How Can You Effectively Watch for Changes in Custom Resources?
In the ever-evolving landscape of cloud-native applications and Kubernetes, the ability to monitor and respond to changes in custom resources is paramount. As organizations increasingly rely on Kubernetes to manage their applications, understanding how to effectively watch for changes in custom resources becomes a critical skill for developers and operators alike. This capability not only enhances operational efficiency but also ensures that applications remain resilient and responsive to dynamic environments.
Watching for changes in custom resources involves leveraging Kubernetes’ powerful API and event-driven architecture to track modifications, additions, or deletions of resources that are tailored to specific application needs. By setting up efficient watchers, developers can create systems that react in real-time to changes, enabling automated workflows and improved resource management. This practice is essential for maintaining the integrity of applications and ensuring that they adapt seamlessly to the demands of users and the infrastructure.
As we delve deeper into the intricacies of monitoring custom resources, we will explore the various tools and techniques available for implementing effective change detection. From understanding the underlying mechanics of Kubernetes’ watch functionality to integrating with existing CI/CD pipelines, this article will equip you with the knowledge needed to harness the full potential of custom resources in your Kubernetes environment. Prepare to unlock new levels of agility and responsiveness in your application management strategies.
Monitoring Custom Resource Changes
To effectively watch for changes in a custom resource within a Kubernetes environment, it is essential to understand the mechanisms that enable real-time monitoring and the tools that can be utilized for this purpose. The primary method for monitoring changes in custom resources involves leveraging Kubernetes’ built-in capabilities, such as watches and event notifications.
Kubernetes provides a watch API that allows clients to subscribe to changes in resources. When a change occurs, clients receive an event that notifies them of the modification. This functionality is crucial for applications that need to react to state changes in real time.
Setting Up Watches
To set up a watch on a custom resource, the following steps are typically involved:
- Define the Custom Resource Definition (CRD): Ensure that your custom resource is properly defined in the Kubernetes cluster.
- Use the Kubernetes Client: Utilize a Kubernetes client library (e.g., client-go for Go, kubernetes-client for Java) to create a watch on the custom resource.
- Handle Events: Implement event handlers to process the notifications received when changes occur.
Here is a simple example of how to set up a watch using client-go in Go:
“`go
package main
import (
“context”
“fmt”
“time”
metav1 “k8s.io/apimachinery/pkg/apis/meta/v1”
“k8s.io/client-go/kubernetes”
“k8s.io/client-go/tools/clientcmd”
“k8s.io/client-go/util/retry”
)
func main() {
config, err := clientcmd.BuildConfigFromFlags(“”, “path/to/kubeconfig”)
if err != nil {
panic(err)
}
clientset, err := kubernetes.NewForConfig(config)
if err != nil {
panic(err)
}
watch, err := clientset.CustomResources().Watch(context.TODO(), metav1.ListOptions{})
if err != nil {
panic(err)
}
for event := range watch.ResultChan() {
fmt.Printf(“Event Type: %s, Object: %v\n”, event.Type, event.Object)
}
}
“`
Event Types
When monitoring custom resources, it is important to understand the different types of events that can be emitted:
- ADDED: Indicates that a new resource has been created.
- MODIFIED: Signifies that an existing resource has been updated.
- DELETED: Shows that a resource has been removed.
These event types can be handled specifically in your application logic to perform appropriate actions based on the resource’s state.
Considerations for Efficient Monitoring
When implementing watches on custom resources, consider the following best practices:
- Rate Limiting: Implement rate limiting to avoid overwhelming your application with too many events in a short period.
- Error Handling: Ensure robust error handling to gracefully manage failures in the watch stream.
- Resource Cleanup: Clean up resources and properly close watch connections to prevent resource leaks.
Event Type | Description | Action |
---|---|---|
ADDED | A new resource has been created | Initialize related resources |
MODIFIED | An existing resource has been updated | Update application state |
DELETED | A resource has been removed | Clean up resources |
By following these guidelines and utilizing the watch API effectively, you can create robust applications that respond to changes in custom resources within your Kubernetes environment efficiently.
Monitoring Changes in Custom Resources
To effectively watch for changes in custom resources, it is essential to understand the various methods available within Kubernetes and related tools. This allows developers and system administrators to respond promptly to modifications, ensuring that applications maintain their desired state.
Using Kubernetes Watch API
Kubernetes provides a built-in mechanism for monitoring changes through its Watch API. This API allows clients to subscribe to changes in resources, receiving notifications whenever an event occurs.
- Steps to Implement Watch API:
- Utilize the Kubernetes client libraries (e.g., Go, Python, Java).
- Establish a connection to the Kubernetes API server.
- Use the `/watch` endpoint for the specific custom resource.
- Listen for events such as `ADDED`, `MODIFIED`, and `DELETED`.
- Example Code Snippet (Go):
“`go
clientset := kubernetes.NewForConfigOrDie(config)
watchInterface, err := clientset.CustomResources(“v1”).Watch(context.TODO(), metav1.ListOptions{})
if err != nil {
log.Fatalf(“Error watching custom resources: %v”, err)
}
for event := range watchInterface.ResultChan() {
switch event.Type {
case watch.Added:
fmt.Println(“Resource added:”, event.Object)
case watch.Modified:
fmt.Println(“Resource modified:”, event.Object)
case watch.Deleted:
fmt.Println(“Resource deleted:”, event.Object)
}
}
“`
Leveraging Informers
Informers are a higher-level abstraction built on top of the Watch API. They provide a more efficient way to watch for changes by maintaining a local cache of resources and handling the logic for event processing.
- Advantages of Using Informers:
- Reduces the load on the API server by minimizing the number of requests.
- Automatically handles reconnections and errors.
- Simplifies event handling with built-in event handlers.
- Implementation Steps:
- Create a shared informer factory.
- Define an informer for the custom resource.
- Register event handlers for `Add`, `Update`, and `Delete` events.
- Example Code Snippet (Go):
“`go
factory := informers.NewSharedInformerFactory(clientset, time.Second*30)
informer := factory.CustomResources().V1().CustomResources().Informer()
informer.AddEventHandler(cache.ResourceEventHandlerFuncs{
AddFunc: func(obj interface{}) {
fmt.Println(“Resource added:”, obj)
},
UpdateFunc: func(oldObj, newObj interface{}) {
fmt.Println(“Resource updated:”, newObj)
},
DeleteFunc: func(obj interface{}) {
fmt.Println(“Resource deleted:”, obj)
},
})
stopCh := make(chan struct{})
defer close(stopCh)
factory.Start(stopCh)
“`
Using Custom Controllers
Custom controllers can be built to watch and manage the lifecycle of custom resources. These controllers can encapsulate business logic and ensure that the actual state of the system matches the desired state.
- Key Components:
- Reconcile Loop: Continuously checks the state of resources and takes action as needed.
- Event Handlers: Respond to changes in custom resources, invoking the reconcile logic.
- Implementation Overview:
- Create a controller that implements the Controller interface.
- Use informers to watch for changes in the custom resource.
- Define the reconcile logic to handle events appropriately.
- Example Pseudocode:
“`go
type CustomResourceController struct {
// dependencies and configurations
}
func (c *CustomResourceController) Reconcile() {
// logic to ensure desired state
}
func (c *CustomResourceController) Start() {
// set up watchers and event handlers
}
“`
Utilizing Third-Party Tools
Several third-party tools and frameworks can help monitor changes in custom resources effectively. Some popular options include:
Tool | Description |
---|---|
K8s Event Exporter | Exports Kubernetes events to various outputs for monitoring. |
Prometheus | Monitors metrics and can be configured to alert on resource changes. |
ArgoCD | Manages GitOps workflows and monitors the state of custom resources. |
By employing these methods and tools, organizations can ensure that they are well-equipped to monitor changes in custom resources, thereby maintaining operational integrity and responsiveness.
Monitoring Changes in Custom Resources: Expert Insights
Dr. Emily Chen (Senior Software Architect, Cloud Innovations Inc.). “In the realm of custom resources, it is imperative to implement robust monitoring solutions that can detect changes in real-time. Utilizing tools like Kubernetes’ built-in event system can significantly enhance your ability to respond to modifications proactively.”
James Patel (DevOps Specialist, Agile Systems). “To effectively watch for changes in custom resources, integrating automated workflows that trigger alerts upon resource modifications is crucial. This not only streamlines the response process but also minimizes the risk of human error during critical updates.”
Linda Martinez (Cloud Security Consultant, SecureTech Solutions). “Monitoring custom resources should also encompass security aspects. Implementing audit logging and change tracking mechanisms will ensure that any unauthorized modifications are promptly identified and addressed.”
Frequently Asked Questions (FAQs)
What is a custom resource in Kubernetes?
A custom resource in Kubernetes is an extension of the Kubernetes API that allows users to define their own resource types. This enables the creation of new object types that can be managed through Kubernetes, facilitating the customization of applications and services.
How can I watch for changes in a custom resource?
You can watch for changes in a custom resource by using the Kubernetes client libraries, such as client-go in Go or kubernetes-client in Python. These libraries provide methods to establish a watch on specific resources, allowing you to receive notifications for create, update, or delete events.
What are the benefits of watching for changes in custom resources?
Watching for changes in custom resources allows for real-time updates and automation within your Kubernetes environment. It enables efficient resource management, immediate response to state changes, and facilitates event-driven architectures.
Are there any performance considerations when watching custom resources?
Yes, performance considerations include the potential for increased load on the API server and network traffic. Establishing too many watches or not handling events efficiently can lead to degraded performance. It is essential to implement proper backoff strategies and limit the number of concurrent watches.
Can I filter events when watching for changes in custom resources?
Yes, you can filter events by specifying label selectors or field selectors when initiating a watch. This allows you to receive only the events that match your criteria, reducing unnecessary data processing and improving efficiency.
What happens if the watch connection is lost?
If the watch connection is lost, the client will receive a notification of the disconnection. The client should implement a reconnection strategy to re-establish the watch. Upon reconnection, the client can retrieve the current state of the resource to synchronize and continue monitoring changes.
In the realm of Kubernetes, monitoring changes in custom resources is crucial for maintaining the integrity and performance of applications. Custom resources extend the Kubernetes API, allowing users to define their own resource types tailored to specific needs. As these resources evolve, it is essential to implement effective strategies to watch for changes, ensuring that any modifications are promptly detected and managed. This vigilance helps in maintaining system stability and responsiveness to user requirements.
One of the most effective methods for monitoring changes in custom resources is through the use of Kubernetes controllers and operators. These components can watch for events related to custom resources, responding to create, update, and delete actions. By leveraging the Kubernetes watch API, developers can build robust systems that react to changes in real-time, thereby facilitating automated workflows and enhancing operational efficiency.
Another key takeaway is the importance of implementing proper logging and alerting mechanisms. By capturing detailed logs of changes to custom resources, teams can analyze trends and identify potential issues before they escalate. Additionally, setting up alerts for significant changes allows for immediate attention and intervention, which is vital for maintaining application performance and reliability.
watching for changes in custom resources is a fundamental practice in Kubernetes management. By utilizing controllers, operators, and effective logging
Author Profile
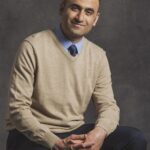
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?