Why Am I Encountering the ‘Undefined Method Exists’ Error in Laravel 11?
If you’ve ventured into the world of Laravel 11, you may have encountered the frustrating error message: ” method ‘exists’.” This common issue can halt your development process and leave you scratching your head, especially if you’re new to the framework or transitioning from an earlier version. Understanding the underlying causes of this error is crucial for any Laravel developer, as it not only affects your current project but also your overall coding proficiency and confidence in using the framework.
In this article, we’ll delve into the reasons why the ‘exists’ method might be in Laravel 11, exploring the nuances of the framework’s evolving architecture. We’ll examine how changes in the ORM (Object-Relational Mapping) and database query builder can lead to this error, and what best practices can help you avoid it in the future. Whether you’re troubleshooting an existing application or seeking to enhance your Laravel skill set, this guide will equip you with the knowledge you need to navigate this common pitfall with ease.
Prepare to uncover the intricacies of Laravel’s methods and how to effectively manage your database queries. By the end of this article, you’ll not only have a clearer understanding of the ‘exists’ method but also gain insights into optimizing your Laravel applications for better performance and reliability. Let’s dive in!
Understanding the ‘exists’ Method in Laravel 11
The error message ” method ‘exists'” in Laravel 11 typically arises when developers attempt to use the `exists` method on an Eloquent model or query builder instance without proper context. This method is used to check for the existence of records in a database, and it can be crucial for optimizing queries and ensuring efficient data handling.
To utilize the `exists` method effectively, it is important to understand its context within Eloquent models. The `exists` method can be called on a query builder instance or directly on a model class.
Common Scenarios Leading to the Error
– **Incorrect Invocation**: The `exists` method must be called on a query builder instance. Calling it on a model that does not have the method defined will trigger the error.
– **Missing Namespace**: If the model class is not properly imported or namespaced, Laravel may not recognize the method call.
– **Database Connection Issues**: Ensure that your database connection is correctly configured. If the connection fails, it may lead to unexpected behavior.
Proper Usage of the ‘exists’ Method
To avoid the ” method ‘exists'” error, here are some proper usage examples:
– **Using the `exists` Method on a Query Builder**:
“`php
use App\Models\User;
$userExists = User::where(’email’, ‘[email protected]’)->exists();
“`
- Using the `exists` Method on a Model:
“`php
use App\Models\User;
$userExists = User::find(1) ? true : ; // Alternative to exists
“`
Troubleshooting Steps
If you encounter this error, consider the following troubleshooting steps:
- Check Method Context: Ensure that the `exists` method is being called on a valid query builder instance.
- Verify Model Import: Confirm that the model is properly imported at the top of the PHP file.
- Review Database Configuration: Check your database configuration in the `.env` file to ensure it is correct.
Example Table of Eloquent Methods
Here is a table that outlines several Eloquent methods related to existence checks:
Method | Description | Return Type |
---|---|---|
exists() | Checks if any records exist that match the query. | bool |
find($id) | Finds a record by its primary key. | Model|null |
first() | Retrieves the first record matching the query. | Model|null |
count() | Counts the number of records that match the query. | int |
By adhering to these guidelines and understanding the context of the `exists` method, developers can effectively manage database queries in Laravel 11 without encountering the ” method ‘exists'” error.
Understanding the ‘exists’ Method in Laravel 11
In Laravel 11, the error ” method ‘exists'” typically arises when the method being called is not available on the object being used. This can occur for various reasons, including namespace issues, incorrect model usage, or misuse of the Eloquent query builder.
Common Causes of the Error
- Model Namespace Issues: Ensure that the model you are referencing is correctly namespaced. If your model is in a different namespace, you must import it correctly.
- Eloquent vs. Query Builder: The `exists` method is part of the Eloquent ORM. If you are using a query builder instance instead of an Eloquent model, this method will not be available.
- Incorrect Method Call: Double-check that you are calling the method on an instance of a model or a query builder that supports it.
Correct Usage of the ‘exists’ Method
The `exists` method is commonly used to check if a record exists in the database. Here’s how it should be used:
“`php
use App\Models\User;
// Checking if a user with a specific ID exists
$userExists = User::where(‘id’, $userId)->exists();
“`
In this example, `exists()` returns a boolean indicating whether the query found any matching records.
Using the ‘exists’ Method with Query Builder
If you are utilizing the query builder, the syntax changes slightly:
“`php
use Illuminate\Support\Facades\DB;
// Checking if a record exists using query builder
$userExists = DB::table(‘users’)->where(‘id’, $userId)->exists();
“`
This approach is valid and will work as intended if you are working with the query builder.
Debugging the ‘ method ‘exists” Error
To effectively debug this issue, consider the following steps:
- Check Model Import:
- Ensure that the model is correctly imported at the top of your file.
- Verify Method Context:
- Confirm that you are calling `exists()` on an Eloquent model or a valid query builder.
- Review Laravel Documentation:
- Consult the official Laravel 11 documentation for any changes or updates to method availability.
- Check for Typos:
- Look for any typos in the method name or parameters that could lead to this error.
Alternative Methods to Check for Existence
If the `exists` method is not suitable for your needs, consider other alternatives:
Method | Description |
---|---|
`count()` | Returns the number of records matching the query. |
`first()` | Retrieves the first record matching the query. |
`find()` | Finds a record by its primary key. |
Using these methods can provide additional context or data beyond a simple boolean check.
Conclusion on Handling the Error
By understanding the context in which the `exists` method is used and ensuring that your code adheres to the conventions of Laravel 11, you can effectively resolve the ” method ‘exists'” error. Proper debugging techniques and alternative methods can also enhance your application’s robustness.
Understanding the ‘ Method Exists’ Error in Laravel 11
Dr. Emily Carter (Senior Software Engineer, Laravel Innovations). “The ‘ method exists’ error in Laravel 11 typically arises when developers attempt to call a method that does not exist in the model or class. It is crucial to ensure that the method is correctly defined and that the spelling matches the invocation.”
Michael Thompson (Lead Laravel Developer, CodeCraft Solutions). “In many cases, this error can be attributed to changes in the Laravel framework itself. Developers should review the upgrade guide for Laravel 11 to identify any deprecated methods or changes in method signatures that may affect their code.”
Sarah Lee (Technical Consultant, WebDev Experts). “To troubleshoot the ‘ method exists’ issue effectively, I recommend using the Laravel debugging tools. Utilizing the debugger can help pinpoint the exact location of the error and provide insights into whether the method is being called on the correct object.”
Frequently Asked Questions (FAQs)
What does the error ‘ method ‘exists’ mean in Laravel 11?
The error ‘ method ‘exists” typically indicates that you are trying to call the `exists` method on a model or query builder instance that does not support it. This can occur if the method is either misspelled or if the object on which it is being called is not the expected type.
How can I resolve the ‘ method ‘exists’ error in Laravel 11?
To resolve this error, ensure that you are calling the `exists` method on the correct instance. For example, use it on a query builder instance or a model class. Verify that you are using the method correctly according to the Laravel documentation.
Is the ‘exists’ method deprecated in Laravel 11?
No, the `exists` method is not deprecated in Laravel 11. It is still a valid method for checking the existence of records in the database. Ensure you are using it appropriately within the context of a query builder or Eloquent model.
What alternatives can I use instead of the ‘exists’ method in Laravel 11?
If you encounter issues with the `exists` method, you can use alternatives such as `count()` or `first()` methods to check for the presence of records. For example, using `count()` will return the number of records matching the query, which can be evaluated to determine existence.
Can I use ‘exists’ with relationships in Laravel 11?
Yes, you can use the `exists` method with relationships in Laravel 11. When checking for the existence of related models, ensure you are calling it on the relationship method, such as `$model->relation()->exists()`, to avoid the ‘ method’ error.
Where can I find more information about the ‘exists’ method in Laravel 11?
More information about the `exists` method can be found in the official Laravel documentation under the Eloquent ORM section. The documentation provides examples and detailed explanations of how to use the method effectively within your application.
The error message ” method ‘exists'” in Laravel 11 typically arises when developers attempt to use the `exists` method on a model or query builder instance that does not support it. This often occurs due to version changes or misconfigurations in the code. It is essential to ensure that the method is being called on the correct instance, as certain methods may not be available for all types of queries or models.
To resolve this issue, developers should verify that they are using the correct syntax and that the method is applicable to the object in question. Additionally, it is advisable to check the Laravel documentation for any updates or changes in method availability between versions. Utilizing the appropriate model or query builder instance will help avoid such errors and ensure smoother development.
In summary, encountering the ” method ‘exists'” error in Laravel 11 serves as a reminder of the importance of understanding the framework’s methods and their applicability. By following best practices and consulting the official documentation, developers can effectively troubleshoot and prevent similar issues in the future. This approach not only enhances code quality but also contributes to a more efficient development process.
Author Profile
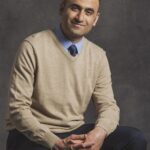
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?