How Can You Use VBA in Outlook to Group Emails by Field?
In the realm of data management and automation, Microsoft Outlook stands out as a powerful tool for organizing communications and managing tasks. For users who are familiar with Visual Basic for Applications (VBA), the ability to manipulate and analyze data within Outlook can significantly enhance productivity. One of the most useful techniques in this context is the ability to group data by specific fields, allowing users to streamline their workflows and gain insights from their email and task lists. Whether you’re looking to categorize emails by sender, date, or subject, mastering the art of grouping in VBA can transform the way you interact with your Outlook data.
Grouping data in Outlook using VBA can seem daunting at first, but it opens up a world of possibilities for customization and efficiency. By leveraging the power of VBA, users can automate repetitive tasks, create dynamic reports, and ultimately save time. This functionality is particularly beneficial for professionals who handle large volumes of emails or tasks, as it allows for quick access to relevant information without the clutter.
As we delve deeper into the topic of grouping by field in Outlook through VBA, we will explore various techniques and practical examples that can help you harness this powerful feature. From understanding the syntax to implementing advanced grouping strategies, this article will equip you with the knowledge needed to elevate your Outlook
Understanding Grouping in VBA for Outlook
Grouping in VBA for Outlook is a powerful feature that allows users to organize data based on specific criteria. This can be particularly useful for managing emails, contacts, or calendar items. By grouping items, users can easily analyze and visualize data, enhancing productivity and decision-making.
When working with Outlook objects in VBA, you can utilize various properties and methods to group items effectively. For instance, if you want to group emails by sender, you can loop through the items in a folder and categorize them accordingly. Below is an example of how to achieve this:
“`vba
Sub GroupEmailsBySender()
Dim olApp As Outlook.Application
Dim olNamespace As Outlook.Namespace
Dim olFolder As Outlook.MAPIFolder
Dim olMail As Outlook.MailItem
Dim GroupDict As Object
Dim Sender As String
Dim Item As Object
Set olApp = New Outlook.Application
Set olNamespace = olApp.GetNamespace(“MAPI”)
Set olFolder = olNamespace.GetDefaultFolder(olFolderInbox)
Set GroupDict = CreateObject(“Scripting.Dictionary”)
For Each Item In olFolder.Items
If TypeOf Item Is Outlook.MailItem Then
Sender = Item.SenderName
If Not GroupDict.Exists(Sender) Then
GroupDict.Add Sender, 1
Else
GroupDict(Sender) = GroupDict(Sender) + 1
End If
End If
Next Item
‘ Output grouped results
Dim Key As Variant
For Each Key In GroupDict.Keys
Debug.Print Key & “: ” & GroupDict(Key) & ” emails”
Next Key
End Sub
“`
This script creates a dictionary to group emails by their sender. It iterates through each email in the inbox, counts the number of emails from each sender, and prints the results to the Immediate Window.
Grouping by Custom Fields
In addition to grouping by standard fields like sender or subject, you can also group items by custom fields. Custom fields can be added to Outlook items, allowing for more tailored data categorization. To group by a custom field, follow these steps:
- Create a Custom Field: Use Outlook’s field customization options to add a new field to your items.
- Access the Field in VBA: Reference the custom field in your VBA code using the appropriate property.
Here’s an example of grouping tasks by a custom field named “Project”:
“`vba
Sub GroupTasksByProject()
Dim olApp As Outlook.Application
Dim olNamespace As Outlook.Namespace
Dim olFolder As Outlook.MAPIFolder
Dim olTask As Outlook.TaskItem
Dim GroupDict As Object
Dim ProjectName As String
Dim Item As Object
Set olApp = New Outlook.Application
Set olNamespace = olApp.GetNamespace(“MAPI”)
Set olFolder = olNamespace.GetDefaultFolder(olFolderTasks)
Set GroupDict = CreateObject(“Scripting.Dictionary”)
For Each Item In olFolder.Items
If TypeOf Item Is Outlook.TaskItem Then
ProjectName = Item.UserProperties(“Project”)
If Not GroupDict.Exists(ProjectName) Then
GroupDict.Add ProjectName, 1
Else
GroupDict(ProjectName) = GroupDict(ProjectName) + 1
End If
End If
Next Item
‘ Output grouped results
Dim Key As Variant
For Each Key In GroupDict.Keys
Debug.Print Key & “: ” & GroupDict(Key) & ” tasks”
Next Key
End Sub
“`
In this example, the script counts tasks based on the value of the “Project” custom field.
Best Practices for Grouping in VBA
When implementing grouping in your VBA scripts, consider the following best practices:
- Optimize Performance: Minimize the number of times you access the Outlook object model within loops, as this can slow down execution.
- Error Handling: Implement error handling to manage unexpected situations, such as missing fields or empty folders.
- User-Friendly Outputs: Format output data for clarity, possibly using message boxes or Excel exports for easier analysis.
Best Practice | Description |
---|---|
Optimize Performance | Reduce object model calls in loops. |
Error Handling | Use structured error handling techniques. |
User-Friendly Outputs | Present results clearly for user comprehension. |
By implementing these techniques, you can leverage the full potential of grouping in VBA for Outlook, streamlining your workflow and enhancing data management capabilities.
Understanding Grouping in VBA for Outlook
Grouping items in Outlook using VBA can streamline the organization of emails, appointments, and tasks based on specific fields. This process often involves the use of the `Items` collection and applying the `GroupBy` method, which helps to segment items for easier access and management.
Key Concepts for Grouping
When implementing grouping in VBA for Outlook, consider the following concepts:
- Items Collection: Represents a collection of Outlook objects such as MailItems, AppointmentItems, etc.
- GroupBy Method: Allows you to group items based on a specified property or field, enhancing data organization.
- Custom Fields: Utilize custom fields for grouping if default properties do not meet your needs.
VBA Code Example for Grouping Emails
The following code snippet demonstrates how to group emails in the Inbox by the sender’s email address:
“`vba
Sub GroupEmailsBySender()
Dim olApp As Outlook.Application
Dim olNamespace As Outlook.Namespace
Dim olFolder As Outlook.MAPIFolder
Dim olItems As Outlook.Items
Dim olMail As Outlook.MailItem
Dim grp As Collection
Dim key As Variant
Set olApp = New Outlook.Application
Set olNamespace = olApp.GetNamespace(“MAPI”)
Set olFolder = olNamespace.GetDefaultFolder(olFolderInbox)
Set olItems = olFolder.Items
Set grp = New Collection
On Error Resume Next
For Each olMail In olItems
If TypeOf olMail Is Outlook.MailItem Then
key = olMail.SenderEmailAddress
grp.Add olMail, key
End If
Next olMail
On Error GoTo 0
‘ Output the grouped emails
For Each key In grp
Debug.Print “Sender: ” & key & ” – Count: ” & grp.Count
Next key
End Sub
“`
Common Properties for Grouping
When grouping items, it’s essential to choose the appropriate properties. Here are some commonly used properties:
Property | Type | Description |
---|---|---|
`SenderEmailAddress` | String | The email address of the sender. |
`ReceivedTime` | Date | The date and time when the email was received. |
`Subject` | String | The subject line of the email. |
`Start` | Date | Start time for calendar appointments. |
`DueDate` | Date | Due date for tasks. |
Advanced Grouping Techniques
For more sophisticated grouping, consider these advanced techniques:
- Nested Grouping: Group by multiple fields. For example, first by `SenderEmailAddress`, then by `ReceivedTime` to categorize emails by sender and time.
- Sorting Grouped Items: After grouping, sort items within each group to enhance accessibility. Use the `Sort` method on the `Items` collection.
- User-defined Properties: Implement user-defined properties for custom grouping scenarios, allowing personalized organization of items.
Debugging and Testing
When developing VBA scripts for grouping, it’s crucial to incorporate debugging practices:
- Use `Debug.Print` to log output to the Immediate Window.
- Implement error handling to manage unexpected runtime errors.
- Test scripts with a small dataset before scaling to ensure accurate grouping.
By understanding and utilizing these principles, users can effectively group Outlook items through VBA, enhancing their workflow and productivity.
Expert Insights on Grouping by Field in VBA for Outlook
Dr. Emily Chen (Senior VBA Developer, Tech Solutions Inc.). “Grouping by field in VBA for Outlook can significantly enhance the organization of email data. By leveraging the built-in Collection objects, developers can create custom groupings that streamline data retrieval and improve user experience.”
Michael Thompson (Outlook Automation Specialist, Office Efficiency Experts). “Implementing grouping functionality in VBA requires a deep understanding of the Outlook object model. Utilizing the ‘Items’ collection allows for effective sorting and filtering, which is crucial for managing large volumes of emails efficiently.”
Sarah Patel (Data Management Consultant, Corporate Insights). “When grouping by field in VBA, it is essential to consider performance implications. Proper indexing and minimizing the number of iterations can lead to significant speed improvements, especially when dealing with extensive datasets within Outlook.”
Frequently Asked Questions (FAQs)
What does “group by field” mean in VBA for Outlook?
“Group by field” in VBA for Outlook refers to the process of organizing items in a collection, such as emails or calendar events, based on a specific property or field, like date, sender, or subject.
How can I group Outlook emails by sender using VBA?
To group Outlook emails by sender using VBA, you can loop through the items in a folder, storing them in a dictionary or collection based on the sender’s email address, then display or process them accordingly.
Is it possible to group calendar items by date in VBA?
Yes, it is possible to group calendar items by date in VBA. You can filter calendar items by their start date and then organize them into collections based on the date for easier access and manipulation.
What are the common fields used for grouping in Outlook VBA?
Common fields used for grouping in Outlook VBA include SenderName, Subject, Start, DueDate, and Categories. These fields allow for effective organization of items based on user needs.
Can I automate grouping in Outlook using a VBA macro?
Yes, you can automate grouping in Outlook using a VBA macro. By writing a macro that processes items in a folder and groups them based on specified fields, you can streamline your workflow and enhance productivity.
Are there any limitations when grouping items in Outlook using VBA?
Yes, limitations may include performance issues with large data sets, the complexity of the grouping logic, and the need for proper error handling to manage unexpected data formats or missing fields.
In the context of using VBA (Visual Basic for Applications) with Microsoft Outlook, grouping by a specific field is a powerful technique that enhances data organization and retrieval. Users can automate the process of sorting and categorizing emails, appointments, or contacts based on various attributes such as date, sender, subject, or any custom field. This functionality not only streamlines workflow but also improves efficiency in managing large volumes of data within Outlook.
Implementing grouping through VBA involves utilizing the Outlook Object Model, where developers can access and manipulate items in folders. By leveraging properties and methods available in the model, users can create scripts that dynamically group items, allowing for better visibility and easier access to related information. This capability is particularly beneficial for users who handle extensive email correspondence or need to track multiple projects simultaneously.
Moreover, understanding how to effectively group items in Outlook using VBA can lead to significant productivity gains. It allows users to focus on relevant information without the distraction of unrelated items. Additionally, mastering this technique can empower users to customize their Outlook experience, tailoring it to their specific needs and preferences. As a result, investing time in learning and implementing VBA for grouping tasks can yield long-term benefits in personal and professional settings.
Author Profile
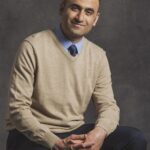
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?