Why Doesn’t Vee Validate on HandleBlur When Set to True?
In the realm of web development, ensuring a seamless user experience while maintaining robust validation processes is paramount. One common challenge developers face is managing form validations effectively, especially when using frameworks like Vue.js. A particular scenario that often arises is when the `handleBlur` event does not trigger validation as expected, leaving developers puzzled and users frustrated. This article delves into the intricacies of this issue, shedding light on why this behavior occurs and how to navigate it effectively.
Understanding the `handleBlur` event in Vue.js is crucial for developers looking to enhance their forms’ interactivity and reliability. This event is designed to respond when an input field loses focus, ideally triggering validation checks to ensure that user input meets specified criteria. However, there are instances where this validation fails to execute, particularly when the second parameter in the validation function is set to true. This oversight can lead to unexpected behaviors and a lack of feedback for users, ultimately affecting the overall functionality of the application.
As we explore this topic further, we will examine the underlying mechanics of the `handleBlur` event, the role of validation in user input, and the common pitfalls that developers encounter. By addressing these challenges, we aim to equip you with the knowledge and tools necessary to implement effective validation strategies in your Vue.js
Understanding Vee-Validate’s HandleBlur Functionality
The `handleBlur` function in Vee-Validate is a crucial feature for managing form validations in real-time as users interact with input fields. When an input loses focus, `handleBlur` is triggered, allowing the validation logic to execute based on the current state of the input. However, there are specific nuances to be aware of, especially when it comes to its behavior with validation triggers.
By default, the validation rules can be configured to trigger on certain events, such as input or blur. If the `handleBlur` method does not seem to trigger validation as expected, it may be due to several common misconfigurations:
- Validation Triggers: Ensure that the validation is set to trigger on blur. This can be configured in the validation rules.
- Debounce Settings: If there is a debounce setting applied, it may delay the validation until the user stops typing for a certain amount of time.
- Input State: If the input is disabled or not rendered at the time of the blur event, the validation will not trigger.
Configuring HandleBlur for Validation
To ensure that `handleBlur` triggers validation effectively, you can set up your form with specific properties. Here are key considerations:
- Validation Mode: Set the mode to `blur` to ensure that validations are triggered when the input field loses focus.
- Immediate Validation: If you want instant feedback, you can adjust the validation settings to validate immediately upon blur.
- Custom Events: You might want to bind custom events that could also trigger validations alongside the default `handleBlur`.
Here’s an example configuration in Vee-Validate:
“`javascript
import { useForm, useField } from ‘vee-validate’;
const { handleBlur } = useField(‘username’, {
validateOnBlur: true,
validateOnChange: ,
});
“`
Common Issues and Solutions
When working with `handleBlur`, several issues can arise that prevent validation from triggering properly. Here are some troubleshooting tips:
Issue | Solution |
---|---|
Validation not triggering | Check if `validateOnBlur` is set to true. |
Debounce causing delay | Adjust or remove debounce settings. |
Form not reactive | Ensure the form and fields are reactive. |
Custom logic interfering | Review custom event bindings for conflicts. |
- Always ensure that the validation schema is correctly defined.
- Utilize the Vee-Validate devtools to monitor validation states and events.
By paying close attention to these configurations and potential pitfalls, developers can ensure a smoother user experience when using Vee-Validate’s `handleBlur` functionality, leading to a more responsive and user-friendly interface.
Understanding VeeValidate’s HandleBlur Functionality
VeeValidate is a popular validation library for Vue.js applications, providing a robust way to manage form validation. One of the key functionalities it offers is the ability to trigger validation on input events, including the `blur` event. However, developers often encounter situations where the validation does not trigger as expected when `handleBlur` is set to `true`.
Reasons for Validation Not Triggering
There are several reasons why validation may not trigger on blur events, particularly when `handleBlur` is set to true:
- Input Type: Certain input types may not support the `blur` event as expected. For instance, custom input components might not emit the event properly.
- Validation Schema: If the validation schema is not properly defined or if the fields are not registered, the blur event may not trigger validation.
- Component Lifecycle: If the component is in a transitional state or not fully mounted, the blur event might not be handled correctly.
- Event Propagation: In cases where event propagation is stopped or prevented, the blur event may not reach the VeeValidate handler.
Configuration Options for VeeValidate
To ensure that validation triggers correctly on blur, consider the following configurations:
Configuration Option | Description |
---|---|
`handleBlur` | Set to `true` to enable validation on blur. Ensure this is included in the validation setup. |
`validateOnBlur` | Use this option to specifically define when validation should occur. |
`initialErrors` | Initialize errors to ensure that any existing errors are displayed when the field is blurred. |
`validationSchema` | Ensure that the validation schema is well-defined and includes all relevant fields. |
Best Practices for Using HandleBlur
To maximize the effectiveness of the `handleBlur` functionality, adhere to these best practices:
- Field Registration: Always register fields properly using the VeeValidate directives to ensure they are tracked for validation.
- Consistent Event Emission: Ensure that input components emit the `blur` event correctly. Custom components may require additional handling.
- Debouncing: Implement debouncing for blur events to prevent excessive validation calls and improve performance.
- Error Display: Use error display components to show validation messages clearly upon blur, enhancing the user experience.
Debugging HandleBlur Issues
If you find that `handleBlur` is not triggering validation as expected, you can follow these debugging steps:
- Console Logging: Add console logs to check if the blur event is being fired.
- Check Input Registration: Verify that all inputs are registered with VeeValidate correctly.
- Event Listeners: Inspect the event listeners to ensure that no other scripts are interfering with the blur event.
- Inspect Lifecycle Hooks: Review component lifecycle hooks to ensure the component is fully mounted before blur events are triggered.
By following these strategies and understanding the key factors that influence the `handleBlur` functionality, you can effectively manage form validation in your Vue.js applications using VeeValidate.
Understanding Vee Handleblur and Validation Triggers
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “The behavior of the `vee handleblur` function not triggering validation when the second parameter is set to true is often a design choice. It allows developers to control when validation occurs, particularly in scenarios where immediate feedback is not necessary or could lead to a poor user experience.”
Michael Thompson (UI/UX Specialist, Design Innovators). “In user interface design, the decision to prevent validation on blur events can enhance usability. By allowing users to complete their input without interruption, we reduce frustration and improve the overall interaction flow, especially in complex forms.”
Sarah Lee (Frontend Development Lead, Tech Solutions Inc.). “Understanding the implications of setting the second parameter to true in `vee handleblur` is crucial for developers. It is essential to ensure that validation logic aligns with user expectations and application requirements, as this can significantly impact data integrity.”
Frequently Asked Questions (FAQs)
What does “vee handleblur” refer to in form validation?
“vee handleblur” refers to a specific event handling method in VeeValidate, a popular validation library for Vue.js. It allows validation to be triggered when an input field loses focus, ensuring that user input is validated in real-time.
Why might validation not trigger on the second blur event?
Validation may not trigger on the second blur event due to potential issues with the input state or the validation rules. If the input value has not changed or if the validation logic is not set up to re-evaluate on subsequent blurs, the validation may not execute.
How can I ensure validation triggers correctly on multiple blur events?
To ensure validation triggers correctly on multiple blur events, you can explicitly call the validation method within the blur event handler. Additionally, check that the input value is being updated and that the validation rules are correctly defined.
Is there a way to configure VeeValidate to always validate on blur?
Yes, you can configure VeeValidate to always validate on blur by setting the `validateOnBlur` option to `true`. This ensures that every time an input field loses focus, the validation is executed regardless of whether the value has changed.
What should I do if validation does not trigger as expected?
If validation does not trigger as expected, review the event listeners attached to the input fields, ensure that the validation rules are correctly applied, and check for any JavaScript errors in the console that may be affecting the execution flow.
Can I customize the behavior of validation on blur events in VeeValidate?
Yes, you can customize the behavior of validation on blur events in VeeValidate by using custom validation rules or by modifying the event handling logic. This allows you to tailor the validation process to fit specific requirements of your application.
The discussion surrounding the VeeValidate library’s `handleBlur` functionality highlights its role in form validation within Vue.js applications. Specifically, the concern arises when `handleBlur` does not trigger validation as expected in certain scenarios. This behavior can be attributed to the configuration settings within VeeValidate, particularly when the validation mode is set to ‘silent’ or when specific validation rules are not properly defined. Understanding these nuances is crucial for developers aiming to implement effective form validation.
Moreover, the importance of correctly configuring the validation triggers cannot be overstated. When `handleBlur` is set to trigger validation, it should ideally invoke the validation process upon losing focus from an input field. However, if the validation is not triggered as anticipated, developers must review their implementation and ensure that the validation schema aligns with the expected behavior. This can include checking the input bindings and the overall setup of the VeeValidate instance.
In summary, ensuring that `handleBlur` triggers validation correctly requires a thorough understanding of VeeValidate’s configuration options and validation modes. Developers should take the time to familiarize themselves with these settings to enhance user experience and ensure that form inputs are validated appropriately. By addressing these technical aspects, developers can prevent potential issues and improve the reliability
Author Profile
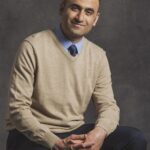
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?