What Are Nesting Blocks in Python and How Do They Work?
### Introduction
In the world of programming, the ability to organize and structure code effectively is paramount for creating efficient and maintainable applications. Among the myriad of tools and techniques available to developers, nesting blocks in Python stands out as a powerful concept that enhances code readability and functionality. Whether you’re a novice programmer or a seasoned developer, understanding how to utilize nesting blocks can significantly elevate your coding skills and streamline your workflow. In this article, we will delve into the intricacies of nesting blocks in Python, exploring their purpose, benefits, and practical applications.
Nesting blocks refer to the practice of placing one block of code within another, creating a hierarchy that allows for more complex and organized programming constructs. This technique is often employed in control structures, such as loops and conditional statements, where the need for clarity and logical flow is essential. By nesting blocks, developers can encapsulate functionality and manage scope more effectively, leading to cleaner and more efficient code.
As we navigate the nuances of nesting blocks in Python, we’ll uncover how this approach not only simplifies the coding process but also enhances collaboration among developers. With a focus on best practices and real-world examples, this article aims to equip you with the knowledge to harness the full potential of nesting blocks, paving the way for more robust and scalable Python applications
Nesting Blocks in Python
Nesting blocks in Python refers to the practice of placing one block of code inside another. This is a fundamental concept in Python, as it governs how the structure and flow of the code are organized. A block is defined by indentation and can include control structures such as loops, conditionals, and function definitions.
The primary benefit of nesting blocks is to create more complex control flows while maintaining code readability. However, excessive nesting can lead to code that is difficult to read and maintain. It’s essential to balance the depth of nesting with clarity.
Types of Nesting
There are several types of nesting in Python, including:
- Nested Functions: Functions defined within other functions. This allows for encapsulation and can help manage scope effectively.
- Nested Loops: A loop that runs inside another loop, useful for iterating over multi-dimensional data structures such as lists or arrays.
- Nested Conditionals: Conditionals placed inside other conditionals, enabling more complex decision-making processes.
Examples of Nesting
Here are some examples demonstrating different types of nesting:
Nested Functions
python
def outer_function():
def inner_function():
return “Hello from the inner function!”
return inner_function()
Nested Loops
python
for i in range(3):
for j in range(2):
print(f”i: {i}, j: {j}”)
Nested Conditionals
python
x = 10
if x > 5:
if x < 15:
print("x is between 5 and 15")
Best Practices for Nesting
To maintain code quality while using nested blocks, consider the following best practices:
- Limit Nesting Depth: Aim for a maximum of three levels of nesting to avoid complexity.
- Use Meaningful Names: Clearly name functions and variables to indicate their purpose, which aids in readability.
- Refactor When Necessary: If a block of code becomes too nested, consider refactoring it into smaller functions.
Performance Considerations
While nesting is a powerful feature, it can impact performance, particularly with deeply nested structures. The following table summarizes the performance implications of various types of nesting:
Nesting Type | Performance Impact | Use Case |
---|---|---|
Nested Functions | Low | Encapsulation and scope management |
Nested Loops | Medium to High | Iterating through multi-dimensional data |
Nested Conditionals | Low | Complex decision-making |
By adhering to these guidelines and understanding the types of nesting, developers can write efficient and maintainable Python code.
Nesting Blocks in Python
Nesting blocks in Python refers to the practice of placing one block of code inside another, which is a common feature in many programming languages that support structured programming. In Python, this is primarily achieved through the use of indentation, which defines the scope of loops, functions, classes, and conditional statements.
Understanding Code Blocks
A code block is a section of code that is grouped together. This can include:
- Functions
- Loops (for, while)
- Conditional statements (if, else, elif)
- Classes
In Python, code blocks are defined by their indentation level. Each block of code that is nested inside another must have a greater level of indentation.
Nesting Examples
Here are a few examples that illustrate how nesting works in Python:
**Nested If Statements**
python
x = 10
if x > 5:
print(“x is greater than 5”)
if x > 8:
print(“x is also greater than 8”)
In this example, the second `if` statement is nested within the first. The inner block will only execute if the outer condition is true.
Nested Loops
python
for i in range(3):
for j in range(2):
print(f”i: {i}, j: {j}”)
This code features a nested loop where the inner loop executes completely for each iteration of the outer loop.
Use Cases for Nesting Blocks
Nesting blocks can be useful in various scenarios, including:
- Complex Conditions: Handling multiple conditional statements where certain conditions rely on the outcome of others.
- Multi-dimensional Data Structures: Iterating over lists of lists, such as matrices or grids.
- Organizing Logic: Structuring code in a way that reflects logical hierarchies, making it easier to read and maintain.
Best Practices for Nesting Blocks
To maintain code readability and avoid confusion, consider the following best practices:
- Limit Nesting Depth: Keep nesting levels shallow to avoid complex and difficult-to-read code.
- Use Functions: Break down complex nested blocks into functions. This improves modularity and enhances readability.
- Consistent Indentation: Stick to a consistent indentation style (typically 4 spaces) to clearly delineate block levels.
Common Pitfalls of Nesting
While nesting is a powerful tool, it can lead to certain issues if not handled correctly:
- Readability: Deeply nested structures can become hard to follow.
- Logic Errors: Misplaced indentation can lead to unintended behavior or logic errors.
- Performance: Excessive nesting may impact performance in terms of complexity, especially with loops.
Nesting Blocks
Nesting blocks in Python is an essential concept that allows developers to write structured and organized code. Understanding how to effectively use nesting can lead to better coding practices and improved software design.
Understanding Nesting Blocks in Python: Perspectives from Experts
Dr. Emily Carter (Senior Software Engineer, Python Development Institute). “Nesting blocks in Python refer to the practice of placing one block of code inside another, such as defining functions within functions or using loops inside conditionals. This structure enhances code organization and readability, allowing developers to encapsulate logic effectively.”
Michael Thompson (Lead Python Instructor, Code Academy). “The concept of nesting blocks is crucial for mastering Python. It allows for more complex data structures and control flows, enabling programmers to manage scope and variable accessibility. Understanding how to properly nest blocks is essential for writing efficient and maintainable code.”
Sarah Johnson (Software Architect, Tech Innovations Inc.). “In Python, nesting blocks can lead to cleaner code through the use of indentation to define scope. However, developers must be cautious of excessive nesting, which can lead to code that is difficult to read and maintain. Striking a balance is key to leveraging this powerful feature.”
Frequently Asked Questions (FAQs)
What are nesting blocks in Python?
Nesting blocks in Python refer to the practice of placing one block of code inside another, such as using loops or conditionals within functions or other loops. This structure allows for complex logic and organization within the code.
Why are nesting blocks important in Python?
Nesting blocks are important because they enable developers to create more complex and organized code. They facilitate the implementation of hierarchical logic, allowing for better control flow and functionality in programs.
How does indentation relate to nesting blocks in Python?
Indentation is crucial in Python as it defines the scope of nesting blocks. Proper indentation indicates which statements belong to which block, thereby ensuring the correct execution of the code.
Can nesting blocks lead to performance issues in Python?
Excessive nesting can lead to performance issues, such as increased time complexity and reduced readability. It is advisable to keep nesting levels manageable to maintain code efficiency and clarity.
What are some best practices for using nesting blocks in Python?
Best practices include limiting the depth of nesting to enhance readability, using descriptive names for functions and variables, and refactoring complex nested structures into separate functions when necessary.
Are there any limitations to nesting blocks in Python?
While Python allows for deep nesting, there is a practical limit imposed by readability and maintainability. Python’s interpreter also raises an error if the maximum recursion depth is exceeded, which can occur with excessive nesting in recursive functions.
Nesting blocks in Python refer to the practice of organizing code structures, such as functions, classes, and control flow statements, within one another. This technique allows developers to create more complex and modular programs by encapsulating functionality and maintaining a clear hierarchy. Nesting enhances code readability and maintainability, as it logically groups related operations and allows for better scoping of variables and functions.
One of the key advantages of nesting blocks is the ability to define local scopes. Variables defined within a nested block are not accessible outside of it, which helps prevent name collisions and unintended side effects. This encapsulation is particularly beneficial in larger codebases, where managing variable lifetimes and scopes becomes increasingly important. Additionally, nesting can facilitate the implementation of closures, where inner functions can capture and remember the environment in which they were created.
Another important aspect of nesting blocks is its role in improving code organization. By structuring code into nested blocks, developers can create a clear and logical flow that mirrors the problem domain. This practice not only aids in debugging but also enhances collaboration among teams, as the code becomes easier to understand and modify. Overall, nesting blocks is a fundamental concept in Python that contributes significantly to writing clean, efficient, and maintainable code.
Author Profile
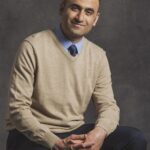
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?