What Does ‘Mean’ in JavaScript Really Mean?
### Introduction
JavaScript, the backbone of modern web development, is a language that thrives on its versatility and dynamism. Among its many features, the concept of “mean” often emerges in discussions about data manipulation and analysis. Whether you’re a seasoned developer or a curious newcomer, understanding what “mean” signifies in JavaScript can unlock a deeper comprehension of how to handle numerical data effectively. This article delves into the nuances of calculating the mean, its applications, and the various methods available to achieve it within the JavaScript ecosystem.
### Overview
At its core, the mean is a statistical measure that represents the average of a set of numbers. In JavaScript, calculating the mean involves summing a collection of values and dividing that sum by the total number of values. This fundamental concept is crucial for tasks ranging from data analysis to performance metrics, making it an essential skill for any developer working with numerical datasets.
In JavaScript, there are multiple ways to compute the mean, each suited to different scenarios and data structures. From simple arrays to more complex data types, understanding how to implement mean calculations can enhance your programming toolkit. As we explore this topic further, you’ll discover practical examples and techniques that can be seamlessly integrated into your JavaScript projects, empowering you to manipulate and analyze
Understanding the `mean` Function in JavaScript
The term `mean` in JavaScript typically refers to the calculation of the average of a set of numbers. While JavaScript does not have a built-in `mean` function, it can be implemented easily using basic array and mathematical operations. The mean, or average, is computed by summing all the elements in a collection and then dividing by the number of elements.
To calculate the mean in JavaScript, you can follow these steps:
- **Sum all elements in the array.**
- **Divide the total sum by the number of elements.**
Here’s a simple implementation of a `mean` function in JavaScript:
javascript
function calculateMean(numbers) {
const total = numbers.reduce((acc, val) => acc + val, 0);
return total / numbers.length;
}
### Example Usage
javascript
const data = [10, 20, 30, 40, 50];
const average = calculateMean(data);
console.log(average); // Output: 30
Performance Considerations
When calculating the mean for large datasets, it is essential to consider performance. The above method uses the `reduce` function which iterates through the array, making it efficient for most use cases. However, when handling extremely large arrays, memory management and performance optimization become crucial.
#### Performance Tips
- Avoid unnecessary computations: Only compute the mean when necessary.
- Use typed arrays: For very large datasets, consider using `TypedArray` for better performance.
- Parallel processing: If working in a web environment, consider Web Workers for offloading computation.
Mean vs. Other Statistical Measures
While the mean is a commonly used measure of central tendency, it’s important to understand how it compares to other statistical measures such as median and mode:
Measure | Description | Use Case |
---|---|---|
Mean | The average of all numbers. | Best for normally distributed data. |
Median | The middle value when data is sorted. | Best for skewed distributions. |
Mode | The most frequently occurring value. | Useful for categorical data. |
Understanding these differences can help in choosing the right measure based on the data characteristics and the analysis requirements.
Mean Calculation
Calculating the mean in JavaScript is straightforward but must be done with consideration for the data set’s characteristics and size. Implementing efficient methods and understanding the context of the data will lead to better performance and more accurate analysis.
Understanding `mean` in JavaScript
The term `mean` in the context of JavaScript typically refers to the statistical average of a set of numbers. While JavaScript does not have a built-in method specifically named `mean`, you can easily calculate the mean using various approaches. The mean is calculated by summing all the values and dividing by the count of those values.
Calculating the Mean
To compute the mean of an array of numbers in JavaScript, you can follow these steps:
- **Sum all elements** of the array.
- **Count the number of elements** in the array.
- **Divide** the total sum by the count.
Here is a simple function that implements this logic:
javascript
function calculateMean(numbers) {
const total = numbers.reduce((accumulator, currentValue) => accumulator + currentValue, 0);
return total / numbers.length;
}
// Example usage:
const data = [10, 20, 30, 40, 50];
console.log(calculateMean(data)); // Output: 30
Detailed Breakdown of the Function
Component | Description |
---|---|
`reduce` | Method that executes a reducer function on each element of the array, resulting in a single output value. |
`accumulator` | The accumulated value previously returned in the last invocation of the callback, or the initial value if supplied. |
`currentValue` | The current element being processed in the array. |
`total / numbers.length` | Division that calculates the mean. |
Handling Edge Cases
When calculating the mean, it is important to handle potential edge cases:
– **Empty array**: Dividing by zero should be avoided. You can return `null` or `undefined` if the array is empty.
– **Non-numeric values**: Filter out non-numeric values to avoid errors in calculations.
Here is an updated function that includes these considerations:
javascript
function calculateMean(numbers) {
if (numbers.length === 0) return null; // Handle empty array
const validNumbers = numbers.filter(num => typeof num === ‘number’); // Filter non-numeric values
const total = validNumbers.reduce((acc, curr) => acc + curr, 0);
return total / validNumbers.length;
}
// Example usage:
const mixedData = [10, “20”, 30, null, 40, 50];
console.log(calculateMean(mixedData)); // Output: 30
Performance Considerations
When calculating the mean for large datasets, consider the following:
- Efficiency of `reduce`: The `reduce` method performs in O(n) time complexity, which is efficient for summing numbers in an array.
- Memory Usage: Filtering and creating new arrays can increase memory usage. For large datasets, optimize the function to avoid unnecessary allocations.
The concept of calculating the mean in JavaScript is straightforward. By using array methods like `reduce` and `filter`, you can efficiently compute the mean while handling various edge cases. This approach is widely applicable in data analysis, statistics, and any scenario requiring numerical computations.
Understanding the Role of `mean` in JavaScript
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In JavaScript, the concept of `mean` typically refers to the arithmetic mean, which is a fundamental statistical measure. It is crucial for developers to understand how to calculate this value, especially when working with datasets, to derive meaningful insights from their applications.”
Michael Chen (Data Scientist, Analytics Hub). “When we talk about `mean` in JavaScript, it often involves using functions to process arrays of numbers. Understanding how to implement this correctly ensures that developers can accurately analyze data trends and make informed decisions based on statistical evidence.”
Sarah Thompson (JavaScript Framework Specialist, CodeCraft Academy). “The `mean` function is not built into JavaScript by default, but creating a custom function to calculate it is a common practice. This showcases the flexibility of JavaScript and highlights the importance of mastering basic programming concepts for effective data manipulation.”
Frequently Asked Questions (FAQs)
What do mean in JavaScript?
The `mean` function is not a built-in JavaScript function. However, it typically refers to calculating the average of a set of numbers. This can be achieved by summing the numbers and dividing by the count of the numbers.
How can I calculate the mean of an array in JavaScript?
To calculate the mean of an array, use the `reduce` method to sum the elements and then divide by the length of the array. For example:
javascript
const numbers = [1, 2, 3, 4, 5];
const mean = numbers.reduce((a, b) => a + b, 0) / numbers.length;
Is there a built-in method for calculating the mean in JavaScript?
JavaScript does not have a built-in method specifically for calculating the mean. Developers typically implement this functionality using custom functions or by leveraging array methods like `reduce`.
What is the difference between mean, median, and mode?
The mean is the average of a set of numbers, calculated by dividing the sum of the numbers by their count. The median is the middle value when the numbers are sorted, and the mode is the value that appears most frequently in the dataset.
Can I use libraries to calculate the mean in JavaScript?
Yes, libraries like Lodash and Underscore provide utility functions that can simplify the calculation of the mean and other statistical measures. For instance, Lodash has a `_.mean` function that directly computes the mean of an array.
What are common use cases for calculating the mean in JavaScript?
Common use cases include statistical analysis, data visualization, performance metrics, and any scenario where summarizing numerical data is necessary for decision-making or reporting.
In JavaScript, the keyword “mean” does not have a specific, predefined function or purpose within the language itself. However, it can be interpreted in various contexts, particularly when discussing statistical calculations or data analysis. In programming, “mean” typically refers to the average value of a set of numbers, which can be computed using JavaScript functions or methods. Understanding how to calculate the mean is essential for developers who work with data manipulation and analysis.
To calculate the mean in JavaScript, one can create a function that sums up all the elements in an array and divides the total by the number of elements. This basic operation underscores the importance of arrays and iteration in JavaScript, as they are fundamental to handling collections of data. Additionally, utilizing built-in array methods such as `reduce()` can streamline the process, making code more efficient and readable.
In summary, while “mean” is not a keyword in JavaScript, it represents a common mathematical concept that can be implemented through code. Developers should be familiar with how to compute the mean as part of their toolkit for data processing. Mastering such calculations enhances one’s ability to analyze data effectively and contributes to more robust programming practices.
Author Profile
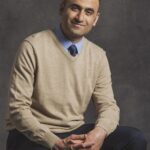
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?