Why Am I Getting a TypeError: ‘float’ Object is Not Subscriptable?
In the world of programming, encountering errors is an inevitable part of the journey. Among the myriad of error messages that can pop up, the `TypeError: ‘float’ object is not subscriptable` stands out as a particularly perplexing one for many developers. This error serves as a reminder of the intricacies of data types and the importance of understanding how they interact within your code. Whether you’re a seasoned programmer or just starting your coding adventure, unraveling the mystery behind this error can enhance your debugging skills and deepen your grasp of Python’s data handling.
At its core, this TypeError arises when you attempt to access an index or a key from a float, which is a data type that simply doesn’t support such operations. This seemingly simple mistake can lead to confusion, especially for those who may not fully appreciate the nuances of Python’s type system. As you delve deeper into the topic, you’ll discover how this error often stems from common coding practices and how a few adjustments can lead to smoother, error-free execution of your programs.
Understanding the `TypeError: ‘float’ object is not subscriptable` not only helps in troubleshooting your code but also fosters a more robust programming mindset. By exploring the underlying causes and potential solutions, you will be better
Understanding the TypeError
The `TypeError: ‘float’ object is not subscriptable` indicates that a float value is being treated as a subscriptable object, like a list or a dictionary. In Python, subscripting refers to the ability to access elements of a collection using an index or a key. This error commonly occurs when you attempt to index or slice a float, which does not support such operations.
Common Causes
- Incorrect Variable Assignment: A variable expected to hold a list or a dictionary might accidentally be assigned a float value.
- Function Return Values: Functions that are supposed to return a collection might instead return a float under certain conditions, leading to this error.
- Data Manipulation Errors: While processing data, especially in loops or conditional statements, a float may be generated and later accessed as if it were a list.
Example Scenario
“`python
value = 3.14
result = value[0] This will raise TypeError
“`
In this example, attempting to access the first element of a float triggers the `TypeError`.
Debugging Techniques
When faced with this error, consider the following debugging techniques:
- Print Variable Types: Use `print(type(variable))` to check the type of the variable in question.
- Traceback Analysis: Examine the full traceback to identify where the erroneous subscript operation occurred.
- Conditional Checks: Implement checks to ensure the variable is of the expected type before attempting to access it.
Example Debugging
“`python
value = 3.14
Check variable type
print(type(value)) Output:
Attempting to subscript
try:
result = value[0]
except TypeError as e:
print(f”Error: {e}”) Output the error message
“`
Prevention Strategies
To minimize the risk of encountering this error, adhere to the following best practices:
– **Type Annotations**: Utilize type hints to ensure that functions return the expected types.
– **Input Validation**: Always validate inputs and outputs, especially when dealing with functions that manipulate data.
– **Consistent Data Structures**: Maintain consistency in the data types used throughout your code to avoid unexpected behaviors.
Type Checking Example
“`python
from typing import Union
def process_data(data: Union[list, float]) -> None:
if isinstance(data, list):
print(data[0]) Safe to subscript
else:
print(“Data is not a list.”)
“`
Handling Floats in Data Structures
When working with data that may include floats, it is crucial to manage how these values are stored and accessed. Consider using structures that can accommodate both floats and subscriptable types.
Data Structure | Description |
---|---|
List | A collection that can hold multiple items, including floats. |
Dictionary | A key-value store where values can be floats or other types. |
Tuple | An immutable sequence that can contain floats alongside other data types. |
In situations where floats are mixed with lists or dictionaries, ensure that the logic accounts for these variations. Use appropriate checks to ascertain the data type before performing operations that assume a subscriptable structure.
Understanding the TypeError
The error message `TypeError: ‘float’ object is not subscriptable` typically occurs in Python when an attempt is made to access an index or key of a float variable as if it were a list, tuple, or dictionary. This confusion arises due to the misconception that the float type can be indexed, leading to code execution errors.
Common Causes
- Incorrect Variable Type Usage:
- Attempting to access an element of a float as if it were an iterable.
- Example:
“`python
my_number = 3.14
print(my_number[0]) This raises a TypeError
“`
- Function Return Mismatch:
- Functions that are expected to return a list or a similar data structure but return a float instead.
- Example:
“`python
def compute_average(numbers):
return sum(numbers) / len(numbers) Returns a float
result = compute_average([1, 2, 3])
print(result[0]) This raises a TypeError
“`
- Data Structure Mismanagement:
- Handling collections where floats are mistakenly treated as containers.
- Example:
“`python
data = [1.0, 2.0, 3.0]
print(data[0][0]) This raises a TypeError
“`
Debugging Steps
To resolve this error, follow these steps:
- Identify the Variable:
- Check the variable causing the error.
- Use the `type()` function to understand its data type.
- Trace the Code:
- Look at the operations leading up to the error.
- Ensure that you are not mistakenly treating a float as an iterable.
- Review Function Returns:
- Ensure that functions return the expected types.
- If a float is not suitable for indexing, modify the function to return a list or tuple when necessary.
Example Scenarios
Scenario | Code Example | Result |
---|---|---|
Incorrect indexing of float | `x = 5.6; print(x[0])` | TypeError |
Function returning float | `def f(): return 3.14; print(f()[0])` | TypeError |
Float in a list as iterable | `arr = [1.1, 2.2]; print(arr[0][0])` | TypeError |
Prevention Tips
- Always check the data types of variables before accessing them via indexing.
- Use assertions or type checks to ensure that functions return expected data types.
- Implement unit tests to catch type errors during the development phase.
Understanding these principles can help in identifying and resolving the `TypeError: ‘float’ object is not subscriptable` efficiently, leading to more robust and error-free code.
Understanding the ‘float’ Object Subscriptability Error in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error message ‘typeerror: ‘float’ object is not subscriptable’ typically arises when a programmer attempts to access an index of a float variable as if it were a list or a dictionary. This misunderstanding often stems from a lack of clarity regarding data types in Python, which can lead to significant debugging challenges.”
Michael Chen (Lead Python Developer, CodeMaster Solutions). “In Python, floats are immutable numeric types and do not support indexing. This error serves as a reminder for developers to ensure they are working with the correct data structures. A common pitfall is mistakenly treating a float as a collection, which can be avoided by implementing type checks before data manipulation.”
Sarah Johnson (Data Scientist, Analytics Hub). “When encountering the ‘float’ object is not subscriptable error, it is crucial to trace back to the source of the data. Often, this issue can be resolved by reviewing the data pipeline to ensure that the intended data structure is being used. Proper validation of input types can significantly reduce the likelihood of such errors.”
Frequently Asked Questions (FAQs)
What does the error ‘float’ object is not subscriptable mean?
The error indicates that you are trying to access an index or key of a float object, which is not possible because floats are not iterable.
When might I encounter a ‘float’ object is not subscriptable error?
This error typically occurs when you mistakenly attempt to index or slice a float variable, often due to a programming oversight or misunderstanding of the data types involved.
How can I fix the ‘float’ object is not subscriptable error?
To resolve this error, ensure you are not trying to index a float. Check your code to confirm that you are working with a list, tuple, or dictionary when attempting to access elements by index or key.
What are common scenarios that lead to this error?
Common scenarios include attempting to access elements from a float variable returned by a function, or mistakenly assigning a float value to a variable that was expected to hold a list or dictionary.
Can this error occur in a specific programming language?
Yes, this error is commonly encountered in Python, where the language enforces strict type handling and indexing rules. Similar errors may appear in other languages with similar type systems.
Is there a way to prevent the ‘float’ object is not subscriptable error?
To prevent this error, always validate the data type of your variables before attempting to index them. Utilize type checking functions or assertions to ensure that you are working with the expected data types.
The error message “TypeError: ‘float’ object is not subscriptable” typically occurs in Python when a programmer attempts to access an index or key of a float variable as if it were a list or dictionary. This indicates a fundamental misunderstanding of data types in Python, where only certain types, such as lists, tuples, and dictionaries, support indexing or subscripting. Understanding this error is crucial for debugging and ensuring that the correct data types are being used in the intended context.
Common scenarios that lead to this error include mistakenly treating a float as a sequence or trying to access an element of a float variable. For example, if a programmer attempts to execute an operation like `my_float[0]`, they will encounter this TypeError because floats do not support indexing. Identifying the source of this error often involves reviewing the code to ensure that the variable in question is indeed a list or another subscriptable type before attempting to access its elements.
To prevent this error, it is essential to maintain a clear understanding of data types and their properties in Python. Developers should implement type-checking mechanisms or use debugging tools to verify the types of variables before performing operations that assume a variable is subscriptable. Additionally, thorough testing and
Author Profile
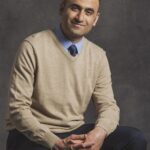
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?