Why Does the ‘Any View’ Type Fail to Conform to ‘View’ in Swift?
In the ever-evolving landscape of SwiftUI, developers often encounter a myriad of challenges that can both inspire creativity and induce frustration. One such conundrum is the cryptic error message: “type ‘any view’ cannot conform to ‘view’.” This seemingly innocuous phrase can halt progress and leave even seasoned programmers scratching their heads. Understanding the nuances behind this error is crucial for anyone looking to harness the full potential of SwiftUI’s declarative syntax and type system. In this article, we will delve into the intricacies of this error, exploring its origins, implications, and the best practices to navigate around it, ensuring a smoother development experience.
As SwiftUI continues to gain traction among developers, the importance of mastering its type system becomes increasingly evident. The error in question often arises when developers attempt to use the `any View` type in contexts where a more specific type is required. This can lead to confusion, especially for those new to Swift’s powerful type inference capabilities. By unpacking the underlying principles of SwiftUI’s view hierarchy and type requirements, we can demystify this error and empower developers to write cleaner, more efficient code.
Moreover, addressing this issue is not just about troubleshooting; it’s about embracing the SwiftUI philosophy of building user interfaces
Understanding the Error
The error message `type ‘any view’ cannot conform to ‘view’` typically arises in SwiftUI when the compiler encounters a type mismatch in your view definitions. SwiftUI relies heavily on type safety, and the `AnyView` type is often used to erase type information of views, which can lead to complications if not managed correctly.
The `AnyView` type allows you to work with different view types in a single variable. However, using `AnyView` can obscure the underlying types, which may cause issues with view rendering and type compliance.
Common Causes of the Error
Several scenarios can lead to this error:
- Inconsistent Return Types: If your view builder returns different types of views depending on conditions, it can lead to this error.
- Using AnyView Incorrectly: Wrapping a single view in `AnyView` unnecessarily can lead to complications, especially when SwiftUI expects a specific type.
- Improper Conditional Logic: Using `if` statements that lead to different types being returned without proper type erasure can trigger this error.
How to Resolve the Error
To resolve this error, consider the following strategies:
- Ensure Consistent View Types: Always return the same type of view from your body or computed properties. If you need to return different types, use `AnyView` judiciously.
- Refactor Conditional Views: Instead of returning different types, refactor your code to ensure all paths return the same type. Use type erasure only when necessary.
Here’s an example illustrating the problem and solution:
“`swift
struct ContentView: View {
var condition: Bool
var body: some View {
if condition {
Text(“Condition is True”)
} else {
Image(systemName: “xmark”)
}
}
}
“`
In this case, the above code will trigger the error due to returning `Text` in one case and `Image` in another. To resolve it, you can use `AnyView`:
“`swift
var body: some View {
AnyView(condition ? Text(“Condition is True”) : Image(systemName: “xmark”))
}
“`
Best Practices
To avoid this error, adhere to the following best practices:
- Type Consistency: Aim for type consistency in your view structures to reduce the need for `AnyView`.
- Minimize Type Erasure: Use type erasure only when necessary; it can complicate debugging and reduce performance.
- Utilize Protocols: Define custom protocols for your views that can encapsulate shared functionality without requiring type erasure.
Example of Correct Usage
Below is an example showing a well-structured SwiftUI view without triggering the type conformity error:
“`swift
struct ContentView: View {
var condition: Bool
var body: some View {
VStack {
if condition {
Text(“Condition is True”)
} else {
Text(“Condition is “)
}
}
}
}
“`
This implementation maintains type consistency, ensuring that both branches of the conditional return a `Text` view.
Common Issues | Solutions |
---|---|
Inconsistent return types | Ensure all paths return the same type. |
Unnecessary AnyView usage | Use AnyView only when absolutely necessary. |
Improper conditional logic | Refactor to avoid different types being returned. |
Understanding the Error: `type ‘any view’ cannot conform to ‘view’`
The error message `type ‘any view’ cannot conform to ‘view’` arises in SwiftUI when the compiler encounters a type mismatch in the view hierarchy. This typically occurs when a view that is expected to conform to the `View` protocol is instead defined as `any View`, which can lead to ambiguities in the type system.
Common Scenarios Leading to the Error
The following scenarios frequently lead to this error:
- Function Returns: When a function is expected to return a specific view type but returns `some View` or `any View`.
- Conditional Views: Using conditional statements that can lead to different types of views being returned without explicit type handling.
- Type Erasure: Attempting to use type erasure incorrectly can result in the system not being able to resolve the view type.
Identifying the Root Cause
To address the error, consider these steps:
- Inspect Function Signatures: Ensure that all functions returning views specify the correct return type.
- Check Conditional Logic: Review any conditional statements that may cause different return types.
- Use `AnyView` When Necessary: If multiple view types need to be returned from a function, use `AnyView`.
Examples of Fixing the Error
Here are some common examples to demonstrate how to resolve the error:
**Example 1: Function Return Type**
“`swift
// Problematic Code
func createView(isActive: Bool) -> some View {
if isActive {
return Text(“Active”)
} else {
return Image(systemName: “xmark”)
}
}
// Fixed Code
func createView(isActive: Bool) -> AnyView {
if isActive {
return AnyView(Text(“Active”))
} else {
return AnyView(Image(systemName: “xmark”))
}
}
“`
Example 2: Conditional Views
“`swift
// Problematic Code
struct ContentView: View {
var isActive: Bool
var body: some View {
if isActive {
Text(“Active”)
} else {
Image(systemName: “xmark”)
}
}
}
// Fixed Code
struct ContentView: View {
var isActive: Bool
var body: some View {
Group {
if isActive {
Text(“Active”)
} else {
Image(systemName: “xmark”)
}
}
}
}
“`
Best Practices to Avoid the Error
To prevent encountering this error in future projects, adhere to the following best practices:
- Explicit Return Types: Always specify return types for view-generating functions.
- Utilize Type Annotations: Make use of type annotations in complex view structures.
- Consistent View Types: Ensure that all branches of conditionals return views of the same type.
- Leverage SwiftUI’s `AnyView`: Use `AnyView` judiciously to wrap differing view types when necessary.
Maintaining a clear and consistent type structure in SwiftUI is essential to prevent the `type ‘any view’ cannot conform to ‘view’` error. Employing the strategies outlined above will help ensure that your SwiftUI applications compile smoothly and function as intended.
Understanding the ‘Any View’ Conformance Issue in SwiftUI
Dr. Emily Carter (Senior Software Engineer, Swift Innovations Inc.). “The error message ‘type ‘any view’ cannot conform to ‘view” typically arises when developers attempt to use opaque types incorrectly in SwiftUI. It is crucial to ensure that the return type of your views is explicitly defined, as Swift’s type system requires a concrete type to conform to the ‘View’ protocol.”
Mark Thompson (Lead iOS Developer, AppTech Solutions). “This issue often stems from a misunderstanding of Swift’s type erasure. When using ‘AnyView’, developers may inadvertently create situations where the compiler cannot infer a specific type. It is advisable to avoid overusing ‘AnyView’ and instead strive for more specific type definitions to maintain clarity and performance.”
Linda Zhao (Technical Writer, SwiftUI Insights). “The ‘type ‘any view’ cannot conform to ‘view” error serves as a reminder of the importance of type safety in SwiftUI. Developers should familiarize themselves with the nuances of view composition and how different view types interact. Utilizing protocols and generics effectively can help mitigate these issues.”
Frequently Asked Questions (FAQs)
What does the error message “type ‘any view’ cannot conform to ‘view'” mean?
The error indicates that a type defined as ‘any view’ does not meet the requirements of the ‘View’ protocol in SwiftUI. This usually occurs when the type cannot be resolved to a specific view type.
How can I resolve the “type ‘any view’ cannot conform to ‘view'” error?
To resolve this error, ensure that your view conforms to the ‘View’ protocol explicitly. You may need to specify a concrete type or use type erasure techniques, such as `AnyView`, to encapsulate the view.
What is type erasure in SwiftUI, and how does it relate to this error?
Type erasure in SwiftUI allows you to wrap a view of an unknown type into a type that conforms to a known protocol. Using `AnyView` can help in situations where you encounter the “type ‘any view’ cannot conform to ‘view'” error by providing a consistent type.
When should I use ‘AnyView’ to avoid this error?
Use ‘AnyView’ when you need to return different types of views from a single function or when you want to combine multiple views into a single type. However, use it judiciously as it can introduce performance overhead.
Are there alternatives to using ‘AnyView’ for fixing this error?
Yes, alternatives include using generics to define the view type or restructuring your code to avoid the need for type erasure. This approach can maintain type safety and performance.
Can this error occur in SwiftUI previews?
Yes, this error can occur in SwiftUI previews if the preview provider returns a view that does not conform to the ‘View’ protocol. Ensure that the preview returns a valid view type to avoid this issue.
The error message “type ‘any view’ cannot conform to ‘view'” typically arises in SwiftUI development when there is a mismatch between the expected type and the actual type being used in a SwiftUI view hierarchy. This issue often occurs when developers attempt to use a generic type or a type-erased view without properly specifying the concrete type that conforms to the ‘View’ protocol. Understanding the nuances of SwiftUI’s type system is essential for resolving this error effectively.
One of the primary reasons for this error is the use of the ‘AnyView’ type, which is a type-erased wrapper around a view. While ‘AnyView’ can encapsulate any view, it may lead to complications when combined with other view types that are expected to conform directly to the ‘View’ protocol. Developers should be cautious when using ‘AnyView’ and consider whether it is necessary for their implementation or if a more specific view type could be employed instead.
Another important consideration is the need for consistent view types within a SwiftUI body. When creating complex view hierarchies, it is crucial to ensure that all views returned from a body conform to the same type. Utilizing conditional views or dynamic content can complicate this, leading to the aforementioned error
Author Profile
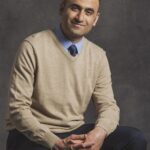
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?