How Can You Clear a VBA Table Without Headers Without Deleting It?
In the world of Excel and VBA (Visual Basic for Applications), managing data efficiently is crucial for any user looking to streamline their workflow. One common task that often arises is the need to clear the contents of a table without affecting its structure or headers. Whether you’re updating data regularly or simply wanting to reset a table for new entries, knowing how to clear a table while preserving its format can save you time and effort. This article delves into the nuances of using VBA to achieve this goal, ensuring that your tables remain intact while allowing for easy data refresh.
Clearing a table in Excel can seem straightforward, but when you want to maintain the header and overall design, it requires a more nuanced approach. VBA provides powerful tools that allow users to manipulate their spreadsheets programmatically, making it possible to clear data without deleting the table itself. This functionality is particularly useful in scenarios where data is frequently updated or when creating templates that need to be reused without losing their formatting.
In this article, we will explore the methods and techniques for clearing table contents using VBA, focusing on practical examples and code snippets that you can easily implement. Whether you’re a seasoned programmer or a novice just starting with VBA, you’ll find valuable insights that will enhance your Excel experience and empower you to manage your data more effectively.
Understanding VBA Table Structures
When working with tables in Excel using VBA, it’s essential to understand the structure of these tables. A table in Excel consists of a header row and a body of data. In many cases, users may need to clear the contents of the table without affecting the headers or deleting the table itself.
Clearing Table Data with VBA
To clear the contents of a table while preserving the header, you can utilize the `ListObject` feature in VBA. This allows you to target specific ranges within the table to clear their contents effectively. The following code snippet demonstrates how to achieve this:
“`vba
Sub ClearTableContents()
Dim ws As Worksheet
Dim tbl As ListObject
Dim dataRange As Range
‘ Set the worksheet and table
Set ws = ThisWorkbook.Sheets(“Sheet1”) ‘ Change to your sheet name
Set tbl = ws.ListObjects(“Table1”) ‘ Change to your table name
‘ Define the data range excluding the header
Set dataRange = tbl.DataBodyRange
‘ Clear the data without deleting the table or headers
dataRange.ClearContents
End Sub
“`
In this code:
- `ws` represents the worksheet where the table resides.
- `tbl` is the table object that you want to clear.
- `dataRange` refers specifically to the body of the table, which is cleared using the `ClearContents` method.
Key Points to Remember
- Always reference the correct worksheet and table name in your code.
- The `DataBodyRange` property specifically targets the area of the table that contains data, excluding the header.
- Using `ClearContents` ensures that only the data is removed, leaving the table structure intact.
Example Table Structure
To illustrate, consider the following example of a table layout in Excel:
Name | Age | City |
---|---|---|
John Doe | 30 | New York |
Jane Smith | 25 | Los Angeles |
In this table, if you execute the `ClearTableContents` subroutine, only the cells containing “John Doe,” “30,” “New York,” “Jane Smith,” “25,” and “Los Angeles” will be cleared, while the headers “Name,” “Age,” and “City” will remain unaffected.
Additional Considerations
When working with VBA to manipulate tables, consider the following best practices:
- Test your code on a copy of your workbook to avoid accidental data loss.
- Use error handling to manage potential issues, such as referencing a non-existent table or worksheet.
- Document your code for clarity and future reference, especially if others may work with it.
By leveraging these techniques, you can efficiently manage table data in Excel through VBA, ensuring that your headers remain intact while clearing out old or unnecessary information.
Clearing Table Data with VBA
To clear the data in a table without deleting the table itself, you can utilize VBA (Visual Basic for Applications). This method effectively removes all entries from a specified table while keeping the table structure intact, including headers and formatting.
VBA Code Example
Here is a sample code snippet that demonstrates how to clear a table’s data without affecting the headers:
“`vba
Sub ClearTableData()
Dim ws As Worksheet
Dim tbl As ListObject
Dim cell As Range
‘ Set the worksheet and the table you want to clear
Set ws = ThisWorkbook.Sheets(“Sheet1”) ‘ Change “Sheet1” to your sheet name
Set tbl = ws.ListObjects(“Table1”) ‘ Change “Table1” to your table name
‘ Loop through each cell in the data range of the table and clear its content
For Each cell In tbl.DataBodyRange
cell.ClearContents
Next cell
End Sub
“`
Understanding the Code
- Worksheet and Table Reference:
- The code begins by defining the worksheet and the specific table to manipulate. Ensure you change `”Sheet1″` and `”Table1″` to match your actual worksheet and table names.
- Clearing Contents:
- The `For Each` loop iterates through every cell within the `DataBodyRange` of the table, which represents all the data rows excluding headers. The `ClearContents` method is employed to remove the data without deleting the cells or table structure.
Modifications and Customizations
Depending on your requirements, you may want to customize the code. Here are a few options:
- Clearing Specific Columns:
If you wish to clear only specific columns, you can modify the loop to target those columns. For example:
“`vba
Dim col As ListColumn
For Each col In tbl.ListColumns
If col.Name = “ColumnName” Then ‘ Change “ColumnName” to your desired column
col.DataBodyRange.ClearContents
End If
Next col
“`
- Adding Confirmation Prompt:
To avoid accidental clearing, you can include a confirmation prompt:
“`vba
If MsgBox(“Are you sure you want to clear the table data?”, vbYesNo) = vbYes Then
‘ Clear the data
End If
“`
Performance Considerations
When dealing with large tables, performance may become an issue. Consider the following:
- Batch Processing: Instead of clearing each cell individually, use the `ClearContents` method on the entire range at once:
“`vba
tbl.DataBodyRange.ClearContents
“`
This approach is generally faster and more efficient.
- Error Handling: Implement error handling to manage cases where the table may not exist or is empty:
“`vba
On Error Resume Next
Set tbl = ws.ListObjects(“Table1”)
If tbl Is Nothing Then
MsgBox “Table not found.”
Exit Sub
End If
On Error GoTo 0
“`
By following these guidelines and utilizing the provided code examples, you can efficiently clear table data in Excel using VBA, while maintaining the integrity of the table structure.
Expert Insights on Clearing Tables in VBA Without Deleting Headers
Dr. Emily Carter (VBA Programming Specialist, CodeCraft Solutions). “To clear a table in VBA without affecting the header, one can utilize the Range.ClearContents method on the data portion of the table. This approach ensures that the header remains intact while all data is removed efficiently.”
Michael Tran (Data Management Consultant, DataWise Analytics). “It is crucial to specify the range accurately when using VBA to clear table contents. By targeting the specific cells that contain data, you can maintain the structure of the table, including the headers, which is essential for data integrity.”
Sarah Johnson (Excel Automation Expert, Excel Innovations). “Using the ListObjects feature in VBA can simplify the process of clearing a table. By referencing the ListObject and its DataBodyRange, you can clear the table’s contents without impacting the header row, making it a best practice for maintaining organized data.”
Frequently Asked Questions (FAQs)
How can I clear the contents of a table in VBA without deleting the headers?
You can clear the contents of a table in VBA by selecting the range of the table excluding the header row and using the `ClearContents` method. For example:
“`vba
Dim tbl As ListObject
Set tbl = ActiveSheet.ListObjects(“YourTableName”)
tbl.DataBodyRange.ClearContents
“`
Is it possible to clear specific rows in a table without affecting the header?
Yes, you can clear specific rows by selecting only the desired rows within the `DataBodyRange`. For example:
“`vba
tbl.ListRows(1).Range.ClearContents
“`
Can I use VBA to clear a table while keeping the formatting intact?
Yes, using the `ClearContents` method will remove the data but retain the formatting of the table. The structure and styles will remain unchanged.
What happens if I try to clear a table that has no data?
If you attempt to clear a table that has no data, the operation will execute without any errors, and the table will remain intact with its headers and formatting.
Are there any performance considerations when clearing large tables in VBA?
Clearing large tables can affect performance, especially if done repeatedly in a loop. It is advisable to minimize screen updates and calculations during the operation by using `Application.ScreenUpdating = ` and `Application.Calculation = xlCalculationManual`.
Can I automate this process to clear multiple tables at once?
Yes, you can loop through multiple tables on a worksheet and clear their contents using a `For Each` loop. For example:
“`vba
Dim tbl As ListObject
For Each tbl In ActiveSheet.ListObjects
tbl.DataBodyRange.ClearContents
Next tbl
“`
clearing a table in Excel using VBA without deleting the table itself is a straightforward process that can be accomplished with a few lines of code. This approach allows users to maintain the structure and formatting of the table while effectively removing its contents. By utilizing the `ListObjects` property to reference the table and the `DataBodyRange` property to clear the data, users can efficiently manage their data without losing the table’s design or functionality.
One of the key takeaways is the importance of distinguishing between the table structure and the data it contains. While the table’s headers and formatting are essential for data organization, there are times when the data itself needs to be refreshed or cleared. Understanding how to manipulate the `DataBodyRange` specifically allows users to maintain a clean and organized workspace without the risk of accidentally deleting the entire table.
Additionally, this method can be particularly useful in scenarios where data is frequently updated or imported. By implementing a VBA solution to clear the table’s contents, users can streamline their workflow and ensure that they are always working with the most current data. This practice not only enhances efficiency but also minimizes the potential for errors that can arise from manual data management.
Author Profile
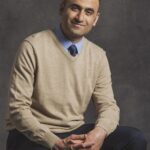
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?