What Are Literals in Python? Understanding the Basics of Python Programming
In the world of programming, every language has its own set of rules and conventions that define how we interact with data. Among these foundational elements, literals stand out as the building blocks of code, representing fixed values that we can manipulate and utilize in various ways. In Python, a language renowned for its simplicity and readability, literals play a crucial role in making code intuitive and expressive. Whether you’re a novice coder or a seasoned developer, understanding literals is essential for mastering Python and unlocking its full potential.
Literals in Python are essentially the raw data values that you can use directly in your code. They come in various forms, including numbers, strings, and booleans, each serving a distinct purpose. By defining these values explicitly, programmers can create variables, perform calculations, and control the flow of their applications with ease. The beauty of literals lies in their straightforwardness; they allow you to convey complex ideas in a simple, unambiguous manner.
As you delve deeper into the concept of literals, you’ll discover how they interact with Python’s dynamic typing system and how they can be utilized in different contexts. From numeric operations to string manipulations, literals are omnipresent in Python programming, forming the backbone of data representation. Join us as we explore the various types
Understanding Literals in Python
Literals in Python are the fixed values that are directly represented in the code. They are used to denote data without requiring any computation or evaluation. Literals can be classified into several categories, each serving a distinct purpose in programming.
Types of Literals
Python supports various types of literals, including:
- String Literals: Represent sequences of characters. They can be defined using single quotes, double quotes, or triple quotes for multi-line strings.
Examples:
“`python
single_quote = ‘Hello’
double_quote = “World”
multi_line = “””This is a
multi-line string.”””
“`
- Numeric Literals: Represent numbers and can be classified into:
- Integer Literals: Whole numbers without a decimal point.
- Floating Point Literals: Numbers with a decimal point.
- Complex Literals: Numbers that have a real and an imaginary part.
Examples:
“`python
integer_literal = 42
float_literal = 3.14
complex_literal = 1 + 2j
“`
- Boolean Literals: Represent the truth values, either `True` or “.
Example:
“`python
is_active = True
is_complete =
“`
- None Literal: Represents the absence of a value or a null value. It is denoted by `None`.
Example:
“`python
no_value = None
“`
Literal Representation in Python
Literals are represented in code as direct values. The following table summarizes the different types of literals and their representations:
Type of Literal | Example |
---|---|
String | ‘Hello’, “World”, “””Multi-line””” |
Integer | 42 |
Float | 3.14 |
Complex | 1 + 2j |
Boolean | True, |
None | None |
Usage of Literals
Literals are fundamental in defining data within your Python programs. They are used extensively for:
- Initializing variables
- Passing values to functions
- Configuring settings in applications
Understanding and utilizing literals effectively can enhance code readability and maintainability.
Understanding Literals in Python
Literals in Python are fixed values that are represented directly in the code. They can be classified into several categories based on their types, such as strings, numbers, booleans, and more. Each type of literal serves a specific purpose in programming, allowing developers to convey data and instructions effectively.
Types of Literals
The main types of literals in Python include:
- String Literals: Used to represent text data.
- Can be enclosed in single quotes (`’…’`) or double quotes (`”…”`).
- Multi-line strings can be created using triple quotes (`”’…”’` or `”””…”””`).
- Numeric Literals: Represent numbers, which can be further categorized.
- Integer Literals: Whole numbers, e.g., `10`, `-5`.
- Floating-point Literals: Decimal numbers, e.g., `3.14`, `-0.001`.
- Complex Literals: Numbers with a real and imaginary part, e.g., `2 + 3j`.
- Boolean Literals: Represent truth values.
- Can take one of two values: `True` or “.
- Special Literals:
- None: Represents the absence of a value or a null value, denoted as `None`.
Examples of Each Literal Type
The following table provides examples of different types of literals:
Literal Type | Example | Description |
---|---|---|
String Literal | `’Hello, World!’` | A simple string enclosed in quotes |
Integer Literal | `42` | An integer value |
Floating-point Literal | `3.14159` | A decimal number |
Complex Literal | `1 + 2j` | A complex number |
Boolean Literal | `True` | A boolean value |
Special Literal | `None` | Represents a null value |
Using Literals in Python
Literals are fundamental to writing Python code, as they provide the means to define values directly. Here are some practical uses of literals:
– **Variable Assignment**: Assigning literals to variables for later use.
“`python
message = “Hello, World!”
count = 5
pi = 3.14159
is_active = True
“`
– **Function Parameters**: Passing literals as arguments to functions.
“`python
print(“Welcome to Python!”)
“`
– **List and Dictionary Initialization**: Creating collections with literals.
“`python
numbers = [1, 2, 3, 4, 5]
user_info = {“name”: “Alice”, “age”: 30}
“`
– **Control Flow**: Utilizing literals in conditional statements.
“`python
if count > 0:
print(“Count is positive.”)
“`
Literals are inherently immutable, meaning their values cannot be changed once defined. Understanding how to use literals effectively allows for clearer and more efficient code.
Understanding Literals in Python: Perspectives from Experts
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Literals in Python are fundamental building blocks that represent fixed values within the code. They can be of various types, including strings, integers, floats, and booleans, each serving a distinct purpose in programming.”
Michael Chen (Lead Python Developer, CodeCrafters). “Understanding literals is crucial for any Python programmer. They allow for the direct representation of data, making code more readable and maintainable. For instance, using string literals for user input enhances clarity in the code.”
Sarah Thompson (Python Educator, LearnPythonNow). “In Python, literals play a significant role in defining constants. They provide a way to express values directly in the code without the need for variables, which can simplify the logic and improve performance.”
Frequently Asked Questions (FAQs)
What are literals in Python?
Literals in Python are fixed values that are directly represented in the code. They can be of various data types, such as integers, floats, strings, and booleans.
What types of literals are available in Python?
Python supports several types of literals, including integer literals (e.g., 10), floating-point literals (e.g., 10.5), string literals (e.g., “Hello”), boolean literals (e.g., True, ), and special literals like None.
How do string literals work in Python?
String literals in Python can be defined using single quotes (‘ ‘), double quotes (” “), triple single quotes (”’ ”’), or triple double quotes (“”” “””). Triple quotes allow for multi-line strings.
Can literals be assigned to variables in Python?
Yes, literals can be assigned to variables in Python. For example, `x = 10` assigns the integer literal 10 to the variable x.
Are there any special considerations for using literals in Python?
When using literals, ensure that the data type matches the intended use. For example, using a string literal where an integer is expected may lead to type errors.
What is the difference between literals and variables in Python?
Literals are fixed values that do not change, while variables are symbolic names that reference data stored in memory. Variables can hold different values over time, whereas literals remain constant.
Literals in Python are fixed values that are explicitly defined in the code. They represent data directly and can take various forms, including numeric literals, string literals, boolean literals, and special literals like None. Each type of literal serves a specific purpose and is integral to the language’s syntax, allowing developers to express data in a straightforward manner.
Numeric literals can be integers or floating-point numbers, enabling arithmetic operations and calculations. String literals, enclosed in quotes, are essential for handling text data. Boolean literals, which are either True or , are crucial for making logical decisions in programming. Additionally, the None literal signifies the absence of a value, playing a vital role in functions and variable assignments.
Understanding the different types of literals is fundamental for effective programming in Python. They form the building blocks of data manipulation and control flow within the language. By mastering literals, developers can write clearer, more efficient code and leverage Python’s capabilities to their fullest potential.
Author Profile
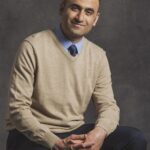
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?