Why Am I Seeing ‘The Index Was Outside the Bounds of the Array?’ – Common Causes and Solutions
In the world of programming, few errors are as universally dreaded as the infamous “index was outside the bounds of the array” message. This seemingly cryptic notification can send even seasoned developers into a spiral of debugging, as it often signifies a fundamental misunderstanding of how data structures operate. Whether you’re a novice coder or a seasoned software engineer, encountering this error can be both frustrating and enlightening. Understanding the underlying principles of array indexing is crucial for writing efficient, error-free code. Join us as we delve into the intricacies of this common issue, exploring its causes, implications, and best practices for resolution.
Overview
At its core, the error “index was outside the bounds of the array” arises when a program attempts to access an element of an array using an index that is either too high or too low. Arrays, as fundamental data structures, have specific limits defined by their size, and any attempt to breach these boundaries results in this disruptive error. This issue not only halts program execution but can also lead to unexpected behavior, making it imperative for developers to grasp the concept of indexing thoroughly.
As we navigate through the various scenarios that lead to this error, we will uncover the common pitfalls that programmers encounter when working with arrays. From off-by-one errors
Understanding Array Bounds
When working with arrays in programming, the concept of bounds is critical. Each array has a fixed size, and its elements are accessed using indices that typically start at zero. An “index out of bounds” error occurs when a program attempts to access an element outside the limits of the array. This can lead to runtime exceptions, potentially crashing applications or causing unexpected behavior.
Common scenarios that lead to this error include:
- Attempting to access an index that is negative.
- Accessing an index greater than or equal to the array length.
- Failing to validate user input that determines array indices.
Causes of Index Out of Bounds Errors
Understanding the various causes of this error can help programmers avoid pitfalls when handling arrays. Some prevalent causes include:
- Incorrect Loop Conditions: Iterating beyond the length of the array can happen if the loop condition is not set accurately.
- Dynamic Array Resizing: When arrays are resized, references to the old array may still try to access elements based on the original size.
- User Input: Not validating user-supplied indices can lead to attempts to access non-existent array elements.
Best Practices to Avoid Index Out of Bounds Errors
To mitigate the risk of encountering index out of bounds errors, consider implementing the following best practices:
- Always check the length of the array before accessing an index.
- Use exception handling to manage potential errors gracefully.
- Validate input thoroughly, especially if it comes from external sources.
Example of Index Bound Checking
Here is a simple example in pseudocode that demonstrates how to safely access an array:
“`pseudocode
array = [1, 2, 3, 4, 5]
index = getUserInput()
if index >= 0 and index < length(array) then print array[index] else print "Error: Index is out of bounds." end if ```
Common Programming Languages and Their Handling of Array Bounds
Different programming languages have varying approaches to handling array bounds. Below is a comparison table illustrating how some popular languages address this issue:
Language | Array Bounds Checking | Exception Handling |
---|---|---|
Java | Checked (throws ArrayIndexOutOfBoundsException) | Yes (try-catch blocks) |
C | Checked (throws IndexOutOfRangeException) | Yes (try-catch blocks) |
C++ | Unchecked ( behavior if out of bounds) | No built-in exception for arrays |
Python | Checked (raises IndexError) | Yes (try-except blocks) |
By adhering to these practices and understanding how different programming languages handle array bounds, developers can significantly reduce the occurrence of index out of bounds errors in their applications.
Understanding the Error Message
The error message “the index was outside the bounds of the array” typically arises in programming languages that utilize arrays for data storage, such as C, Java, or Python. This error indicates that an attempt has been made to access an element in an array using an index that is not valid, meaning it is either negative or exceeds the array’s length.
Common Causes of the Error
- Negative Indexing: Arrays generally do not support negative indices unless explicitly allowed (e.g., Python lists).
- Exceeding Array Length: Accessing an index that is greater than or equal to the size of the array will trigger this error.
- Uninitialized Arrays: Trying to access elements of an array that has not been properly initialized can lead to this error.
- Loop Errors: Off-by-one errors in loops can also result in trying to access invalid indices.
Identifying the Source of the Error
To effectively diagnose the cause of this error, consider the following steps:
- Review the Array Declaration: Check if the array was initialized correctly, with a defined size.
- Check Index Values: Print or log the index value being accessed to ensure it falls within the allowed range.
- Debugging Tools: Utilize debugging tools to step through your code and monitor array access and index values.
Example Scenario
In the following example, a common cause of the error is illustrated:
“`csharp
int[] numbers = new int[5]; // Valid array of size 5
int index = 5; // Invalid index
Console.WriteLine(numbers[index]); // This line will cause the error
“`
Preventative Measures
Implementing certain practices can help prevent encountering this error in future coding endeavors:
- Boundary Checks: Always verify that indices are within the valid range before accessing array elements.
- Utilize Language Features: Some programming languages offer built-in features to handle such errors gracefully.
- Exception Handling: Implement try-catch blocks to manage potential index out-of-bounds exceptions.
Best Practices for Array Management
To further minimize the risk of triggering this error, consider the following best practices:
Practice | Description |
---|---|
Initialize Properly | Ensure arrays are initialized with a specific size. |
Use Constants | Define constants for array sizes to avoid magic numbers. |
Validate Inputs | Always validate any input used as an index before access. |
Employ Data Structures | Use collections like lists or dictionaries that handle resizing. |
Handling the Error in Code
When the error does occur, a robust handling mechanism can improve user experience. Here’s how to handle the exception in C:
“`csharp
try {
int[] numbers = new int[5];
Console.WriteLine(numbers[5]); // Potentially problematic line
} catch (IndexOutOfRangeException e) {
Console.WriteLine(“An error occurred: ” + e.Message);
}
“`
This code snippet demonstrates how to catch the exception and provide feedback without crashing the application, enhancing robustness.
Understanding Array Index Errors in Programming
Dr. Emily Carter (Software Development Specialist, Tech Innovations Inc.). “The error message ‘the index was outside the bounds of the array’ typically indicates that a program is attempting to access an element of an array using an index that does not exist. This can often be attributed to off-by-one errors, where developers mistakenly assume the array starts at index 1 instead of index 0.”
James Liu (Senior Software Engineer, CodeSecure Solutions). “To effectively prevent this issue, it is crucial for developers to implement proper boundary checks before accessing array elements. Utilizing tools like static code analysis can help identify potential out-of-bounds errors during the development phase.”
Dr. Sarah Thompson (Data Structures and Algorithms Professor, University of Technology). “Understanding how arrays function in memory is essential for avoiding out-of-bounds errors. Educating programmers on the specifics of data structures and their indexing can significantly reduce the frequency of these common mistakes.”
Frequently Asked Questions (FAQs)
What does “the index was outside the bounds of the array” mean?
This error indicates that an attempt was made to access an element of an array using an index that is either negative or greater than or equal to the array’s length, which is not permissible.
What are common causes of this error in programming?
Common causes include off-by-one errors in loops, incorrect array size calculations, and using dynamic indices that exceed the array limits during runtime.
How can I troubleshoot this error in my code?
To troubleshoot, check the index values being used to access the array, ensure they are within the valid range, and review any loops or calculations that determine these indices.
What programming languages commonly encounter this error?
This error is prevalent in languages such as C, Java, and Python, where array indexing is strictly enforced and out-of-bounds access is not allowed.
Can this error occur with other data structures?
Yes, similar errors can occur with other data structures, such as lists or collections, when attempting to access elements using invalid indices.
How can I prevent this error in my applications?
To prevent this error, always validate index values before accessing array elements, use bounds-checking methods, and implement error handling to manage exceptions gracefully.
The phrase “the index was outside the bounds of the array” typically refers to an error encountered in programming, particularly in languages that use zero-based indexing, such as C, Java, and Python. This error occurs when a program attempts to access an element of an array using an index that is either negative or exceeds the length of the array. Understanding this error is crucial for developers as it can lead to application crashes or unexpected behavior if not handled appropriately.
One of the main points to consider is that this error emphasizes the importance of validating index values before accessing array elements. Implementing checks to ensure that an index is within the valid range can prevent runtime exceptions and improve the robustness of the code. Additionally, developers should familiarize themselves with the specific indexing rules of the programming language they are using, as different languages may have varying conventions and error handling mechanisms.
Another key takeaway is the significance of debugging practices when dealing with such errors. Utilizing debugging tools and techniques, such as breakpoints and logging, can help identify the source of the error quickly. Furthermore, writing unit tests that cover edge cases can also mitigate the risk of encountering this error during production. By prioritizing error handling and testing, developers can enhance the overall quality and reliability of their
Author Profile
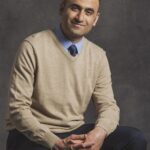
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?