How Can I Resolve the ValueError: ‘Index Contains Duplicate Entries’ When Reshaping Data?
In the world of data manipulation and analysis, encountering errors can be a frustrating yet enlightening experience. One such error that often perplexes both novice and seasoned data scientists alike is the notorious “ValueError: Index contains duplicate entries, cannot reshape.” This seemingly cryptic message can halt your workflow, leaving you scrambling to understand its implications. But fear not! In this article, we will delve into the intricacies of this error, exploring its causes, implications, and, most importantly, how to resolve it effectively. Whether you’re working with pandas in Python or handling data in another environment, understanding this error is crucial for maintaining the integrity of your datasets.
When dealing with DataFrames or matrices, the structure and organization of your data are paramount. The “ValueError” typically arises when attempting to reshape or pivot data that contains duplicate indices, leading to ambiguity in how the data should be rearranged. This can occur in various scenarios, such as when merging datasets, aggregating data, or simply trying to reshape your data for analysis. Recognizing the underlying issues that lead to this error is the first step in preventing it from disrupting your analytical processes.
Moreover, the implications of ignoring this error can be significant. Duplicate entries can skew your analysis, lead to inaccurate results,
Understanding the ValueError: Index Contains Duplicate Entries
When working with data manipulation in Python, particularly with libraries like Pandas, encountering a `ValueError` indicating that the index contains duplicate entries can be a common issue. This error typically arises during operations that require unique indices, such as reshaping or pivoting data frames.
The presence of duplicate indices can lead to ambiguity in how the data should be organized, ultimately resulting in an inability to reshape the data as intended. Understanding the underlying causes and how to address them is crucial for effective data handling.
Common Scenarios Leading to Duplicate Indices
Several scenarios can lead to duplicate entries in the index of a DataFrame:
- Data Importation: When importing data from sources such as CSV files, duplicate entries can occur if the data source itself has repeated rows or if the index is not properly set.
- Merging DataFrames: Performing joins or concatenations without ensuring unique identifiers can create duplicates.
- Data Manipulation: Operations that involve filtering or grouping data may inadvertently lead to duplicate indices if not managed carefully.
Resolving Duplicate Index Issues
To resolve the `ValueError`, consider the following strategies:
- Check for Duplicates: Use the `duplicated()` method to identify duplicates in your DataFrame.
- Reset the Index: Employ `reset_index(drop=True)` to create a new integer index that eliminates duplicates.
- Aggregate Data: If duplicates are valid, consider aggregating the data using functions like `mean()`, `sum()`, or `count()` to summarize the entries.
The following table illustrates how to identify and handle duplicate indices:
Method | Description | Example Code |
---|---|---|
Check Duplicates | Identify duplicate indices in the DataFrame. | df[df.index.duplicated()] |
Reset Index | Create a new index without duplicates. | df.reset_index(drop=True) |
Aggregate Data | Combine duplicate entries using an aggregation function. | df.groupby(df.index).mean() |
Best Practices for Managing Indices
To prevent encountering the `ValueError` in the future, adhere to the following best practices:
- Set Unique Indices: Ensure that your DataFrame is initialized with a unique index. Use the `set_index()` method with a column that contains unique values.
- Validate Data Before Operations: Always validate the integrity of your data before performing operations that depend on unique indices.
- Regularly Clean Data: Implement data cleaning procedures to remove or correct duplicate entries as part of your data preprocessing workflow.
By following these guidelines, you can minimize the chances of encountering the `ValueError` related to duplicate indices and enhance the robustness of your data manipulation processes.
Understanding the Error
A `ValueError` indicating that an index contains duplicate entries and cannot reshape typically occurs in data manipulation tasks using libraries such as pandas or NumPy. This error arises when attempting to reshape or re-index a DataFrame or array that has non-unique index values. Understanding the nature of your dataset is crucial in resolving this issue.
Common Causes:
- Duplicate index values in a DataFrame.
- Attempting to reshape data where the total number of elements does not align with the new shape.
- Merging or concatenating DataFrames with overlapping indices.
Identifying Duplicate Entries
To address this error, first identify any duplicate indices in your DataFrame. You can achieve this using the following methods in pandas:
“`python
import pandas as pd
Sample DataFrame
data = {‘A’: [1, 2, 3], ‘B’: [4, 5, 6]}
df = pd.DataFrame(data, index=[0, 1, 1]) Duplicate index
Checking for duplicates
duplicates = df.index.duplicated()
print(df[duplicates]) Displays rows with duplicate indices
“`
This code snippet will help you locate rows that are causing the problem due to duplicate indices.
Resolving the Error
There are several approaches to resolve the duplicate index issue:
- Remove Duplicate Indices: If the duplicate entries are not necessary, you can drop them.
“`python
df = df[~df.index.duplicated(keep=’first’)]
“`
- Reset the Index: Resetting the index will create a new sequential index.
“`python
df.reset_index(drop=True, inplace=True)
“`
- Aggregate Data: If duplicates represent valid data, consider aggregating them to consolidate rows.
“`python
df = df.groupby(df.index).sum() Example of summing duplicates
“`
- Set a Unique Index: If a specific column can serve as a unique index, set it using:
“`python
df.set_index(‘column_name’, inplace=True)
“`
Reshaping Data Safely
When reshaping data, ensure that the total number of elements matches the new shape. Use the following guidelines:
- Check the number of elements:
“`python
num_elements = df.size
desired_shape = (rows, columns) Define your desired shape
if num_elements != rows * columns:
raise ValueError(“Cannot reshape; total elements do not match.”)
“`
- Utilize the `pivot()` or `pivot_table()` methods for reshaping while handling duplicate indices effectively.
Example Scenario
Consider a DataFrame with duplicate indices:
Index | A | B |
---|---|---|
0 | 1 | 4 |
1 | 2 | 5 |
1 | 3 | 6 |
Attempting to reshape this DataFrame will trigger the ValueError. To resolve it, use one of the previously mentioned strategies.
Resolution Steps:
- Identify duplicates.
- Choose an appropriate method to handle duplicates (remove, reset, aggregate).
- Ensure reshaping conditions are met before proceeding.
By following these steps, you can effectively manage the `ValueError` related to duplicate entries and reshape your data as intended.
Understanding ValueErrors in Data Manipulation
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “The ValueError indicating that the index contains duplicate entries typically arises when attempting to reshape a DataFrame in libraries like Pandas. It is essential to ensure that the index is unique before performing operations that require reshaping, such as pivoting or unstacking.”
Michael Tran (Software Engineer, Data Solutions Corp.). “When you encounter a ValueError related to duplicate entries, it is a clear signal to inspect your data for redundancy. Utilizing methods such as ‘drop_duplicates()’ can help clean the dataset, allowing for successful reshaping and manipulation.”
Linda Zhao (Machine Learning Specialist, AI Research Labs). “Duplicate indices can lead to unexpected behavior during data reshaping processes. It is advisable to apply techniques like resetting the index or aggregating data to ensure that each entry is unique, thus preventing the ValueError from occurring.”
Frequently Asked Questions (FAQs)
What does the error “ValueError: Index contains duplicate entries, cannot reshape” mean?
This error indicates that the operation you are attempting to perform requires unique indices, but the data being processed contains duplicate indices, which prevents reshaping.
How can I identify duplicate entries in my DataFrame?
You can use the `DataFrame.duplicated()` method in pandas to identify duplicate entries. This method returns a boolean Series indicating whether each row is a duplicate or not.
What steps can I take to resolve the “ValueError: Index contains duplicate entries” issue?
To resolve this issue, you can either drop the duplicate entries using `DataFrame.drop_duplicates()`, or you can reset the index with `DataFrame.reset_index(drop=True)` to create a new index without duplicates.
Can I reshape a DataFrame with duplicate indices?
No, reshaping operations such as `pivot` or `pivot_table` require unique indices. You must ensure that the DataFrame has unique indices before attempting to reshape it.
What are some common methods to ensure unique indices in a DataFrame?
Common methods include using `DataFrame.drop_duplicates()` to remove duplicates, or using `DataFrame.groupby()` to aggregate data, which can help create unique indices based on specified criteria.
Is it possible to keep duplicates while reshaping data?
Yes, you can keep duplicates by using methods like `groupby` or `melt`, which allow for aggregation or transformation of data without requiring unique indices.
The ValueError indicating that an index contains duplicate entries and cannot be reshaped is a common issue encountered in data manipulation, particularly when using libraries such as Pandas in Python. This error arises when attempting to reshape data structures, like DataFrames or Series, that have non-unique indices. The reshaping process requires unique identifiers to align data correctly, and the presence of duplicates disrupts this requirement, leading to the error.
To resolve this issue, it is essential to identify and address the duplicate entries in the index. Techniques such as using the `drop_duplicates()` method can help in removing duplicates, while the `reset_index()` method allows for the creation of a new index that is unique. Additionally, understanding the context of the data and the intended reshaping operation can guide the selection of appropriate methods to handle duplicates effectively.
Key takeaways include the importance of ensuring data integrity before performing operations that require unique indices. It is advisable to conduct preliminary checks for duplicates and to implement data cleaning practices as part of the data preparation process. By proactively managing indices and understanding the implications of duplicates, one can avoid encountering the ValueError and ensure smoother data manipulation workflows.
Author Profile
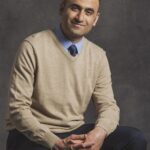
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?