Why Am I Getting a ‘Tuple Object is Not Callable’ Error?
In the world of Python programming, encountering errors is an inevitable part of the learning and development process. Among the myriad of error messages, few can be as perplexing as the “tuple object is not callable” error. This seemingly cryptic message can leave even seasoned developers scratching their heads, as it hints at a deeper issue within the code. Understanding this error is crucial, not only for debugging but also for mastering the nuances of Python’s data structures.
At its core, the “tuple object is not callable” error arises when a tuple—a fundamental data type in Python—is mistakenly treated as a function. This common pitfall often stems from variable naming conflicts or misinterpretations of syntax. As we delve into the intricacies of this error, we will explore how tuples function within Python, the scenarios that lead to this error, and the best practices to avoid it. By grasping the underlying principles, you will enhance your coding skills and improve your ability to troubleshoot effectively.
Join us as we unravel the mystery behind this error, equipping you with the knowledge to navigate Python’s rich landscape with confidence. Whether you are a novice programmer or a seasoned developer, understanding the nuances of tuple behavior will empower you to write cleaner, more efficient code and sidestep
Common Causes of the Error
The error message “tuple object is not callable” typically arises when a tuple is mistakenly treated as a function. This can occur due to various programming pitfalls. Below are some common causes:
- Misnamed Variables: If a variable is named the same as a built-in function or an imported module, it may cause confusion.
- Parentheses Misuse: Using parentheses instead of square brackets can lead to unintended behavior, particularly in indexing or slicing operations.
- Tuple Packing: When packing values into a tuple, if parentheses are used incorrectly, it may lead to the impression that you are trying to call a function.
Examples of the Error
To better understand how this error can occur, consider the following examples:
“`python
Example 1: Misnamed Variables
max = (1, 2, 3)
print(max(2)) Raises TypeError: ‘tuple’ object is not callable
Example 2: Parentheses Misuse
my_tuple = (1, 2, 3)
print(my_tuple(1)) Raises TypeError: ‘tuple’ object is not callable
Example 3: Tuple Packing
def create_tuple():
return (1, 2, 3)
result = create_tuple()
print(result(0)) Raises TypeError: ‘tuple’ object is not callable
“`
How to Fix the Error
To resolve this error, consider the following strategies:
- Rename Variables: Avoid using names that shadow built-in functions or modules. For instance, instead of `max`, use `max_values`.
- Check Parentheses: Ensure that you are using the correct brackets for tuples and lists. Use square brackets `[]` for lists and parentheses `()` for tuples.
- Verify Function Calls: Double-check that you are invoking functions correctly and that you are not accidentally trying to call a tuple.
Best Practices
To minimize the risk of encountering this error, adhere to the following best practices:
- Use Descriptive Variable Names: This helps avoid naming conflicts with built-in functions.
- Consistent Use of Parentheses: Be consistent in your use of parentheses and brackets to avoid confusion.
- Code Reviews: Regularly review code with peers to catch potential issues early.
Summary Table
Cause | Example | Solution |
---|---|---|
Misnamed Variables | max = (1, 2, 3) | Rename variable |
Parentheses Misuse | my_tuple(1) | Use square brackets |
Tuple Packing | result(0) | Check function calls |
Understanding the Error
The error message `tuple object is not callable` typically occurs in Python when you attempt to call a tuple as if it were a function. This can happen due to variable name conflicts or incorrect usage of parentheses.
Common Causes
- Variable Name Conflicts: If you inadvertently assign a tuple to a variable name that shadows a function name, subsequent calls may lead to confusion.
- Parentheses Misuse: Using parentheses when attempting to access elements of a tuple can trigger this error, as Python interprets it as a function call.
Example Scenarios
To illustrate this error, consider the following examples:
Example 1: Variable Name Conflict
“`python
def my_function():
return “Hello, World!”
my_function = (1, 2, 3) Overwrites the function
result = my_function() Raises TypeError: ‘tuple’ object is not callable
“`
Example 2: Incorrect Parentheses Usage
“`python
my_tuple = (1, 2, 3)
value = my_tuple(0) Raises TypeError: ‘tuple’ object is not callable
“`
How to Troubleshoot
When you encounter this error, follow these troubleshooting steps:
- Check for Variable Shadowing:
- Ensure that no variable has been assigned the same name as a function you intend to call.
- Use `print()` to display variable types to help identify conflicts.
- Review Parentheses Usage:
- Inspect your code for any instances where parentheses are used incorrectly with tuples.
- Remember to access tuple elements using square brackets `[]`, not parentheses `()`.
- Use Meaningful Names:
- Opt for descriptive variable names to reduce the likelihood of conflicts.
- For example, instead of naming a tuple `data`, use `data_tuple` or `my_data`.
Best Practices
To avoid encountering the `tuple object is not callable` error, consider the following best practices:
- Naming Conventions:
- Use clear and distinct names for functions and tuples.
- Avoid single-letter variable names, which can lead to confusion.
- Code Organization:
- Structure code into functions and modules to encapsulate logic and reduce variable conflicts.
- Type Checking:
- Implement type checks using `isinstance()` to verify object types before calling functions.
Debugging Tips
When debugging this error, consider the following strategies:
Strategy | Description |
---|---|
Print Type | Use `print(type(variable))` to check the type of variables. |
Traceback Analysis | Examine the traceback for clues about where the error occurs. |
Commenting Out Code | Temporarily comment out sections to isolate the issue. |
Use IDE Features | Utilize IDE features like linting and real-time feedback to catch errors early. |
By adhering to these guidelines and understanding the underlying causes of the `tuple object is not callable` error, you can effectively debug and prevent this issue in your Python projects.
Understanding the ‘Tuple Object is Not Callable’ Error in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘tuple object is not callable’ error typically arises when a tuple is mistakenly used as a function. This can occur if a variable name shadows a built-in function or if parentheses are incorrectly placed. Developers should carefully check their variable assignments and ensure that they are not inadvertently treating tuples as callable objects.”
Mark Thompson (Python Developer, CodeMaster Solutions). “This error is a common pitfall for beginners in Python. It serves as a reminder to differentiate between data structures and functions. When debugging, I recommend using print statements to verify the types of variables at runtime, which can help identify where the confusion arises between tuples and callable functions.”
Sarah Jenkins (Lead Python Instructor, LearnPython Academy). “Understanding the nature of tuples and their immutability is crucial for avoiding this error. When a tuple is defined, it should not be followed by parentheses unless you are accessing its elements. Educating new programmers about the differences between data types is essential to prevent such errors from occurring in their code.”
Frequently Asked Questions (FAQs)
What does the error “tuple object is not callable” mean?
This error indicates that you are trying to call a tuple as if it were a function. In Python, tuples are collections of items and cannot be invoked like functions.
What causes the “tuple object is not callable” error?
The error typically arises when a variable name is reused, shadowing a function name with a tuple. For example, if you assign a tuple to a variable that previously held a function, attempting to call that variable as a function will result in this error.
How can I fix the “tuple object is not callable” error?
To resolve this error, ensure that you are not using a tuple where a function is expected. Check variable names for conflicts and rename tuples if necessary to avoid shadowing function names.
Can you provide an example that causes the “tuple object is not callable” error?
Certainly. Consider the following code:
“`python
def my_function():
return “Hello, World!”
my_function = (1, 2, 3) This shadows the function
result = my_function() This raises the error
“`
In this case, `my_function` is redefined as a tuple, leading to the error when trying to call it.
Is the “tuple object is not callable” error specific to Python?
Yes, this error is specific to Python and occurs due to the language’s handling of callable objects. Other programming languages may have different mechanisms for handling similar situations.
What best practices can prevent the “tuple object is not callable” error?
To avoid this error, use distinct and descriptive variable names, especially when defining functions. Additionally, refrain from reassigning built-in names or function names to other data types, such as tuples.
The error message “tuple object is not callable” typically arises in Python programming when a programmer attempts to call a tuple as if it were a function. This situation occurs when parentheses are mistakenly used with a tuple, which leads to confusion between function calls and tuple instantiation. Understanding the context in which this error occurs is crucial for debugging and resolving the issue effectively.
One common scenario that leads to this error is when a variable name is reused, particularly if it has been assigned a tuple previously. For instance, if a variable named `my_tuple` is defined as a tuple and later, a function is expected to be called with the same name, Python will throw this error. This highlights the importance of maintaining clear and distinct variable names to avoid such conflicts.
To prevent encountering the “tuple object is not callable” error, developers should adopt best practices in naming conventions and code organization. Utilizing descriptive variable names and avoiding the reuse of names for different data types can significantly reduce the likelihood of this error. Additionally, implementing thorough testing and debugging techniques can help identify and rectify such issues early in the development process.
Author Profile
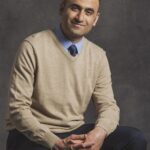
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?