How Can You Exclude Test Files in Your Webpack Build Using Esbuild?
In the ever-evolving landscape of web development, efficient build processes are crucial for delivering high-performance applications. As developers increasingly turn to modern tools like Webpack and esbuild, understanding how to optimize these tools becomes essential. One common challenge developers face is managing their build configurations to exclude unnecessary files, particularly test files, which can bloat the final output and slow down the build process. If you’re looking to streamline your workflow and ensure that your production builds are as lean as possible, mastering the art of excluding test files in Webpack while leveraging esbuild can be a game changer.
When working with Webpack, the flexibility it offers can sometimes lead to complexity, especially when it comes to file inclusion and exclusion. Test files, while critical during development, are often not needed in production environments. By learning how to configure Webpack effectively, you can prevent these files from being bundled, thus enhancing build speed and reducing the size of your application. Meanwhile, esbuild, known for its lightning-fast performance, provides a unique approach to handling file exclusions that can complement your Webpack setup.
In this article, we will explore the best practices for excluding test files from your Webpack builds, while also integrating esbuild’s capabilities. Whether you’re a seasoned developer or just starting out, understanding
Excluding Test Files in Webpack Builds
To efficiently manage your codebase in a Webpack build, it’s crucial to exclude unnecessary test files from the production bundle. This not only optimizes the build process but also reduces the final bundle size. You can achieve this by configuring your Webpack setup to ignore specific file patterns, typically those designated for testing.
One effective method is to use the `exclude` property within your module rules. Here’s an example of how to set it up in your `webpack.config.js`:
“`javascript
module.exports = {
module: {
rules: [
{
test: /\.js$/,
exclude: /__tests__|\.test\.js$/, // Exclude all test files
use: ‘babel-loader’,
},
],
},
};
“`
This configuration specifies that any JavaScript files located in a `__tests__` directory or files that end with `.test.js` will not be processed by Babel, keeping them out of the final bundle.
Using Esbuild to Exclude Test Files
When using Esbuild, excluding test files can be accomplished in a similar manner, though the configuration differs slightly due to Esbuild’s command-line interface or API usage. You can specify the files to include or exclude directly in your build command.
Here’s an example of how to exclude test files using Esbuild in a command line:
“`bash
esbuild src/index.js –bundle –outdir=dist –external:__tests__ –external:*.test.js
“`
Alternatively, when using Esbuild in a JavaScript configuration file, you can specify the `entryPoints` and filter out test files:
“`javascript
const esbuild = require(‘esbuild’);
esbuild.build({
entryPoints: [‘src/index.js’],
outdir: ‘dist’,
entryPoints: fs.readdirSync(‘src’)
.filter(file => !/\.test\.js$/.test(file)) // Exclude test files
.map(file => `src/${file}`),
}).catch(() => process.exit(1));
“`
Comparison of Exclusion Methods
The following table illustrates the key differences between excluding test files in Webpack and Esbuild:
Feature | Webpack | Esbuild |
---|---|---|
Configuration File | webpack.config.js | JavaScript/CLI |
Exclusion Method | Using `exclude` in module rules | Using `–external` or filtering in entry points |
Pattern Matching | Regex based | Regex or file system methods |
By understanding these methods and configurations, you can effectively manage your Webpack and Esbuild builds, ensuring that only the necessary files are included in your production output.
Excluding Test Files in Webpack Builds with ESBuild
To exclude test files from your Webpack build process when using ESBuild as a loader, you can configure your Webpack setup accordingly. This typically involves modifying the Webpack configuration file to ensure that test files are not included in the final bundle.
Configuration Steps
- Install Required Packages
Ensure you have the necessary packages installed:
“`bash
npm install –save-dev webpack webpack-cli esbuild-loader
“`
- Modify Webpack Configuration
Update your `webpack.config.js` file to exclude test files. You can achieve this by using a regular expression in the `exclude` option of your module rules.
Here’s an example configuration:
“`javascript
const path = require(‘path’);
module.exports = {
entry: ‘./src/index.js’,
output: {
filename: ‘bundle.js’,
path: path.resolve(__dirname, ‘dist’),
},
module: {
rules: [
{
test: /\.js$/,
exclude: /(__tests__|\.test\.js$)/, // Exclude test files
use: {
loader: ‘esbuild-loader’,
options: {
loader: ‘js’, // Set the loader type
},
},
},
],
},
resolve: {
extensions: [‘.js’],
},
};
“`
In this example, the `exclude` field uses a regular expression to exclude any files in the `__tests__` directory or any files ending with `.test.js`.
Alternative Exclusion Methods
You can also use other methods to exclude test files:
- Using the `include` Option
Specify the exact directories or files to include. This can be useful if you want to include specific files while excluding others.
“`javascript
{
test: /\.js$/,
include: path.resolve(__dirname, ‘src’), // Only include source files
use: ‘esbuild-loader’,
}
“`
- Using Environment Variables
Set an environment variable to control which files to include or exclude during the build process.
“`javascript
const isTest = process.env.NODE_ENV === ‘test’;
{
test: /\.js$/,
exclude: isTest ? /.*\.js$/ : /__tests__/, // Conditionally exclude based on environment
use: ‘esbuild-loader’,
}
“`
Testing the Configuration
After updating your Webpack configuration, run your build process to ensure that test files are correctly excluded. Use the following command:
“`bash
npx webpack –config webpack.config.js
“`
Verify the output directory (`dist` in the example) to confirm that test files are not included in the final bundle. You can also check the console output for any warnings or errors related to excluded files.
Further Considerations
When configuring your Webpack build:
- Maintain Consistency: Ensure that your configuration aligns with other parts of your build setup, such as Babel or TypeScript configurations.
- Documentation Reference: For more detailed options, refer to the official Webpack and ESBuild documentation.
By following these guidelines, you can effectively exclude test files from your Webpack builds while utilizing ESBuild for efficient bundling.
Strategies for Excluding Test Files in Webpack Builds with Esbuild
Jordan Lee (Senior Frontend Developer, CodeCraft Solutions). “To effectively exclude test files from your Webpack build when using Esbuild, consider utilizing the `exclude` option in your Webpack configuration. This allows you to specify patterns that match your test files, ensuring they are not included in the final build output.”
Maria Gonzalez (Build Tools Specialist, DevOps Insights). “A practical approach is to leverage the `ignore` plugin in Esbuild. By configuring this plugin within your Webpack setup, you can easily filter out test directories or specific file types, streamlining your build process and reducing unnecessary overhead.”
Kevin Tran (Software Architect, Modern Web Technologies). “For projects that heavily utilize testing frameworks, it is advisable to maintain a clear separation of test files from production code. Implementing a naming convention for test files, such as suffixing them with `.test.js`, can simplify the exclusion process in your Webpack configuration.”
Frequently Asked Questions (FAQs)
How can I exclude test files from a Webpack build using esbuild?
You can exclude test files by configuring the `exclude` option in your Webpack configuration. Specify a glob pattern that matches your test files, such as `exclude: /.*\.test\.js$/`.
Is it possible to exclude specific directories containing test files in Webpack?
Yes, you can exclude entire directories by using the `exclude` option in your Webpack configuration. For instance, you can use `exclude: /tests/` to exclude all files within a directory named `tests`.
What is the role of the `esbuild-loader` in excluding test files?
The `esbuild-loader` allows you to integrate esbuild with Webpack. You can configure it to exclude test files by using the `exclude` property within the loader’s options, ensuring that those files are not processed.
Can I use environment variables to control the exclusion of test files in Webpack?
Yes, you can use environment variables to dynamically set the exclusion of test files. In your Webpack configuration, check for an environment variable and adjust the `exclude` option accordingly.
How do I verify that test files are excluded from the Webpack build?
You can verify the exclusion by checking the output directory for the absence of test files after running the build. Additionally, you can enable verbose logging in Webpack to see which files are being processed.
Are there any performance benefits to excluding test files from the Webpack build?
Excluding test files can significantly improve build performance, as it reduces the number of files Webpack needs to process, leading to faster compilation times and reduced bundle size.
In the context of using webpack and esbuild for JavaScript applications, excluding test files from the build process is a common requirement to optimize performance and reduce unnecessary bundle size. Both tools offer mechanisms to filter out specific files or directories, allowing developers to focus on the production-ready code. This is particularly important in larger projects where test files can clutter the output and increase build times.
To exclude test files when using webpack, developers can utilize the `exclude` property in their module rules or configure the `ignore` plugin. This approach ensures that any files matching the specified patterns, such as files located in a `__tests__` directory or those ending with `.test.js`, are not included in the final build. On the other hand, esbuild provides a more straightforward method by using the `–ignore` flag during the build process, allowing for quick exclusions without extensive configuration.
In summary, effectively excluding test files from webpack and esbuild builds enhances the efficiency of the build process and results in cleaner output. By leveraging the appropriate configuration options in each tool, developers can streamline their workflow and focus on delivering high-quality production code without the overhead of test files. Understanding these configurations is essential for maintaining optimal build performance in any JavaScript project
Author Profile
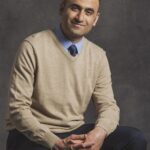
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?