Why Am I Getting a TypeError: String Indices Must Be Integers?
In the world of programming, encountering errors is an inevitable part of the journey. Among the myriad of issues developers face, the `TypeError: string indices must be integers` stands out as a common yet perplexing challenge. This error often surfaces in Python, a language celebrated for its readability and simplicity. However, even the most seasoned programmers can find themselves scratching their heads when this error pops up, disrupting the flow of their code. Understanding the root causes of this error not only enhances your coding skills but also empowers you to write more robust and error-resistant programs.
At its core, the `TypeError: string indices must be integers` error signals a mismatch between expected data types in your code. It typically arises when a programmer attempts to access elements of a string using non-integer indices, leading to confusion and frustration. This seemingly simple mistake can stem from various scenarios, such as mismanaging data structures or misunderstanding how Python handles strings and lists. By delving into the intricacies of this error, you can uncover the underlying principles of Python’s data types and improve your debugging prowess.
As we explore the nuances of this error, we will examine common pitfalls that lead to its occurrence, practical examples to illustrate these situations, and effective strategies to resolve it.
Understanding the TypeError
When working with Python, encountering a `TypeError` indicating “string indices must be integers” is a common issue, particularly for those manipulating string data types. This error typically arises when attempting to access elements of a string using non-integer indices, such as strings or floats.
To illustrate, consider the following code snippet:
“`python
my_string = “Hello”
print(my_string[“0”])
“`
This would raise a `TypeError` because string indices must be integers. Instead, the correct approach would be:
“`python
print(my_string[0]) Output: H
“`
Common Scenarios Leading to TypeError
Several scenarios can lead to this specific `TypeError`:
- Using String as Index: Attempting to use a string to index into a string or a list.
- Data Structure Misinterpretation: Treating strings like dictionaries or lists when they are not.
- Incorrect Function Returns: Functions returning string values instead of expected data types, leading to erroneous indexing attempts.
Examples of TypeError
Here are a few examples that demonstrate this error in action:
Code Snippet | Error Explanation |
---|---|
`my_list = [“a”, “b”, “c”]` `print(my_list[“1”])` |
Indexing with a string instead of an integer. |
`data = {“key”: “value”}` `print(data[“key”][0])` |
Correct usage; prints ‘v’ but could confuse if misused. |
`number = “12345”` `print(number[1.0])` |
Using a float as an index, leading to TypeError. |
How to Fix the TypeError
To resolve the “string indices must be integers” error, follow these guidelines:
- Always use integer indices to access string characters.
- Ensure that any variable used as an index is explicitly cast to an integer if necessary. For example:
“`python
index = “2”
print(my_string[int(index)]) Correctly accesses the third character.
“`
- Validate data types before performing operations that involve indexing.
- When dealing with dictionaries, ensure you are correctly accessing keys and that they are of the appropriate type.
By adhering to these practices, you can minimize the likelihood of encountering this error and ensure smoother execution of your Python programs.
Understanding the TypeError
The error message “TypeError: string indices must be integers” commonly occurs in Python when attempting to access elements of a string using non-integer indices. In Python, strings are sequences of characters, and each character can be accessed using an integer index that indicates its position in the string.
Causes of the Error
- Incorrect Indexing: Attempting to access a string using a string index rather than an integer.
- Data Type Mismatch: Treating a string as a dictionary or list where the expected keys or indices are not integers.
- Iterating Over a String: When iterating over a string, if you mistakenly use a string value as an index, this error can arise.
Example Scenarios
- Accessing Characters:
“`python
my_string = “Hello”
print(my_string[‘H’]) Raises TypeError
“`
In this case, the code attempts to access the string using another string as an index.
- Using Wrong Data Types:
“`python
my_string = “World”
index = “1” index is a string
print(my_string[index]) Raises TypeError
“`
How to Fix the Error
To resolve this error, ensure that all string indices are integers. Here are some common fixes:
- Convert String Indices to Integers:
“`python
my_string = “Example”
index = “2”
print(my_string[int(index)]) Corrected to use int()
“`
- Check Data Types:
Before accessing string indices, verify that the index is an integer:
“`python
my_string = “Sample”
index = 3
if isinstance(index, int):
print(my_string[index])
“`
Debugging Tips
When encountering this error, consider the following debugging strategies:
- Print the Variable Types:
Use the `type()` function to check the data type of your variables.
“`python
print(type(index)) This will help identify if index is a string or integer
“`
- Review Your Logic:
Trace through your logic to ensure that you’re not mistakenly treating a string as a list or dictionary.
Summary of Common Fixes
Error Scenario | Resolution |
---|---|
Using a string as an index | Convert to integer |
Misunderstanding data structures | Check and adjust data types |
Iterating incorrectly | Ensure correct indexing |
By applying these strategies and understanding the underlying causes of the TypeError, you can effectively troubleshoot and resolve issues related to string indexing in Python.
Understanding the TypeError: String Indices Must Be Integers
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘TypeError: string indices must be integers’ typically arises when a string is accessed using a non-integer index. This often occurs when a developer mistakenly treats a string as a dictionary or list. It is crucial to ensure that the data types are correctly identified before attempting to access their elements.”
Michael Thompson (Lead Python Developer, CodeCraft Solutions). “When encountering this error, developers should first check the variable type they are working with. If they expect a dictionary but have a string instead, they need to trace back to where the data is being defined or modified. Debugging tools can be invaluable in pinpointing the source of the issue.”
Sarah Kim (Data Scientist, Analytics Hub). “This error is particularly common in data manipulation tasks, especially when handling JSON data. It is essential to parse the JSON correctly and verify that the expected structures are in place. Misunderstanding the data format can lead to trying to access string elements incorrectly.”
Frequently Asked Questions (FAQs)
What does the error “TypeError: string indices must be integers” mean?
This error indicates that you are trying to access a character in a string using a non-integer index, such as a string or a float, which is not allowed in Python.
When does this error typically occur in Python programming?
This error commonly occurs when you mistakenly treat a string as a dictionary or list, attempting to access its elements using string keys instead of integer indices.
How can I fix the “TypeError: string indices must be integers” error?
To resolve this error, ensure that you are using integer indices when accessing string elements. Verify your code logic to confirm that you are not mistakenly using string keys.
Can this error occur when using JSON data in Python?
Yes, this error can occur when parsing JSON data if you attempt to access string values using keys instead of converting them to dictionaries. Ensure you are correctly handling the data types.
What are some common scenarios that lead to this error?
Common scenarios include iterating over a string and trying to access it as if it were a dictionary, or when mistakenly using a string variable in place of a list or dictionary.
Is there a way to prevent this error from happening in the future?
To prevent this error, always validate the data types before accessing elements. Implement type checks and use debugging tools to trace variable types throughout your code.
The error message “TypeError: string indices must be integers” typically arises in Python programming when there is an attempt to access a character in a string using a non-integer index. Strings in Python are indexed using integers, where each character corresponds to a specific position in the string, starting from zero. This error often indicates a misunderstanding of data types, particularly when a programmer mistakenly treats a string as a dictionary or list, leading to incorrect indexing attempts.
Common scenarios that trigger this error include trying to access elements of a string using another string or a variable that is not an integer. For instance, if a programmer attempts to retrieve a substring using a string variable instead of an integer, the interpreter will raise a TypeError. This highlights the importance of ensuring that the correct data types are utilized in indexing operations to avoid such errors.
To mitigate this issue, it is crucial for developers to carefully check their code for any instances where strings are being accessed. Implementing type checks or using debugging techniques can help identify the source of the error. Additionally, understanding the distinction between different data structures in Python, such as lists, dictionaries, and strings, can prevent similar mistakes in the future. By adhering to best practices in coding and maintaining a clear
Author Profile
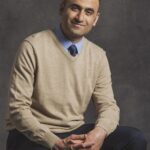
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?