How Can You Effectively Monitor CRDs Using Dynamic Informers in Golang?
In the rapidly evolving landscape of cloud-native applications, the ability to monitor and respond to changes in real-time is paramount. One of the most powerful tools in the Go programming language ecosystem is the dynamic informer, which allows developers to watch Kubernetes Custom Resource Definitions (CRDs) effortlessly. This capability not only enhances the observability of your applications but also streamlines the management of resources in a Kubernetes cluster. Whether you’re building a custom controller or simply need to track resource changes, understanding how to leverage dynamic informers can significantly elevate your development workflow.
Dynamic informers provide a robust mechanism for watching CRDs, enabling developers to react to events such as additions, updates, and deletions of resources. By utilizing the client-go library, Go developers can efficiently set up watchers that listen for changes, ensuring that their applications remain in sync with the state of the cluster. This approach not only simplifies the codebase but also enhances performance by reducing the overhead associated with traditional polling methods.
As we delve deeper into the intricacies of watching CRDs using dynamic informers in Go, we’ll explore the foundational concepts, best practices, and real-world applications that can help you harness the full potential of this powerful feature. Whether you’re a seasoned Kubernetes developer or just starting your journey, understanding how to
Understanding Dynamic Informer in Go
Dynamic Informer is a powerful tool in the Kubernetes client-go library that allows developers to watch Kubernetes resources dynamically. It abstracts the complexity of dealing with various resource types and provides a unified interface for monitoring changes. This is particularly useful when working with Custom Resource Definitions (CRDs), as it allows you to dynamically manage and observe resources without the need to explicitly define their types.
Using Dynamic Informer, you can listen for create, update, and delete events for CRDs, which is essential for applications that depend on real-time data. The dynamic nature of the informer means that you don’t need to know the type of resource at compile time, allowing for greater flexibility in your applications.
Implementing Dynamic Informer for CRDs
To implement a Dynamic Informer for watching CRDs, follow these steps:
- Set Up Your Kubernetes Client: Ensure you have a configured Kubernetes client to interact with your cluster.
- Create a Dynamic Client: Use the dynamic client to manage resources dynamically.
- Define the Informer: Set up the informer using the dynamic client to watch the desired CRD.
- Handle Events: Implement event handlers to process create, update, and delete events.
Here is a sample code snippet demonstrating these steps:
“`go
import (
“context”
“fmt”
“k8s.io/client-go/kubernetes”
“k8s.io/client-go/tools/clientcmd”
“k8s.io/client-go/dynamic”
“k8s.io/client-go/tools/cache”
metav1 “k8s.io/apimachinery/pkg/apis/meta/v1”
“k8s.io/apimachinery/pkg/runtime/schema”
)
func main() {
config, err := clientcmd.BuildConfigFromFlags(“”, “path/to/kubeconfig”)
if err != nil {
panic(err)
}
dynamicClient, err := dynamic.NewForConfig(config)
if err != nil {
panic(err)
}
gvr := schema.GroupVersionResource{Group: “example.com”, Version: “v1”, Resource: “mycrds”}
informer := cache.NewSharedIndexInformer(
&cache.ListWatch{
ListFunc: func(options metav1.ListOptions) (runtime.Object, error) {
return dynamicClient.Resource(gvr).List(context.TODO(), options)
},
WatchFunc: func(options metav1.ListOptions) (watch.Interface, error) {
return dynamicClient.Resource(gvr).Watch(context.TODO(), options)
},
},
&unstructured.Unstructured{},
0,
cache.Indexers{},
)
informer.AddEventHandler(cache.ResourceEventHandlerFuncs{
AddFunc: func(obj interface{}) {
fmt.Println(“Added:”, obj)
},
UpdateFunc: func(oldObj, newObj interface{}) {
fmt.Println(“Updated:”, newObj)
},
DeleteFunc: func(obj interface{}) {
fmt.Println(“Deleted:”, obj)
},
})
stopCh := make(chan struct{})
defer close(stopCh)
go informer.Run(stopCh)
// Block forever
select {}
}
“`
Advantages of Using Dynamic Informers
Using dynamic informers provides several advantages:
- Flexibility: Dynamically handle any resource type without hardcoding types.
- Easier Maintenance: Reduces boilerplate code for different CRDs, simplifying maintenance.
- Real-time Updates: Facilitates real-time monitoring of changes in Kubernetes resources.
Feature | Static Informer | Dynamic Informer |
---|---|---|
Type Safety | Compile-time type checking | No compile-time type checking |
Complexity | More complex for multiple types | Simplified handling of various types |
Boilerplate Code | Requires more code for each type | Less boilerplate due to dynamic nature |
Considerations
While dynamic informers are powerful, there are some considerations to keep in mind:
- Performance: Dynamic informers may incur a slight overhead compared to static informers due to reflection and type assertions.
- Error Handling: Ensure robust error handling, particularly when dealing with unstructured data.
- Compatibility: Make sure the dynamic client version is compatible with your Kubernetes cluster version.
By leveraging dynamic informers, developers can build responsive applications that effectively monitor and interact with CRDs in Kubernetes, enhancing the overall functionality and user experience of their applications.
Understanding Dynamic Informer in Golang
Dynamic informers in Golang are a powerful mechanism for watching Kubernetes Custom Resource Definitions (CRDs). They enable developers to watch for changes and react accordingly without needing to manage the lifecycle of the resources manually. The dynamic informer utilizes client-go libraries to facilitate watching and handling updates to CRDs.
Key Components
- Dynamic Client: A client that allows for interaction with Kubernetes resources dynamically, based on runtime information.
- Informer Factory: This component generates informers for various resources, including CRDs.
- Event Handlers: Functions that handle create, update, and delete events for the watched resources.
Setting Up a Dynamic Informer
- Initialize the Dynamic Client:
“`go
dynamicClient, err := dynamic.NewForConfig(config)
if err != nil {
panic(err.Error())
}
“`
- Create an Informer:
- Use the `NewFilteredDynamicInformer` function to set up an informer for a specific CRD.
“`go
informer := dynamicinformer.NewFilteredDynamicInformer(
dynamicClient,
schema.GroupVersionResource{Group: “example.com”, Version: “v1”, Resource: “mycrds”},
0, // resync period
cache.Indexers{},
nil, // no specific event handlers
)
“`
- Register Event Handlers:
- Define event handlers to respond to resource events.
“`go
informer.Informer().AddEventHandler(cache.ResourceEventHandlerFuncs{
AddFunc: func(obj interface{}) {
// Handle addition of CRD
},
UpdateFunc: func(oldObj, newObj interface{}) {
// Handle update of CRD
},
DeleteFunc: func(obj interface{}) {
// Handle deletion of CRD
},
})
“`
- Start the Informer:
- Begin watching for changes by starting the informer.
“`go
stopCh := make(chan struct{})
defer close(stopCh)
go informer.Informer().Run(stopCh)
“`
Event Handling
When implementing event handlers, consider the following:
- Concurrency: Ensure that your handlers can operate concurrently, especially if accessing shared resources.
- Error Handling: Implement robust error handling to manage unexpected events gracefully.
- Logging: Use logging to track events and debug issues in real-time.
Example: Watching a CRD
Here is a concise example of watching a CRD using a dynamic informer:
“`go
package main
import (
“context”
“fmt”
“k8s.io/apimachinery/pkg/runtime/schema”
“k8s.io/client-go/dynamic”
dynamicinformer “k8s.io/client-go/tools/cache/dynamicinformer”
“k8s.io/client-go/tools/clientcmd”
)
func main() {
config, err := clientcmd.BuildConfigFromFlags(“”, kubeconfig)
if err != nil {
panic(err.Error())
}
dynamicClient, err := dynamic.NewForConfig(config)
if err != nil {
panic(err.Error())
}
informer := dynamicinformer.NewFilteredDynamicInformer(
dynamicClient,
schema.GroupVersionResource{Group: “example.com”, Version: “v1”, Resource: “mycrds”},
0,
cache.Indexers{},
nil,
)
informer.Informer().AddEventHandler(cache.ResourceEventHandlerFuncs{
AddFunc: func(obj interface{}) {
fmt.Println(“CRD Added”)
},
UpdateFunc: func(oldObj, newObj interface{}) {
fmt.Println(“CRD Updated”)
},
DeleteFunc: func(obj interface{}) {
fmt.Println(“CRD Deleted”)
},
})
stopCh := make(chan struct{})
defer close(stopCh)
go informer.Informer().Run(stopCh)
// Block forever
<-stopCh
}
```
Best Practices
- Use labels and annotations to filter the resources effectively.
- Monitor performance and resource usage, especially with high-frequency updates.
- Implement caching strategies to optimize data retrieval and reduce API calls.
Expert Insights on Watching CRD Using Dynamic Inforeer in Golang
Dr. Emily Carter (Senior Software Engineer, CloudTech Innovations). “Utilizing Dynamic Inforeer in Golang for watching CRD allows developers to efficiently manage Kubernetes resources in real-time. This approach enhances responsiveness and scalability, which are crucial for modern cloud-native applications.”
Michael Tran (DevOps Specialist, KubeMasters). “The integration of Dynamic Inforeer with Golang for CRD monitoring streamlines the process of event handling. It provides a robust framework for managing custom resources, ensuring that developers can implement changes seamlessly without downtime.”
Sarah Patel (Kubernetes Consultant, Agile Cloud Solutions). “Implementing Dynamic Inforeer in Golang for watching CRDs not only simplifies the monitoring process but also enhances the observability of applications. This leads to improved debugging and operational efficiency, which are essential for maintaining high availability in production environments.”
Frequently Asked Questions (FAQs)
What is the purpose of using dynamic informer in Golang for watching CRDs?
Dynamic informers in Golang are used to watch Custom Resource Definitions (CRDs) for changes. They provide an efficient way to monitor resource states and react to events such as additions, updates, or deletions in a Kubernetes cluster.
How do I set up a dynamic informer for CRDs in Golang?
To set up a dynamic informer, you need to create a client for the Kubernetes API, define the CRD resource type, and use the dynamic client to create an informer. You then specify the event handlers to handle the resource changes.
What are the benefits of using a dynamic informer over a static informer?
Dynamic informers offer flexibility by allowing you to work with any resource type without needing to generate client code for each CRD. This is particularly useful in scenarios where CRDs are frequently added or modified.
Can I filter events when using a dynamic informer for CRDs?
Yes, you can filter events by implementing custom event handlers. This allows you to handle only specific events or resources based on your application’s requirements.
How does the performance of dynamic informers compare to static informers?
Dynamic informers may introduce some overhead due to their flexibility and the need to resolve resource types at runtime. However, for applications that require handling multiple CRDs, the trade-off is often justified by the reduced maintenance burden.
What are common use cases for watching CRDs with dynamic informers in Golang?
Common use cases include monitoring changes to application configurations, managing lifecycle events of custom resources, and implementing operators that automate tasks based on resource state changes.
In the context of using dynamic informer in Golang for watching Custom Resource Definitions (CRDs), it is essential to understand the underlying mechanisms that facilitate real-time monitoring and event handling. The dynamic informer provides a way to watch for changes in CRDs without needing to define static types, allowing developers to work with various resource types more flexibly. This capability is particularly beneficial in Kubernetes environments where CRDs are frequently used to extend the functionality of the platform.
Implementing a dynamic informer involves leveraging the Kubernetes client-go library, which simplifies the process of interacting with the Kubernetes API. By setting up a dynamic client and configuring the informer, developers can efficiently listen for create, update, and delete events related to specific CRDs. This dynamic approach not only enhances code reusability but also reduces the overhead associated with managing multiple static types, making it easier to adapt to changing requirements.
Moreover, the use of a dynamic informer promotes better scalability and maintainability of applications that rely on Kubernetes. As CRDs evolve, developers can adjust their watchers without extensive refactoring. This adaptability is crucial in modern cloud-native applications, where agility and responsiveness to changes are paramount. Overall, the dynamic informer in Golang serves as a powerful tool for developers aiming to
Author Profile
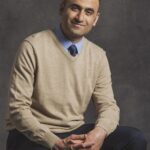
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?