarios.
One of the main points to consider is the importance of selecting the right elements and frameworks that support value setting. Many modern frameworks and libraries provide built-in mechanisms for data binding, which can alleviate the issues associated with elements lacking value setters. Developers should familiarize themselves with the capabilities of the tools they are using to avoid potential pitfalls and enhance the user experience.
Additionally, it is essential to recognize that workarounds may exist for elements without value setters. For instance, developers can often use event listeners or alternative methods to manipulate the element’s state indirectly. However, relying on such workarounds can lead to increased complexity and maintenance challenges. Therefore, thorough documentation and understanding of the elements in use are imperative for effective development.
the absence of a
Author Profile
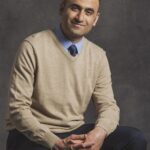
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?