How Can You Send Values to a UserForm Using VBA?
Visual Basic for Applications (VBA) is a powerful tool that empowers users to automate tasks and enhance functionality within Microsoft Office applications. One of the most dynamic features of VBA is its ability to create userforms, which serve as interactive interfaces for data entry and user interaction. However, many users often find themselves grappling with the question: how do you effectively send values from your VBA code to these userforms? This article delves into the nuances of this process, unraveling the techniques and best practices that will elevate your VBA projects.
When working with userforms in VBA, the ability to send values dynamically can significantly streamline your workflow and improve user experience. Whether you’re populating fields with data from a spreadsheet or retrieving user input for further processing, understanding how to manipulate values within userforms is essential. This article will guide you through the fundamental concepts of transferring data between your VBA code and userform controls, ensuring that you can create responsive and user-friendly applications.
As we explore this topic, we will touch on various methods for passing values, including direct assignments and leveraging event-driven programming. By mastering these techniques, you can unlock the full potential of your userforms, making them not only functional but also intuitive for users. Prepare to enhance your VBA skills and transform your
Sending Values to UserForms
When working with VBA in Excel, sending values to a UserForm can enhance user interaction and streamline data entry processes. This involves programmatically populating controls on the UserForm with data, which can be achieved through various methods, depending on the context.
Methods for Sending Values
There are several approaches to send values to controls within a UserForm:
- Direct Assignment: You can directly assign values to the properties of controls on the UserForm.
- Using Variables: Store values in variables before assigning them to controls.
- Loading Data from Sheets: Populate UserForm controls with data fetched from Excel worksheets.
Direct Assignment Example
To illustrate direct assignment, consider a UserForm containing a TextBox named `txtName`. The following code snippet demonstrates how to set its value:
“`vba
UserForm1.txtName.Value = “John Doe”
“`
This method is straightforward and effective for static values.
Using Variables
Variables can be used when the data to be sent is dynamic. For instance, if you are retrieving user input from a worksheet, you can store it in a variable and then assign it to the UserForm controls.
“`vba
Dim userName As String
userName = Sheets(“Data”).Range(“A1”).Value
UserForm1.txtName.Value = userName
“`
Loading Data from Sheets
When populating a UserForm with multiple data points, it is efficient to retrieve values from a worksheet. The following example demonstrates how to load various fields into a UserForm upon initialization:
“`vba
Private Sub UserForm_Initialize()
txtName.Value = Sheets(“Data”).Range(“A1”).Value
txtAge.Value = Sheets(“Data”).Range(“B1”).Value
txtEmail.Value = Sheets(“Data”).Range(“C1”).Value
End Sub
“`
This method is particularly useful when dealing with forms that require multiple inputs.
Example Table for UserForm Control Mappings
The following table outlines common UserForm controls and their corresponding properties:
Control Type | Property to Set | Example Code |
---|---|---|
TextBox | Value | txtName.Value = “John Doe” |
ComboBox | Value | cmbOptions.Value = “Option1” |
ListBox | Selected | lstItems.Selected(0) = True |
CheckBox | Value | chkAgree.Value = True |
By utilizing these methods and following the examples provided, you can efficiently send values to UserForm controls, enhancing the functionality and user experience of your VBA applications.
Understanding UserForms in VBA
UserForms in VBA (Visual Basic for Applications) are custom dialog boxes that allow users to interact with applications in a more user-friendly manner. They can capture input, display information, and even control the flow of a program. To effectively send values to a UserForm, it is essential to understand how to manipulate its properties and controls.
Setting Up a UserForm
Before sending values to a UserForm, you must ensure that it is properly set up. Follow these steps to create a UserForm in Excel VBA:
- Open the Visual Basic for Applications editor (ALT + F11).
- Insert a new UserForm:
- Right-click on any of the items in the Project Explorer.
- Select `Insert` and then `UserForm`.
- Add controls such as TextBoxes, Labels, and Buttons from the Toolbox.
Sending Values to Controls
To send values to the controls on a UserForm, you need to reference the UserForm and its controls within your VBA code. Below are examples demonstrating how to send values to different types of controls.
TextBox Example
To send a value to a TextBox control:
“`vba
UserForm1.TextBox1.Value = “Your Value Here”
“`
Label Example
To send a value to a Label control:
“`vba
UserForm1.Label1.Caption = “Your Label Text”
“`
ComboBox Example
To set the selected value of a ComboBox:
“`vba
UserForm1.ComboBox1.Value = “Your ComboBox Value”
“`
Using a Subroutine to Populate the UserForm
You can create a subroutine to populate your UserForm with values dynamically. This approach enhances reusability and organization. Here’s an example:
“`vba
Sub ShowUserFormWithValues()
UserForm1.TextBox1.Value = “John Doe”
UserForm1.Label1.Caption = “User Information”
UserForm1.ComboBox1.AddItem “Option 1”
UserForm1.ComboBox1.AddItem “Option 2”
UserForm1.ComboBox1.Value = “Option 1”
UserForm1.Show
End Sub
“`
Call this subroutine to display the UserForm with the specified values.
Passing Values from a Worksheet to a UserForm
In many cases, you may want to send values from a worksheet to a UserForm. Here’s how to retrieve values from a specific cell and display them in the UserForm:
“`vba
Sub LoadValuesToUserForm()
UserForm1.TextBox1.Value = Sheets(“Sheet1”).Range(“A1”).Value
UserForm1.Label1.Caption = Sheets(“Sheet1”).Range(“B1”).Value
UserForm1.Show
End Sub
“`
This example retrieves the values from cells A1 and B1 in “Sheet1” and assigns them to the UserForm controls.
Best Practices for UserForms
When working with UserForms, consider the following best practices:
- Clear Values: Ensure that controls are cleared before loading new data to avoid confusion.
- Validation: Implement input validation to ensure users enter the correct type of data.
- User Guidance: Use Labels and ToolTips effectively to guide users on what input is expected.
- Error Handling: Include error handling to manage unexpected issues during data entry.
Implementing these techniques will allow for a seamless interaction between your VBA code and UserForms, enhancing the user experience and functionality of your applications.
Expert Insights on Sending Values to UserForms in VBA
Dr. Emily Carter (Senior VBA Developer, Tech Solutions Inc.). “When sending values to a UserForm in VBA, it’s crucial to ensure that the controls on the UserForm are properly initialized. This can be achieved by using the ‘Initialize’ event of the UserForm, which allows you to set the values dynamically based on the context of the application.”
Mark Thompson (Lead Software Engineer, CodeCraft Ltd.). “Utilizing the ‘Show’ method of the UserForm in conjunction with public variables can streamline the process of passing values. This approach not only enhances code readability but also maintains the integrity of data throughout the UserForm’s lifecycle.”
Linda Nguyen (VBA Consultant, Excel Experts Group). “It’s essential to validate the data before sending it to the UserForm. Implementing error handling mechanisms ensures that any discrepancies in the data are caught early, preventing runtime errors and enhancing user experience.”
Frequently Asked Questions (FAQs)
What is a UserForm in VBA?
A UserForm in VBA is a custom dialog box that allows users to interact with a program through a graphical interface. It can contain various controls such as text boxes, buttons, and labels for data entry and display.
How can I send a value to a UserForm in VBA?
You can send a value to a UserForm by creating a public property or method within the UserForm’s code. This allows you to set the value from another module or procedure before displaying the UserForm.
What is the syntax to set a value in a UserForm control?
The syntax to set a value in a UserForm control is `UserFormName.ControlName.Value = YourValue`. Replace `UserFormName` with the name of your UserForm, `ControlName` with the specific control’s name, and `YourValue` with the value you want to assign.
Can I pass multiple values to a UserForm?
Yes, you can pass multiple values to a UserForm by defining multiple public properties or by using a method that accepts parameters. This allows you to set various controls within the UserForm simultaneously.
How do I show the UserForm after setting values?
After setting the desired values in the UserForm controls, you can display the UserForm using the `UserFormName.Show` method. This will render the UserForm on the screen for user interaction.
What should I do if the UserForm does not update with the new values?
If the UserForm does not update with the new values, ensure that you are setting the values before calling the `Show` method. Additionally, check for any code that might reset the controls after you set the values.
sending values to a UserForm in VBA is a fundamental aspect of creating interactive and user-friendly applications within Microsoft Excel. This process allows developers to populate form controls with data dynamically, enhancing the overall functionality of the UserForm. By utilizing the appropriate VBA code, developers can efficiently transfer values from worksheets, variables, or other sources directly to the UserForm controls, such as text boxes, labels, and combo boxes.
Moreover, understanding how to manipulate UserForms with VBA not only improves the user experience but also streamlines data entry and retrieval processes. By implementing event-driven programming techniques, developers can respond to user actions effectively, ensuring that the UserForm behaves as intended. This capability is essential for creating robust applications that meet specific user needs and requirements.
Key takeaways from this discussion include the importance of properly initializing UserForm controls with relevant data and ensuring that the data flow between the UserForm and the underlying Excel workbook is seamless. Additionally, leveraging techniques such as the ‘Initialize’ event and using properties of form controls can significantly enhance the performance and usability of the UserForm. Overall, mastering these concepts is crucial for any VBA developer aiming to create sophisticated and efficient Excel applications.
Author Profile
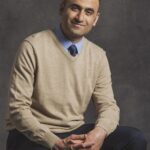
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?