How Can I Resolve the ‘TypeError: Object of Type Tensor is Not JSON Serializable’ Error?
In the world of machine learning and deep learning, the use of tensors has become increasingly prevalent. These multi-dimensional arrays are essential for handling the vast amounts of data that modern algorithms require. However, as developers and researchers dive deeper into their projects, they often encounter various challenges—one of the most perplexing being the infamous `TypeError: object of type tensor is not JSON serializable`. This error can halt progress and lead to frustration, especially when working with data serialization and APIs. Understanding the nuances of this error is crucial for anyone looking to harness the power of tensors in their applications.
At its core, this TypeError arises from the incompatibility between tensor objects and the JSON format, which is widely used for data interchange in web applications. While JSON is excellent for representing simple data types like strings, numbers, and arrays, it struggles to handle more complex structures like tensors directly. This limitation can lead to unexpected roadblocks when developers attempt to convert their tensor data into a JSON-compatible format for storage or transmission.
To navigate this issue effectively, it is essential to grasp the underlying principles of both tensors and JSON serialization. By understanding how to properly convert tensors into a format that can be easily serialized, developers can avoid the pitfalls of this TypeError and ensure smooth data handling in
Understanding the TypeError
The `TypeError: object of type tensor is not JSON serializable` occurs when there is an attempt to convert a PyTorch tensor to JSON format. This error arises because the JSON serialization process does not inherently understand PyTorch tensor objects. JSON supports basic data types like strings, numbers, arrays, and objects, but it lacks the capability to serialize complex data structures like tensors directly.
To resolve this issue, one must convert the tensor into a format that can be serialized, such as a list or a numpy array. Here are the common approaches to handle this error:
- Convert to List: Use the `.tolist()` method of the tensor.
- Convert to Numpy Array: Use the `.numpy()` method if the tensor is on the CPU.
- Custom Serialization: Implement a custom serialization function.
Conversion Methods
The following table outlines the different methods to convert a PyTorch tensor for JSON serialization:
Method | Description | Example Code |
---|---|---|
tolist() | Converts a tensor to a nested list. | tensor.tolist() |
numpy() | Converts a tensor to a numpy array (only if on CPU). | tensor.numpy().tolist() |
Custom Function | Define a function to handle serialization. | json.dumps(tensor, default=custom_serializer) |
Example Code
Here is an example of how to convert a PyTorch tensor into a JSON-serializable format:
“`python
import torch
import json
Create a tensor
tensor = torch.tensor([[1, 2], [3, 4]])
Method 1: Convert to list
tensor_list = tensor.tolist()
json_data = json.dumps(tensor_list)
Method 2: Convert to numpy array then to list
tensor_numpy = tensor.numpy().tolist()
json_data_numpy = json.dumps(tensor_numpy)
Custom serialization
def custom_serializer(obj):
if isinstance(obj, torch.Tensor):
return obj.tolist()
raise TypeError(f”Type {type(obj)} not serializable”)
json_data_custom = json.dumps(tensor, default=custom_serializer)
“`
These methods will ensure that the tensor is converted into a format suitable for JSON serialization, thus preventing the `TypeError` from occurring. By employing these techniques, users can effectively manage tensor data and integrate it with JSON-based workflows.
Understanding the Error
The error message “TypeError: object of type tensor is not JSON serializable” typically arises when attempting to convert a PyTorch tensor into a JSON format. JSON, or JavaScript Object Notation, is a widely-used data interchange format that only supports specific data types, such as strings, numbers, arrays, and objects.
- Tensor Type: A tensor is a multi-dimensional array commonly used in machine learning frameworks like PyTorch.
- Serialization: This process involves converting an object into a format that can be easily stored or transmitted. JSON serialization has limitations that do not include tensors.
Common Causes
Several scenarios may lead to this error:
- Direct Serialization: Attempting to directly serialize a tensor using `json.dumps()`.
- Data Structures: Including tensors within data structures (like dictionaries or lists) that are being serialized.
- Return Values: Returning tensor objects from functions that expect JSON-serializable data.
Solutions to Resolve the Error
To effectively address the issue, consider the following approaches:
- Convert Tensor to List: Before serialization, convert the tensor to a list using `.tolist()`.
“`python
import json
import torch
tensor_data = torch.tensor([1, 2, 3])
json_data = json.dumps(tensor_data.tolist())
“`
- Convert Tensor to Numpy Array: Utilize PyTorch’s `.numpy()` method to convert the tensor to a Numpy array and then to a list.
“`python
json_data = json.dumps(tensor_data.numpy().tolist())
“`
- Use a Custom Encoder: Implement a custom JSON encoder to handle tensor serialization.
“`python
class NumpyEncoder(json.JSONEncoder):
def default(self, obj):
if isinstance(obj, torch.Tensor):
return obj.tolist()
return json.JSONEncoder.default(self, obj)
json_data = json.dumps(tensor_data, cls=NumpyEncoder)
“`
Best Practices
When working with tensors and JSON serialization, adhere to the following best practices:
- Always Check Data Types: Ensure the data type is compatible with JSON serialization.
- Avoid Nesting Tensors: Try to limit the nesting of tensors within complex data structures.
- Use Serialization Libraries: Consider using libraries like `pickle` or `torch.save()` for serializing tensors when JSON is not a requirement.
Example Use Cases
Here are some practical situations where this error may occur and how to handle them:
Scenario | Solution |
---|---|
Directly serializing a tensor | Convert tensor to list before serialization. |
Including tensor in a dict | Ensure each tensor is converted before adding. |
Returning tensors from APIs | Convert tensors to JSON-serializable formats. |
By understanding the limitations of JSON and the nature of PyTorch tensors, you can efficiently navigate and resolve the “TypeError: object of type tensor is not JSON serializable.”
Understanding Tensor Serialization Issues in JSON
Dr. Emily Chen (Data Scientist, AI Innovations Lab). “The error ‘typeerror: object of type tensor is not json serializable’ typically arises when attempting to convert PyTorch tensors directly into JSON format. This is due to the fact that JSON does not natively understand tensor objects, which are specific to libraries like PyTorch. To resolve this, one must first convert the tensor to a format that JSON can serialize, such as a list or a NumPy array.”
Michael Thompson (Software Engineer, Machine Learning Solutions). “When dealing with tensors in Python, it is crucial to remember that serialization requires compatible data types. The ‘typeerror’ indicates that the tensor must be transformed into a JSON-compatible structure. Utilizing the .tolist() method on the tensor before serialization can effectively mitigate this issue.”
Lisa Patel (AI Researcher, Neural Networks Institute). “The challenge of serializing tensors to JSON is a common pitfall in machine learning workflows. It is essential to implement a conversion step that translates tensor data into a serializable format. This not only prevents errors but also ensures that data integrity is maintained during the serialization process.”
Frequently Asked Questions (FAQs)
What does the error “typeerror: object of type tensor is not json serializable” mean?
This error indicates that you are attempting to serialize a PyTorch tensor object into JSON format, which is not directly supported since JSON can only serialize basic data types like strings, numbers, and lists.
How can I convert a PyTorch tensor to a JSON serializable format?
To convert a PyTorch tensor to a JSON serializable format, you can first convert the tensor to a NumPy array using `.numpy()` and then convert that array to a list using `.tolist()`, which can then be serialized to JSON.
What are the steps to handle this error in my code?
To handle this error, ensure that you convert tensors to a compatible format before serialization. Use the following approach: `json.dumps(tensor.numpy().tolist())` to serialize the tensor correctly.
Are there any alternatives to using JSON for serializing PyTorch tensors?
Yes, alternatives include using libraries such as `pickle` or `torch.save()`, which can serialize PyTorch objects directly without conversion, preserving their structure and data types.
Can I customize the serialization process for PyTorch tensors?
Yes, you can create a custom serialization function that checks for tensor types and converts them appropriately before passing them to the JSON encoder, allowing for more control over the serialization process.
What should I do if I encounter this error while using a web framework?
If you encounter this error in a web framework, ensure that any data being sent as JSON is converted to a serializable format before returning it in the response. Implement conversion logic in your view or controller functions.
The error message “typeerror: object of type tensor is not json serializable” typically arises when attempting to convert a PyTorch tensor into a JSON format. JSON, or JavaScript Object Notation, is a widely used data interchange format that supports basic data types such as strings, numbers, arrays, and objects. However, PyTorch tensors are complex objects that cannot be directly serialized into JSON without first converting them into a compatible format, such as a list or a NumPy array.
To resolve this issue, developers can utilize methods provided by PyTorch to convert tensors into a JSON-serializable format. Common approaches include using the `.tolist()` method to convert a tensor into a Python list or employing the `numpy()` method to transform the tensor into a NumPy array before converting it to a list. These conversions ensure that the data structure is compatible with JSON serialization, thus preventing the TypeError from occurring.
Additionally, it is crucial to be aware of the specific context in which this error may arise, particularly when dealing with machine learning models and data processing pipelines. Understanding the data types and their compatibility with JSON can significantly streamline the workflow and enhance the efficiency of data handling. By implementing proper conversions and maintaining awareness of data types,
Author Profile
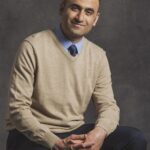
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?