Why Am I Getting a TypeError: Object of Type NoneType Has No Length?
In the world of programming, encountering errors is an inevitable part of the development process. Among the many error messages that can pop up, the `TypeError: object of type ‘NoneType’ has no len()` is particularly notorious for leaving developers puzzled and frustrated. This error typically arises in Python when a programmer attempts to determine the length of an object that is, in fact, `None`. Understanding the underlying causes of this error not only helps in debugging but also enhances overall coding practices. In this article, we will delve into the intricacies of this error, exploring its common triggers and offering strategies to avoid it in your code.
At its core, the `NoneType` error serves as a reminder of the importance of data validation and type checking in programming. When a variable is expected to hold a collection, such as a list or a string, but instead holds `None`, it can lead to unexpected behavior and crashes. This issue often arises from functions that may not return a value or from variables that have not been properly initialized. By examining the scenarios that lead to this error, developers can gain insights into how to structure their code more robustly.
In addressing this error, it is essential to cultivate a mindset that prioritizes error handling and debugging techniques. By implementing checks
Understanding TypeError: Object of Type NoneType Has No Length
When working with Python, encountering a `TypeError` that states “object of type NoneType has no length” typically indicates that you are trying to use the `len()` function on an object that is `None`. This error arises from the attempt to evaluate the length of an object that is not defined or has not been initialized correctly.
In Python, the `None` type is a special type used to signify the absence of a value. It is essential to handle cases where a variable may inadvertently be set to `None` before performing operations that assume the presence of a valid object.
Common Scenarios Leading to the Error
Several common scenarios can lead to this error, including:
- Uninitialized Variables: Attempting to access a variable that has not been assigned a value.
- Function Returns: Functions that do not return a value explicitly will return `None` by default, which can be used in subsequent operations.
- Data Structure Manipulation: Operations that manipulate data structures (like lists or dictionaries) may inadvertently lead to a `None` value being assigned.
Preventative Measures
To prevent encountering this error, it is crucial to implement checks and ensure that variables are initialized properly. Here are some strategies to consider:
- Use Conditional Statements: Before calling `len()`, check if the variable is not `None`.
“`python
if my_var is not None:
length = len(my_var)
else:
print(“Variable is None”)
“`
- Default Values: When defining functions, consider returning an empty list or string instead of `None`.
- Type Annotations: Utilize type hints to indicate expected variable types, making it easier to catch potential issues during development.
Example Scenarios
Below are some examples illustrating how the error can occur and how to resolve it:
Scenario | Code Example | Resolution |
---|---|---|
Uninitialized Variable | len(my_list) | Initialize my_list: my_list = [] |
Function Returns None | result = my_function(); len(result) | Ensure my_function returns a valid object |
Data Manipulation | my_dict.get(‘key’); len(value) | Check if value is None: value = my_dict.get(‘key’, []) |
By understanding the contexts in which the `TypeError: object of type NoneType has no length` can occur and implementing the appropriate checks, developers can write more robust and error-free code.
Understanding the TypeError
The error message “TypeError: object of type ‘NoneType’ has no len()” typically arises in Python when an operation is attempted on a `None` object that is expected to have a length. This can happen in various scenarios, particularly when dealing with data structures or functions that may return `None`.
Common Scenarios Leading to the Error
Several common situations can lead to encountering this error:
- Return Values: A function that is expected to return a list or string may instead return `None`.
- Empty Variables: An uninitialized variable or one that has been explicitly set to `None` is used in a context where its length is queried.
- Data Processing: When processing data from external sources (like files or APIs), if the expected data is missing, the result may be `None`.
Identifying the Source of the Error
To effectively identify where the error is occurring, consider the following steps:
- Check Function Returns:
- Ensure that all functions return expected values, especially lists or strings.
- Example:
“`python
def get_data():
Potentially returns None
return None
data = get_data()
length = len(data) This will raise the TypeError
“`
- Use Debugging Techniques:
- Employ print statements or logging to output variable states before length checks.
- Utilize debuggers to step through code and inspect variable values.
- Implement Type Checks:
- Before checking for length, verify that the variable is not `None`.
- Example:
“`python
if data is not None:
length = len(data)
else:
print(“Data is None”)
“`
Handling the Error Gracefully
To manage and prevent this error effectively, you can use several strategies:
- Default Values: Assign default values to variables that may not be set.
- Error Handling: Use try-except blocks to catch the TypeError and handle it appropriately.
- Assertions: Use assertions to ensure that variables are of the expected type before performing operations.
Example Code Implementation
Below is an illustrative example that demonstrates how to handle the TypeError:
“`python
def process_data(data):
if data is None:
return “No data available”
return len(data)
data = None
result = process_data(data)
print(result) Output: No data available
“`
Conclusion on Error Prevention
To summarize, preventing the “TypeError: object of type ‘NoneType’ has no len()” involves proactive coding practices. By ensuring functions return valid types, using debugging techniques, and implementing robust error handling, developers can minimize the occurrence of this type of error in their applications.
Understanding the TypeError: Object of Type NoneType Has No Length
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The TypeError indicating that an object of type NoneType has no length typically arises when a function or operation attempts to measure the length of a variable that is currently set to None. This often reflects an oversight in the code where a variable was expected to hold a list or string but instead was not initialized properly.”
Michael Chen (Lead Python Developer, CodeCraft Solutions). “To resolve the TypeError related to NoneType, developers should implement checks to ensure that variables are not None before attempting to use functions like len(). Adopting a defensive programming approach can significantly reduce such errors and improve code robustness.”
Sarah Thompson (Data Science Consultant, Insight Analytics). “In data manipulation tasks, encountering a TypeError due to NoneType can indicate issues with data retrieval or processing. It is crucial to validate data inputs and ensure that all expected data structures are correctly populated before performing operations that depend on their length.”
Frequently Asked Questions (FAQs)
What does the error “TypeError: object of type ‘NoneType’ has no len()” mean?
The error indicates that you are trying to determine the length of an object that is `None`. In Python, `NoneType` represents the absence of a value, and it does not have a length.
What causes a variable to be of type ‘NoneType’?
A variable can be of type `NoneType` if it has been explicitly assigned the value `None`, or if a function that is expected to return a value does not return anything, resulting in an implicit return of `None`.
How can I fix the “TypeError: object of type ‘NoneType’ has no len()” error?
To fix this error, ensure that the variable you are checking with `len()` is not `None`. You can add a conditional check before calling `len()` to confirm that the variable contains a valid object.
What are common scenarios where this error occurs?
This error commonly occurs when working with functions that may return `None`, such as searching in a list or retrieving data from a database. It can also happen when trying to access elements of a list or dictionary that do not exist.
How can I debug issues related to ‘NoneType’ in my code?
To debug, use print statements or logging to track the values of your variables before the point of failure. This will help identify where a variable is being set to `None` unexpectedly.
Is ‘None’ a valid input for functions that expect a sequence?
No, `None` is not a valid input for functions that expect a sequence, such as `len()`, `list()`, or `str()`. Always ensure that the input is a valid sequence type before performing operations on it.
The error message “TypeError: object of type ‘NoneType’ has no len()” typically arises in Python programming when an operation attempts to determine the length of an object that is currently set to None. This situation often occurs when a function that is expected to return a list, string, or other iterable types instead returns None due to some logical flaw or oversight in the code. Understanding the context in which this error arises is crucial for debugging and ensuring that functions return the expected types.
One of the primary causes of this error is not handling the return values of functions properly. Developers may overlook scenarios where a function could return None, especially if the function is designed to return a collection or perform a search operation that yields no results. It is essential to implement checks for None before attempting to use functions like len() on the returned value. This proactive approach can prevent the TypeError from occurring and improve the robustness of the code.
Another key takeaway is the importance of thorough testing and validation of function outputs. By incorporating unit tests and assertions, developers can catch potential issues early in the development process. Additionally, using type hints in Python can help clarify the expected return types of functions, making it easier to identify when a None return value is possible
Author Profile
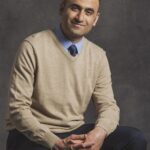
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?