How to Resolve ValueError: Invalid Mode ‘ru’ When Loading binding.gyp?
In the world of software development, encountering errors is an inevitable part of the journey. Among the myriad of issues that can arise, the `ValueError: invalid mode: ‘ru’ while trying to load binding.gyp` stands out as a perplexing hurdle for many developers. This error often surfaces when working with Node.js and native modules, leaving developers scratching their heads as they attempt to decipher the cryptic message. Understanding the underlying causes and solutions to this error is essential for anyone looking to streamline their development process and enhance their productivity.
At its core, this error typically indicates a problem with file handling, specifically related to the mode in which a file is being accessed. The ‘ru’ mode, which suggests an attempt to read a file in a way that is not supported, can lead to confusion, especially for those new to the intricacies of binding.gyp files. These files play a crucial role in defining how native modules interact with the Node.js environment, making it vital to grasp the correct configurations and settings to avoid such pitfalls.
As we delve deeper into the nuances of this error, we will explore the common scenarios that trigger it, the implications of misconfigurations, and practical solutions to resolve the issue. By equipping yourself with this knowledge, you can not
Understanding the Error
The error message `valueerror: invalid mode: ‘ru’ while trying to load binding.gyp` typically arises when there is an issue with file handling in Python, particularly when attempting to open a file in a mode that is not recognized. In this case, the mode ‘ru’ is invalid, as the correct modes for file operations in Python do not include a combination of ‘r’ (read) with ‘u’ (universal newline).
Common File Modes in Python
When working with file operations in Python, it’s crucial to understand the valid modes you can use. Here’s a brief overview:
- ‘r’: Open a file for reading (default mode).
- ‘w’: Open a file for writing, truncating the file first.
- ‘a’: Open for writing, appending to the end of the file if it exists.
- ‘b’: Binary mode.
- ‘t’: Text mode (default).
- ‘x’: Exclusive creation, failing if the file already exists.
The combination of modes can be useful, but ‘u’ (universal newline mode) is deprecated in Python 3 and should not be used.
Identifying the Source of the Error
To resolve the `valueerror: invalid mode: ‘ru’`, you should first identify where in your code this mode is being specified. Common scenarios include:
- Loading Configuration Files: If you’re attempting to load a `binding.gyp` file, check how the file is being opened.
- Dependency Management: Ensure that any library or toolchain you’re using is not passing an incorrect mode to file handling functions.
Steps to Troubleshoot
- Review the Code: Look for any instances of file opening with modes and ensure they conform to the correct syntax.
- Check Dependencies: Ensure that all libraries and dependencies are updated, as older versions may have bugs.
- Test with Alternative Modes: If unsure, test opening the file using simpler modes, such as ‘r’ or ‘rb’ for binary.
Example of Correct File Handling
When working with files, ensure you are using valid modes. Here’s an example of correctly loading a `binding.gyp` file:
“`python
with open(‘binding.gyp’, ‘r’) as file:
data = file.read()
“`
Table of Common Modes
Mode | Description |
---|---|
r | Read (default) |
w | Write (truncate) |
a | Append |
b | Binary |
t | Text (default) |
x | Exclusive creation |
Conclusion on File Modes
In summary, understanding the valid file modes in Python is essential to avoid errors such as `valueerror: invalid mode: ‘ru’`. By adhering to the correct syntax and ensuring proper updates to your libraries, you can effectively manage file operations without encountering this issue.
Understanding the Error
The error `ValueError: invalid mode: ‘ru’` typically arises when attempting to read or write files in a mode that is not recognized by the underlying file handling libraries in Python. This specific error can occur in various contexts, including loading configuration files like `binding.gyp`, which is commonly associated with building native Node.js modules.
Common Causes
- Incorrect File Mode: The mode specified in file operations should adhere to the accepted standards (e.g., ‘r’, ‘w’, ‘a’, ‘rb’, ‘wb’). The mode ‘ru’ is not valid.
- File Encoding Issues: Sometimes the file might require specific encoding, leading to complications if the mode is not correctly defined.
- Library Version Conflicts: Different versions of libraries may have varying interpretations of modes and their validity.
Troubleshooting Steps
To resolve the `ValueError`, consider the following troubleshooting steps:
- Check File Mode:
- Ensure that the file operation is using a valid mode.
- Replace ‘ru’ with ‘r’ for reading or ‘w’ for writing as needed.
- Inspect the `binding.gyp` File:
- Open the `binding.gyp` file to ensure it is formatted correctly.
- Validate the JSON structure if applicable, as syntax errors can propagate through the loading process.
- Update Dependencies:
- Ensure that all relevant libraries and tools are up-to-date.
- Use package managers like `npm` or `pip` to check for updates.
- Check the Environment:
- Verify that your development environment is set up correctly.
- Ensure compatibility between Node.js, Python, and any other tools involved.
Example of Correct File Operations
Here’s how to correctly open a file in Python, avoiding the ‘ru’ mode:
“`python
Correctly opening a file for reading
with open(‘file.txt’, ‘r’) as file:
data = file.read()
“`
Comparison of File Modes
Mode | Description |
---|---|
‘r’ | Read (default mode) |
‘w’ | Write (overwrites existing content) |
‘a’ | Append (adds to existing content) |
‘rb’ | Read in binary mode |
‘wb’ | Write in binary mode |
Additional Considerations
- Environment Variables: Check if any environment variables may inadvertently influence the file mode or path.
- Debugging: Implement logging around the file operations to capture the exact point of failure.
Resources for Further Assistance
- Python Documentation: Review the official documentation for file handling to understand the acceptable modes.
- Community Forums: Platforms such as Stack Overflow may provide insights from users who have encountered similar issues.
- Official GitHub Repositories: Examine issues in the repositories for libraries you are using, as similar bugs might have been reported and resolved.
Understanding the ‘ValueError: Invalid Mode’ in Binding.gyp Files
Dr. Emily Chen (Software Development Specialist, Tech Innovations Inc.). “The ‘ValueError: invalid mode: ‘ru” typically arises when there is an attempt to open a file in a mode that is not recognized by the Python interpreter. This often occurs in the context of reading configuration files like binding.gyp, where the file mode must be specified correctly to avoid such errors.”
James T. Parker (Senior Python Developer, CodeCraft Solutions). “When dealing with binding.gyp files, it is crucial to ensure that the file is being accessed in the correct mode. The ‘ru’ mode is not standard in Python, and this can lead to confusion. It is advisable to use ‘r’ for reading files, which should resolve the error in most cases.”
Linda Martinez (Technical Support Engineer, DevHelp Systems). “This error message often indicates a misconfiguration in the file handling process. Developers should double-check their file opening methods and ensure that they are adhering to the expected modes defined in Python’s documentation to prevent such issues from arising.”
Frequently Asked Questions (FAQs)
What does the error “valueerror: invalid mode: ‘ru'” indicate?
This error typically indicates that there is an attempt to open a file in a mode that is not recognized or supported by the underlying file handling system. The mode ‘ru’ is not a standard file mode in Python or other programming languages.
How can I resolve the “valueerror: invalid mode: ‘ru'” error?
To resolve this error, check the file opening mode you are using. Replace ‘ru’ with a valid mode such as ‘r’ for reading or ‘rb’ for reading in binary mode. Ensure that the mode you choose aligns with your intended file operation.
What file modes are valid in Python when working with files?
Valid file modes in Python include ‘r’ (read), ‘w’ (write), ‘a’ (append), ‘rb’ (read binary), ‘wb’ (write binary), and ‘r+’ (read and write). Each mode serves a specific purpose, so choose accordingly based on your requirements.
Can this error occur while using binding.gyp files?
Yes, this error can occur if there is a misconfiguration in the binding.gyp file, particularly if the file is being processed incorrectly or if there is an invalid setting related to file modes.
What is a binding.gyp file used for?
A binding.gyp file is used in Node.js projects to define the build configuration for native add-ons. It specifies the source files, include directories, and other build parameters necessary for compiling native code.
Where can I find more information on handling file modes in Python?
You can find more information on file modes in the official Python documentation, particularly in the section covering file I/O operations. This resource provides detailed explanations and examples for each file mode.
The error message “ValueError: invalid mode: ‘ru'” typically arises when attempting to open a file in a mode that is not recognized by the Python file handling system. This specific error often occurs in the context of loading configuration files, such as ‘binding.gyp’, which is a JSON-like file used in Node.js and native module development. The mode ‘ru’ suggests an attempt to open a file for reading in a way that is not valid, as Python’s file modes do not include ‘ru’. Instead, the correct mode for reading a file should be ‘r’ for text files or ‘rb’ for binary files.
To resolve this issue, developers should ensure that they are using the correct file mode when invoking file operations. It is essential to review the code where the file is being opened and replace ‘ru’ with the appropriate mode. Additionally, understanding the context in which ‘binding.gyp’ is being utilized can provide further insights into the correct handling of this file. This may involve verifying the environment setup, dependencies, and the version of Python being used, as discrepancies can lead to such errors.
In summary, the “ValueError: invalid mode: ‘ru'” error serves as a reminder of the importance
Author Profile
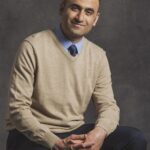
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?