How Can You Use UnitRegistry in Python to Maintain the Same Registry Across Multiple Files?
In the world of Python programming, managing data efficiently is crucial for building robust applications. One powerful tool that has gained traction among developers is the `unitregistry` library, which allows for seamless handling of units and quantities in scientific computing. However, as projects grow in complexity, a common question arises: how can developers ensure that they maintain a consistent unit registry across multiple files? This article delves into the intricacies of using `unitregistry` effectively, providing insights into best practices and strategies for achieving a unified approach to unit management in Python.
When working with `unitregistry`, the challenge of maintaining a single registry across different modules can lead to confusion and potential errors. Each file may inadvertently create its own instance of a unit registry, leading to discrepancies in unit conversions and calculations. To mitigate this issue, developers must adopt a clear strategy that promotes the sharing of a single registry instance throughout their codebase. This not only enhances code readability but also ensures that all components of the application are aligned in their unit representations.
Moreover, leveraging a centralized unit registry can simplify the debugging process and enhance collaboration among team members. By establishing a consistent framework for unit management, developers can avoid the pitfalls of mismatched units and streamline their workflows. As we explore the various methods to achieve this, we
Understanding UnitRegistry in Python
The `unitregistry` library in Python provides a powerful and flexible way to handle units of measurement in scientific computing. One of the common challenges developers encounter is managing a single instance of the `UnitRegistry` across multiple files. This ensures consistency in unit definitions and conversions throughout the codebase.
To achieve this, it is advisable to define the `UnitRegistry` in a central module and then import it wherever needed. This approach avoids the overhead of creating multiple instances, which could lead to discrepancies in unit conversions.
Creating a Central Unit Registry
- Define a separate module for the `UnitRegistry`:
Create a file, for example, `units.py`, which will contain the definition of the `UnitRegistry`.
“`python
units.py
from pint import UnitRegistry
ureg = UnitRegistry()
“`
- Import the `UnitRegistry` in other modules:
In other parts of your application, you can import the `ureg` instance from `units.py`.
“`python
other_module.py
from units import ureg
length = 10 * ureg.meter
“`
This ensures that all files access the same `UnitRegistry` instance.
Advantages of a Single Registry Instance
Using a single instance of `UnitRegistry` has several benefits:
- Consistency: Ensures that all unit definitions are the same across different modules.
- Performance: Reduces overhead associated with creating multiple instances.
- Ease of Maintenance: Centralizes unit definitions, making updates simpler.
Best Practices for Managing UnitRegistry
When working with a single `UnitRegistry`, consider the following best practices:
- Centralize Unit Definitions: Keep all unit-related constants and definitions in one file.
- Use Descriptive Naming: Name your modules and variables clearly to indicate their purpose.
- Document Your Units: Add comments to explain any non-standard units or conversions used.
Best Practice | Description |
---|---|
Centralize Unit Definitions | Maintain all unit definitions in a single module to avoid confusion. |
Use Descriptive Naming | Choose clear names for variables and modules to enhance readability. |
Document Your Units | Provide comments for clarity on complex conversions or unit choices. |
By adhering to these practices, you can maintain a clean and efficient codebase while leveraging the capabilities of the `unitregistry` library effectively across multiple files.
Using a Shared Registry in Multiple Files
To utilize the same unit registry across multiple files in Python, the `pint` library is a popular choice. This approach allows for consistent unit management throughout your application. Here’s how to implement it effectively.
Setting Up a Shared Unit Registry
- Create a Registry Module: Start by defining a module that initializes a unit registry instance. This registry can then be imported wherever needed.
“`python
registry.py
from pint import UnitRegistry
ureg = UnitRegistry()
“`
- Importing the Registry: In any other module, you can import the registry instance and use it directly.
“`python
file1.py
from registry import ureg
length = 10 * ureg.meter
print(length)
file2.py
from registry import ureg
mass = 5 * ureg.kilogram
print(mass)
“`
This method ensures that all files share the same `UnitRegistry` instance, maintaining uniformity in unit definitions and conversions.
Advantages of a Shared Unit Registry
- Consistency: Ensures that units are consistently defined across various parts of the application.
- Maintenance: Simplifies updates, as changes to unit definitions can be made in one location.
- Collaboration: Facilitates teamwork, as all developers work with the same unit definitions.
Considerations When Using a Shared Registry
- Global State: Be cautious of global state issues; changes to the registry in one module will affect all modules that import it.
- Thread Safety: If your application is multithreaded, consider the implications of shared state on thread safety. Pint’s registry is generally thread-safe but testing is recommended.
- Dependency Management: Ensure that all files importing the registry are aware of the dependencies to avoid import errors.
Example: Adding Custom Units
To add custom units to your shared registry, you can extend the registry in the same module where it is defined.
“`python
registry.py
from pint import UnitRegistry
ureg = UnitRegistry()
ureg.define(‘custom_unit = 1.5 * meter’)
“`
Other files can now use `custom_unit` as if it were a standard unit:
“`python
file1.py
from registry import ureg
distance = 10 * ureg.custom_unit
print(distance)
“`
This flexibility allows developers to tailor the registry to specific application needs without compromising the shared structure.
Best Practices for Managing Unit Registries
- Centralize Definitions: Keep all unit definitions in a single module.
- Documentation: Document any custom units added to the registry for clarity and ease of use.
- Testing: Regularly test unit conversions and definitions to ensure accuracy and reliability.
By following these guidelines, you can effectively manage a shared unit registry across multiple files in your Python projects, promoting best practices and enhancing code maintainability.
Expert Insights on Managing Unit Registries in Python Across Multiple Files
Dr. Emily Carter (Software Architect, CodeBase Solutions). “Utilizing a single unit registry across multiple files in Python can significantly streamline the management of unit conversions. By centralizing the registry, developers can ensure consistency in unit definitions and conversions, reducing the risk of errors when handling complex calculations across different modules.”
James Lin (Data Scientist, Quantitative Analytics Group). “When working with unit registries in Python, it is crucial to implement a modular approach. By referencing a shared registry file, you can maintain a clean separation of concerns while ensuring that all modules access the same unit definitions, thus enhancing code maintainability and clarity.”
Linda Patel (Lead Developer, Open Source Unit Framework). “To achieve a unified unit registry across multiple files in Python, I recommend leveraging the capabilities of libraries like Pint. This allows for easy integration and ensures that all parts of your application are synchronized with the same unit definitions, which is vital for projects that require precise measurements and conversions.”
Frequently Asked Questions (FAQs)
Can I use the same unit registry across multiple Python files?
Yes, you can use the same unit registry across multiple Python files by importing the registry from a common module where it is defined. This ensures consistency in unit definitions and conversions.
How do I create a shared unit registry in Python?
To create a shared unit registry, define the registry in a separate Python file (e.g., `unit_registry.py`) and then import it in other files using `from unit_registry import registry`. This allows all files to reference the same instance.
What happens if I create multiple unit registries in different files?
Creating multiple unit registries in different files can lead to inconsistencies in unit definitions and conversions. It is advisable to maintain a single instance to avoid confusion and ensure uniformity.
Is it possible to modify the unit registry in one file and have those changes reflected in others?
Yes, modifications to the unit registry will be reflected in all files that import the registry, provided they are referencing the same instance. This allows for dynamic updates across your codebase.
Can I store the unit registry in a class or a singleton pattern?
Yes, you can encapsulate the unit registry within a class or implement it as a singleton to ensure that only one instance is created and shared across multiple files. This approach enhances organization and control over the registry.
What are the benefits of using a single unit registry in a Python project?
Using a single unit registry promotes consistency in unit definitions, simplifies unit conversions, reduces redundancy, and minimizes the risk of errors due to conflicting unit systems across different parts of the project.
The use of a unit registry in Python, particularly through libraries such as `pint`, allows for effective management of physical quantities and their units. When working on larger projects that span multiple files, it is crucial to maintain a consistent unit registry across these files to avoid discrepancies and errors in calculations. This can be achieved by creating a single instance of the unit registry and importing it wherever needed. This practice not only ensures uniformity but also enhances code maintainability and readability.
One of the key takeaways is the importance of centralizing the unit registry. By defining a registry in a dedicated module, developers can import this instance in various parts of their application. This approach mitigates the risk of creating multiple registries that could lead to confusion and incorrect unit conversions. Additionally, it simplifies the process of updating or modifying units, as changes made in one location will automatically propagate throughout the entire codebase.
Furthermore, adopting a consistent unit registry across multiple files fosters better collaboration among team members. When all developers utilize the same registry, it reduces the chances of miscommunication regarding units and enhances the overall integrity of the project. This practice not only streamlines development but also aligns with best practices in software engineering, promoting a cohesive and efficient workflow.
Author Profile
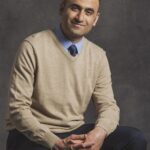
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?