How Can I Use waitForSelector in Puppeteer to Get All
Tags?
In the world of web automation and scraping, Puppeteer stands out as a powerful tool for developers looking to interact with web pages programmatically. One of its most useful features is the ability to wait for specific elements to load before performing actions, ensuring that your scripts run smoothly and efficiently. Among the myriad of elements you might want to target, paragraph tags (“) are ubiquitous in web content, making them a common focus for data extraction. But how do you effectively use Puppeteer to wait for these elements and gather them all at once?
In this article, we will explore the intricacies of using Puppeteer to wait for and retrieve all paragraph tags from a web page. We’ll delve into the `waitForSelector` method, which allows you to pause your script until the desired elements are present in the DOM. Understanding this functionality is crucial for anyone looking to scrape text, analyze content, or automate interactions with web applications.
As we navigate through the capabilities of Puppeteer, you’ll learn how to efficiently select and manipulate multiple paragraph tags, ensuring that your web scraping tasks yield accurate and comprehensive results. Whether you’re a seasoned developer or a newcomer to web automation, this guide will equip you with the knowledge to harness Puppeteer’s full potential in gathering textual data from the
Using `waitForSelector` in Puppeteer
The `waitForSelector` method in Puppeteer is essential for ensuring that your script only continues execution once a specified element is present in the DOM. This is particularly useful when dealing with dynamic web pages where elements may take time to load. When you want to retrieve all `
` tags from a page, you can utilize this method effectively.
To retrieve all `
` tags after ensuring they are loaded, you can follow these steps:
- Use `waitForSelector` to wait for at least one `
` tag to appear.
- Once the element is detected, use `page.$$eval` or `page.evaluate` to extract the text content of all `
` tags.
Here is an example snippet of code that illustrates this approach:
“`javascript
const puppeteer = require(‘puppeteer’);
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto(‘https://example.com’);
// Wait for at least one
tag to appear
await page.waitForSelector(‘p’);
// Get all
tags’ text content
const paragraphs = await page.$$eval(‘p’, ps => ps.map(p => p.textContent));
console.log(paragraphs);
await browser.close();
})();
“`
Understanding the Code
In the above code, several important components are at play:
- `puppeteer.launch()`: This initializes a new Puppeteer browser instance.
- `page.goto(url)`: This navigates to the desired URL.
- `waitForSelector(‘p’)`: This pauses the script until at least one `
` tag is present in the DOM.
- `$$eval(‘p’, …)`: This method retrieves all `
` elements and processes them to return their text content.
Best Practices
When using `waitForSelector`, consider the following best practices to enhance your Puppeteer scripts:
- Timeout Management: Set appropriate timeout values to avoid hanging indefinitely.
- Error Handling: Implement try-catch blocks to gracefully handle errors that may occur when elements are not found.
- Specificity: Use more specific selectors if necessary to avoid waiting for the wrong elements.
Example: Error Handling with `waitForSelector`
To improve reliability, you can add error handling as follows:
“`javascript
try {
await page.waitForSelector(‘p’, { timeout: 5000 }); // waits for 5 seconds
} catch (error) {
console.error(‘No
tags found within the timeout period.’);
}
“`
This example will log an error message if no `
` tags are found within the specified timeout, preventing your script from failing silently.
Method | Description |
---|---|
waitForSelector | Waits for an element to appear in the DOM. |
$$eval | Retrieves multiple elements and evaluates them in the page context. |
evaluate | Executes JavaScript code in the context of the page. |
By following these guidelines and examples, you can effectively use `waitForSelector` in Puppeteer to work with dynamic web content, ensuring that your scripts are robust and responsive to the loading state of the page.
Using Puppeteer to Wait for and Retrieve All `
` Tags
Puppeteer provides a powerful way to interact with web pages, enabling users to control headless Chrome or Chromium instances. When working with Puppeteer, you may need to wait for specific elements to load before you can manipulate or extract data from them. Below is a method to wait for all `
` tags on a page and retrieve their content.
Implementing `waitForSelector`
The `waitForSelector` function is essential for ensuring that your script pauses until the specified elements are present in the DOM. To retrieve all `
` tags, you can follow these steps:
- **Launch Puppeteer and Navigate to the Page**:
Begin by launching Puppeteer and navigating to the desired web page.
“`javascript
const puppeteer = require(‘puppeteer’);
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto(‘https://example.com’);
“`
- Use `waitForSelector`:
Call `waitForSelector` to ensure that the `
` tags are loaded.
“`javascript
await page.waitForSelector(‘p’, { visible: true });
“`
- Retrieve All `
` Tags:
Use `page.$$eval` to select all `
` tags and extract their text content.
“`javascript
const paragraphs = await page.$$eval(‘p’, ps => ps.map(p => p.textContent));
console.log(paragraphs);
“`
- Close the Browser:
After data extraction, properly close the browser instance.
“`javascript
await browser.close();
})();
“`
Complete Code Example
The following code snippet combines the steps mentioned above into a complete example:
“`javascript
const puppeteer = require(‘puppeteer’);
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto(‘https://example.com’);
await page.waitForSelector(‘p’, { visible: true });
const paragraphs = await page.$$eval(‘p’, ps => ps.map(p => p.textContent));
console.log(paragraphs);
await browser.close();
})();
“`
Considerations for Error Handling
While working with Puppeteer, it’s important to implement error handling to manage potential issues such as timeouts or missing elements. Consider the following:
– **Timeout Handling**: Use the `timeout` option in `waitForSelector` to specify how long to wait before throwing an error.
“`javascript
await page.waitForSelector(‘p’, { visible: true, timeout: 5000 });
“`
– **Try-Catch Blocks**: Wrap your code in a try-catch block to gracefully handle any errors.
“`javascript
try {
// your code here
} catch (error) {
console.error(‘Error:’, error);
}
“`
– **Check Element Existence**: Before attempting to extract data, verify that elements exist to avoid null references.
“`javascript
const exists = await page.$(‘p’);
if (exists) {
const paragraphs = await page.$$eval(‘p’, ps => ps.map(p => p.textContent));
console.log(paragraphs);
}
“`
Implementing these practices will enhance the robustness of your Puppeteer scripts when retrieving `
` tags from web pages.
Expert Insights on Using waitForSelector with Puppeteer to Retrieve All
Tags
Dr. Emily Carter (Senior Software Engineer, Web Automation Solutions). “When using Puppeteer, the waitForSelector method is crucial for ensuring that the DOM is fully loaded before attempting to retrieve elements. To get all
tags, it’s essential to use an appropriate selector like ‘p’ and ensure that the page has rendered completely.”
Mark Thompson (Lead Developer, Modern Web Technologies). “In Puppeteer, after invoking waitForSelector, developers can utilize the page.$$ method to fetch all
tags. This approach is efficient and avoids common pitfalls associated with asynchronous loading of content.”
Jessica Lin (Web Scraping Specialist, Data Insights Corp). “To effectively gather all
tags using Puppeteer, one should consider implementing a loop that waits for the elements to appear. This ensures that even dynamically loaded content is captured, providing a comprehensive dataset.”
Frequently Asked Questions (FAQs)
What is the purpose of using `waitForSelector` in Puppeteer?
`waitForSelector` is used to pause the execution of the script until a specific element appears in the DOM. This ensures that subsequent actions, such as clicking or extracting data, are performed only when the element is available.
How can I select all “ tags using Puppeteer?
To select all `
` tags, you can use the `page.$$eval` method combined with a selector. For example: `const paragraphs = await page.$$eval(‘p’, ps => ps.map(p => p.textContent));` This retrieves the text content of all `
` elements on the page.
Can I use `waitForSelector` to wait for multiple `
` tags?
Yes, you can use `waitForSelector` to wait for the presence of at least one `
` tag. For example: `await page.waitForSelector(‘p’);` However, if you need to ensure multiple `
` tags are present, you may need to check their count after waiting.
What happens if the selector does not match any elements?
If the selector does not match any elements within the specified timeout, `waitForSelector` will throw an error. You can handle this by using a try-catch block to manage the exception gracefully.
Is it possible to specify a timeout for `waitForSelector`?
Yes, you can specify a timeout by passing an options object to `waitForSelector`. For example: `await page.waitForSelector(‘p’, { timeout: 5000 });` This sets a timeout of 5000 milliseconds before throwing an error if the element is not found.
How do I handle dynamic content when waiting for `
` tags?
For dynamic content, it is advisable to use `waitForSelector` with a suitable timeout and possibly a polling interval. This allows the script to wait for the `
` tags to be rendered after any asynchronous operations, ensuring that you capture the content accurately.
Puppeteer is a powerful Node.js library that provides a high-level API for controlling headless Chrome or Chromium browsers. One of its essential features is the ability to wait for specific elements to appear on a page before interacting with them. The `waitForSelector` method plays a crucial role in this process, allowing developers to pause execution until a particular element, such as a `
` tag, is present in the DOM. This capability is vital for ensuring that scripts do not attempt to manipulate elements that are not yet available, which could lead to errors or unexpected behavior.
To retrieve all `
` tags from a web page using Puppeteer, one can combine the `waitForSelector` method with the appropriate DOM querying methods. After ensuring that the desired elements are present, developers can utilize methods like `page.$$eval` or `page.evaluate` to extract the content or attributes of all `
` tags efficiently. This approach not only enhances the reliability of web scraping tasks but also allows for the collection of structured data from web pages.
In summary, leveraging `waitForSelector` in Puppeteer is essential for robust web automation and scraping tasks. By ensuring that the script waits for elements to load, developers can avoid common pitfalls
Author Profile
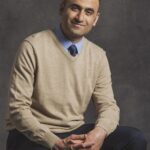
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?