Why Am I Getting a TypeError: Tuple Object is Not Callable?
In the world of programming, particularly in Python, encountering errors is an inevitable part of the learning and development process. One of the more perplexing errors that developers often face is the “TypeError: ‘tuple’ object is not callable.” This error can halt your progress and leave you scratching your head, wondering what went wrong. Understanding the root causes of this error not only aids in debugging your code but also enhances your overall programming skills.
When you see the “TypeError: ‘tuple’ object is not callable,” it typically indicates that somewhere in your code, you are attempting to use a tuple as if it were a function. This can happen due to a variety of reasons, such as naming conflicts, incorrect syntax, or misunderstanding how tuples work in Python. As you delve deeper into the intricacies of Python’s data structures, recognizing the nuances of tuples and their intended use becomes crucial in avoiding such pitfalls.
In this article, we will explore the common scenarios that lead to this error, providing clarity on how to identify and rectify the issue. We will also discuss best practices for working with tuples, ensuring that you can harness their power without falling prey to this frustrating type error. Whether you’re a novice programmer or an experienced developer, understanding this error will empower
Understanding the Error
A `TypeError` in Python occurs when an operation or function is applied to an object of an inappropriate type. Specifically, the message “tuple object is not callable” indicates that there is an attempt to call a tuple as if it were a function. This error often arises from a naming conflict or a misunderstanding of how tuples work in Python.
Tuples are immutable sequences typically used to store collections of heterogeneous data. Unlike lists, they cannot be modified after creation, which is one of their defining characteristics. The error usually surfaces when a variable that holds a tuple is mistakenly invoked as a function.
Common Causes of the Error
Several common scenarios lead to this error:
- Variable Naming Conflict: If you have a variable with the same name as a built-in function or a callable object, Python may confuse the two.
- Misplaced Parentheses: Using parentheses after a tuple can lead to the interpreter attempting to call the tuple.
- Accidental Overwriting of Functions: Assigning a tuple to a variable that previously referenced a function will result in the loss of the callable reference.
Examples of the Error
To illustrate these scenarios, consider the following examples:
“`python
Example 1: Variable Naming Conflict
def my_function():
return “Hello, World!”
Overwriting the function with a tuple
my_function = (1, 2, 3)
This will raise TypeError: ‘tuple’ object is not callable
print(my_function())
“`
“`python
Example 2: Misplaced Parentheses
my_tuple = (1, 2, 3)
Incorrect usage of parentheses
result = my_tuple() Raises TypeError: ‘tuple’ object is not callable
“`
“`python
Example 3: Accidental Overwriting of Functions
def calculate_sum(a, b):
return a + b
Overwriting the function
calculate_sum = (5, 10)
This will raise TypeError: ‘tuple’ object is not callable
print(calculate_sum(5, 10))
“`
How to Fix the Error
Resolving the “tuple object is not callable” error involves identifying the source of the conflict and making appropriate adjustments. Here are steps to consider:
- Check for Variable Names: Ensure that variable names do not conflict with built-in functions or previously defined callable objects.
- Review Tuple Usage: Confirm that you are not mistakenly attempting to call a tuple. Use square brackets for indexing.
- Use Unique Names: When defining variables, use descriptive and unique names to avoid accidental overwriting.
- Debugging: Utilize debugging tools or print statements to trace variable assignments and ensure the expected callable types are in place.
Cause | Example | Solution |
---|---|---|
Variable Naming Conflict | my_function = (1, 2, 3) | Rename the variable to avoid conflict. |
Misplaced Parentheses | my_tuple() | Remove parentheses; use indexing instead. |
Accidental Overwriting | calculate_sum = (5, 10) | Choose a different name for the tuple. |
Understanding the TypeError
A `TypeError` indicating that a “tuple object is not callable” typically arises in Python when an attempt is made to call a tuple as if it were a function. This error can stem from several common mistakes in code.
Common Causes
Identifying the root cause of this error is critical for effective debugging. Here are some prevalent scenarios:
- Misusing Parentheses: Using parentheses instead of brackets when trying to access elements of a tuple.
“`python
my_tuple = (1, 2, 3)
print(my_tuple(0)) TypeError: ‘tuple’ object is not callable
“`
- Variable Overwriting: Assigning a tuple to a variable that was previously a function, leading to a conflict.
“`python
def my_function():
return “Hello”
my_function = (1, 2, 3) Overwriting the function
my_function() TypeError: ‘tuple’ object is not callable
“`
- Incorrect Function Syntax: Attempting to use tuple unpacking or other tuple-related syntax incorrectly.
How to Fix the Error
To resolve the “tuple object is not callable” error, consider the following approaches:
- Check for Variable Overwrites:
- Ensure that no function names are being reused as variable names.
- Rename the variable if necessary to prevent shadowing.
- Use Correct Syntax:
- Always use square brackets `[]` for indexing tuples.
“`python
print(my_tuple[0]) Correct way to access the first element
“`
- Debugging Tips:
- Use print statements to trace variable types and values before the line causing the error.
- Utilize the `type()` function to confirm the expected data type of variables.
Examples of Fixes
Here are examples demonstrating the corrections:
Scenario | Code Before | Code After |
---|---|---|
Misusing Parentheses | `result = my_tuple(1)` | `result = my_tuple[1]` |
Variable Overwriting | `my_function = (1, 2, 3)` | `my_tuple = (1, 2, 3)` |
Incorrect Function Syntax | `result = my_tuple(0, 1)` | `result = (my_tuple[0], my_tuple[1])` |
Best Practices to Avoid TypeError
Implementing best practices can significantly reduce the likelihood of encountering this error:
- Naming Conventions: Use clear and distinct names for functions and variables to avoid clashes.
- Code Reviews: Regularly review your code to catch potential overwrites or misuse of types.
- Type Checking: Consider using type hints and tools like `mypy` to enforce type correctness in your code.
By adhering to these practices, you can enhance your code quality and minimize runtime errors.
Understanding the ‘TypeError: Tuple Object is Not Callable’ in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘TypeError: tuple object is not callable’ typically arises when a tuple is mistakenly treated as a function. This often occurs when parentheses are used instead of square brackets, leading to confusion between function calls and tuple definitions. Careful attention to syntax can prevent this common error.”
Michael Chen (Lead Python Developer, CodeMaster Solutions). “In Python, tuples are immutable sequences, and trying to invoke them as if they were functions will result in a TypeError. Developers should ensure that they are not inadvertently using parentheses when they intend to create or access a tuple, especially in complex expressions.”
Sarah Johnson (Technical Writer, Python Programming Journal). “This error serves as a reminder of the importance of understanding data types in Python. When debugging, it is crucial to verify the type of the object being called. Using the built-in `type()` function can help clarify whether an object is indeed a callable function or just a tuple.”
Frequently Asked Questions (FAQs)
What does the error “TypeError: ‘tuple’ object is not callable” mean?
This error indicates that you are trying to call a tuple as if it were a function. In Python, tuples are immutable sequences, and attempting to invoke them like a callable will result in this TypeError.
What are common scenarios that lead to this error?
Common scenarios include accidentally using parentheses instead of square brackets when accessing tuple elements or mistakenly assigning a tuple to a variable name that shadows a built-in function.
How can I resolve the “TypeError: ‘tuple’ object is not callable” error?
To resolve this error, check your code for any instances where you are using parentheses with a tuple. Ensure you are using square brackets for indexing and verify that you are not overwriting any built-in function names with tuples.
Can this error occur due to variable name conflicts?
Yes, this error can occur if you assign a tuple to a variable name that conflicts with an existing function name. For example, if you create a variable named `list` and then try to call `list()`, Python will raise this TypeError.
Is there a way to prevent this error in my code?
To prevent this error, use meaningful variable names that do not conflict with built-in Python functions. Additionally, regularly review your code for any potential naming conflicts or incorrect usage of parentheses versus brackets.
What should I do if I encounter this error in a third-party library?
If you encounter this error in a third-party library, check the library’s documentation for any known issues or updates. You may also consider reaching out to the library’s support community or reviewing the source code to identify the cause of the error.
The “TypeError: ‘tuple’ object is not callable” is a common error encountered in Python programming, typically arising when a programmer mistakenly attempts to call a tuple as if it were a function. This error often occurs due to variable name conflicts, where a variable intended to hold a function reference is inadvertently overwritten by a tuple. Understanding the nature of this error is crucial for debugging and ensuring that functions and tuples are properly distinguished in code.
One of the key insights from discussions surrounding this error is the importance of maintaining clear and distinct variable names. By avoiding the reuse of names for different data types, programmers can significantly reduce the risk of confusion and errors. Additionally, utilizing tools such as linters can help identify potential variable shadowing issues before they lead to runtime errors.
Moreover, it is essential for developers to adopt a systematic approach to debugging. When encountering a TypeError related to tuples, carefully reviewing the code to trace variable assignments can help pinpoint the source of the issue. This practice not only aids in resolving the immediate problem but also enhances overall coding proficiency and error prevention strategies in future projects.
Author Profile
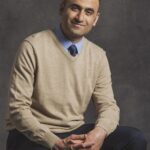
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?