Why Am I Seeing ‘TypeError: Unsupported Data Type: HTTPSConnectionPool’ and How Can I Fix It?
In the ever-evolving landscape of web development and data management, encountering errors can be both frustrating and enlightening. One such error that has puzzled many developers is the `TypeError: unsupported data type: HTTPSConnectionPool`. This cryptic message often emerges when working with HTTP requests in Python, particularly when using libraries like `requests` or `urllib`. Understanding this error is crucial for developers who wish to streamline their applications and ensure seamless data transactions over the web. In this article, we will delve into the intricacies of this error, exploring its causes, implications, and solutions.
Overview
At its core, the `TypeError: unsupported data type: HTTPSConnectionPool` typically signals a mismatch between the expected data types in your code and the actual data types being processed. This error can arise in various scenarios, particularly when making network requests or handling responses from web servers. Developers often encounter it when they inadvertently pass an incorrect data type to a function that requires a specific format, leading to confusion and debugging challenges.
Moreover, the implications of this error extend beyond mere annoyance; they can hinder the functionality of applications that rely on reliable data exchange. Understanding the nuances of this error not only aids in troubleshooting but also enhances a developer’s ability to write robust and error
Understanding the TypeError
A `TypeError` in Python typically indicates that an operation or function has been applied to an object of inappropriate type. In the context of networking, particularly when using libraries like `requests`, the error message `unsupported data type: HTTPSConnectionPool` suggests that the operation is trying to handle data that is incompatible with the expected type.
When working with HTTP connections, it is crucial to ensure that the data types being passed through your functions and methods are compatible with the requirements of those methods. The `HTTPSConnectionPool` refers to the pool of connections maintained for efficient HTTP requests. An unsupported data type error may arise when attempting to pass a connection pool where a different data type is expected.
Common Causes of the Error
Several scenarios may lead to encountering this `TypeError`:
- Incorrect Data Type: If a function expects a string or integer but receives an object like `HTTPSConnectionPool`, this error will occur.
- Improper Configuration: Misconfiguration of the requests or the connection pool can lead to data being sent in an unexpected format.
- Library Version Mismatch: Using incompatible versions of libraries can result in unexpected data types being introduced into your code.
How to Resolve the TypeError
To address the `TypeError: unsupported data type: HTTPSConnectionPool`, you can follow these steps:
- Check Data Types: Ensure that all data being passed to functions is of the correct type. You can use the `type()` function to verify the data type of your variables.
“`python
print(type(my_variable))
“`
- Review Function Signatures: Look at the documentation for the libraries you are using. Make sure you understand what data types are expected by each function.
- Update Libraries: Ensure that your libraries, especially `requests`, are up-to-date. Use package managers like `pip` to check for updates.
“`bash
pip install –upgrade requests
“`
- Debugging: Utilize debugging techniques, such as printing out variable types and values before the line where the error occurs. This can help isolate the source of the problem.
Example of TypeError in Practice
Consider the following example where the error might occur:
“`python
import requests
def fetch_data(url):
response = requests.get(url)
return response.json()
Incorrectly passing an HTTPSConnectionPool object
pool = requests.adapters.HTTPAdapter()
fetch_data(pool)
“`
In this case, passing the `pool` object instead of a valid URL string to `fetch_data` would raise the `TypeError`.
Preventive Measures
To prevent similar errors in your code, follow these best practices:
- Type Annotations: Utilize type annotations to clarify expected data types in function definitions.
- Unit Testing: Implement unit tests to catch type-related issues before deployment.
- Error Handling: Use try-except blocks to gracefully handle exceptions and provide informative error messages.
Best Practice | Description |
---|---|
Type Annotations | Clarifies expected input and output types in functions. |
Unit Testing | Helps identify type issues through automated tests. |
Error Handling | Allows the program to continue running and provides feedback. |
Understanding the Error
A `TypeError: unsupported data type` typically arises in Python when an operation or function receives an argument of an inappropriate type. In the context of `HTTPSConnectionPool`, this error can manifest when working with libraries such as `requests`, which facilitates HTTP requests.
Common scenarios leading to this error include:
- Incorrect Data Types: Sending data types that the library does not support.
- Malformed URLs: Providing an improperly formatted URL to the connection pool.
- Unsupported Payloads: Attempting to send unsupported data formats in requests, like trying to use a custom object where a string or bytes are expected.
Common Causes
Understanding the specific causes of the `TypeError` can help in troubleshooting:
- Invalid URL Format: Ensure that the URL being passed is correct and adheres to standard formatting.
- Data Serialization Issues: When sending JSON data, ensure that the data is serializable (i.e., it can be converted to a JSON string).
- Protocol Mismatch: Using HTTP instead of HTTPS or vice versa can also lead to this error if the server expects a different protocol.
- Library Version Mismatch: Using incompatible versions of libraries like `requests` or `urllib3` can lead to unexpected behavior.
Troubleshooting Steps
To resolve the `TypeError`, consider the following steps:
- Check URL Format:
- Ensure the URL is properly formatted.
- Use `urlparse` from the `urllib.parse` module to validate the URL.
- Inspect Data Types:
- Confirm that the data being sent matches expected types. For example, when posting JSON data, ensure it’s a dictionary.
- Use `json.dumps()` to convert dictionaries to JSON strings where applicable.
- Update Dependencies:
- Ensure all libraries are up-to-date. Use `pip list –outdated` to check for outdated libraries and update them using `pip install –upgrade
`.
- Review Code:
- Go through the portion of the code that initiates the request and check for any discrepancies in data types.
- Example code to check URL:
“`python
from urllib.parse import urlparse
url = “https://example.com/api”
parsed_url = urlparse(url)
if not all([parsed_url.scheme, parsed_url.netloc]):
raise ValueError(“Invalid URL format”)
“`
Example Code Snippet
Here’s an example to illustrate how to handle a request safely, addressing potential causes of the `TypeError`:
“`python
import requests
import json
url = “https://api.example.com/data”
data = {“key”: “value”}
try:
response = requests.post(url, json=data) Correctly using json parameter
response.raise_for_status() Raises an error for bad responses
except requests.exceptions.HTTPError as http_err:
print(f”HTTP error occurred: {http_err}”) Log HTTP errors
except TypeError as type_err:
print(f”Type error occurred: {type_err}”) Log Type errors
except Exception as err:
print(f”An error occurred: {err}”) Catch-all for other exceptions
“`
This snippet ensures that the data is sent in the correct format and handles exceptions gracefully, making it easier to debug issues such as `TypeError`.
Understanding the TypeError: Unsupported Data Type in HTTPS Connections
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error ‘TypeError: unsupported data type: httpsconnectionpool’ typically arises when the data being processed does not conform to the expected types within the HTTPS connection context. It is crucial to ensure that the data structures used in API calls are compatible with the libraries being utilized.”
James Liu (Lead Data Scientist, Cloud Solutions Group). “In many cases, this TypeError can indicate an issue with how the connection pool is being managed, particularly if custom data types are being introduced. Developers should verify that all data types passed to the connection pool are standard and well-defined to avoid such errors.”
Sarah Thompson (DevOps Specialist, SecureNet Technologies). “Resolving the ‘unsupported data type’ error often involves debugging the data being sent through the HTTPS connection. Utilizing logging tools to trace the data flow can help identify where the unsupported types are being introduced, allowing for more efficient troubleshooting.”
Frequently Asked Questions (FAQs)
What does the error “typeerror: unsupported data type: httpsconnectionpool” indicate?
This error typically indicates that there is an issue with the data type being passed to a function or method that expects a different type, specifically related to the handling of HTTP connections.
How can I troubleshoot the “typeerror: unsupported data type: httpsconnectionpool” error?
To troubleshoot this error, check the data types of the variables being used in your HTTP requests. Ensure that you are passing the correct types expected by the libraries or frameworks you are using.
What libraries or frameworks commonly cause this error?
This error is often encountered in Python applications using libraries such as `requests`, `urllib`, or `http.client` when there is a mismatch in expected data types during HTTP connection pooling.
Can this error occur due to incorrect usage of the requests library?
Yes, incorrect usage of the requests library, such as passing an incorrect object type to a function that expects a connection pool or URL, can lead to this type error.
What steps should I take if I encounter this error in a production environment?
In a production environment, first, log the error details to understand the context. Then, review the code for data type mismatches and implement appropriate error handling or type checking before making HTTP requests.
Is there a way to prevent the “typeerror: unsupported data type: httpsconnectionpool” error?
To prevent this error, ensure rigorous type checking and validation of variables before making HTTP requests. Additionally, refer to the documentation of the libraries you are using to understand the expected data types.
The error message “TypeError: unsupported data type: HTTPSConnectionPool” typically indicates a problem with the data types being used in a connection request to a server. This error often arises when the parameters being passed to a function or method do not match the expected types, leading to a failure in establishing a secure connection. It is crucial to ensure that all data types align with the requirements of the libraries and functions being utilized in the code.
Common scenarios that may trigger this error include incorrectly formatted URLs, improper handling of request headers, or issues with the payload being sent in an HTTP request. Developers should verify that the URL is a string, headers are in the correct dictionary format, and any data being sent is appropriately serialized. Additionally, ensuring that the libraries used for making HTTP requests are up to date can mitigate compatibility issues that may lead to this error.
To resolve the “TypeError: unsupported data type: HTTPSConnectionPool,” it is advisable to conduct thorough debugging. This includes reviewing the stack trace to identify where the error originates and checking the types of all variables involved in the connection process. Implementing robust error handling and logging can also provide insights into the data types being processed, which can be invaluable for troubleshooting and ensuring a smooth
Author Profile
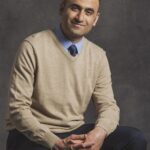
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?