How Can I Resolve the ‘TypeError: ‘NoneType’ Object is Not Iterable’ in My Python Code?
In the world of programming, encountering errors is as common as breathing. Among the myriad of issues developers face, the `TypeError: ‘NoneType’ object is not iterable` stands out as a particularly perplexing conundrum. This error often leaves programmers scratching their heads, wondering how a seemingly innocuous piece of code could lead to such a frustrating outcome. Understanding the nuances of this error is crucial for anyone looking to enhance their coding skills and streamline their debugging process.
This article delves into the intricacies of the `NoneType` error, exploring its origins and the scenarios that typically give rise to it. By unraveling the underlying causes, we aim to equip you with the knowledge to not only identify this error when it arises but also to prevent it from occurring in the first place. Whether you are a seasoned developer or a novice just starting your coding journey, grasping the concept of iterability in Python and the role of `None` can significantly improve your programming acumen.
As we navigate through the common pitfalls and best practices related to this error, you’ll discover practical strategies to enhance your coding efficiency. From understanding the implications of `None` in your functions to learning how to handle data structures effectively, this exploration promises to illuminate the path toward
Understanding the TypeError: ‘NoneType’ Object is Not Iterable
When encountering the `TypeError: ‘NoneType’ object is not iterable`, it is crucial to understand the context in which this error arises. This error typically indicates that a piece of code is attempting to iterate over a variable that has been assigned a value of `None`, rather than a list, tuple, or any other iterable type. In Python, `None` signifies the absence of a value, and trying to iterate over it results in this specific TypeError.
To effectively troubleshoot and resolve this error, consider the following points:
- Identify the Source: Determine where the variable that is causing the issue is being set. This can often involve tracing back through the function calls or logic to find where `None` is being assigned.
- Check Function Returns: If the variable is the result of a function call, ensure that the function is designed to return an iterable. If there are conditional paths in the function, make sure that all paths return an appropriate value.
- Use Debugging Tools: Employ debugging techniques, such as print statements or using a debugger, to inspect the value of the variable before the iteration occurs. This will help to confirm if it is indeed `None`.
Common Scenarios Leading to the Error
Several common scenarios can lead to encountering this error:
- Uninitialized Variables: A variable that is expected to hold a list may not have been initialized correctly.
- Conditional Logic: A variable may be assigned `None` in certain conditions, leading to the error when those conditions are met.
- Failed API Calls: If fetching data from an API fails, the returned value might be `None`, which can lead to this error when trying to iterate over the response.
To illustrate these scenarios, refer to the table below:
Scenario | Potential Cause | Solution |
---|---|---|
Uninitialized Variable | Variable not set before usage | Initialize the variable with an empty list: `my_list = []` |
Conditional Logic | Variable assigned `None` under certain conditions | Ensure all conditions lead to a valid iterable return |
API Call Failure | API returns `None` on failure | Check the response before iterating: `if response is not None:` |
Best Practices to Avoid the Error
To mitigate the risk of encountering the `TypeError: ‘NoneType’ object is not iterable`, consider implementing the following best practices:
- Use Default Values: When defining functions, use default values for return statements to ensure that an iterable is always returned.
- Type Checking: Before iterating, check the type of the variable using `isinstance()` to confirm it is an iterable.
- Graceful Error Handling: Implement try-except blocks to handle the potential TypeError gracefully, allowing the program to respond appropriately without crashing.
By following these strategies, developers can significantly reduce the likelihood of encountering this error and enhance the robustness of their code.
Understanding the TypeError
The error message `TypeError: ‘NoneType’ object is not iterable` indicates that a piece of code is attempting to iterate over an object that is `None`, meaning it has no value. This error typically arises in Python when a function that is expected to return an iterable (like a list, tuple, or dictionary) instead returns `None`.
Common Scenarios Leading to This Error
Several programming patterns can lead to this TypeError. Here are some common scenarios:
- Function Returns None: If a function does not explicitly return a value, it defaults to returning `None`.
- Example:
“`python
def my_function():
print(“Hello”)
result = my_function() result is None
for item in result: TypeError occurs here
print(item)
“`
- Data Retrieval from a Dictionary: Attempting to access a key that does not exist can return `None`.
- Example:
“`python
my_dict = {‘a’: 1, ‘b’: 2}
result = my_dict.get(‘c’) result is None
for item in result: TypeError occurs here
print(item)
“`
- Function Calls in Loops: Misusing a function that should return a list or other iterable.
- Example:
“`python
def get_data():
Some condition
return None Should return a list
for item in get_data(): TypeError occurs here
print(item)
“`
Debugging Steps
To resolve this TypeError, consider the following debugging steps:
- Check Function Returns: Ensure all functions that are expected to return an iterable do so explicitly.
- Use Print Statements: Add print statements before the iteration to check if the variable is `None`.
- Conditional Statements: Before iterating, check if the object is `None`.
“`python
if result is not None:
for item in result:
print(item)
“`
- Default Values: Use default values when retrieving data from dictionaries or other data structures.
“`python
result = my_dict.get(‘c’, []) Return empty list if ‘c’ is not found
for item in result:
print(item)
“`
Best Practices to Avoid TypeError
Implementing best practices can help prevent this error from occurring:
- Always Return Values: Ensure functions have a return statement that provides a valid iterable.
- Type Checking: Use `isinstance()` to confirm the type of the returned object before iterating.
- Use Type Hints: Define the expected return types of functions for better code clarity and type checking.
- Exception Handling: Implement try-except blocks to gracefully handle potential errors during iteration.
“`python
try:
for item in result:
print(item)
except TypeError:
print(“Handled TypeError: result is not iterable.”)
“`
Example Code Fix
Here’s an example of how to fix the issue:
“`python
def fetch_data():
Simulate a condition where data might not be available
return None Intentionally causing a potential TypeError
result = fetch_data()
if result is not None:
for item in result:
print(item)
else:
print(“No data to iterate.”)
“`
By following these guidelines and best practices, you can effectively address and prevent the `TypeError: ‘NoneType’ object is not iterable` in your Python projects.
Understanding the ‘NoneType’ Object Error in Python
Dr. Emily Carter (Senior Software Engineer, CodeMaster Solutions). “The ‘TypeError: ‘NoneType’ object is not iterable’ typically arises when a function that is expected to return an iterable, such as a list or tuple, instead returns None. This can often be traced back to a logical error in the code where a return statement is missing or a variable is not properly initialized.”
Michael Thompson (Python Developer, Tech Innovations Inc.). “In my experience, this error is most commonly encountered when working with data that may not be present. Implementing checks to ensure that the data exists before attempting to iterate over it can prevent this error. Using conditional statements can help mitigate the risk of encountering a NoneType issue.”
Sarah Lee (Data Scientist, Analytics Pro). “When debugging this error, it is crucial to trace back through the function calls to identify where None is being returned. Utilizing debugging tools or print statements can help clarify the flow of data and pinpoint the exact location of the issue. Understanding the expected return types of functions is essential for effective debugging.”
Frequently Asked Questions (FAQs)
What does the error ‘TypeError: ‘NoneType’ object is not iterable’ mean?
This error indicates that you are attempting to iterate over an object that is `None`, which is not a valid iterable type in Python. Iteration requires an object like a list, tuple, or string.
What causes a ‘NoneType’ object to be returned?
A function may return `None` if it does not explicitly return a value or if it encounters an issue that prevents it from producing a valid return value. This can occur in functions designed to modify data in place rather than return a new value.
How can I troubleshoot this error in my code?
To troubleshoot, check the function or method that is expected to return an iterable. Ensure that it is not returning `None` due to a missing return statement or an error in logic. Adding print statements or using a debugger can help identify the source of the `None` value.
What are common scenarios where this error occurs?
Common scenarios include trying to loop through the result of a function that may return `None`, accessing elements of a list that was not properly initialized, or using a dictionary method that returns `None` when the key does not exist.
How can I prevent ‘TypeError: ‘NoneType’ object is not iterable’ in my code?
To prevent this error, always ensure that functions return a valid iterable. You can also add checks to verify that the object is not `None` before attempting to iterate over it, using conditions like `if my_variable is not None:`.
Can I handle this error using exception handling in Python?
Yes, you can use try-except blocks to handle this error gracefully. By catching the `TypeError`, you can provide a fallback mechanism or a user-friendly message, thus preventing the program from crashing unexpectedly.
The TypeError: ‘NoneType’ object is not iterable is a common error encountered in Python programming. This error typically arises when a programmer attempts to iterate over a variable that has been assigned a value of None, which is not an iterable object. Understanding the context in which this error occurs is crucial for effective debugging and code optimization. The error often indicates that a function expected to return an iterable, such as a list or a tuple, has instead returned None, leading to confusion and unintended behavior in the code.
To resolve this error, developers should first trace the source of the None value. This involves reviewing the functions and methods that are expected to return iterable objects. It is essential to ensure that all code paths return a valid iterable and to implement appropriate checks or defaults to handle cases where None might be returned. Additionally, utilizing debugging tools or print statements can help identify where the None value is introduced into the program, allowing for more targeted fixes.
Key takeaways from the discussion on this error include the importance of thorough testing and validation of function outputs. Developers should adopt defensive programming practices, such as adding assertions or type checks, to prevent None from being returned when an iterable is expected. Furthermore, understanding the flow of data within a
Author Profile
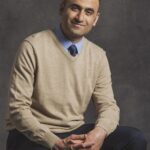
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?