Why Am I Getting a TypeError: ‘NoneType’ Object is Not Callable?
In the dynamic world of programming, encountering errors is an inevitable part of the journey. Among the myriad of error messages that can pop up, few are as perplexing as the `TypeError: ‘NoneType’ object is not callable`. This cryptic message often leaves developers scratching their heads, wondering how a seemingly innocuous piece of code could lead to such a frustrating roadblock. Whether you’re a seasoned programmer or just starting out, understanding this error is crucial for debugging and enhancing your coding skills.
The `TypeError: ‘NoneType’ object is not callable` typically arises when a function or method is mistakenly treated as a callable object, yet it has returned `None`. This can occur for various reasons, such as overwriting a function with a `None` value or failing to return a value from a function altogether. As you delve deeper into the intricacies of Python and its behavior, you’ll uncover the subtle nuances that can lead to this error, empowering you to write cleaner, more efficient code.
In this article, we will explore the common scenarios that trigger this error, dissect the underlying mechanics of Python’s data types, and provide practical strategies for troubleshooting and resolving the issue. By the end, you’ll not only have a clearer understanding of the `
Understanding the Error
The error message `TypeError: ‘NoneType’ object is not callable` typically indicates that a variable that was expected to reference a function is actually pointing to `None`. This often arises from a programming mistake, such as overwriting a function with a `None` value or failing to return a valid function from another function.
Common scenarios where this error may occur include:
- Assigning the result of a function that returns `None` to a variable that is later called as if it were a function.
- Accidentally naming a variable the same as a built-in function, thus shadowing it.
Here’s an example of code that may lead to this error:
“`python
def my_function():
print(“Hello, World!”)
my_function = None Overwriting the function
my_function() This will raise TypeError: ‘NoneType’ object is not callable
“`
Debugging Steps
When faced with this error, a systematic approach to debugging can help identify the root cause:
- Check Variable Assignments: Review where functions are assigned to variables and ensure they are not reassigned to `None` or other non-callable objects.
- Inspect Function Returns: Ensure that functions intended to return callable objects do not accidentally return `None`.
- Use Descriptive Naming: Avoid variable names that are the same as function names to prevent shadowing.
Common Mistakes Leading to the Error
Here are some frequent programming patterns that can lead to encountering this TypeError:
- Reassigning Functions: Assigning `None` to a function reference.
- Missing Return Statements: Forgetting to include a return statement in a function that is supposed to return a callable.
- Misusing Built-in Functions: Overriding built-in functions (like `list`, `str`, etc.) with non-callable variables.
Issue | Example Code | Correction |
---|---|---|
Reassignment | “` my_func = None my_func()“` |
“` my_func = original_function my_func()“` |
Missing Return | “` def faulty(): pass“` |
“` def fixed(): return some_function“` |
Shadowing | “` list = None my_list = list()“` |
“` my_list = original_list_function()“` |
By being aware of these common pitfalls and following a structured debugging process, developers can effectively resolve the `TypeError: ‘NoneType’ object is not callable` error.
Understanding the Error
A `TypeError: ‘NoneType’ object is not callable` typically occurs in Python when you attempt to call a function or method that is actually `None`. This can happen for several reasons:
- Overwriting a Function: If a variable name is assigned the value of `None` or another object, it can unintentionally shadow a function.
- Returning None: A function may return `None`, and if you attempt to call the result of that function, you will encounter this error.
- Using a Method Incorrectly: If you mistakenly try to call an attribute or method that has not been properly defined or initialized.
Common Scenarios for the Error
Identifying the specific scenario that leads to this error can help in troubleshooting effectively. Here are some typical cases:
- Function Name Clashing: If you define a function and then later assign `None` to the same name, any subsequent calls will fail.
“`python
def my_function():
return “Hello”
my_function = None Function is now overwritten
my_function() Raises TypeError
“`
- Returning None from a Function: If a function is expected to return a callable but instead returns `None`, calling its result will lead to the error.
“`python
def another_function():
return None
result = another_function() result is None
result() Raises TypeError
“`
- Incorrect Method Calls: Attempting to call a method that hasn’t been initialized can also produce this error.
“`python
class MyClass:
def __init__(self):
self.method = None
obj = MyClass()
obj.method() Raises TypeError
“`
Troubleshooting the Error
To resolve this error, consider the following steps:
- Check Variable Assignments: Ensure that function names have not been overwritten by variables assigned to `None` or other non-callable objects.
- Review Function Returns: Confirm that all functions expected to return a callable indeed return a valid function object.
- Debugging: Use debugging tools or print statements to track variable types and values leading up to the error.
“`python
print(type(result)) Check the type before calling
“`
- Refactor Code: If necessary, refactor your code to avoid name clashes and ensure clarity in function assignments.
Best Practices to Avoid the Error
To minimize the chances of encountering this error in the future, follow these best practices:
- Use Unique Names: Avoid using the same name for functions and variables.
- Explicit Returns: Always ensure your functions have explicit return statements that return callable objects when necessary.
- Type Hinting: Leverage Python’s type hinting to clarify what types are expected as return values.
Practice | Description |
---|---|
Unique Naming | Use distinct names for functions and variables. |
Explicit Returns | Ensure functions return expected types. |
Type Hinting | Utilize type hints for better code clarity. |
By adhering to these practices, you can significantly reduce the likelihood of encountering the `TypeError: ‘NoneType’ object is not callable` in your Python projects.
Understanding the ‘NoneType’ Call Error in Python
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “The ‘TypeError: ‘NoneType’ object is not callable’ typically arises when a function or method returns None, and you attempt to call it as if it were a function. This often indicates a logical error in the code where a variable expected to hold a callable object is instead set to None.”
James Patel (Lead Python Developer, CodeCraft Solutions). “To resolve this error, developers should carefully trace the flow of their code to identify where a None value is being assigned to a variable that is later invoked. Adding print statements or using a debugger can be effective in pinpointing the exact location of the issue.”
Lisa Tran (Technical Writer, Python Programming Journal). “Understanding the context in which this error occurs is crucial. It often happens in callback functions or when dealing with optional return values. Developers should implement proper checks to ensure that objects are not None before attempting to call them.”
Frequently Asked Questions (FAQs)
What does the error “TypeError: ‘NoneType’ object is not callable” mean?
This error indicates that you are attempting to call a function or method that is actually set to `None`, meaning it has not been defined or initialized properly.
What are common causes of this TypeError?
Common causes include assigning `None` to a variable that is expected to be a callable function, inadvertently overwriting a function name with `None`, or returning `None` from a function that is expected to return another callable.
How can I troubleshoot this error in my code?
To troubleshoot, check the variable assignments and ensure that no function names are being overwritten. Use print statements or debugging tools to trace the flow of your program and identify where the `None` assignment occurs.
Can this error occur in specific programming languages?
Yes, this error can occur in languages such as Python, where the concept of `NoneType` is prevalent. It can also appear in other languages that have similar null or types, though the exact error message may vary.
How can I prevent this error from occurring in the future?
To prevent this error, always initialize your variables properly, avoid shadowing built-in functions, and implement error handling to manage unexpected `None` values gracefully.
What should I do if I encounter this error in a library or framework?
If the error arises from a library or framework, check the documentation for proper usage. Ensure that you are passing the correct parameters and that the functions you are calling are not returning `None`.
The error message “TypeError: ‘NoneType’ object is not callable” typically arises in Python programming when a piece of code attempts to call a function or method that has not been properly defined or has returned a value of `None`. This often occurs when a variable that is expected to hold a function reference instead holds a `None` value, leading to confusion in the code execution. Understanding the context in which this error occurs is crucial for debugging and resolving the issue effectively.
To prevent this error, developers should ensure that functions are correctly defined and that any variables intended to reference functions are not inadvertently assigned a `None` value. Common scenarios that lead to this error include mistakenly overwriting a function name with `None`, returning `None` from a function that is expected to return another callable, or failing to instantiate an object before calling its methods. Proper code organization and thorough testing can mitigate the risk of encountering this error.
In summary, the “TypeError: ‘NoneType’ object is not callable” serves as a reminder of the importance of careful variable management and function handling in Python. By adhering to best practices in coding, such as maintaining clear and distinct naming conventions and implementing robust error handling, developers can enhance the reliability
Author Profile
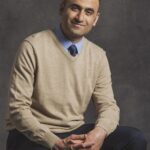
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?