What Are Logical Operators in Python and How Do They Work?
In the realm of programming, the ability to make decisions is paramount, and this is where logical operators come into play. Python, one of the most popular programming languages today, offers a robust set of logical operators that empower developers to create complex conditions and control the flow of their code. Whether you’re building a simple script or a sophisticated application, understanding how to leverage these operators is essential for writing efficient and effective code.
Logical operators in Python—namely `and`, `or`, and `not`—serve as the backbone of decision-making processes in your programs. They allow you to combine multiple boolean expressions, enabling you to evaluate conditions in a nuanced way. For instance, you can determine whether a certain condition is true based on multiple criteria or invert a boolean value to control the execution of specific code blocks. This functionality not only enhances the clarity of your code but also increases its power and flexibility.
As you delve deeper into the world of Python programming, mastering logical operators will significantly enhance your ability to implement complex logic and algorithms. By understanding how to utilize these operators effectively, you will be better equipped to tackle a wide range of programming challenges, making your code more robust and adaptable to various scenarios. So, let’s explore the fascinating world of logical operators in Python and unlock the
Understanding Logical Operators in Python
Logical operators in Python are essential for performing logical operations on Boolean values, which are either `True` or “. These operators allow for the construction of complex conditional statements and play a crucial role in control flow and decision-making in programming.
The three primary logical operators in Python are:
- and: This operator returns `True` if both operands are `True`. If either operand is “, the result is “.
- or: This operator returns `True` if at least one of the operands is `True`. The result is “ only if both operands are “.
- not: This operator negates the value of the operand. If the operand is `True`, it returns “, and if the operand is “, it returns `True`.
Here is a truth table that illustrates the behavior of these logical operators:
Expression | Result |
---|---|
True and True | True |
True and | |
and | |
True or True | True |
True or | True |
or | |
not True | |
not | True |
Using these logical operators allows for the creation of complex conditional statements. For instance, consider the following example:
“`python
a = 10
b = 20
c = 30
if a < b and b < c:
print("Both conditions are true.")
if a < b or b > c:
print(“At least one condition is true.”)
if not (a > b):
print(“The condition is .”)
“`
In this example, the `and` operator checks if both conditions are satisfied, the `or` operator checks if at least one condition is satisfied, and the `not` operator negates the result of the comparison.
Logical operators are fundamental for building more complex logical expressions and can be combined to form intricate conditions as necessary in programming tasks.
Logical Operators in Python
Logical operators in Python are used to combine conditional statements. These operators return Boolean values—either `True` or “—based on the evaluation of the expressions involved. The three primary logical operators are `and`, `or`, and `not`.
Types of Logical Operators
Operator | Description | Example |
---|---|---|
and |
Returns `True` if both operands are true. | True and True yields True |
or |
Returns `True` if at least one operand is true. | True or yields True |
not |
Returns `True` if the operand is , and “ if the operand is true. | not True yields
|
Usage of Logical Operators
Logical operators are often used in control flow statements such as `if` statements, loops, and while evaluating multiple conditions. Here’s how each operator can be applied:
Using `and` Operator
When using the `and` operator, both conditions must be satisfied for the overall expression to return `True`.
“`python
age = 20
is_student = True
if age >= 18 and is_student:
print(“Eligible for student discount.”)
“`
Using `or` Operator
The `or` operator allows for flexibility; if at least one condition is true, the overall expression is true.
“`python
age = 16
is_student =
if age < 18 or is_student: print("Eligible for youth discount.") ```
Using `not` Operator
The `not` operator negates the boolean value of the condition it precedes.
“`python
is_logged_in =
if not is_logged_in:
print(“Please log in to continue.”)
“`
Short-Circuit Evaluation
Python employs short-circuit evaluation with logical operators, meaning:
- In an expression using `and`, if the first operand evaluates to “, the second operand is not evaluated because the overall result cannot be `True`.
- In an expression using `or`, if the first operand evaluates to `True`, the second operand is not evaluated, as the overall result is already `True`.
This behavior can improve performance and prevent unnecessary computations or function calls.
Understanding logical operators in Python is crucial for controlling flow and making decisions in code. Their proper application can lead to more efficient and readable code.
Understanding Logical Operators in Python: Expert Insights
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “Logical operators in Python, such as ‘and’, ‘or’, and ‘not’, are fundamental for controlling the flow of logic in programming. They allow developers to combine conditional statements, making it possible to execute code based on multiple criteria.”
Mark Thompson (Lead Python Developer, CodeCrafters). “In Python, logical operators play a crucial role in decision-making processes. Understanding how to effectively utilize these operators can significantly enhance the readability and efficiency of your code.”
Sarah Patel (Computer Science Educator, Future Coders Academy). “Teaching logical operators is essential for beginners in Python. These operators not only help in building complex conditions but also encourage critical thinking about how different conditions interact with each other.”
Frequently Asked Questions (FAQs)
What are logical operators in Python?
Logical operators in Python are used to combine conditional statements. They evaluate expressions and return Boolean values, either `True` or “. The primary logical operators are `and`, `or`, and `not`.
How does the `and` operator work in Python?
The `and` operator returns `True` if both operands are true; otherwise, it returns “. For example, `True and ` evaluates to “, while `True and True` evaluates to `True`.
What is the function of the `or` operator in Python?
The `or` operator returns `True` if at least one of the operands is true. If both operands are , it returns “. For instance, ` or True` evaluates to `True`.
How does the `not` operator function in Python?
The `not` operator negates the Boolean value of its operand. If the operand is `True`, `not` returns “, and if the operand is “, `not` returns `True`.
Can logical operators be used with non-Boolean values in Python?
Yes, logical operators can be applied to non-Boolean values in Python. Non-Boolean values are evaluated based on their truthiness; for instance, non-empty strings and non-zero numbers are considered `True`, while empty strings and zero are considered “.
What is short-circuit evaluation in Python regarding logical operators?
Short-circuit evaluation is a feature where Python stops evaluating expressions as soon as the result is determined. For example, in an `and` operation, if the first operand is “, Python does not evaluate the second operand, as the entire expression will be “.
Logical operators in Python are fundamental tools used to perform logical operations on boolean values. The primary logical operators include `and`, `or`, and `not`. These operators allow developers to combine multiple conditions and evaluate them in a way that facilitates decision-making in code. Understanding how to effectively utilize these operators is crucial for writing efficient and functional programs.
The `and` operator returns `True` only if both operands are true, while the `or` operator returns `True` if at least one operand is true. Conversely, the `not` operator negates the boolean value of its operand, returning `True` if the operand is and vice versa. This logical structure enables complex conditional statements, which are essential for controlling the flow of programs.
Key takeaways include the importance of logical operators in constructing conditional statements, which are vital for implementing control flow in programming. Mastery of these operators enhances a programmer’s ability to create robust and dynamic applications. Additionally, understanding operator precedence is critical, as it determines the order in which expressions are evaluated, thereby influencing the outcome of logical operations.
Author Profile
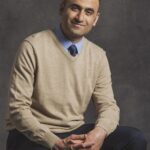
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?