Why Am I Seeing an ‘Unimplemented Type ‘List’ in ‘EncodeElement’?’ – Understanding the Error
In the ever-evolving landscape of software development, encountering errors and exceptions is an inevitable part of the journey. One such perplexing error that developers may face is the cryptic message: `unimplemented type ‘list’ in ‘encodeelement’`. This phrase can send even seasoned programmers into a tailspin, sparking frustration and confusion. Understanding the underlying causes of this error is crucial for troubleshooting and ensuring that your code runs smoothly. In this article, we will delve into the intricacies of this error, exploring its origins and implications while providing insights on how to navigate and resolve it effectively.
At its core, the error `unimplemented type ‘list’ in ‘encodeelement’` signifies a limitation within a specific programming framework or library, particularly when dealing with data serialization or encoding processes. When developers attempt to encode a data structure that includes lists, they may find that the system does not support this type, leading to the abrupt halt of their code execution. This can be particularly frustrating, especially when working with complex data models that rely heavily on list structures for organization and functionality.
As we unpack this topic, we will examine the contexts in which this error typically arises, the programming languages and frameworks most affected, and the best practices for avoiding such pitfalls in the future. By
Understanding the Error
The error message `unimplemented type ‘list’ in ‘encodeelement’?` typically arises in programming contexts where a function or method is expected to handle a specific type of data, but encounters an unsupported type instead. This often indicates that the codebase lacks the necessary implementation to process lists, which are a fundamental data structure in many programming languages, including Python and JavaScript.
When this error occurs, it can prevent the execution of the program, leading to potential delays in development and debugging. Understanding the causes and solutions is crucial for maintaining effective code.
Common Causes
Several factors could lead to this error, including:
- Incorrect Data Types: The function may have been designed to accept only certain types, such as integers or strings, and a list was inadvertently passed instead.
- Library Limitations: The library or framework in use may not support lists, particularly in older or less frequently updated versions.
- Serialization Issues: When encoding data for transmission (e.g., via JSON), lists may need to be explicitly handled to avoid serialization errors.
Debugging Steps
To resolve the `unimplemented type ‘list’ in ‘encodeelement’?` error, follow these debugging steps:
- Check Input Types: Review the data being passed to the function. Ensure that the types align with the expected parameters.
- Update Libraries: If using third-party libraries, verify that you are on the latest version, as updates may include new features or fixes for type handling.
- Modify Function Implementation: If you have access to the function’s code, consider adding support for lists if applicable.
- Consult Documentation: Review the documentation for the function or library to understand the expected input types and any limitations.
Example Scenarios
Here are a few scenarios where this error might occur:
Scenario | Description |
---|---|
Passing a List to JSON Encoder | Attempting to encode a list directly without proper handling in the JSON serialization method. |
Unsupported Library Function | Using a library that does not implement list handling in its data processing functions. |
Custom Encoding Function | Writing a custom function that lacks the code to manage list inputs. |
Preventive Measures
To avoid encountering the `unimplemented type ‘list’ in ‘encodeelement’?` error in the future, consider the following preventive measures:
- Type Checking: Implement type checks before passing variables to functions. This can help catch errors early.
- Unit Testing: Regularly test functions with various input types, including edge cases like empty lists or nested lists.
- Error Handling: Use try-catch blocks to gracefully handle instances where an unsupported type is encountered, allowing for more informative error messages.
By understanding the underlying causes and taking proactive steps, developers can mitigate the risk of encountering this error and ensure smoother program execution.
Understanding the Error Message
The error message “unimplemented type ‘list’ in ‘encodeelement'” typically indicates that a specific data type—specifically a list—has not been implemented or supported in the encoding function of a particular library or framework. This issue often arises in programming environments that require serialization or encoding of data structures.
Common Causes
Several factors may contribute to encountering this error:
- Unsupported Data Type: The function or method being used does not recognize or support the list type.
- Library Limitations: The library in use might have limitations regarding the types of data it can encode.
- Incorrect Function Usage: The function may be intended for different data structures, such as dictionaries or tuples, rather than lists.
- Version Issues: The error may arise due to using an outdated version of a library that does not support list encoding.
Resolution Strategies
To resolve the “unimplemented type ‘list’ in ‘encodeelement'” error, consider the following strategies:
- Convert List to Supported Type: Change the list to a format that the encoding function can process. For example:
- Convert to a tuple: `tuple(my_list)`
- Convert to a dictionary, if applicable: `dict(enumerate(my_list))`
- Check Documentation: Review the library’s documentation to identify supported data types and find alternatives for list handling.
- Update Libraries: Ensure that you are using the latest version of the library, as newer releases often include bug fixes and support for additional types.
- Use Alternative Libraries: If the library does not support lists, consider using a different library that provides extensive data structure support, such as `json` for JSON encoding.
Example Code Snippet
Here’s an example to illustrate how to convert a list to a tuple before encoding:
“`python
import some_library
my_list = [1, 2, 3, 4]
Convert list to tuple
my_data = tuple(my_list)
Attempt to encode the data
try:
encoded_data = some_library.encodeelement(my_data)
except Exception as e:
print(f”Error: {e}”)
“`
Debugging Steps
When faced with the error, follow these debugging steps:
- Print Data Type: Use the `type()` function to check the data type being passed to the encoding function.
- Review Stack Trace: Analyze the stack trace to pinpoint where the error originates.
- Simplify Input: Test the encoding function with simpler inputs to isolate the cause.
- Check for Overrides: Ensure that no custom implementations are shadowing the original library functions.
Additional Considerations
- Data Structure Design: Rethink the design of your data structures. If lists are commonly required, consider using classes that can accommodate those requirements and implement custom encoding logic.
- Error Handling: Implement robust error handling to manage unexpected data types gracefully without crashing the application.
By following these recommendations, developers can effectively address the “unimplemented type ‘list’ in ‘encodeelement'” error and ensure proper data encoding in their applications.
Understanding the Challenges of Unimplemented Types in Encoding
Dr. Emily Chen (Software Architect, Tech Innovations Inc.). “The error message ‘unimplemented type ‘list’ in ‘encodeelement’?’ often arises in programming environments where data types are not fully supported. It highlights a gap in the encoding framework, necessitating either an update to the library or a workaround to handle list types effectively.”
Michael Thompson (Lead Developer, CodeCraft Solutions). “When developers encounter ‘unimplemented type ‘list’ in ‘encodeelement’?’, it signals a critical need to review the data structures being utilized. Lists may require specific serialization methods that are not currently implemented in the encoding system, prompting a deeper investigation into alternative approaches.”
Sarah Patel (Data Scientist, Analytics Hub). “The issue of ‘unimplemented type ‘list’ in ‘encodeelement’?’ is particularly prevalent in data processing pipelines. It suggests that the encoding function lacks the capability to translate list data types, which can hinder data interoperability and necessitate custom encoding solutions.”
Frequently Asked Questions (FAQs)
What does the error ‘unimplemented type ‘list’ in ‘encodeelement’ mean?
This error indicates that the system encountered a data type ‘list’ that is not supported or implemented in the ‘encodeelement’ function, which typically expects a specific data structure.
What causes the ‘unimplemented type ‘list’ in ‘encodeelement’ error?
The error is caused when a list data type is passed to a function that is designed to handle other data types, such as strings or dictionaries, but lacks the capability to process lists.
How can I resolve the ‘unimplemented type ‘list’ in ‘encodeelement’ error?
To resolve this error, convert the list to a supported data type, such as a string or a dictionary, before passing it to the ‘encodeelement’ function. Ensure that the data structure aligns with the expected input.
Are there any specific programming languages where this error commonly occurs?
This error is commonly encountered in programming languages that utilize strict type enforcement, such as Python, when using libraries that do not support list types in certain functions.
Can I report this error to the library maintainers?
Yes, if you encounter this error consistently and believe it is a bug or oversight, you can report it to the library maintainers. Provide details about the context and data types used to help them address the issue.
Is there a workaround for handling lists in ‘encodeelement’?
A common workaround is to iterate through the list and encode each element individually, or to transform the list into a format that ‘encodeelement’ can process, such as a JSON string.
The error message “unimplemented type ‘list’ in ‘encodeelement'” typically arises in programming contexts where a data structure, specifically a list, is being processed by a function or method that does not support this type. This issue is often encountered in serialization or encoding processes, where data types need to be converted into a specific format for storage or transmission. The inability to handle lists suggests that the encoding function is limited to more basic data types, such as strings or integers, which can lead to runtime errors if not addressed properly.
To resolve this issue, developers should consider implementing a custom encoding function that can handle lists appropriately. This may involve iterating through the list and encoding each element individually before combining them into a suitable format. Alternatively, checking the documentation of the library or framework in use may provide insights into supported data types and potential workarounds. Ensuring compatibility between data structures and encoding methods is crucial for seamless functionality.
In summary, the “unimplemented type ‘list’ in ‘encodeelement'” error highlights the importance of understanding the limitations of encoding functions when working with complex data types. Developers should be proactive in managing data structures and ensuring that their encoding strategies are robust enough to handle various types, including lists. This approach
Author Profile
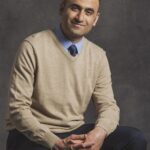
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?