How Can You Resolve the ‘ValueError: DataFrame Constructor Not Properly Called’ Issue?
In the world of data manipulation and analysis, pandas has emerged as a go-to library for Python developers. Its powerful DataFrame structure allows for efficient handling of large datasets, making it an essential tool for data scientists and analysts alike. However, even seasoned users can encounter frustrating errors that halt their progress. One such common pitfall is the notorious `ValueError: DataFrame constructor not properly called`. This error can leave users scratching their heads, wondering what went wrong in their code. In this article, we will delve into the nuances of this error, exploring its causes and providing clarity on how to resolve it effectively.
Understanding the intricacies of the DataFrame constructor is crucial for anyone working with pandas. The error typically arises when the input data provided to create a DataFrame is not structured in a way that pandas expects. Whether it’s a mismatch in data shapes, incorrect types, or simply a misconfiguration, this error serves as a reminder of the importance of data integrity and format. By examining the common scenarios that lead to this issue, we can better equip ourselves to handle similar challenges in the future.
As we navigate through the causes and solutions to the `ValueError`, we’ll also highlight best practices for constructing DataFrames. This knowledge will not only help you troubleshoot errors
Understanding the ValueError in DataFrame Construction
The `ValueError: DataFrame constructor not properly called!` is a common error encountered by users when attempting to create a DataFrame in pandas. This error typically indicates that the input data provided to the DataFrame constructor is not in a format that pandas can interpret correctly. Understanding the root causes of this error is crucial for efficient debugging.
Several factors can contribute to this error, including:
- Incorrect Data Type: The data passed to the DataFrame constructor must be either a 2D array-like structure (like lists of lists, or a 2D NumPy array), a dictionary of arrays, or a structured array. Providing incompatible data types can lead to this error.
- Improper Structure of Input Data: If the input data is a list or dictionary but not structured properly, pandas will not be able to interpret it as a valid DataFrame format.
- Missing Index or Columns: When creating a DataFrame from a dictionary, if the lists contained within the dictionary are of different lengths, pandas cannot infer a proper structure.
To resolve the `ValueError`, consider the following approaches:
- Check Data Type: Ensure that the data passed to the DataFrame is in a suitable format. For instance, if using a list, it should be a list of lists.
- Inspect Input Structure: Verify that the input data is structured correctly. For example, when using a dictionary, all values must have the same length.
- Provide Index and Columns: When creating a DataFrame from a dictionary with lists of different lengths, explicitly provide the `columns` and `index` parameters.
Examples of Common Scenarios
Here are some examples illustrating how to create DataFrames properly and the types of input that will trigger the ValueError.
Scenario | Input Data | Error |
---|---|---|
List of Lists | [[1, 2], [3, 4]] | No Error |
Dictionary with Same Length | {‘A’: [1, 2], ‘B’: [3, 4]} | No Error |
Dictionary with Different Lengths | {‘A’: [1, 2], ‘B’: [3]} | ValueError |
Single Value | 5 | ValueError |
Debugging Steps
When faced with the `ValueError`, follow these debugging steps to isolate and resolve the issue:
- Print the Input Data: Check the structure and type of the data being passed to the DataFrame constructor. This can often reveal mismatched types or unexpected formats.
- Use Type Checking: Utilize Python’s built-in `type()` function to confirm the data types of the variables you are trying to convert into a DataFrame.
- Review Documentation: Consult the pandas documentation to ensure that you are using the DataFrame constructor correctly and understand the expected input formats.
By carefully analyzing the input data and following structured debugging practices, you can effectively resolve the `ValueError` and successfully create DataFrames in pandas.
Understanding the ValueError
A `ValueError` in Python, particularly when working with Pandas DataFrames, often indicates that the input data is not formatted correctly for the DataFrame constructor. This error can arise from various issues, including:
- Mismatched data shapes
- Incorrect data types
- Improperly structured data
When you encounter the error message “DataFrame constructor not properly called!”, it is essential to examine the input data closely.
Common Causes of the Error
- Incorrect Data Structure: Data passed to the DataFrame constructor must be in a format such as a dictionary of lists, a list of dictionaries, or a 2D array.
- Example of correct usage:
“`python
import pandas as pd
data = {
‘Column1’: [1, 2, 3],
‘Column2’: [‘A’, ‘B’, ‘C’]
}
df = pd.DataFrame(data)
“`
- Example of incorrect usage:
“`python
data = [1, 2, 3] This will raise ValueError
df = pd.DataFrame(data)
“`
- Inconsistent Lengths of Data: When constructing a DataFrame from a dictionary, all lists must have the same length.
- Example of inconsistency:
“`python
data = {
‘Column1’: [1, 2],
‘Column2’: [‘A’, ‘B’, ‘C’] Different length
}
df = pd.DataFrame(data) Raises ValueError
“`
- Using None or NaN Values Improperly: While Pandas can handle `None` or `NaN`, inappropriate placement in the data structure can lead to errors.
- Example:
“`python
data = {
‘Column1’: [1, None],
‘Column2’: [‘A’, ‘B’]
}
df = pd.DataFrame(data) This is acceptable
“`
Debugging Steps
To resolve the `ValueError`, follow these debugging steps:
- Check Data Structure: Ensure the data passed is a valid structure.
- Verify Lengths: Confirm that all lists or arrays have the same length if using a dictionary.
- Print Data Types: Use `type()` to verify the types of the input data.
- Inspect Data: Print or log the data before passing it to the DataFrame constructor.
“`python
print(data) Helpful to see the actual structure
“`
Best Practices for DataFrame Creation
To prevent encountering the `ValueError`, adhere to these best practices:
- Use well-structured lists or dictionaries.
- Validate input data types before passing them to the constructor.
- Consider using `pd.DataFrame.from_records()` for more complex data structures.
- Always check for missing values and handle them appropriately before DataFrame creation.
Example of Correct DataFrame Creation
Here’s a structured example that demonstrates the correct way to create a DataFrame:
“`python
import pandas as pd
Correctly structured data
data = [
{‘Column1’: 1, ‘Column2’: ‘A’},
{‘Column1’: 2, ‘Column2’: ‘B’},
{‘Column1’: 3, ‘Column2’: ‘C’}
]
df = pd.DataFrame(data)
print(df)
“`
The output will be:
Column1 | Column2 |
---|---|
1 | A |
2 | B |
3 | C |
Ensuring your data is formatted correctly will help avoid `ValueError` and streamline the DataFrame creation process.
Understanding the ValueError in DataFrame Construction
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “The ‘ValueError: DataFrame constructor not properly called’ typically arises when the input data to the DataFrame constructor is not in an expected format. This can happen if the data is not structured as a list, dictionary, or array-like object. Ensuring that the data is properly formatted before passing it to the DataFrame is crucial for avoiding this error.”
Mark Thompson (Senior Software Engineer, Data Solutions Group). “This error often indicates that the parameters provided to the DataFrame constructor are either missing or incorrectly specified. It is essential to verify that the data being passed is not empty and adheres to the expected shape. Debugging the input data can help in pinpointing the exact cause of the issue.”
Linda Garcia (Lead Python Developer, Analytics Hub). “When encountering the ‘ValueError’ during DataFrame creation, developers should consider checking the data type of the input. Using functions like type() or isinstance() can help ensure that the data is compatible with the DataFrame constructor. A common mistake is passing a single value instead of a collection, which leads to this error.”
Frequently Asked Questions (FAQs)
What does the error “ValueError: DataFrame constructor not properly called” mean?
This error indicates that the parameters passed to the pandas DataFrame constructor are not in the expected format, leading to an inability to create a DataFrame.
What are common causes for this error in pandas?
Common causes include passing an incorrect data type (e.g., a single scalar value instead of a list or dictionary), providing a malformed dictionary, or using an unsupported structure like a nested list with inconsistent lengths.
How can I troubleshoot this error?
To troubleshoot, verify the data structure being passed to the DataFrame constructor. Ensure it is a valid format such as a list of lists, a dictionary of lists, or a NumPy array. Print the data before passing it to identify any discrepancies.
What is the correct way to create a DataFrame?
A DataFrame can be created using various formats, such as: `pd.DataFrame(data)` where `data` can be a dictionary, list of lists, or a NumPy array. Ensure that the data conforms to the expected structure.
Can this error occur when using other pandas functions?
Yes, similar errors can occur when using functions that expect a DataFrame as input but receive an improperly formatted object. Always check the expected input format for each function.
How can I prevent this error in my code?
To prevent this error, always validate your data structure before passing it to the DataFrame constructor. Use type checks and print statements to ensure that the data conforms to the expected formats.
The error message “ValueError: DataFrame constructor not properly called!” typically arises when attempting to create a pandas DataFrame but the input data is not formatted correctly. This can occur due to various reasons, such as passing incompatible data types, using incorrect data structures, or failing to adhere to the expected input formats. Understanding the structure and requirements of the DataFrame constructor is crucial for resolving this issue effectively.
One common cause of this error is passing a single scalar value instead of an array-like structure, such as a list or dictionary. Additionally, ensuring that the data being passed is of uniform length can prevent this error. When constructing a DataFrame from a dictionary, the keys should represent the column names, and the values should be lists or arrays of equal length. It is also important to verify that any nested structures are properly formatted to align with pandas’ expectations.
To mitigate this error, it is advisable to validate the data before attempting to create a DataFrame. This includes checking the types and shapes of the input data. Utilizing debugging techniques, such as printing the data structure before passing it to the DataFrame constructor, can provide insights into potential issues. By adhering to these best practices, users can enhance their proficiency in using pandas and
Author Profile
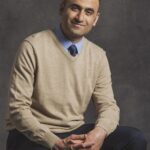
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?