Exploring the Possibilities: What Can Be Traversed via a Loop in Python?
Introduction
In the world of Python programming, loops serve as one of the fundamental building blocks that empower developers to write efficient and elegant code. Whether you’re processing data, automating tasks, or creating complex algorithms, understanding what can be traversed via a loop is crucial for unlocking the full potential of this versatile language. From simple lists to intricate data structures, the ability to iterate over elements allows for dynamic and responsive applications that can handle a variety of tasks with ease. In this article, we will explore the myriad of objects that can be traversed using loops in Python, shedding light on their characteristics and how they can enhance your coding experience.
When we talk about traversable objects in Python, we’re referring to any iterable that can be looped over to access its elements. This includes built-in data types such as lists, tuples, and dictionaries, each offering unique ways to store and manipulate data. Additionally, Python supports custom objects that can be made iterable through the implementation of specific methods, providing a pathway for developers to create their own loopable structures tailored to specific needs. Understanding the nuances of these iterables is essential for writing clean and efficient code.
Moreover, the versatility of Python’s looping constructs extends beyond traditional data types. With the advent of generator functions and
Data Structures That Can Be Traversed via a Loop
In Python, several data structures can be traversed using loops, allowing for efficient iteration and manipulation of data. The most commonly traversed structures include lists, tuples, dictionaries, sets, and strings. Each of these structures can be utilized with various loop constructs, such as `for` loops and `while` loops.
Lists and Tuples
Lists and tuples are both ordered collections of items that can be traversed using loops. Lists are mutable, meaning their contents can be changed, while tuples are immutable. Traversing these data structures is straightforward:
- Lists: Use a `for` loop to iterate through each element.
- Tuples: Similar to lists, you can use a `for` loop for traversal.
Example of traversing a list:
python
my_list = [1, 2, 3, 4]
for item in my_list:
print(item)
Example of traversing a tuple:
python
my_tuple = (1, 2, 3, 4)
for item in my_tuple:
print(item)
Dictionaries
Dictionaries in Python are unordered collections of key-value pairs. You can traverse a dictionary to access its keys, values, or both.
- Keys: Iterate through dictionary keys using `for key in my_dict`.
- Values: Use `for value in my_dict.values()` to access values.
- Key-Value Pairs: Use `for key, value in my_dict.items()` to access both.
Example of traversing a dictionary:
python
my_dict = {‘a’: 1, ‘b’: 2, ‘c’: 3}
for key, value in my_dict.items():
print(f”{key}: {value}”)
Sets
Sets are unordered collections of unique elements. They can be traversed in a similar manner to lists and dictionaries. Since sets are unordered, the order of traversal may vary.
Example of traversing a set:
python
my_set = {1, 2, 3, 4}
for item in my_set:
print(item)
Strings
Strings in Python are sequences of characters and can also be traversed using loops. Each character in the string can be accessed individually.
Example of traversing a string:
python
my_string = “Hello”
for char in my_string:
print(char)
Comparison of Data Structures
Below is a comparison table of various data structures that can be traversed in Python:
Data Structure | Ordered | Mutable | Example of Traversal |
---|---|---|---|
List | Yes | Yes | for item in my_list |
Tuple | Yes | No | for item in my_tuple |
Dictionary | No | Yes | for key, value in my_dict.items() |
Set | No | Yes | for item in my_set |
String | Yes | No | for char in my_string |
Traversing these data structures efficiently is crucial for data manipulation and analysis in Python programming. Understanding the properties of each allows developers to choose the appropriate structure based on their specific needs.
Data Structures That Can Be Traversed via a Loop in Python
In Python, several built-in data structures can be traversed using loops. These include:
- Lists
- Tuples
- Sets
- Dictionaries
- Strings
Each of these data structures has unique characteristics that influence how they can be traversed.
Traversing Lists and Tuples
Lists and tuples are ordered collections that can contain elements of varying data types. They can be traversed using a `for` loop as follows:
python
my_list = [1, 2, 3, 4]
for item in my_list:
print(item)
- Lists: Mutable, allowing modification of elements.
- Tuples: Immutable, preventing modification after creation.
Traversing Sets
Sets are unordered collections of unique elements. Traversing a set is similar to traversing a list, but the order of elements is not guaranteed.
python
my_set = {1, 2, 3, 4}
for item in my_set:
print(item)
- Characteristics: Useful for membership testing and eliminating duplicate entries.
Traversing Dictionaries
Dictionaries are key-value pairs, and they can be traversed in several ways:
- Keys: To access keys in a dictionary.
- Values: To access values.
- Items: To access both keys and values.
Example of traversing keys:
python
my_dict = {‘a’: 1, ‘b’: 2}
for key in my_dict:
print(key)
Example of traversing values:
python
for value in my_dict.values():
print(value)
Example of traversing items:
python
for key, value in my_dict.items():
print(key, value)
Traversing Strings
Strings in Python can also be traversed character by character. This is particularly useful for tasks like validation or transformation of string data.
python
my_string = “Hello”
for char in my_string:
print(char)
- Characteristics: Strings are immutable, so they cannot be modified during traversal.
Advanced Traversal Techniques
Python supports various advanced techniques for traversing data structures:
- List Comprehensions: A concise way to create lists based on existing lists.
python
squares = [x**2 for x in range(10)]
- Generator Expressions: Similar to list comprehensions but yield items one at a time.
python
squares_gen = (x**2 for x in range(10))
for square in squares_gen:
print(square)
- Enumerate: Provides a counter alongside the elements of a list or tuple.
python
my_list = [‘a’, ‘b’, ‘c’]
for index, value in enumerate(my_list):
print(index, value)
The ability to traverse various data structures using loops is fundamental in Python. Understanding these structures and their traversal techniques enhances programming efficiency and effectiveness.
Exploring Loop Traversals in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “In Python, loops can traverse various data structures, including lists, tuples, sets, and dictionaries. Each of these structures allows for efficient iteration, enabling developers to manipulate and access data seamlessly.”
Michael Chen (Data Scientist, AI Solutions Group). “When working with loops in Python, one can traverse not only standard collections like lists and dictionaries but also user-defined objects. This flexibility is crucial for data analysis and machine learning tasks, where custom data structures are often employed.”
Sarah Thompson (Software Engineer, CodeCraft Labs). “Python’s loop constructs, such as for and while loops, allow for traversal of iterable objects. This includes strings, which can be iterated character by character, making Python particularly powerful for text processing and manipulation.”
Frequently Asked Questions (FAQs)
What data structures can be traversed via a loop in Python?
Lists, tuples, dictionaries, sets, and strings are common data structures that can be traversed using loops in Python. Each of these structures allows iteration through their elements using constructs like `for` and `while` loops.
How do you traverse a list using a loop in Python?
You can traverse a list using a `for` loop by iterating over each element. For example:
python
for item in my_list:
print(item)
Can you traverse a dictionary in Python? If so, how?
Yes, you can traverse a dictionary using a loop. You can iterate over keys, values, or key-value pairs. For example:
python
for key, value in my_dict.items():
print(key, value)
What is the difference between traversing a list and a set in Python?
The primary difference is that lists maintain order and allow duplicate elements, while sets are unordered collections that do not allow duplicates. Traversal methods are similar, using `for` loops.
Is it possible to traverse a string in Python? How?
Yes, strings can be traversed using a loop. Each character in the string can be accessed sequentially. For example:
python
for char in my_string:
print(char)
Can you traverse a nested data structure in Python?
Yes, nested data structures, such as lists within lists or dictionaries within dictionaries, can be traversed using nested loops. For example:
python
for sublist in my_nested_list:
for item in sublist:
print(item)
In Python, various data structures can be traversed using loops, with the most common being lists, tuples, dictionaries, sets, and strings. Each of these data types allows for iteration, enabling developers to access and manipulate their elements efficiently. For instance, lists and tuples can be traversed using a simple for loop, while dictionaries require a slightly different approach, often iterating over keys, values, or key-value pairs. Sets, being unordered collections, can also be looped through, providing unique elements without duplication.
Additionally, Python supports nested loops, which allow for the traversal of multi-dimensional data structures, such as lists of lists. This capability is particularly useful in scenarios where data is organized in a matrix format, enabling developers to access each element systematically. Furthermore, Python’s built-in functions, such as `enumerate()` and `zip()`, enhance loop functionality by providing index-based access and simultaneous iteration over multiple sequences, respectively.
Key takeaways from the discussion include the versatility of Python loops in handling various data types and the importance of understanding the specific characteristics of each data structure when traversing them. Mastery of looping constructs is crucial for effective data manipulation and algorithm implementation in Python. By leveraging the power of loops,
Author Profile
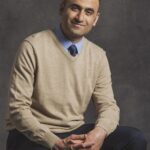
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?