Why Am I Getting a ‘Type’ Object is Not Subscriptable Error?
Have you ever encountered the frustrating error message: “`type’ object is not subscriptable”? If you’re a programmer or someone dabbling in Python, this cryptic phrase can feel like an insurmountable roadblock. It often appears when you’re trying to access elements of a data structure in a way that Python simply doesn’t understand. But fear not! This article will unravel the mystery behind this common error, helping you not only to troubleshoot it effectively but also to deepen your understanding of Python’s data types and their behaviors.
In the world of Python programming, understanding how to manipulate data types is crucial for writing efficient code. The error message “`type’ object is not subscriptable” typically surfaces when you attempt to index or slice an object that isn’t designed to support such operations. This can happen when you mistakenly treat a type as if it were a list, dictionary, or another subscriptable object. By exploring the underlying causes of this error, we can gain insight into the fundamental principles of Python’s type system and how to work with it effectively.
As we delve deeper into the nuances of this error, we will examine common scenarios that lead to its occurrence, practical strategies for debugging, and best practices to avoid similar pitfalls in the future. Whether you’re
Understanding the Error
The error message `type’ object is not subscriptable` typically occurs in Python when you attempt to use indexing or subscripting on a type object rather than an instance of that type. This can happen with built-in types like `int`, `str`, `list`, or even custom classes, and usually indicates a misunderstanding of how these objects are meant to be used.
For example, trying to index a class directly, such as:
“`python
my_value = int[0]
“`
will raise this error because you cannot index the `int` class itself; you need to create an instance of it first.
Common Scenarios Leading to the Error
Several scenarios can trigger this error:
- Incorrect Use of Class Names: Attempting to access elements from a class name rather than an instance.
- Mistaken Variable Types: Assuming a variable is a list or dictionary when it is actually a type.
- Misconfigured Function Returns: Functions intended to return a list or dictionary may inadvertently return a type due to incorrect return statements.
Examples of the Error
Here are a few code examples that illustrate how the error might occur:
“`python
Example 1: Incorrect indexing on a class
class MyClass:
pass
This will raise a TypeError
value = MyClass[0]
Example 2: Mistaken variable type
my_list = list
This will raise a TypeError
element = my_list[0]
Example 3: Function returning a type
def get_type():
return str
This will raise a TypeError
value = get_type()[0]
“`
How to Resolve the Error
To fix the `type’ object is not subscriptable` error, you can follow these strategies:
- Instantiate Objects: Ensure you are working with instances of objects, not the types themselves.
- Check Variable Types: Use the `type()` function to verify that the variable is of the expected type before attempting subscripting.
- Return Correct Objects: Modify functions to ensure they return the correct type (e.g., a list or dictionary) rather than a type object.
Here’s a corrected version of the previous examples:
“`python
Corrected Example 1
instance = MyClass()
Now we can work with instance
Corrected Example 2
my_list = [1, 2, 3] Create a list instance
element = my_list[0] This works
Corrected Example 3
def get_list():
return [“apple”, “banana”, “cherry”]
This now returns a list
value = get_list()[0] Now this works
“`
Debugging Tips
When encountering this error, consider the following debugging tips:
- Use Print Statements: Insert print statements to check the types of variables before subscripting.
- Utilize Type Checking: Implement checks such as `isinstance()` to confirm the variable type before attempting to access elements.
- Review Function Logic: Ensure that functions return the expected objects, and review the control flow to confirm that the correct data types are being used.
Error Source | Description | Solution |
---|---|---|
Class Indexing | Attempting to index a class type directly. | Instantiate the class first. |
Variable Misinterpretation | Assuming a variable is a list when it is a type. | Ensure the variable is an instance of the intended type. |
Function Return Type | Returning a type instead of an instance. | Modify the function to return the correct object. |
Understanding the Error
The error message “`type’ object is not subscriptable`” occurs in Python when you attempt to index or subscript a data type that does not support this operation. Subscriptable objects include lists, tuples, dictionaries, and strings, which can be accessed via an index or key. Non-subscriptable types typically include built-in types like `int`, `float`, and `NoneType`.
Common Causes
- Incorrect Variable Assignment
- Assigning a non-subscriptable type to a variable that is later used as a list or dictionary.
- Example:
“`python
x = int Incorrect assignment
print(x[0]) Raises TypeError
“`
- Using a Class Instead of an Instance
- Attempting to subscript a class directly instead of an instance of the class.
- Example:
“`python
class MyClass:
pass
print(MyClass[0]) Raises TypeError
“`
- Returning a Non-Subscriptable Object from a Function
- Functions that are expected to return a list or dictionary but return an incompatible type.
- Example:
“`python
def get_value():
return None Returns NoneType
value = get_value()
print(value[0]) Raises TypeError
“`
Debugging Strategies
To effectively resolve the “`type’ object is not subscriptable`” error, consider the following debugging strategies:
- Check Variable Types:
- Use the `type()` function to determine the type of the variable you are trying to subscript.
- Trace Function Returns:
- Ensure that functions are returning the expected subscriptable objects.
- Review Class Definitions:
- Verify that you are working with instances of classes rather than the class itself.
- Print Statements:
- Insert print statements before the line causing the error to show the variable values and types.
Example of Debugging
“`python
def get_list():
return [1, 2, 3]
result = get_list()
print(result[0]) Works fine
Incorrect usage
result = None
print(result[0]) Raises TypeError
“`
Preventive Measures
To avoid encountering this error in the future, follow these preventive measures:
- Type Checking:
- Implement type checks using `isinstance()` before subscripting.
- Consistent Data Structures:
- Stick to consistent data structures throughout your code to minimize type-related errors.
- Unit Testing:
- Write unit tests for functions to ensure they return the expected data types and structures.
Preventive Measure | Description |
---|---|
Type Checking | Verify the type of variables before subscripting. |
Consistent Data Structures | Use the same types in similar contexts to reduce errors. |
Unit Testing | Ensure functions behave as expected with automated tests. |
By implementing these strategies, you can minimize the risk of encountering the “`type’ object is not subscriptable`” error in your Python code.
Understanding the ‘Type’ Object is Not Subscriptable Error
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error ‘type’ object is not subscriptable typically arises when a programmer attempts to access elements of a type object as if it were a list or dictionary. This often occurs when a class is mistakenly referred to instead of an instance of that class.”
Michael Chen (Python Programming Instructor, Code Academy). “In Python, this error indicates that you are trying to use indexing on a type that does not support it. It is crucial to ensure that you are working with an instance of a class rather than the class itself when attempting to access its attributes or methods.”
Sarah Thompson (Lead Developer, Open Source Solutions). “Common scenarios leading to this error include misusing built-in types or failing to instantiate a class. Debugging involves checking variable types and ensuring that you are not confusing class definitions with their instances.”
Frequently Asked Questions (FAQs)
What does the error ‘type’ object is not subscriptable mean?
The error ‘type’ object is not subscriptable indicates that you are trying to use indexing or slicing on a type object, which is not allowed in Python. This typically occurs when you attempt to access elements of a type rather than an instance of that type.
What are common scenarios that lead to this error?
Common scenarios include trying to index a class or type directly instead of an instance, such as using `MyClass[0]` instead of creating an instance like `my_instance = MyClass()` and then accessing its attributes or methods.
How can I resolve the ‘type’ object is not subscriptable error?
To resolve this error, ensure that you are working with an instance of the type rather than the type itself. Check your code for any instances where you might be mistakenly using the class name instead of an object.
Can this error occur with built-in types like lists or dictionaries?
Yes, this error can occur with built-in types if you mistakenly reference the type itself instead of an instance. For example, using `list[0]` instead of creating a list instance first will trigger this error.
Is this error specific to Python, or can it occur in other programming languages?
This error is specific to Python, as it relates to Python’s handling of types and instances. Other programming languages may have similar concepts but may not raise the same error message.
What debugging techniques can help identify the source of this error?
To debug this error, review the traceback provided by Python, check variable types using the `type()` function, and ensure that you are accessing elements from instances rather than types. Adding print statements can also help clarify the flow of your code.
The error message “type’ object is not subscriptable” typically arises in Python programming when an attempt is made to index or slice a type object, which is not a valid operation. This error often occurs when a programmer mistakenly tries to access elements of a class or type itself rather than an instance of that class. Understanding the context in which this error appears is crucial for effective debugging and resolution.
Common scenarios that lead to this error include trying to subscript a class instead of an instance, or when using built-in types incorrectly. For example, if a programmer attempts to access elements from a type like `list` or `dict` directly, rather than from an instance of those types, the error will be triggered. This highlights the importance of differentiating between types and instances in Python’s object-oriented programming paradigm.
To prevent this error, developers should ensure that they are working with instances of classes and not the classes themselves. Utilizing proper instance creation and accessing methods or attributes correctly can help avoid such issues. Moreover, thorough testing and debugging practices can assist in identifying the source of the error quickly, allowing for more efficient coding practices.
Author Profile
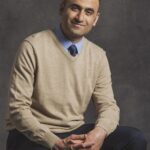
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?