How Can I Rename a Field in TypeScript While Preserving JSDoc Comments?
In the world of TypeScript, maintaining clean and efficient code is paramount, especially when it comes to managing complex data structures. As projects evolve, developers often find themselves needing to rename fields within their interfaces or classes to better reflect their purpose or to adhere to new naming conventions. However, this seemingly straightforward task can lead to the unfortunate loss of valuable documentation if not handled carefully. Enter the challenge: how can developers rename fields while preserving the accompanying JSDoc comments that provide essential context and guidance?
This article delves into the best practices for renaming fields in TypeScript without sacrificing the integrity of your documentation. We’ll explore techniques that allow for seamless transitions, ensuring that your code remains not only functional but also well-documented. Whether you’re working on a small project or a large codebase, understanding how to manage these changes effectively is crucial for maintaining clarity and consistency in your code.
Join us as we navigate the intricacies of TypeScript field renaming, examining tools and strategies that can help you keep your JSDoc comments intact. By the end of this article, you’ll be equipped with the knowledge to make thoughtful adjustments to your code while preserving the invaluable insights that JSDoc provides. Let’s dive in!
Renaming Fields in TypeScript
Renaming fields in TypeScript while preserving JSDoc comments can be a straightforward process if done correctly. The key is to ensure that the JSDoc annotations remain associated with the correct properties after the renaming process.
To rename a field in TypeScript, follow these steps:
- Identify the Field to Rename: Locate the field in your TypeScript interface or class where the JSDoc is associated.
- Update the JSDoc Comment: Before renaming the field, update the JSDoc comment if necessary to reflect the new name.
- Rename the Field: Change the name of the field in the TypeScript code.
- Maintain JSDoc Association: Ensure that the JSDoc remains above the renamed field so that it continues to provide documentation for that property.
Here’s an example of how to rename a field while keeping the JSDoc intact:
“`typescript
/**
- Represents a user in the system.
*/
interface User {
/** The user’s unique identifier */
id: number;
/** The user’s full name */
fullName: string; // Rename this field to ‘name’
}
/**
- Represents a user in the system.
*/
interface User {
/** The user’s unique identifier */
id: number;
/** The user’s name */
name: string; // Updated the field name here
}
“`
Using IDE Features for Renaming
Most modern Integrated Development Environments (IDEs) such as Visual Studio Code or WebStorm offer refactoring tools that can automate the renaming process. These tools ensure that both the field name and its associated JSDoc comments are adjusted accordingly.
Key benefits of using IDE refactoring tools include:
- Automatic Updates: The tool will automatically update all instances of the field throughout your codebase.
- Preservation of JSDoc: The tool typically preserves the associated JSDoc comments without requiring manual adjustment.
- Error Reduction: Reduces the likelihood of introducing errors when renaming fields manually.
Best Practices for Field Renaming
When renaming fields, consider the following best practices to ensure clarity and maintainability:
- Consistency: Maintain consistent naming conventions throughout your codebase.
- Descriptive Names: Choose names that are descriptive and indicate the purpose of the field.
- Review Dependencies: Check for dependencies where the field is used to avoid breaking changes.
Old Field Name | New Field Name | Description |
---|---|---|
fullName | name | The user’s name |
createdAt | dateCreated | Date when created |
isActive | activeStatus | Indicates active status |
By following these practices, you can ensure that renaming fields in your TypeScript code is efficient and maintains clarity in your documentation through JSDoc comments.
Renaming Fields in TypeScript While Preserving JSDoc Comments
Renaming fields in TypeScript can be straightforward, but maintaining JSDoc comments requires specific practices. Here are effective methods to achieve this.
Using TypeScript Type Aliases
TypeScript type aliases can be beneficial when renaming fields. They allow you to create an alias for an existing type without losing JSDoc comments.
“`typescript
/** Original field documentation */
type OriginalType = {
/** This is the old field name */
oldFieldName: string;
};
type NewType = {
/** This is the new field name */
newFieldName: OriginalType[‘oldFieldName’];
};
“`
In this example, the JSDoc comment for `oldFieldName` remains intact while the field is renamed to `newFieldName`.
Using Interfaces for Renaming
When working with interfaces, you can extend the original interface while renaming fields and keeping the JSDoc comments.
“`typescript
/** This interface represents user data */
interface User {
/** The user’s name */
name: string;
}
/** Extending the User interface to rename the field */
interface RenamedUser extends User {
/** The user’s name, now renamed */
username: User[‘name’];
}
“`
This approach allows you to create a new interface that retains the documentation of the original field.
Utility Types for Mapped Types
You can also use TypeScript’s mapped types to rename fields dynamically while preserving documentation.
“`typescript
/** User object with documentation */
type User = {
/** The user’s email address */
email: string;
};
/** Utility type to rename fields */
type RenameField
[P in keyof T as P extends K ? NewK : P]: T[P];
};
/** New type with renamed field */
type RenamedUser = RenameField
“`
This utility type allows for flexibility in renaming while keeping JSDoc comments available for the fields.
Tools and IDE Support
Several tools and IDEs can assist in renaming fields while keeping JSDoc comments intact:
- Visual Studio Code: Offers refactoring options that preserve comments.
- TypeScript Language Server: Integrates with various editors to maintain documentation during renames.
- TSlint/ESLint: Use configuration rules to manage JSDoc comments effectively during code transformations.
Best Practices
- Consistent Documentation: Always keep JSDoc comments updated when renaming fields.
- Refactor with Care: Use IDE features to minimize errors during the renaming process.
- Review Changes: After renaming, review the code to ensure all comments are correctly associated with their respective fields.
By following these methods, you can effectively rename fields in TypeScript while ensuring that your JSDoc comments remain intact and informative.
Best Practices for Renaming Fields in TypeScript While Preserving JSDoc Comments
Dr. Emily Carter (Senior Software Engineer, TypeScript Development Team). “When renaming fields in TypeScript, it is crucial to ensure that JSDoc comments remain intact to maintain code documentation integrity. Utilizing IDE features that support refactoring can help automate this process, preserving the comments associated with the renamed fields.”
Mark Thompson (Lead Developer Advocate, CodeCraft). “In TypeScript, renaming fields while keeping JSDoc comments can be efficiently handled by using TypeScript’s language services. Developers should leverage tools like Visual Studio Code, which automatically updates JSDoc annotations when fields are renamed, ensuring documentation accuracy.”
Linda Zhang (Technical Writer, DevDocs). “It’s essential to document any changes made during the renaming process, especially if JSDoc comments are involved. Clear documentation helps other developers understand the context of changes and ensures that the purpose of the fields is preserved even after renaming.”
Frequently Asked Questions (FAQs)
How can I rename a field in TypeScript while preserving JSDoc comments?
To rename a field in TypeScript while keeping JSDoc comments, you can use the TypeScript language service features in your IDE. Most modern IDEs allow you to rename a symbol and will automatically update references while preserving associated comments.
Is there a specific command in TypeScript to rename a field?
TypeScript itself does not provide a specific command for renaming fields. However, you can use the refactoring tools available in IDEs like Visual Studio Code or WebStorm, which allow you to rename fields and maintain JSDoc comments.
What happens to JSDoc comments when I rename a field manually?
If you rename a field manually without using refactoring tools, the JSDoc comments will remain attached to the old field name. You will need to update the comments manually to reflect the new field name to ensure clarity and accuracy.
Can I use a script to rename fields and preserve JSDoc comments in TypeScript?
Yes, you can write a script using tools like TypeScript Compiler API or AST manipulation libraries (e.g., Babel or TypeScript’s own `ts-morph`) to programmatically rename fields while preserving JSDoc comments. This requires a good understanding of the TypeScript AST.
Are there any best practices for renaming fields in TypeScript?
Best practices include using IDE refactoring tools, ensuring that JSDoc comments are updated accordingly, and running tests after renaming to verify that the functionality remains intact. Additionally, consider documenting the changes in your version control system.
What should I do if the JSDoc comments do not update after renaming?
If the JSDoc comments do not update after renaming, you should manually review and edit the comments to ensure they match the new field name. It’s essential to maintain documentation accuracy to avoid confusion for other developers.
In TypeScript, renaming a field while preserving its associated JSDoc comments is a common task that developers encounter. This process is essential for maintaining code clarity and documentation integrity. When a field is renamed, it is crucial to ensure that any relevant documentation remains attached to the new field name. This practice not only aids in code maintainability but also enhances the understanding of the codebase for future developers.
One effective approach to achieve this is by utilizing TypeScript’s refactoring tools, which allow developers to rename fields seamlessly. These tools often provide options to retain JSDoc comments automatically, ensuring that the documentation is not lost in the renaming process. Additionally, manually copying and pasting the JSDoc comments can be a straightforward solution when automated tools are not available or do not function as expected.
Overall, the ability to rename fields in TypeScript while keeping JSDoc comments intact is vital for preserving the quality of documentation. This practice not only supports better code readability but also fosters collaboration among developers by providing clear context and descriptions of code functionalities. By prioritizing the retention of documentation during refactoring, teams can enhance their development workflow and maintain high standards of code quality.
Author Profile
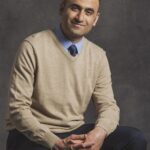
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?