How Can I Resolve the ‘TypeError: ‘float’ object is not subscriptable’ in Python?
In the world of programming, encountering errors is an inevitable part of the development process. Among the myriad of error messages that can pop up, the `TypeError: ‘float’ object is not subscriptable` stands out as a common yet perplexing issue, particularly for those working with Python. This error message can leave developers scratching their heads, especially when they are knee-deep in code and trying to debug a seemingly innocuous snippet. Understanding the root cause of this error is essential for both novice and experienced programmers alike, as it can help streamline the coding process and enhance overall efficiency.
At its core, this TypeError arises when a programmer attempts to access an index or key from a float variable, which is inherently non-subscriptable. This means that while we can easily access elements from lists, tuples, or dictionaries using indices or keys, a float does not support such operations. The implications of this error can range from minor inconveniences to significant roadblocks in the development workflow, making it crucial to grasp the underlying principles that lead to its occurrence.
As we delve deeper into the intricacies of the `TypeError: ‘float’ object is not subscriptable`, we will explore the common scenarios that trigger this error, practical strategies for troubleshooting
Understanding the TypeError
TypeError in Python occurs when an operation or function is applied to an object of inappropriate type. Specifically, the error message “‘float’ object is not subscriptable” indicates that a float value is being treated like a list, tuple, or dictionary, which can be indexed or sliced. In Python, only certain data types support indexing.
Common scenarios that lead to this error include:
- Attempting to access an index of a float variable.
- Using a float in a context that expects a list or string.
- Misconfigured data structures that inadvertently assign float values where sequences are expected.
To illustrate, consider the following example that would raise this error:
“`python
number = 3.14
print(number[0])
“`
This code will produce the TypeError because `number` is a float, and Python does not allow indexing on float types.
Common Causes and Solutions
Understanding the common causes of this error can help in effectively debugging your code. Below are some frequent scenarios:
- Accidental Float Assignment: When performing calculations, ensure that variables intended for indexing are not inadvertently assigned float values.
“`python
index_value = 2.5 This should be an integer for indexing
my_list = [1, 2, 3]
print(my_list[index_value]) TypeError
“`
Solution: Convert the float to an integer if indexing is necessary.
“`python
print(my_list[int(index_value)]) Correct usage
“`
- Function Returns: When a function is expected to return a list but returns a float instead, the error can arise.
“`python
def calculate_average(values):
return sum(values) / len(values)
average = calculate_average([1, 2, 3])
print(average[0]) TypeError
“`
Solution: Ensure the returned data type matches the expected type.
- Data Structure Mismanagement: If you have a data structure that should contain lists but holds floats, this can lead to errors.
“`python
data = [3.14, 2.71]
print(data[0][0]) TypeError
“`
Solution: Verify the contents of your data structure before attempting to index into them.
Best Practices to Avoid TypeError
To prevent encountering a TypeError when dealing with float objects and subscripting, consider adopting the following best practices:
- Type Checking: Use the `isinstance()` function to verify the type of the variable before performing operations.
“`python
if isinstance(variable, list):
print(variable[0])
“`
- Error Handling: Implement try-except blocks to gracefully handle potential TypeErrors.
“`python
try:
print(variable[0])
except TypeError:
print(“Variable is not subscriptable.”)
“`
- Code Reviews: Regularly review code for logical errors, particularly in sections involving type conversions and data structure handling.
- Unit Testing: Implement unit tests to check for expected behavior, particularly when functions return values that will be indexed.
Example Table of Common TypeError Scenarios
Scenario | Code Example | Error Message | Solution |
---|---|---|---|
Indexing a float | print(3.14[0]) | TypeError: ‘float’ object is not subscriptable | Use an integer or convert to a sequence |
Returning float from function | def f(): return 3.14; f()[0] | TypeError: ‘float’ object is not subscriptable | Ensure return type is appropriate |
List containing floats | data = [3.14, 2.71]; data[0][0] | TypeError: ‘float’ object is not subscriptable | Check data structure contents |
Understanding the TypeError
The `TypeError: ‘float’ object is not subscriptable` occurs in Python when you attempt to access an index or a key from a float type variable as if it were a list, tuple, or dictionary. This error signifies a fundamental misunderstanding of data types and their usage.
Common Scenarios Leading to the Error
- Attempting to Index a Float: When you try to access an element of a float variable using square brackets (e.g., `my_float[0]`).
- Mistaking a Float for a List: If a float is expected to be a list or an array but has not been properly initialized or assigned, this error will arise.
- Function Return Values: Functions returning a float instead of a list or other subscriptable types can lead to this error when you attempt to index the output.
Example of the Error
Consider the following code snippet that illustrates the error:
“`python
my_number = 3.14
print(my_number[0]) This will raise TypeError
“`
In this example, `my_number` is a float, and attempting to access `my_number[0]` causes the TypeError.
Troubleshooting Steps
To resolve the `TypeError: ‘float’ object is not subscriptable`, follow these steps:
- Verify Variable Types:
- Use the `type()` function to check the data type of your variable.
- Example:
“`python
print(type(my_number)) Should return
“`
- Check Assignments:
- Ensure that you are assigning the correct data type to your variable.
- If you intended to work with a list or array, ensure you are initializing it properly:
“`python
my_list = [3.14, 2.71]
print(my_list[0]) This is valid
“`
- Review Function Outputs:
- Confirm the return type of any functions you are using. If a function is expected to return a list, make sure it does not return a float due to logic errors.
Handling Data Types Correctly
To avoid this error, it is crucial to understand the data types you are working with:
Data Type | Subscriptable | Example |
---|---|---|
List | Yes | `my_list[0]` |
Tuple | Yes | `my_tuple[1]` |
Dictionary | Yes | `my_dict[‘key’]` |
String | Yes | `my_string[2]` |
Float | No | `my_float[0]` |
Best Practices
- Use Type Annotations: To clarify expected types in function definitions.
- Implement Type Checking: Using `isinstance()` to ensure variables are of expected types before performing operations.
- Employ Try-Except Blocks: To gracefully handle exceptions and provide meaningful error messages.
By adhering to these practices, you can minimize the occurrence of type-related errors in your Python code, fostering clearer and more maintainable codebases.
Understanding the ‘TypeError: float object is not subscriptable’
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error ‘TypeError: float object is not subscriptable’ typically arises when a programmer attempts to access an index of a float variable as if it were a list or a string. This indicates a fundamental misunderstanding of data types in Python, emphasizing the importance of type-checking before attempting to index any variable.”
Michael Thompson (Data Scientist, Analytics World). “In data manipulation, encountering a ‘float’ object being treated as a subscriptable type often signals an error in data preprocessing. It is crucial to ensure that the data structure being worked with is appropriate for the intended operations, especially when dealing with numerical datasets.”
Sarah Lee (Python Developer, CodeMaster Solutions). “This specific TypeError serves as a reminder to developers to maintain clarity in their code. By using clear variable names and implementing type annotations, one can significantly reduce the likelihood of such errors, making the codebase more maintainable and less prone to runtime exceptions.”
Frequently Asked Questions (FAQs)
What does the error ‘float’ object is not subscriptable mean?
The error ‘float’ object is not subscriptable occurs when you attempt to access an index or key of a float variable, which is not a valid operation since floats do not support indexing.
What causes the ‘float’ object is not subscriptable error in Python?
This error typically arises when a float variable is mistakenly treated as a list, dictionary, or string. For example, trying to access a specific element of a float using square brackets will trigger this error.
How can I fix the ‘float’ object is not subscriptable error?
To resolve this error, ensure that you are not attempting to index a float variable. Check your code to confirm that the variable you are trying to subscript is indeed a list, tuple, or string, not a float.
Can you provide an example that triggers the ‘float’ object is not subscriptable error?
Certainly. An example would be:
“`python
number = 3.14
print(number[0]) This will raise the error.
“`
In this case, attempting to access the first index of a float results in the error.
Is it possible to convert a float to a subscriptable type?
Yes, you can convert a float to a string or a list if you need to access its components. For example, converting a float to a string allows you to access its characters:
“`python
number = 3.14
number_str = str(number)
print(number_str[0]) This will output ‘3’.
“`
What should I check if I encounter this error in a larger codebase?
Review the variable assignments and ensure that you are not inadvertently overwriting a list or string with a float. Use debugging techniques, such as printing variable types, to identify where the issue arises.
The error message “TypeError: ‘float’ object is not subscriptable” typically arises in Python programming when an attempt is made to index or slice a float variable as if it were a list, tuple, or string. This indicates a fundamental misunderstanding of data types, as floats are single numerical values that do not support indexing. Understanding the nature of data types in Python is crucial for effective programming and debugging.
To resolve this error, programmers should carefully examine the code to identify where a float is being treated as a subscriptable object. Common scenarios include mistakenly trying to access elements of a float variable or using a float in a context where a list or string is expected. By ensuring that the correct data types are used and that operations are appropriate for those types, developers can prevent this error from occurring.
In summary, the “TypeError: ‘float’ object is not subscriptable” serves as a reminder of the importance of understanding Python’s data types and their limitations. By being mindful of how different data types interact, programmers can write more robust code and avoid common pitfalls. This awareness not only aids in debugging but also enhances overall coding proficiency.
Author Profile
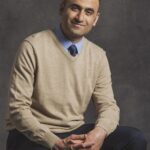
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?