What Does the ‘TypeError: ‘set’ object is not subscriptable’ Mean and How Can You Fix It?
Have you ever encountered a frustrating error message while coding in Python, only to find yourself scratching your head in confusion? One such perplexing error is the infamous `TypeError: ‘set’ object is not subscriptable`. If you’ve stumbled upon this message, you’re not alone; it’s a common pitfall for both novice and seasoned programmers alike. This article will unravel the mystery behind this error, guiding you through its causes, implications, and how to avoid it in your coding endeavors.
Overview
At its core, the `TypeError: ‘set’ object is not subscriptable` error arises when you attempt to access elements in a set using indexing or slicing, much like you would with a list or a tuple. Sets in Python are unordered collections of unique elements, which means they do not support the concept of indexing. This fundamental difference in data structure can lead to unexpected behavior if you’re not aware of how sets operate.
Understanding the nuances of Python data types is crucial for effective programming. As you delve deeper into the world of Python, recognizing when and how to use sets appropriately will not only help you avoid this error but also enhance your coding skills. In this article, we will explore practical examples, common scenarios that trigger this error,
Understanding the TypeError
A `TypeError` in Python occurs when an operation or function is applied to an object of inappropriate type. The specific error message `’set’ object is not subscriptable` indicates that you are trying to access an element of a set using indexing or slicing, which is not supported. Sets in Python are unordered collections that do not support indexing, meaning you cannot retrieve items by their position as you would with lists or tuples.
Common Causes
- Attempting to Index a Set: Trying to use square brackets to access elements in a set.
- Misunderstanding Data Structures: Confusing sets with lists or dictionaries, which do allow indexing.
Example of the Error
“`python
my_set = {1, 2, 3}
print(my_set[0]) This will raise TypeError: ‘set’ object is not subscriptable
“`
In the above example, attempting to access the first element of `my_set` causes the error because sets do not support indexing.
Correcting the Error
To avoid this error, you should use methods appropriate for sets. Here are a few alternatives:
- Convert Set to List: If you need to access elements by index, convert the set to a list.
“`python
my_set = {1, 2, 3}
my_list = list(my_set)
print(my_list[0]) This will print an element from the set
“`
- Iterate Over the Set: If your goal is to process each element, use a loop.
“`python
for item in my_set:
print(item)
“`
Alternative Data Structures
If you frequently need to access elements by index, consider using other data structures. Here’s a comparison of sets and lists:
Feature | Set | List |
---|---|---|
Ordered | No | Yes |
Indexable | No | Yes |
Unique Elements | Yes | No |
Performance on Membership Tests | Faster | Slower |
By carefully choosing the appropriate data structure based on your needs, you can avoid errors and improve the efficiency of your code. Always consider the requirements of your application and the characteristics of the data structures you are using.
Understanding the TypeError
The error message `TypeError: ‘set’ object is not subscriptable` occurs in Python when you attempt to access elements of a set using indexing or slicing. Sets are unordered collections of unique elements and do not support indexing. This is a crucial distinction from other data structures like lists or tuples that do allow for such operations.
Common Scenarios Leading to the Error
Several coding practices can inadvertently lead to this error. Here are some common scenarios:
- Attempting to Access Set Elements by Index: When you try to access an element in a set as if it were a list.
“`python
my_set = {1, 2, 3}
print(my_set[0]) Raises TypeError
“`
- Using Slicing on a Set: Slicing is not applicable to sets due to their unordered nature.
“`python
my_set = {1, 2, 3}
print(my_set[1:2]) Raises TypeError
“`
- Confusion with Other Data Types: Mixing up sets with lists or dictionaries may lead to this error when you assume you can access set elements directly.
“`python
my_set = {1, 2, 3}
print(my_set[‘1’]) Raises TypeError
“`
How to Fix the TypeError
To resolve this error, consider the following approaches depending on your use case:
- Convert the Set to a List: If you need to access elements by index, convert the set to a list.
“`python
my_set = {1, 2, 3}
my_list = list(my_set)
print(my_list[0]) Outputs the first element from the list
“`
- Iterate Over the Set: Use loops to access elements without requiring indexing.
“`python
my_set = {1, 2, 3}
for item in my_set:
print(item) Access elements one by one
“`
- Use Membership Testing: If you are checking for the presence of an item, use the `in` keyword.
“`python
my_set = {1, 2, 3}
if 1 in my_set:
print(“1 is in the set”) Correct way to check membership
“`
Examples of Correct Usage
To illustrate the correct usage of sets, consider the following examples that avoid the TypeError:
Example Type | Code Snippet | Explanation |
---|---|---|
Convert to List | `my_list = list(my_set); print(my_list[0])` | Accessing the first element after conversion. |
Loop Through Set | `for item in my_set: print(item)` | Accessing elements one by one. |
Check Membership | `if 2 in my_set: print(“Found!”)` | Safely checking if an element exists. |
Adhering to these practices will help you avoid the `TypeError: ‘set’ object is not subscriptable` and work effectively with sets in Python.
Understanding the ‘set’ Object TypeError in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “The error ‘TypeError: ‘set’ object is not subscriptable’ arises when developers attempt to access elements of a set using indexing. Unlike lists or tuples, sets are unordered collections and do not support indexing, which is a common source of confusion for those new to Python.”
Michael Tran (Software Engineer, CodeMaster Solutions). “To resolve this error, it is essential to understand the characteristics of sets in Python. When you need to access elements, consider converting the set to a list or using iteration. This approach not only prevents the TypeError but also aligns with Python’s design principles.”
Lisa Chen (Python Educator, LearnPythonNow). “Many beginners encounter the ‘set’ object is not subscriptable error when they mistakenly assume that all collection types in Python behave similarly. Educators should emphasize the differences between sets and other collections to prevent such misunderstandings and promote better coding practices.”
Frequently Asked Questions (FAQs)
What does the error “TypeError: ‘set’ object is not subscriptable” mean?
This error indicates that you are trying to access an element of a set using indexing, which is not supported in Python. Sets are unordered collections and do not support indexing or slicing.
How can I fix the “TypeError: ‘set’ object is not subscriptable” error?
To fix this error, avoid using indexing with sets. Instead, convert the set to a list or use iteration methods to access its elements.
Can I convert a set to a list to access its elements?
Yes, you can convert a set to a list using the `list()` constructor. For example, `my_list = list(my_set)` allows you to access elements using indexing.
What are the common scenarios that lead to this error?
Common scenarios include attempting to access elements of a set directly using an index, such as `my_set[0]`, or when mistakenly treating a set like a list or tuple.
Are there alternatives to using sets that allow indexing?
Yes, if you require indexed access, consider using lists or tuples. These data structures maintain order and support indexing, unlike sets.
What should I remember about the characteristics of a set in Python?
Remember that sets are unordered, mutable collections of unique elements. They do not allow duplicate entries and do not maintain any specific order, which is key to understanding their limitations regarding indexing.
The error message “TypeError: ‘set’ object is not subscriptable” typically arises in Python when a programmer attempts to access elements of a set using indexing or slicing. Sets in Python are unordered collections of unique elements, which means they do not support indexing like lists or tuples. Consequently, any attempt to retrieve an element from a set using a syntax such as `my_set[0]` will lead to this TypeError, as sets do not maintain a specific order of their elements.
To resolve this error, developers should consider using alternative data structures that support indexing, such as lists or tuples, if the need for ordered access is essential. If the intention is to check for membership or to iterate through the elements, using methods like `in` for membership testing or employing loops for iteration is advisable. Understanding the characteristics of different data types in Python is crucial for effective programming and avoiding such errors.
In summary, the “TypeError: ‘set’ object is not subscriptable” serves as a reminder of the unique properties of sets within Python. By recognizing that sets are unordered and do not support indexing, programmers can adapt their code accordingly. This knowledge not only aids in troubleshooting but also enhances overall coding practices by promoting
Author Profile
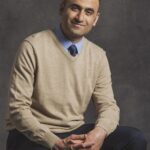
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?