Why Am I Getting a TypeError: ‘set’ Object is Not Subscriptable in Python?
Understanding the TypeError
The error message `TypeError: ‘set’ object is not subscriptable` indicates an attempt to access an element in a set using an index or a key, which is not permissible in Python. A set is an unordered collection of unique items, meaning it does not support indexing or slicing.
Common Causes of the Error
- Indexing a Set: Trying to retrieve an item using an index, like `my_set[0]`.
- Accessing Elements Incorrectly: Using syntax intended for lists or dictionaries on a set.
Example Scenarios
“`python
my_set = {1, 2, 3}
print(my_set[0]) Raises TypeError
“`
How to Fix the Error
To resolve this issue, consider the following alternatives:
- Convert the Set to a List: If you need to access elements by index, convert the set to a list.
“`python
my_set = {1, 2, 3}
my_list = list(my_set)
print(my_list[0]) Accessing first element
“`
- Iterate Over the Set: If your goal is to process each element, use a loop.
“`python
for item in my_set:
print(item) Processes each item in the set
“`
Alternative Data Structures
If indexed access is a frequent requirement, consider using the following data structures:
Data Structure | Characteristics |
---|---|
List | Ordered, allows duplicates, subscriptable |
Tuple | Ordered, immutable, subscriptable |
Dictionary | Key-value pairs, unordered, subscriptable by key |
Additional Considerations
When working with sets, keep in mind the following:
- Unordered Nature: Sets do not maintain the order of elements. Thus, accessing elements by position is inherently flawed.
- Unique Elements: Ensure that all items are unique, as duplicates are automatically removed in a set.
Debugging Tips
When encountering this error:
- Check Variable Types: Use `type(variable)` to confirm if it is a set.
- Examine the Code Context: Look for places where indexing may have been mistakenly applied to a set.
By understanding the nature of sets and the implications of their design, you can avoid common pitfalls and effectively manage data structures in Python.
Understanding the ‘set’ Object TypeError in Python
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “The error ‘typeerror: ‘set’ object is not subscriptable’ arises when a developer attempts to access elements of a set using indexing. Unlike lists or tuples, sets are unordered collections, and therefore do not support indexing or slicing.”
Michael Chen (Python Instructor, Tech Academy). “Many beginners encounter this error when they mistakenly treat a set like a list. It is crucial to understand that sets are designed for membership testing and uniqueness, which is why accessing elements by index is not possible.”
Sarah Johnson (Data Scientist, Analytics Innovations). “To resolve this error, one should convert the set to a list if indexed access is necessary. However, it is important to consider whether the use of a list is appropriate for the intended functionality, given the characteristics of sets.”
Frequently Asked Questions (FAQs)
What does the error “TypeError: ‘set’ object is not subscriptable” mean?
The error indicates that you are trying to access an element of a set using indexing or slicing, which is not supported in Python. Sets are unordered collections and do not support indexing.
How can I fix the “TypeError: ‘set’ object is not subscriptable” error?
To fix this error, you should convert the set to a list or use a loop to iterate through the set elements instead of trying to access them by index.
Can I access elements of a set in Python?
No, you cannot access elements of a set using an index. You can only check for membership, iterate through the set, or convert it to a list or tuple if you need indexed access.
What is the difference between a list and a set in Python?
A list is an ordered collection that allows duplicate elements and supports indexing, while a set is an unordered collection that does not allow duplicates and does not support indexing.
When should I use a set instead of a list?
You should use a set when you need to store unique elements and perform operations like union, intersection, or difference, where the order of elements is not important.
How can I convert a set to a list in Python?
You can convert a set to a list using the `list()` function. For example, `my_list = list(my_set)` will create a list containing all elements from the set.
The error message “TypeError: ‘set’ object is not subscriptable” indicates that an attempt was made to access an element of a set using indexing or slicing, which is not supported in Python. Sets are unordered collections of unique elements, and unlike lists or tuples, they do not maintain a specific order or allow for direct access via indices. This fundamental characteristic of sets is crucial to understanding why this error occurs and how to avoid it in programming.
To resolve this error, developers should recognize that sets should be accessed through iteration or by using membership tests, rather than through indexing. For instance, if a specific element is needed from a set, one can convert the set to a list or iterate through the set using a loop. This approach maintains the integrity of the set’s unique properties while allowing access to its contents in a valid manner.
In summary, the “TypeError: ‘set’ object is not subscriptable” serves as a reminder of the distinct characteristics of different data structures in Python. Understanding these differences is essential for effective programming and debugging. By adhering to the appropriate methods for accessing elements in sets, developers can avoid this common pitfall and write more robust and error-free code.
Author Profile
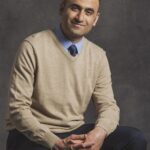
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?