Why Am I Getting a TypeError: ‘str’ Object Cannot Be Interpreted as an Integer?
In the world of programming, few things can be as frustrating as encountering an error message that halts your progress. Among these, the infamous `TypeError: ‘str’ object cannot be interpreted as an integer` stands out as a common yet perplexing issue for both novice and seasoned developers alike. This error often emerges in Python, a language celebrated for its simplicity and readability, leaving many to wonder how a seemingly straightforward operation could lead to such a disruption. Understanding the root causes of this error not only enhances your coding skills but also deepens your grasp of data types and their interactions in Python.
At its core, this TypeError highlights a fundamental aspect of programming: the importance of data types. When Python encounters a situation where it expects an integer but receives a string instead, it raises this error, signaling a mismatch that needs to be addressed. The implications of this error can ripple through your code, affecting everything from loops and indexing to mathematical operations. As you delve deeper into the intricacies of Python, recognizing how and why this error occurs will empower you to write more robust and error-free code.
In the following sections, we will explore the common scenarios that lead to this TypeError, providing clarity on how to identify and resolve it effectively. By examining practical
Understanding the Error
The `TypeError: ‘str’ object cannot be interpreted as an integer` is a common error encountered in Python programming. This error typically arises when there is an attempt to use a string in a context that requires an integer, such as in iterations or indexing. Python is a strongly typed language, meaning that it does not automatically convert data types for you, which can lead to this error if data types are not handled correctly.
For example, consider the following scenarios where this error might occur:
- Using a string in a `range()` function:
“`python
num = “5”
for i in range(num):
print(i)
“`
This code will raise a `TypeError` because `num` is a string and `range()` expects an integer.
- Attempting to index a list with a string:
“`python
my_list = [1, 2, 3]
index = “1”
print(my_list[index])
“`
Here, `my_list[index]` will cause an error because list indices must be integers.
Common Causes of the Error
The `TypeError` can be triggered by various coding mistakes. Below are some typical causes:
- Improper Type Usage: Assigning a string to a variable that is later expected to hold an integer.
- Function Return Types: Functions that return strings instead of integers when their output is used in a numeric context.
- User Input: When taking input from users, data is received as a string, which may need conversion to an integer.
How to Fix the Error
To resolve this error, you will need to ensure that any variables or expressions used in contexts requiring integers are indeed integers. Here are some strategies:
- Convert Strings to Integers: Use the `int()` function to convert strings to integers.
“`python
num = “5”
for i in range(int(num)):
print(i)
“`
- Check Function Outputs: Ensure that functions that are expected to return integers are not returning strings.
- Validate User Input: Use input validation to convert user inputs from strings to integers using `int()` and handle potential exceptions.
“`python
try:
num = int(input(“Enter a number: “))
print(num)
except ValueError:
print(“Invalid input, please enter an integer.”)
“`
Best Practices to Avoid the Error
To prevent encountering this error in the future, consider implementing the following best practices:
- Type Checking: Use `isinstance()` to verify the type of a variable before using it in operations that require a specific type.
- Consistent Data Types: Maintain consistency in data types throughout your code to avoid unexpected errors.
- Utilize Exception Handling: Implement try-except blocks to gracefully handle potential errors.
Common Scenarios | Solution |
---|---|
Using strings in range() | Convert to integer using int() |
Indexing with strings | Ensure index is an integer |
User input as string | Convert input to integer and validate |
Understanding the Error
The error message `TypeError: ‘str’ object cannot be interpreted as an integer` typically occurs in Python when a string type is used in a context that expects an integer. This can happen in various scenarios, particularly when using functions that require numerical input.
Common scenarios that trigger this error include:
- Using Strings in Range Functions: When a string is provided to the `range()` function, which expects integer values.
- Indexing Lists or Strings: Attempting to use a string as an index to access elements in a list or another string.
- Function Arguments: Passing a string to a function that expects an integer argument.
Common Causes
Identifying the exact cause is crucial for troubleshooting. Below are some common situations that lead to this error:
Scenario | Example Code | Explanation |
---|---|---|
Range Function Misuse | `for i in range(‘5’):` | The argument ‘5’ is a string, not an integer. |
List Indexing | `my_list[‘0’]` | Indexing requires an integer, not a string. |
Passing Strings to Functions | `my_function(’10’)` | Function expects an integer, but receives a string. |
How to Fix the Error
To resolve the `TypeError`, ensure that you are passing integers instead of strings. Here are some strategies:
- Convert Strings to Integers: Use the `int()` function to convert string representations of numbers to integers.
“`python
for i in range(int(‘5’)):
print(i)
“`
- Check Input Types: Validate input types before using them in operations that require integers. Use `isinstance()` to ensure the variable is of the expected type.
“`python
if isinstance(my_var, str):
my_var = int(my_var)
“`
- Debugging with Print Statements: Insert print statements to inspect variable types before they are used.
“`python
print(type(my_var)) Check the type before usage
“`
Best Practices to Avoid the Error
Implementing best practices can significantly reduce the likelihood of encountering this error. Consider the following:
- Input Validation: Always validate and sanitize inputs, especially when accepting user data.
- Type Annotations: Utilize Python’s type hints to clarify expected types in function definitions.
- Exception Handling: Implement try-except blocks to gracefully handle potential type errors.
“`python
try:
value = int(input(“Enter a number: “))
except ValueError:
print(“Invalid input; please enter a numeric value.”)
“`
- Consistent Variable Naming: Use clear and consistent names for variables to avoid confusion regarding their types.
By adhering to these practices, you can enhance code reliability and reduce the frequency of type-related errors.
Understanding the ‘str’ Object TypeError in Python
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “The error ‘str’ object cannot be interpreted as an integer typically arises when a string is used in a context that requires an integer, such as in range functions or indexing. It is essential to ensure that data types are correctly matched to avoid such type errors.”
Michael Chen (Python Developer, Tech Innovators Inc.). “To resolve the TypeError involving strings and integers, developers should verify the data type of variables before performing operations. Utilizing functions like int() to convert strings to integers can prevent these runtime errors.”
Sarah Thompson (Data Scientist, Analytics Hub). “Understanding the context in which the error occurs is crucial. Often, this TypeError can be traced back to user inputs or data parsing issues, highlighting the importance of robust input validation in Python applications.”
Frequently Asked Questions (FAQs)
What does the error ‘TypeError: ‘str’ object cannot be interpreted as an integer’ mean?
This error indicates that a string value is being used in a context that requires an integer, such as in a range function or when indexing a list.
What are common scenarios that trigger this TypeError?
Common scenarios include attempting to use a string variable in a `for` loop with `range()`, or passing a string to a function that expects an integer argument.
How can I fix the ‘TypeError: ‘str’ object cannot be interpreted as an integer’ error?
To resolve this error, ensure that the variable being used is converted to an integer using `int()`, or verify that the correct data type is being passed to the function.
Can this error occur in list comprehensions?
Yes, this error can occur in list comprehensions if a string is mistakenly used where an integer is expected, such as in indexing or range specifications.
What should I check if I encounter this error in a loop?
Check the variables being used in the loop, particularly those in `range()` or list indexing, and ensure they are of the correct integer type.
Is it possible to encounter this error when reading input from users?
Yes, user input is typically read as a string. If this input is used directly in a context that requires an integer, it will cause this TypeError. Always convert user input to the appropriate type.
The error message “TypeError: ‘str’ object cannot be interpreted as an integer” typically arises in Python programming when a string is used in a context where an integer is expected. This often occurs in scenarios involving indexing, slicing, or functions that require numerical input. For example, if a programmer attempts to use a string variable to index a list or an array, Python will raise this TypeError, indicating that it cannot interpret the string as an integer. Understanding the context in which this error occurs is crucial for effective debugging and code correction.
To resolve this error, it is essential to ensure that any variable expected to be an integer is indeed of the correct type. This can be achieved by converting strings to integers using the `int()` function, provided that the string represents a valid integer. Additionally, careful attention should be paid to the data types of variables being used in loops, function calls, and other operations where type consistency is critical. By implementing type checks and conversions as necessary, developers can prevent this error from occurring in their code.
In summary, the “TypeError: ‘str’ object cannot be interpreted as an integer” highlights the importance of type management in Python programming. By understanding the contexts in which this error can
Author Profile
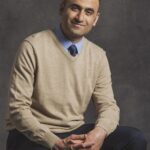
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?