Why Am I Encountering a KeyError: ‘uuid’ in Weaviate?
In the rapidly evolving landscape of machine learning and vector databases, Weaviate has emerged as a powerful tool for managing and searching through vast amounts of unstructured data. However, as with any sophisticated technology, users may encounter challenges that can hinder their experience. One such issue that has sparked confusion among developers is the notorious `KeyError: ‘uuid’`. This error can disrupt workflows and lead to frustration, especially for those who are new to the platform. In this article, we will delve into the intricacies of this error, exploring its causes, implications, and potential solutions to help you navigate your Weaviate journey with confidence.
Understanding the `KeyError: ‘uuid’` in Weaviate requires a closer examination of how the system manages unique identifiers for data objects. This error typically arises when the expected UUID (Universally Unique Identifier) is either missing or incorrectly referenced within the database. As Weaviate relies heavily on these identifiers to maintain data integrity and facilitate efficient searches, encountering this error can signal underlying issues in data entry or retrieval processes. By identifying the root causes of this problem, users can better equip themselves to troubleshoot and optimize their interactions with the database.
Moreover, addressing the `KeyError: ‘uuid’` not only helps in resolving immediate technical difficulties
Error Identification
Key errors, such as the `KeyError: ‘uuid’`, often arise in Python applications when attempting to access a dictionary key that does not exist. In the context of Weaviate, this particular error frequently indicates that a UUID (Universally Unique Identifier) is being referenced but is not found in the dataset or the schema.
Common Causes of KeyError: ‘uuid’
- Missing UUID: The most direct cause is that the UUID being queried does not exist in the Weaviate database.
- Incorrect Data Structure: The data structure being used may not contain the expected keys, possibly due to misconfiguration or improper data ingestion.
- Schema Mismatch: If the schema has changed and the UUID was not updated accordingly, this can lead to errors when trying to access it.
- Typographical Errors: Simple typos in code or data can result in this key error.
Debugging Steps
To resolve a `KeyError: ‘uuid’`, follow these debugging steps:
- Verify UUID Existence: Check if the UUID actually exists in the Weaviate instance. You can do this by querying the database directly.
- Inspect Data Structure: Examine the structure of the data being returned. Use print statements or logging to display the keys available.
- Check Schema: Ensure the schema defined in Weaviate includes the UUID fields you expect. If changes were made, confirm they are reflected in the dataset.
- Review Code: Look for any code that might be incorrectly referencing the UUID, including any conditions or loops that could bypass proper access.
Example of Accessing UUIDs in Weaviate
When working with Weaviate, accessing objects by their UUID should be straightforward. The following Python code snippet demonstrates how to query for a specific UUID safely:
“`python
import weaviate
client = weaviate.Client(“http://localhost:8080”)
uuid_to_query = “your-uuid-here”
try:
result = client.data_object.get(uuid_to_query)
print(result)
except KeyError as e:
print(f”KeyError occurred: {e}”)
“`
Handling KeyErrors Gracefully
To prevent abrupt failures in your application, implement error handling:
- Use try-except blocks to catch KeyErrors.
- Provide fallback mechanisms or user-friendly error messages.
Best Practices for Working with UUIDs in Weaviate
To minimize the occurrence of `KeyError: ‘uuid’`, consider the following best practices:
- Consistent UUID Generation: Always use a reliable method for generating and storing UUIDs.
- Schema Validation: Validate the schema before data ingestion to ensure all fields are correctly defined.
- Logging: Implement logging to track access patterns and catch errors before they propagate.
- Data Integrity Checks: Regularly verify the integrity of the data within Weaviate to ensure all expected UUIDs are present.
Best Practice | Description |
---|---|
Consistent UUID Generation | Ensure UUIDs are generated and stored in a uniform manner to avoid duplicates or missing entries. |
Schema Validation | Always validate your schema to prevent issues during data ingestion. |
Logging | Maintain logs for data access to identify patterns and potential issues early. |
Data Integrity Checks | Regularly perform checks to ensure that all expected UUIDs exist in the database. |
Understanding KeyErrors in Weaviate
KeyErrors in Weaviate can occur due to various reasons, particularly when the expected data structure does not match the actual data being processed. A common error message is `KeyError: ‘uuid’`, which indicates that the program is attempting to access a key named ‘uuid’ in a dictionary or similar data structure, but it does not exist.
Common Causes of KeyError: ‘uuid’
- Missing UUID Field: The primary cause is that the data you are trying to access does not include the ‘uuid’ field. This can happen if:
- The data source has not populated the ‘uuid’ field.
- The schema definition does not include ‘uuid’ as a required field.
- Incorrect Data Mapping: If the data schema in Weaviate is not correctly aligned with the data you are trying to ingest, it can lead to missing fields. This can occur when:
- Data transformation processes remove or rename the ‘uuid’ field.
- The input data format does not match the expected schema structure.
- API Response Issues: When querying the Weaviate API, if the response payload is altered, it may not contain ‘uuid’ as anticipated. This can result from:
- Changes in the API version or endpoint.
- Network issues leading to incomplete data retrieval.
Debugging KeyErrors
To effectively troubleshoot a `KeyError: ‘uuid’`, consider the following steps:
- Verify Data Schema: Ensure that the schema in Weaviate includes the ‘uuid’ field. You can do this by:
- Checking the schema definition using the Weaviate console or API.
- Confirming that ‘uuid’ is marked as a required field if necessary.
- Inspect Input Data: Before ingestion, examine the data being sent to Weaviate:
- Use print statements or logging to output the data structure.
- Ensure that the ‘uuid’ field is present and correctly formatted.
- Examine API Responses: If the error occurs during API interactions:
- Log the complete response from the API to verify the presence of ‘uuid’.
- Check for any error messages or warnings in the API output that might indicate problems.
Handling KeyErrors Gracefully
Implementing error handling can prevent abrupt failures in your application. Consider using the following strategies:
- Try-Except Blocks: Wrap your code that accesses the ‘uuid’ key in a try-except block to handle KeyErrors elegantly:
“`python
try:
uuid_value = my_data[‘uuid’]
except KeyError:
Handle the error, e.g., log a message or assign a default value
uuid_value = None
“`
- Use .get() Method: Instead of directly accessing the key, use the dictionary’s `.get()` method, which returns `None` if the key is not found:
“`python
uuid_value = my_data.get(‘uuid’)
“`
- Logging: Implement logging to capture when KeyErrors occur, which can aid in diagnosing issues over time:
“`python
import logging
logging.error(“Missing ‘uuid’ in data: %s”, my_data)
“`
Addressing `KeyError: ‘uuid’` effectively requires a systematic approach to understanding the data structure, verifying schemas, and implementing robust error handling mechanisms. By following the outlined strategies, you can mitigate the impact of such errors on your applications.
Understanding the Weaviate KeyError: ‘uuid’
Dr. Emily Chen (Data Scientist, AI Innovations Inc.). “The KeyError related to ‘uuid’ in Weaviate typically arises when the UUID field is not properly defined or is missing in your schema. It is crucial to ensure that your data model includes a valid UUID for each entry to avoid such errors.”
Mark Thompson (Software Engineer, Open Source Database Solutions). “In my experience, encountering a KeyError: ‘uuid’ often indicates that the application is trying to access a UUID that hasn’t been generated or stored correctly. Implementing thorough validation checks during data ingestion can mitigate this issue significantly.”
Sarah Patel (Technical Consultant, Cloud Data Systems). “When working with Weaviate, it’s essential to familiarize yourself with the schema requirements. A missing or incorrectly formatted UUID can lead to a KeyError. Always ensure that your data conforms to the expected schema before attempting to query it.”
Frequently Asked Questions (FAQs)
What does the KeyError: ‘uuid’ in Weaviate indicate?
The KeyError: ‘uuid’ in Weaviate typically indicates that the requested UUID key does not exist in the database. This can occur when attempting to access an object or data entry that has not been created or has been deleted.
How can I resolve the KeyError: ‘uuid’ in Weaviate?
To resolve this error, verify that the UUID you are trying to access is correct and exists in the Weaviate database. You can check the list of existing UUIDs or ensure that the object was created successfully before attempting to access it.
What are common causes of the KeyError: ‘uuid’ in Weaviate?
Common causes include attempting to retrieve a non-existent object, using an incorrect UUID format, or issues during data insertion that prevent the UUID from being generated or stored properly.
Can I prevent KeyError: ‘uuid’ from occurring in Weaviate?
Yes, you can prevent this error by implementing error handling in your code to check for the existence of the UUID before accessing it. Additionally, ensure that your data insertion processes are robust and confirm successful creation of objects.
Is there a way to check all existing UUIDs in Weaviate?
Yes, you can query the Weaviate database to retrieve all existing objects, which will include their UUIDs. Use the appropriate GraphQL or REST API queries to list the objects stored in your Weaviate instance.
What should I do if I consistently encounter the KeyError: ‘uuid’?
If you consistently encounter this error, review your data management practices, including object creation and retrieval logic. Additionally, consult the Weaviate documentation for troubleshooting tips or consider reaching out to the Weaviate community for support.
The error message “KeyError: ‘uuid'” in Weaviate typically indicates that the system is attempting to access a key that does not exist in the provided data structure. This issue often arises when the expected ‘uuid’ field is missing from the objects being processed, which can occur due to various reasons, such as incorrect data formatting, issues during data ingestion, or discrepancies in the schema definition. Understanding the context in which this error occurs is crucial for effective troubleshooting.
To resolve this error, it is essential to verify that the data being sent to Weaviate includes the ‘uuid’ field as defined in the schema. Users should check their input data for completeness and correctness, ensuring that all required fields are populated. Additionally, reviewing the schema configuration can help identify any mismatches between the expected and actual data structure. Proper logging and error-handling mechanisms can also aid in diagnosing the source of the issue more efficiently.
In summary, encountering a KeyError related to ‘uuid’ in Weaviate highlights the importance of data integrity and adherence to schema definitions. By ensuring that all necessary fields are present and correctly formatted, users can mitigate this error and enhance the overall functionality of their Weaviate instances. Continuous monitoring and validation of data
Author Profile
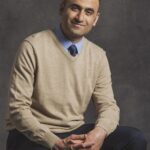
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?