Why Doesn’t ‘vite tsconfig vitest/globals’ Work with Webpack?
In the ever-evolving landscape of web development, the tools we choose can significantly impact our workflow and productivity. Among the most popular choices today are Vite and Webpack, both of which offer unique advantages for building modern applications. However, as developers increasingly adopt TypeScript and test frameworks like Vitest, challenges can arise, particularly when it comes to configuring TypeScript settings effectively. One common issue that many encounter is the integration of `vitest/globals` in their TypeScript configuration, especially when transitioning from Webpack to Vite.
This article delves into the nuances of configuring TypeScript with Vite and highlights the common pitfalls developers face, particularly around the `tsconfig.json` settings. We’ll explore how the differences between Vite and Webpack can lead to confusion, especially for those accustomed to the latter’s configuration style. Understanding these intricacies is crucial for ensuring that your testing environment runs smoothly and efficiently.
As we unpack these topics, we will provide insights into best practices for configuring your TypeScript environment with Vite, alongside practical tips for leveraging Vitest effectively. Whether you’re a seasoned developer or just starting, this guide will equip you with the knowledge to navigate the complexities of modern web development and optimize your setup for success.
Understanding Vite and TypeScript Configuration
Vite is a modern build tool that provides a fast development environment and optimized production builds for web applications. When using TypeScript with Vite, developers often encounter specific configurations that can impact the testing setup, especially when integrating with libraries like Vitest.
To ensure that TypeScript works seamlessly with Vite, it is essential to configure the `tsconfig.json` file correctly. This file provides TypeScript with the necessary information about the project structure and compiler options.
Key configurations to consider in `tsconfig.json` include:
- Compiler Options:
- `target`: Specifies the ECMAScript target version. Common settings are `ESnext` or `ES2020`.
- `module`: Set to `ESNext` for compatibility with Vite.
- `jsx`: Set to `preserve` or `react-jsx` for React projects.
- Include/Exclude: Define which files to include or exclude in the compilation process.
Here’s an example of a simplified `tsconfig.json` for a Vite project:
“`json
{
“compilerOptions”: {
“target”: “ESNext”,
“module”: “ESNext”,
“jsx”: “react-jsx”,
“strict”: true,
“moduleResolution”: “node”,
“esModuleInterop”: true,
“skipLibCheck”: true,
“forceConsistentCasingInFileNames”: true
},
“include”: [“src//*.ts”, “src//*.tsx”],
“exclude”: [“node_modules”]
}
“`
Integrating Vitest with Vite and TypeScript
Vitest is a fast unit testing framework designed for Vite, optimized for TypeScript projects. Configuring Vitest to work with Vite requires setting up a specific environment that includes global definitions for testing.
To ensure the globals from Vitest are recognized in your TypeScript project, you may need to modify your `tsconfig.json` as follows:
- Adding Vitest Globals:
- Include the Vitest types in your `tsconfig.json` to avoid type errors during testing.
Add the following under `compilerOptions`:
“`json
{
“compilerOptions”: {
“types”: [“vitest/globals”]
}
}
“`
This change allows you to use Vitest’s testing functions globally, such as `describe`, `it`, and `expect`, without needing to import them in every test file.
Troubleshooting Configuration Issues
When transitioning from Webpack to Vite, developers may face challenges with configuration and compatibility. Here are some common issues and solutions:
Issue | Potential Solution |
---|---|
TypeScript types not recognized | Ensure `types` in `tsconfig.json` includes `vitest/globals`. |
Module resolution errors | Check `module` and `moduleResolution` options in `tsconfig.json`. |
React JSX not compiling | Set `jsx` to `react-jsx` in `tsconfig.json`. |
Slow test execution | Use Vitest’s built-in features like test caching and parallel execution. |
By addressing these issues and making the necessary configurations, developers can leverage the full potential of Vite and Vitest in TypeScript projects, ensuring a smooth development and testing experience.
Understanding Vite and Vitest with TypeScript
Vite is a modern build tool that significantly enhances development speed and experience, especially when used with frameworks like Vue or React. It leverages native ES modules and provides a fast hot module replacement (HMR) feature. Vitest, on the other hand, is a testing framework designed to work seamlessly with Vite, enabling developers to write tests in a TypeScript-friendly environment.
To configure TypeScript with Vitest, the `tsconfig.json` file must include certain settings to ensure compatibility. Here are essential settings to consider:
- Compiler Options:
- `”target”: “ESNext”`: This setting allows you to use the latest JavaScript features.
- `”module”: “ESNext”`: This is crucial for Vite to work with ES modules.
- `”jsx”: “preserve”`: If you’re using React, this ensures JSX is correctly compiled.
- Include Vitest Types:
- Add `vite` and `vitest` types to the `types` array in the `tsconfig.json`:
“`json
{
“compilerOptions”: {
“types”: [“vite”, “vitest/globals”]
}
}
“`
Common Issues with Vitest and Webpack
While Vite and Vitest are designed to work together efficiently, there are common pitfalls when transitioning from Webpack to Vite, particularly regarding test setups. The following issues often arise:
- Global Variables: Vitest provides global test functions like `describe`, `it`, and `expect`. If these are not recognized, ensure that the `vitest/globals` type is included in your `tsconfig.json`.
- Module Resolution: Unlike Webpack, which uses a different module resolution strategy, Vite relies on native ESM. This can lead to discrepancies if your imports are not properly structured.
- Configuration Differences: Webpack configurations can be complex, often involving multiple loaders and plugins. Vite simplifies this by using a plugin-based architecture, which can lead to confusion if not adjusted correctly.
Here’s a comparison of configuration aspects between Vite and Webpack:
Aspect | Vite | Webpack |
---|---|---|
Configuration File | `vite.config.ts` | `webpack.config.js` |
Module Format | ESM | CommonJS |
Hot Module Replacement | Built-in | Requires `HotModuleReplacementPlugin` |
Testing Integration | Vitest | Jest or Mocha with plugins |
Debugging Tips for Vitest and TypeScript
When encountering issues with Vitest and TypeScript configurations, the following debugging tips can assist in resolving common problems:
- Check TypeScript Version: Ensure you are using a compatible version of TypeScript that supports the latest features required by Vite and Vitest.
- Clear Cache: Sometimes, cached files may cause unexpected behavior. Clear the Vite cache by running:
“`bash
rm -rf node_modules/.vite
“`
- Verbose Logging: Use the `–debug` flag when running Vitest to get more detailed logs, which can provide insights into what might be going wrong.
- Community Resources: Leverage forums, GitHub issues, and the Vite community for troubleshooting specific errors. Often, others have faced similar challenges and can offer solutions.
By understanding these configurations and potential issues, developers can effectively utilize Vite and Vitest in TypeScript projects while avoiding common pitfalls associated with transitioning from Webpack.
Understanding Vite, TypeScript, and Testing Frameworks
Dr. Emily Chen (Senior Software Engineer, Vite Core Team). “When integrating Vite with TypeScript, it is crucial to ensure that your tsconfig.json is properly configured to include the necessary paths and types for Vitest. If globals are not recognized, it often indicates a misconfiguration in your TypeScript settings or missing type definitions.”
Mark Thompson (Lead Developer, Modern Web Solutions). “The transition from Webpack to Vite can introduce challenges, especially with testing frameworks like Vitest. Developers should be aware that certain Webpack-specific configurations may not translate directly. Ensuring that Vitest is set up to recognize TypeScript globals requires careful attention to the testing environment setup.”
Sarah Patel (Technical Consultant, Frontend Innovations). “Common pitfalls when using Vitest with TypeScript include not including the Vitest types in your tsconfig.json. If you encounter issues with globals not working, verify that your TypeScript compiler options are aligned with Vitest’s requirements, and consider reviewing the documentation for any updates or changes.”
Frequently Asked Questions (FAQs)
What is the purpose of the `tsconfig.json` file in a Vite project?
The `tsconfig.json` file in a Vite project configures TypeScript options, including compiler settings, module resolution, and type checking. It ensures that TypeScript compiles the code according to the specified parameters.
How does Vite differ from Webpack in terms of configuration?
Vite offers a more streamlined configuration process compared to Webpack. It utilizes native ES modules for development, resulting in faster builds and hot module replacement. Webpack requires more extensive configuration and setup for similar functionalities.
What are `vitest/globals` and how are they used in a Vite project?
`vitest/globals` provides global testing utilities when using Vitest, a Vite-native testing framework. It allows developers to write tests without needing to import testing functions explicitly, simplifying the test writing process.
Why might `vitest/globals` not work as expected in a Vite project?
If `vitest/globals` does not work, it may be due to incorrect configuration in `tsconfig.json`, missing dependencies, or issues with the test environment setup. Ensuring that Vitest is properly installed and configured is crucial for functionality.
Can I use Vite with existing Webpack configurations?
While it is possible to migrate a project from Webpack to Vite, Vite does not directly support Webpack configurations. Developers must adapt their configurations to align with Vite’s ecosystem and features.
What steps should I take if I encounter issues with TypeScript in Vite?
If issues arise with TypeScript in Vite, verify the `tsconfig.json` settings, ensure all necessary TypeScript dependencies are installed, and check for compatibility with Vite plugins. Debugging TypeScript errors often requires reviewing the specific error messages for guidance.
In the context of using Vite with TypeScript, the integration of Vitest and the configuration of the tsconfig file can present challenges, particularly when compared to traditional setups like Webpack. Vite, being a modern build tool, leverages native ES modules and offers a different approach to project configuration. This can lead to discrepancies in how global types and testing environments are set up, especially for developers transitioning from Webpack to Vite.
One of the primary issues arises from the need to configure the tsconfig.json file correctly to recognize Vitest’s global types. Unlike Webpack, which may rely heavily on plugins and loaders, Vite requires a more streamlined configuration that can sometimes overlook necessary type declarations. Developers must ensure that their tsconfig includes the appropriate paths and types for Vitest to function correctly, which can be a source of confusion for those unfamiliar with Vite’s ecosystem.
Key takeaways from this discussion include the importance of understanding the differences in configuration between Vite and Webpack. Developers should familiarize themselves with Vite’s documentation regarding TypeScript and Vitest integration to avoid common pitfalls. Additionally, leveraging community resources and examples can provide valuable insights into best practices for setting up a Vite project with Vitest, ensuring
Author Profile
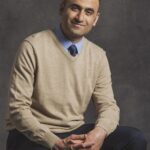
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?