Why Am I Seeing ‘This Declaration Has No Storage Class or Type Specifier?’ – Understanding the Error in C/C++
In the world of programming, particularly in C and C++ languages, encountering errors can be a frustrating yet enlightening experience. One such error that often leaves developers scratching their heads is the cryptic message: “this declaration has no storage class or type specifier.” This seemingly vague notification can halt your coding progress and lead to confusion, especially for those who are new to the intricacies of variable declaration and type management. Understanding the root causes of this error not only enhances your debugging skills but also deepens your grasp of how the language operates at a fundamental level.
When you see this error, it typically signals a problem with how you’ve defined your variables or functions. It may arise from a missing type specifier, such as `int`, `float`, or `char`, or an incorrect declaration of a storage class, such as `static` or `extern`. The implications of this error can range from simple typos to more complex misunderstandings of scope and linkage in your code. By delving into the nuances of variable declarations and the rules governing them, you can learn to identify the sources of these issues and resolve them effectively.
Moreover, this error serves as a reminder of the importance of clarity and precision in programming. As you navigate through the intricacies of C
Understanding the Error Message
When programming in C or C++, you may encounter the error message “this declaration has no storage class or type specifier.” This message typically indicates that the compiler has found a declaration that is incomplete or incorrectly formatted. In essence, the compiler is unable to recognize the type or storage class of the variable, function, or structure being declared.
The storage class specifies the lifetime and visibility of a variable, while the type specifier defines the data type (e.g., `int`, `float`, `char`). If either is missing, the compiler raises this error.
Common Causes of the Error
Several scenarios can lead to this error:
- Omission of Type Specifier: Forgetting to specify the type before a variable name.
- Incorrect Syntax: Misplacing keywords or using invalid identifiers.
- Missing Keywords: Neglecting to include storage class specifiers like `extern`, `static`, or `register`.
- Typographical Errors: Simple typos can lead to declarations that the compiler cannot interpret.
- Incomplete Function Declarations: Declaring a function without its return type.
Examples of Incorrect Declarations
Here are some common examples that trigger this error:
“`c
int main() {
int; // Missing variable name
static; // Missing type or variable name
float 1.0; // Invalid identifier (begins with a digit)
void function() // Missing return type
}
“`
Fixing the Error
To resolve the error, ensure that all declarations are complete and correctly formatted. Here are the key steps:
- Add Type Specifiers: Ensure every variable has a defined type.
- Check Syntax: Review for misplaced semicolons or other punctuation.
- Include Storage Class Specifiers: If required, make sure to include them correctly.
- Correct Typographical Errors: Carefully inspect your code for typos.
Correct Examples
Here are corrected versions of the above examples:
“`c
int main() {
int myVariable; // Correctly defined variable
static int count; // Correctly includes type and storage class
float myFloat = 1.0; // Correct identifier
void function() { // Correct function declaration
// Function implementation
}
}
“`
Summary of Key Points
To avoid the “this declaration has no storage class or type specifier” error, always ensure that your declarations are complete and conform to the expected syntax. Here’s a quick reference table summarizing the key components:
Component | Example | Description |
---|---|---|
Storage Class | static | Defines the lifetime and visibility of a variable. |
Type Specifier | int | Defines the data type of a variable. |
Variable Name | myVariable | The identifier for the variable. |
Understanding the Error Message
The error message “this declaration has no storage class or type specifier” typically occurs in programming languages like C or C++. It indicates that a declaration is missing essential components needed for the compiler to recognize it as a valid statement.
Common reasons for this error include:
- Missing Type Specifier: Variables or functions must have a type defined (e.g., `int`, `float`, `char`).
- Incorrect Syntax: Misplaced semicolons or brackets can lead to confusion regarding the declaration.
- Incomplete Declarations: If a declaration is not fully specified, the compiler cannot infer its type or storage class.
Examples of the Error
Here are some scenarios that might trigger this error:
“`c
int main() {
x; // Error: ‘x’ has no type specifier
return 0;
}
“`
“`c
void function() {
; // Error: empty statement leads to confusion in declaration
}
“`
In both cases, the declarations lack a proper type specifier, which leads to compiler confusion.
Fixing the Error
To resolve the issue, ensure that all declarations are complete and correctly formatted. Here are steps to fix the error:
- Add Type Specifiers: Ensure every variable or function has a defined type. For example:
“`c
int x; // Correctly defined variable
“`
- Check Syntax: Review the code for misplaced symbols or syntax errors. For instance:
“`c
void function() { // Correct function declaration
int y; // Now ‘y’ has a type specifier
}
“`
- Correct Incomplete Declarations: If a declaration is partially complete, finalize it with proper definitions.
Common Scenarios Leading to the Error
Several programming practices can lead to this error. Understanding these scenarios can help in preventing similar mistakes in the future:
Scenario | Example | Fix |
---|---|---|
Missing variable type | `int x; x = 10;` | Ensure `int` is declared before use. |
Misplaced semicolon | `int main();` | Replace with `int main() {}` |
Incorrect function return | `void func;` | Change to `void func() {}` |
Best Practices to Avoid the Error
Adopting best practices in coding can help minimize the occurrence of the error:
- Always Declare Types: Ensure every variable and function has a type specified.
- Maintain Code Structure: Use consistent indentation and spacing to improve readability, which helps in spotting errors.
- Utilize Compiler Warnings: Enable compiler warnings to catch potential issues early in the development process.
- Regular Code Reviews: Collaborate with peers to identify and rectify errors through code reviews.
By adhering to these practices, programmers can effectively reduce the likelihood of encountering the “this declaration has no storage class or type specifier” error, leading to smoother coding experiences.
Understanding the Error: “This Declaration Has No Storage Class or Type Specifier”
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “This error typically arises in C or C++ programming when a variable or function is declared without a proper type or storage class. It’s crucial for developers to ensure that every declaration is accompanied by an appropriate type specifier to maintain code integrity and prevent compilation issues.”
Michael Chen (Lead Compiler Developer, CodeCraft Solutions). “The message ‘this declaration has no storage class or type specifier’ indicates a fundamental oversight in the code structure. Programmers should always define whether a variable is local, static, or external, and specify its type, as this directly influences memory allocation and access within the program.”
Sarah Patel (Programming Language Researcher, Global Tech University). “Understanding the nuances of storage classes and type specifiers is essential for effective programming. This error serves as a reminder that clarity in declarations not only aids in preventing errors but also enhances the readability and maintainability of the codebase.”
Frequently Asked Questions (FAQs)
What does the error “this declaration has no storage class or type specifier” mean?
This error indicates that a variable or function declaration is missing a storage class specifier (such as `static`, `extern`, or `auto`) or a type specifier (like `int`, `float`, or `char`). This leads to ambiguity in the declaration.
How can I resolve the “this declaration has no storage class or type specifier” error?
To resolve this error, ensure that all variable and function declarations include a valid type specifier and, if necessary, a storage class specifier. Review the declaration syntax and correct any missing components.
What are common causes of this error in C or C++ programming?
Common causes include forgetting to declare a variable type, using an incorrect syntax, or misplacing the declaration within the code. Additionally, this error may arise from preprocessor directives that alter the expected declarations.
Can this error occur in other programming languages?
While the specific wording of the error may vary, similar issues can occur in other programming languages that require explicit type declarations. Each language has its own rules regarding variable and function declarations.
What should I check if I encounter this error in a large codebase?
In a large codebase, check for scope issues, misplaced declarations, or missing includes that may affect type visibility. Additionally, verify that all declarations are correctly formatted and follow the language’s syntax rules.
Are there tools available to help identify this error?
Yes, many integrated development environments (IDEs) and static analysis tools can help identify this error. These tools often provide real-time feedback and suggestions for correcting declaration issues.
The error message “this declaration has no storage class or type specifier” typically indicates a problem in C or C++ programming where a variable or function has been declared without specifying its type or storage class. This oversight can lead to compilation errors, as the compiler requires explicit information about how to handle the declared entity. Understanding the context in which this error arises is crucial for debugging and ensuring that code adheres to proper syntax and conventions.
Common causes of this error include missing type keywords such as `int`, `float`, or `char` before variable declarations, or failing to include the necessary storage class specifiers such as `static`, `extern`, or `register`. Developers must ensure that every declaration is complete and follows the language’s syntax rules. This not only aids in preventing compilation issues but also enhances code readability and maintainability.
To resolve this error, programmers should carefully review their code for any declarations that lack the required type or storage class specifier. Utilizing proper coding practices, such as consistently declaring types and following naming conventions, can significantly reduce the likelihood of encountering this error. Additionally, leveraging compiler warnings and code analysis tools can help identify potential issues early in the development process, leading to more robust and error-free code.
Author Profile
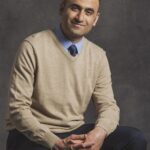
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?