Why Do I Encounter ‘TypeError: String Indices Must Be Integers, Not ‘Str’?’ and How Can I Fix It?
In the world of programming, encountering errors is an inevitable part of the journey. Among the myriad of error messages that developers face, the `TypeError: string indices must be integers, not ‘str’` stands out as a common yet perplexing issue. This error often leaves programmers scratching their heads, particularly those who are new to Python or are transitioning from other programming languages. Understanding the root cause of this error not only aids in debugging but also enhances one’s grasp of Python’s data structures and their appropriate usage.
At its core, this error arises from a fundamental misunderstanding of how to interact with strings and other data types in Python. Strings, being a sequence of characters, can be accessed through integer indices, but when a string index is mistakenly treated as a string itself, the TypeError is triggered. This situation typically occurs in scenarios where developers expect a different data type, such as a dictionary or a list, but mistakenly apply string indexing to a string variable.
As we delve deeper into this topic, we will explore the common scenarios that lead to this error, the underlying principles of Python’s indexing system, and effective strategies for troubleshooting and preventing such issues. By the end of this article, you will not only understand the nuances of this TypeError but
Understanding the TypeError
A `TypeError` in Python often indicates that an operation or function is being applied to an object of an inappropriate type. The specific error message “string indices must be integers, not ‘str'” arises when an attempt is made to access a string using a string index instead of an integer index. This typically occurs when you mistakenly treat a string as if it were a dictionary or a list.
For instance, consider the following code snippet:
“`python
my_string = “Hello, World!”
print(my_string[“H”]) This will raise a TypeError
“`
In this example, the intention may have been to access a character in the string. However, using a string as an index causes the error because Python expects an integer to determine the position of the character.
Common Scenarios Leading to the Error
The error can occur in various scenarios, including:
- Dictionary Access Mistake: Attempting to access a dictionary using a string index while mistakenly using a string variable.
- Improper Data Structure Handling: Treating strings as if they were list-like or dictionary-like without proper conversion or understanding of their types.
- Iteration Errors: Using a loop where the index variable is incorrectly assigned a string value.
Example Cases
Here are some common cases that lead to this error:
“`python
Case 1: Using string indices incorrectly
data = “example”
print(data[“example”]) Raises TypeError
Case 2: Misunderstanding data types
info = {“name”: “Alice”, “age”: 30}
print(info[“name”][“first”]) Raises TypeError, expecting ‘first’ in a string instead of a dictionary
Case 3: Looping through elements
elements = “abc”
for index in elements: index is now ‘a’, ‘b’, ‘c’
print(elements[index]) Raises TypeError
“`
How to Resolve the Error
To resolve this error, you should ensure that you are using integer indices when accessing string elements. Here are some solutions to the common scenarios:
- Correctly index strings: Use integers to index characters in the string.
“`python
print(data[0]) Correctly prints ‘e’
“`
- Validate data types: Before accessing a dictionary, confirm the type of the object to ensure it is indeed a dictionary.
- Use proper iteration: Modify the loop to ensure you are iterating over indices or directly accessing elements as needed.
Here’s a table summarizing the correct ways to access data:
Data Type | Access Method | Correct Example |
---|---|---|
String | Integer Index | my_string[0] |
Dictionary | String Key | my_dict[“key”] |
List | Integer Index | my_list[0] |
By following these guidelines, you can avoid the “string indices must be integers, not ‘str'” TypeError and better understand how to interact with different data types in Python.
Understanding the Error
The `TypeError: string indices must be integers, not ‘str’` occurs in Python when attempting to access a string using a string as an index instead of an integer. Strings in Python are indexed using integers that represent the position of the character within the string.
Common Scenarios Leading to the Error
- Incorrect Access on a String: Attempting to access a string element using another string instead of an integer.
“`python
my_string = “Hello”
print(my_string[‘0’]) Raises TypeError
“`
- Confusion Between Data Types: When a dictionary is expected, but a string is used instead, leading to an attempt to access string elements as if they were dictionary keys.
“`python
my_dict = {‘key’: ‘value’}
print(my_dict[‘key’]) Correct usage
print(my_dict[‘value’]) Raises TypeError if ‘value’ is not a key
“`
Example of the Error
Consider the following code snippet:
“`python
data = “This is a string”
print(data[‘first’]) Raises TypeError
“`
In this case, the attempt to access `data[‘first’]` results in the error because ‘first’ is not a valid index for the string `data`.
How to Fix the Error
To resolve this error, ensure that you are using integer indices when accessing string elements. Here are steps to follow:
- Check Your Data Type: Verify that the variable you are working with is indeed a string when you are using string indices.
- Use Integer Indexing: Access string characters using integer values.
“`python
print(data[0]) Outputs ‘T’
“`
- Debugging Tips:
- Use `type()` to confirm the data type of your variable.
- Utilize print statements to inspect variable contents before accessing them.
Example Correction
If the original intent was to work with a dictionary, correct the code as follows:
“`python
my_data = {‘first’: ‘Hello’, ‘second’: ‘World’}
print(my_data[‘first’]) Outputs ‘Hello’
“`
Preventive Measures
To avoid encountering this error in the future, consider the following practices:
– **Data Validation**: Always validate the data types of variables before performing operations.
– **Descriptive Variable Naming**: Use clear names to indicate whether a variable is expected to be a string or a dictionary.
– **Use of Type Annotations**: Implement type hints in function signatures to clarify expected argument types.
“`python
def process_data(data: dict) -> None:
print(data[‘key’]) Expected dictionary access
“`
By following these practices, you can minimize the chances of encountering the `TypeError: string indices must be integers, not ‘str’` error in your Python code.
Understanding the TypeError: String Indices Must Be Integers
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The TypeError indicating that string indices must be integers arises when a developer attempts to access a character in a string using a string index instead of an integer. This often occurs when working with JSON data, where a dictionary is expected but a string is mistakenly used.”
Mark Thompson (Lead Data Scientist, Data Analytics Group). “This error is particularly common in Python when developers misinterpret the structure of their data. It is crucial to ensure that the data type being accessed is indeed a string or a dictionary, and to verify the expected data structure before performing operations.”
Lisa Nguyen (Python Programming Instructor, Code Academy). “To resolve the TypeError ‘string indices must be integers’, developers should utilize debugging techniques such as print statements or logging to inspect the data types at runtime. Understanding the flow of data and ensuring proper type handling is essential to prevent such errors.”
Frequently Asked Questions (FAQs)
What does the error “TypeError: string indices must be integers, not ‘str'” mean?
This error occurs in Python when you attempt to access a character in a string using a string key instead of an integer index. Strings are indexed numerically, starting from 0.
What are common scenarios that trigger this error?
This error typically arises when you mistakenly treat a string as a dictionary or list. For example, using a string variable with a key access syntax (e.g., `my_string[‘key’]`) will lead to this TypeError.
How can I fix the “TypeError: string indices must be integers, not ‘str'” error?
To resolve this error, ensure you are using integer indices to access characters in a string. If you intend to access a dictionary, verify that you are working with the correct variable type.
Can this error occur when working with JSON data?
Yes, this error can occur if you parse JSON data and then mistakenly try to access string values using string keys instead of accessing them as dictionary items. Always confirm the data type before accessing elements.
What tools or methods can help debug this error?
Utilizing debugging tools such as Python’s built-in `pdb` module or inserting print statements can help identify the variable types and values at runtime, allowing you to trace where the error occurs.
Are there any best practices to avoid this error in Python?
To avoid this error, always validate the data type of variables before accessing them. Use type checking or assertions to ensure you are working with the expected types, especially when dealing with complex data structures.
The error message “TypeError: string indices must be integers, not ‘str'” typically arises in Python programming when an attempt is made to access a string using a string index instead of an integer index. Strings in Python are sequences of characters, and they can only be indexed using integers that represent the position of the character within the string. This error often indicates a misunderstanding of how to manipulate data types, particularly when working with dictionaries or lists that may contain string values.
One common scenario that leads to this error is when a programmer mistakenly tries to access a character in a string using a key that is meant for a dictionary. For example, if a variable is expected to be a dictionary but is actually a string, attempting to use string keys will trigger this TypeError. Understanding the data type being worked with is crucial to resolving this issue. It is important to ensure that the correct data structure is being used and that the appropriate indexing method is applied.
To avoid encountering this error, developers should implement proper type-checking and validation techniques. Utilizing functions such as `isinstance()` can help confirm the type of a variable before attempting to access its elements. Furthermore, thorough debugging practices, including print statements or using debuggers, can assist
Author Profile
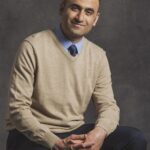
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?