Why Isn’t My Vue Computed Switch Working? Troubleshooting Tips You Need!
In the dynamic world of Vue.js, developers often encounter various challenges that can hinder their productivity and the functionality of their applications. One such issue that frequently arises is the perplexing behavior of computed properties, particularly when used in conjunction with switch statements. If you’ve found yourself scratching your head over why your computed properties aren’t responding as expected, you’re not alone. This article delves into the common pitfalls and misconceptions surrounding computed properties and switch statements in Vue, providing you with the insights needed to troubleshoot and optimize your code effectively.
Computed properties are a powerful feature in Vue.js, allowing developers to define reactive properties based on other data sources. However, when integrating switch statements within these computed properties, many developers experience unexpected results or outright failures. Understanding the nuances of reactivity in Vue and how switch statements interact with computed properties is crucial for building robust applications.
In this article, we will explore the underlying principles of Vue’s reactivity system, the role of computed properties, and the common mistakes that can lead to confusion when using switch statements. By the end, you’ll be equipped with the knowledge to troubleshoot your issues and enhance the functionality of your Vue applications, ensuring that your code works as intended and delivers a seamless user experience.
Common Reasons for Vue Computed Properties Not Working
When working with Vue.js, developers occasionally encounter issues with computed properties that fail to behave as expected. Understanding the common pitfalls can help in diagnosing and resolving these issues effectively. Here are some prevalent reasons:
- Incorrect Dependency Tracking: Computed properties rely on Vue’s reactivity system, which means that they must be dependent on reactive data. If the dependencies are not reactive, the computed property will not update.
- Using Non-Reactive Data: If you assign a computed property to a non-reactive data source or if the data source is not part of the Vue instance, the computed property will not trigger updates.
- Errors in Getter Functions: Any errors in the logic within the getter function of a computed property can prevent it from functioning correctly. Ensure that the function is returning the expected value without throwing errors.
- Modification of Computed Properties: Computed properties should only be used for reading data. If you attempt to modify them directly, it can lead to unexpected behavior.
Debugging Tips for Vue Computed Properties
To effectively debug issues with computed properties in Vue, consider the following strategies:
- Use Vue DevTools: This browser extension allows you to inspect the component tree and observe real-time data changes, helping you identify issues with computed properties.
- Log Outputs: Add console logs within the computed property to track its execution and verify whether it is being called when expected.
- Check Dependencies: Ensure all variables and properties used in the computed property are reactive. If they are part of a data object, confirm that they are defined correctly.
Example of a Vue Computed Property
Here’s a simple example demonstrating how to define a computed property:
“`javascript
new Vue({
el: ‘app’,
data: {
firstName: ‘John’,
lastName: ‘Doe’
},
computed: {
fullName: function() {
return `${this.firstName} ${this.lastName}`;
}
}
});
“`
In this example, `fullName` is a computed property that concatenates `firstName` and `lastName`. If either of these values changes, `fullName` will automatically update.
Computed Property vs. Method
While both computed properties and methods can be used to derive data, their purposes and behaviors differ. Here’s a comparison:
Feature | Computed Property | Method |
---|---|---|
Reactivity | Cached until dependencies change | Re-evaluated every time called |
Use Case | Derived state | Functionality that needs execution |
Performance | More efficient for derived data | Less efficient for repeated calculations |
Understanding these differences can help you choose the right approach based on your application’s requirements.
Troubleshooting Vue Computed Properties with Switch Statements
When working with Vue.js, computed properties are often used to derive values based on reactive data. However, issues can arise, particularly when utilizing switch statements within these computed properties. Here are common pitfalls and their solutions.
Common Issues with Switch Statements in Computed Properties
- Incorrect Data Binding: Ensure the data used in the switch statement is correctly defined in the Vue instance’s data object. If the property is or not reactive, the computed property won’t work as expected.
- Switch Statement Syntax: Verify that the syntax of the switch statement is correct. A typical structure should look like this:
“`javascript
computed: {
computedValue() {
switch (this.someProperty) {
case ‘value1’:
return ‘Output for value1’;
case ‘value2’:
return ‘Output for value2’;
default:
return ‘Default output’;
}
}
}
“`
- Missing Return Values: Ensure that each case in the switch statement has a return value. If no return statement is executed, the computed property will return “.
Best Practices for Using Switch Statements
- Use Default Case: Always include a default case to handle unexpected values.
- Avoid Complex Logic: Keep the logic within switch statements simple. If complex logic is needed, consider extracting it to a method.
- Maintain Reactivity: Ensure that the values used in the switch statement are reactive. This means they should be defined in the `data` section of the Vue instance.
Example of a Working Computed Property with a Switch Statement
Here’s an example that illustrates a correctly implemented computed property using a switch statement:
“`javascript
new Vue({
el: ‘app’,
data: {
status: ‘active’
},
computed: {
statusMessage() {
switch (this.status) {
case ‘active’:
return ‘The status is active’;
case ‘inactive’:
return ‘The status is inactive’;
case ‘pending’:
return ‘The status is pending’;
default:
return ‘Unknown status’;
}
}
}
});
“`
Debugging Steps
To identify issues with computed properties, consider the following steps:
- Console Logging: Add console logs within the switch statement to monitor the flow and value of `this.someProperty`.
- Vue DevTools: Utilize Vue DevTools to inspect the component’s reactive data and computed properties to ensure they are updating as expected.
- Simplify the Computed Property: Temporarily simplify the computed property to isolate the issue. Remove the switch statement and check if basic returns work correctly.
- Reactivity Checks: If the property is derived from another computed property or a method, ensure that those are also reactive and returning the expected values.
By following these guidelines, you should be able to resolve issues related to using switch statements in Vue’s computed properties effectively.
Understanding Vue Computed Properties and Common Issues
Dr. Emily Chen (Senior Frontend Developer, Tech Innovations Inc.). “When dealing with computed properties in Vue, a common issue that arises is the misunderstanding of reactivity. Ensure that the properties you are trying to compute are reactive and correctly defined within the Vue instance. If the properties are not reactive, the computed property will not update as expected.”
Michael Torres (Vue.js Specialist, CodeMaster Academy). “If your computed switch is not functioning, check for any syntax errors or misconfigurations in your Vue component. Often, developers overlook the necessity of returning the correct value from the computed property, which can lead to unexpected behavior.”
Sarah Patel (Software Engineer and Vue.js Advocate, DevTalks). “Another frequent pitfall is the improper use of dependencies in computed properties. If your computed property relies on other data that is not properly tracked or updated, it will not react as intended. Always ensure that all dependencies are correctly referenced and reactive.”
Frequently Asked Questions (FAQs)
What is a computed property in Vue?
A computed property in Vue is a reactive property that derives its value from other data properties. It automatically recalculates when its dependencies change, allowing for efficient data manipulation and rendering.
Why might a computed property not work as expected?
A computed property may not work as expected due to issues such as incorrect dependencies, improper return values, or side effects within the computed function. Ensure that the computed property is returning a value and that all dependencies are properly referenced.
How can I debug a computed property that isn’t updating?
To debug a computed property, check the console for errors, verify that all dependencies are reactive, and use Vue DevTools to inspect the state of your component. Additionally, ensure that the computed property is being accessed in the template or another computed property.
What is the difference between computed properties and methods in Vue?
Computed properties are cached based on their dependencies and only re-evaluate when those dependencies change, while methods are executed every time they are called. Use computed properties for derived state and methods for actions or calculations that do not need caching.
Can I use a switch statement inside a computed property?
Yes, you can use a switch statement inside a computed property. Ensure that the switch statement is correctly structured and that it returns a value. If the computed property is not updating, check for any issues with the conditions or the return statements.
What should I do if my switch statement in a computed property is not functioning?
If the switch statement in a computed property is not functioning, verify the logic within the switch, ensure all cases are covered, and confirm that the computed property is being accessed correctly. Additionally, check for any reactivity issues with the data being evaluated.
In summary, the issue of a Vue computed property not functioning as expected often arises from a misunderstanding of how computed properties operate within the Vue framework. Computed properties are designed to be reactive and should automatically update when their dependencies change. If a computed property is not updating, it may be due to improper dependency tracking or incorrect implementation in the template or script sections of the Vue component.
Another common reason for computed properties failing to work is related to the use of non-reactive data. Vue relies on its reactivity system to detect changes in data. If the data being used in a computed property is not reactive, the computed property will not recalculate as intended. It is essential to ensure that all data used within computed properties is defined in the Vue instance’s data option or is a reactive property.
Additionally, developers should ensure that they are not inadvertently mutating the state within computed properties. Computed properties should be pure functions, meaning they should only compute values based on their dependencies without causing side effects. If mutations occur, it can lead to unexpected behavior and can disrupt the reactivity system.
troubleshooting a non-working Vue computed property involves checking for proper dependency tracking, ensuring data reactivity, and adhering
Author Profile
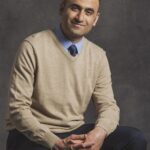
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?