How Can I Use VBA to Clear Slide Notes in PowerPoint?
In the world of presentations, the seamless flow of information is paramount. PowerPoint, a staple tool for professionals and educators alike, offers a plethora of features to enhance the delivery of ideas. Among these features, slide notes play a crucial role, serving as a personal guide for presenters. However, there are times when you might find the need to clear or manage these notes effectively, especially when preparing for a new audience or refining your message. Enter VBA (Visual Basic for Applications), a powerful tool that can automate and streamline your PowerPoint experience. In this article, we will explore how to leverage VBA to clear slide notes effortlessly, ensuring your presentations are always polished and ready for impact.
When working with PowerPoint, slide notes can accumulate over time, leading to clutter and confusion. Whether you’re updating an existing presentation or starting anew, managing these notes can be a daunting task. VBA provides a solution that not only saves time but also enhances your workflow. By utilizing simple scripts, you can clear slide notes in bulk, allowing you to focus on the content that truly matters. This functionality is especially beneficial for those who frequently present on varying topics or need to ensure their notes are concise and relevant.
Moreover, understanding how to manipulate slide notes with VBA opens up a world of
VBA Code to Clear Slide Notes
To effectively clear slide notes in PowerPoint using VBA, you can create a simple macro that iterates through each slide in your presentation and removes any existing notes. This is particularly useful for presentations that need to be updated or repurposed without retaining previous comments or annotations.
Here’s a sample code snippet to achieve this:
“`vba
Sub ClearSlideNotes()
Dim slide As slide
Dim notes As NotesPage
For Each slide In ActivePresentation.Slides
Set notes = slide.NotesPage
notes.Shapes.Placeholders(2).TextFrame.TextRange.Text = “”
Next slide
End Sub
“`
This code will loop through all slides in the active presentation and clear the text of the notes placeholder, effectively removing any notes you may have previously added.
How to Use the VBA Code
To utilize the code provided, follow these steps:
- Open PowerPoint and press `ALT + F11` to open the Visual Basic for Applications editor.
- In the VBA editor, insert a new module:
- Right-click on any of the items in the Project Explorer.
- Click `Insert` > `Module`.
- Copy and paste the above code into the new module window.
- Close the VBA editor.
- Back in PowerPoint, run the macro:
- Press `ALT + F8`, select `ClearSlideNotes`, and click `Run`.
Considerations When Clearing Notes
When clearing slide notes, it is important to keep the following considerations in mind:
- Backup Your Presentation: Always create a backup of your presentation before running any macros that alter content.
- Scope of Change: This macro clears notes for all slides in the active presentation. If you only want to clear notes for specific slides, you will need to modify the code accordingly.
- Undo Functionality: Once notes are cleared, you cannot undo this action through the PowerPoint interface. Ensure that you are certain before executing the macro.
Alternative Approaches
Aside from using VBA, other methods exist to clear slide notes manually:
- Manual Deletion: Navigate to each slide, access the notes pane, and delete the text manually.
- Using PowerPoint’s Interface: Select multiple slides, then access the notes pane for bulk editing, although this is less efficient than using VBA for large presentations.
Method | Efficiency | Ease of Use |
---|---|---|
VBA Macro | High | Moderate (Requires VBA Knowledge) |
Manual Deletion | Low | High |
PowerPoint Interface | Medium | High |
By implementing these methods, you can efficiently manage slide notes in your PowerPoint presentations, ensuring that your content remains relevant and uncluttered.
Clearing Slide Notes in PowerPoint with VBA
To clear slide notes in a PowerPoint presentation using VBA (Visual Basic for Applications), you can utilize the `NotesPage` object associated with each slide. This method allows for the efficient removal of notes from all slides within a presentation or from specific slides as needed.
VBA Code to Clear Notes from All Slides
The following VBA code snippet demonstrates how to iterate through all slides in the active presentation and clear their notes:
“`vba
Sub ClearAllSlideNotes()
Dim slide As slide
For Each slide In ActivePresentation.Slides
slide.NotesPage.Shapes.Placeholders(2).TextFrame.TextRange.Text = “”
Next slide
End Sub
“`
Explanation of the Code:
- `Dim slide As slide`: Declares a variable to represent each slide.
- `For Each slide In ActivePresentation.Slides`: Loops through each slide in the active presentation.
- `slide.NotesPage.Shapes.Placeholders(2).TextFrame.TextRange.Text = “”`: Clears the text in the notes placeholder.
VBA Code to Clear Notes from Specific Slides
If you need to clear notes from specific slides, you can modify the code to target certain slide indexes. Here’s how you can do it:
“`vba
Sub ClearSpecificSlideNotes()
Dim slideIndex As Integer
Dim specificSlides As Variant
specificSlides = Array(1, 3, 5) ‘ Specify slide numbers here
For slideIndex = LBound(specificSlides) To UBound(specificSlides)
With ActivePresentation.Slides(specificSlides(slideIndex)).NotesPage.Shapes.Placeholders(2).TextFrame.TextRange
.Text = “”
End With
Next slideIndex
End Sub
“`
Key Features of the Code:
- `specificSlides = Array(1, 3, 5)`: Defines an array of slide numbers where notes will be cleared.
- `ActivePresentation.Slides(specificSlides(slideIndex)).NotesPage.Shapes.Placeholders(2)`: Accesses the notes for the specified slides.
Running the VBA Code
To run the provided VBA code, follow these steps:
- Open PowerPoint.
- Press `ALT + F11` to open the VBA editor.
- Insert a new module by right-clicking on any item in the Project Explorer, selecting `Insert`, then `Module`.
- Copy and paste the desired code into the module window.
- Close the VBA editor.
- Press `ALT + F8`, select the macro you wish to run, and click `Run`.
Considerations
- Ensure that you save your presentation before running the macro, as this action cannot be undone.
- If the slides do not contain notes, the code will simply skip those slides without error.
- Adjust the slide numbers in the array as needed based on your presentation structure.
Additional Features
You can enhance your VBA script by adding features such as:
- User Confirmation: Prompting the user before clearing notes.
- Logging: Keeping a log of which slides had their notes cleared for future reference.
- Undo Functionality: Implementing a way to restore cleared notes if necessary.
By utilizing these techniques, you can effectively manage slide notes in your PowerPoint presentations using VBA.
Expert Insights on Clearing Slide Notes in VBA for PowerPoint
Emily Carter (Senior VBA Developer, Tech Solutions Inc.). “To effectively clear slide notes in PowerPoint using VBA, one can utilize the Slide object’s NotesPage property. This allows for direct access to the notes and facilitates the removal of any content programmatically, ensuring a clean presentation without manual intervention.”
James Lin (Presentation Specialist, Creative Media Group). “Automating the process of clearing slide notes through VBA not only saves time but also reduces the risk of human error. Implementing a simple loop through the Slides collection can efficiently target and clear notes across multiple slides in a presentation.”
Laura Bennett (Office Automation Consultant, Productivity Experts). “For users looking to streamline their PowerPoint workflow, mastering VBA to clear slide notes is crucial. It enhances the overall quality of presentations by allowing for quick adjustments, especially when dealing with large sets of slides that require consistent formatting.”
Frequently Asked Questions (FAQs)
How can I clear slide notes in PowerPoint using VBA?
You can clear slide notes in PowerPoint using VBA by iterating through each slide and setting the `NotesPage` text to an empty string. Use the following code snippet:
“`vba
Dim slide As slide
For Each slide In ActivePresentation.Slides
slide.NotesPage.Shapes.Placeholders(2).TextFrame.TextRange.Text = “”
Next slide
“`
Is it possible to remove notes from specific slides only?
Yes, you can remove notes from specific slides by referencing the slide index in your VBA code. For example, to clear notes from slide 2, use:
“`vba
ActivePresentation.Slides(2).NotesPage.Shapes.Placeholders(2).TextFrame.TextRange.Text = “”
“`
Will clearing slide notes affect the presentation content?
No, clearing slide notes will not affect the visible content of the slides. It only removes the text associated with the notes, which are not displayed during the presentation.
Can I undo the action of clearing slide notes in PowerPoint?
Once you run the VBA code to clear slide notes, the action cannot be undone through the PowerPoint interface. It is advisable to create a backup of your presentation before executing the code.
What should I do if I encounter an error when running the VBA code?
If you encounter an error, ensure that you have the correct references set in the VBA editor. Also, check that your PowerPoint presentation is not in a read-only state and that the slide index you are referencing exists.
Are there any limitations to clearing slide notes via VBA?
Yes, limitations include the inability to selectively clear notes based on certain criteria without additional coding. Furthermore, if the slide contains multiple placeholders, you may need to adjust the code to target the correct one.
In summary, utilizing VBA (Visual Basic for Applications) in PowerPoint provides a powerful means to manipulate slide notes effectively. Users can automate the process of clearing slide notes, which can be particularly useful when preparing presentations for different audiences or when needing to remove outdated information quickly. The ability to programmatically access and modify slide notes enhances efficiency and ensures that presentations remain relevant and focused.
One of the key takeaways is the simplicity of the VBA code required to clear slide notes. By employing a straightforward script, users can iterate through each slide in a presentation and clear the notes section with minimal effort. This capability not only saves time but also reduces the risk of human error when manually editing slide notes, thereby streamlining the presentation preparation process.
Additionally, understanding how to manipulate slide notes through VBA opens up further opportunities for customization and automation within PowerPoint. Users can create more complex scripts to manage slide content, ensuring that their presentations are not only clear of unnecessary notes but also tailored to meet specific presentation goals. Overall, mastering VBA for PowerPoint enhances a user’s ability to create polished and professional presentations efficiently.
Author Profile
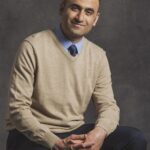
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?